JAVA Web学习记录(三)
一.Servlet Response
1.下载文件
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.FileInputStream;
import java.io.IOException;
import java.net.URLEncoder;
public class DownloadImgServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String realPath = this.getServletContext().getRealPath("WEB-INF/classes/bg.jpg");
String fileName = realPath.substring(realPath.lastIndexOf("")+1);//获得文件名
//URLEncoder.encode(fileName,"utf-8"); 防止文件名是中文,然后乱码
resp.setHeader("Content-Disposition","attachment;filename="+ URLEncoder.encode(fileName,"utf-8"));
FileInputStream fis = new FileInputStream(realPath);
int len = 0;
byte[] buffer = new byte[1024];
ServletOutputStream sos = resp.getOutputStream();
while ((len = fis.read(buffer))>0)
{
sos.write(buffer,0,len);
}
sos.close();
fis.close();
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
2.生成验证码
import javax.imageio.ImageIO;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.IOException;
import java.util.Random;
public class ImageVCodeServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//设置浏览器3秒自动刷新一次
resp.setHeader("refresh","3");
BufferedImage image = new BufferedImage(80,20,BufferedImage.TYPE_INT_RGB);
Graphics2D g = (Graphics2D) image.getGraphics();
//绘制白底
g.setColor(Color.white);
g.fillRect(0,0,80,20);
//绘制验证码
g.setColor(Color.blue);
g.setFont(new Font(null,Font.BOLD,20));
g.drawString(makeRandNum(),0,20);
//响应类型
resp.setContentType("image/jpeg");
//不让浏览器缓存
resp.setDateHeader("expires",-1);
resp.setHeader("Cache-Control","no-cache");
resp.setHeader("Pragma","no-cache");
ImageIO.write(image,"jpeg",resp.getOutputStream());
}
//生成6位随机数
private String makeRandNum() {
Random random = new Random();
String randNum = random.nextInt(9999999)+"";
StringBuffer sb = new StringBuffer();
for (int i = 0;i < 7 - randNum.length();i++)
{
sb.append("0");
}
sb.append(randNum);
System.out.println(sb);
return sb.toString();
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
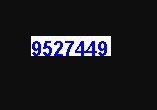
3.页面重定向(HttpServletResponse)
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%>
<html>
<body>
<%--${pageContext.request.contextPath} 代表当前的项目--%>
<form action="${pageContext.request.contextPath}/request" method="get">
用户名:<input type="text" name="username"/>
密码:<input type="password" name="password"/>
<input type="submit" />
</form>
</body>
</html>
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class RequestServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String username = req.getParameter("username");
String password = req.getParameter("password");
System.out.println("username="+username+",password="+password);
resp.sendRedirect(this.getServletContext().getContextPath()+"/success.jsp");
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
重点:
页面重定向: resp.sendRedirect(this.getServletContext().getContextPath()+"/success.jsp");
在Servlet中获取项目当前目录:this.getServletContext().getContextPath()
在Jsp中获取项目当前目录:${pageContext.request.contextPath}
jsp中解决中文乱码:<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%>
4.登陆并内部跳转(HttpServletResponse)
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%>
<html>
<body>
<form action="${pageContext.request.contextPath}/login" method="post">
用户名:<input type="text" name="username" />
密码:<input type="password" name="password"/>
爱好:<input type="checkbox" name="hobby" value="游戏"/>
<input type="checkbox" name="hobby" value="代码"/>
<input type="checkbox" name="hobby" value="羽毛球"/>
<input type="submit" value="提交"/>
</form>
</body>
</html>
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.Arrays;
public class LoginServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
req.setCharacterEncoding("utf-8");
resp.setCharacterEncoding("utf-8");
String username = req.getParameter("username");
String password = req.getParameter("password");
String[] hobbies = req.getParameterValues("hobby");
System.out.println(username);
System.out.println(password);
System.out.println(Arrays.toString(hobbies));
//请求转发,因为是内部转发所以不需要项目的路径 /代表当前web项目
req.getRequestDispatcher("/success.jsp").forward(req,resp);
// resp.sendRedirect(req.getServletContext().getContextPath()+"/success.jsp");
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
重点:内部跳转不需要项目路径,/代表当前web项目
请求过来的中文信息乱码:req.setCharacterEncoding("utf-8");
获取多选框信息: String[] hobbies = req.getParameterValues("hobby");
二.Cookie
1.记录访问时间
import javax.servlet.ServletException;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Date;
public class LastLoginTimeServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
resp.setContentType("text/html;");
resp.setCharacterEncoding("utf-8");
Cookie[] cookies = req.getCookies();
PrintWriter out = resp.getWriter();
out.print("你上一次访问的时间是:");
if (cookies!=null)
{
for (int i = 0; i < cookies.length; i++) {
Cookie cookie = cookies[i];
String name = cookie.getName();
if (name.equals("lastLoginTime"))
{
long lastLoginTime = Long.parseLong(cookie.getValue());
Date date= new Date(lastLoginTime);
out.print(date.toLocaleString());
}
}
}
Cookie cookie = new Cookie("lastLoginTime",System.currentTimeMillis()+"");
//cookie 有效期为1天
cookie.setMaxAge(24*60*60);
resp.addCookie(cookie);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}

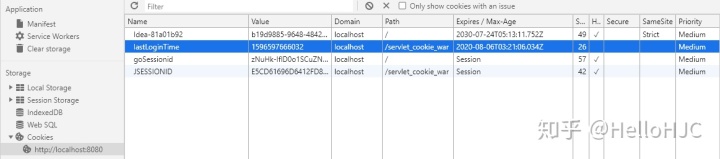
一个网站cookie是否存在上限!聊聊细节问题!
一个Cookie只能保存一个信息
一个web站点可以给浏览器发送多个cookie,300个cookie浏览器上限
2.删除Cookie
1.不设置有效期,关闭浏览器自动失效
2.设置有效期时间为0
Cookie cookie = new Cookie("lastLoginTime",System.currentTimeMillis()+"");
cookie.setMaxAge(0);
resp.addCookie(cookie);
3.编码解码
解决乱码问题
URLEncoder.encode("世界","GBK");
URLDecoder.decode(cookie.getValue(),"GBK");
细节:
重写doGet方法时,super.doGet(req,resp);得删掉,不然会报405错误
intellij idea for循环遍历 数组.for 或者 数组.fori 或者 数组.forr
三.Session
1.创建Session
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
import java.io.PrintWriter;
public class SessionServlet1 extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//解决乱码
req.setCharacterEncoding("UTF-8");
resp.setCharacterEncoding("UTF-8");
resp.setContentType("text/html;charset=utf-8");
HttpSession session = req.getSession();
session.setAttribute("name","世界");
String sessionID = session.getId();
PrintWriter printWriter = resp.getWriter();
if (session.isNew())
{
printWriter.print("session创建成功,id="+sessionID);
}
else
{
printWriter.print("session已经在服务器上创建,id="+sessionID);
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
2.获取Session信息
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
public class SessionServlet2 extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//解决乱码
req.setCharacterEncoding("UTF-8");
resp.setCharacterEncoding("UTF-8");
resp.setContentType("text/html;charset=utf-8");
HttpSession session = req.getSession();
String name = (String) session.getAttribute("name");
resp.getWriter().print(name);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
3.删除Session
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
public class SessionServlet3 extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
HttpSession session = req.getSession();
session.removeAttribute("name");
//删除更新Session
session.invalidate();
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
4.设置Session自动失效
<!-- 设置session失效时间为1分钟 -->
<session-config>
<session-timeout>1</session-timeout>
</session-config>
5.Session和Cookie的区别
- Cookie是把用户的数据给浏览器,浏览器保存(可以保存多个)
- Session把用户的数据写到用户独占Session中,服务器端保存(保存重要信息,减少服务器资源的浪费)
- Session对象由服务器创建
Cookie:把你要存的内容整个都给浏览器
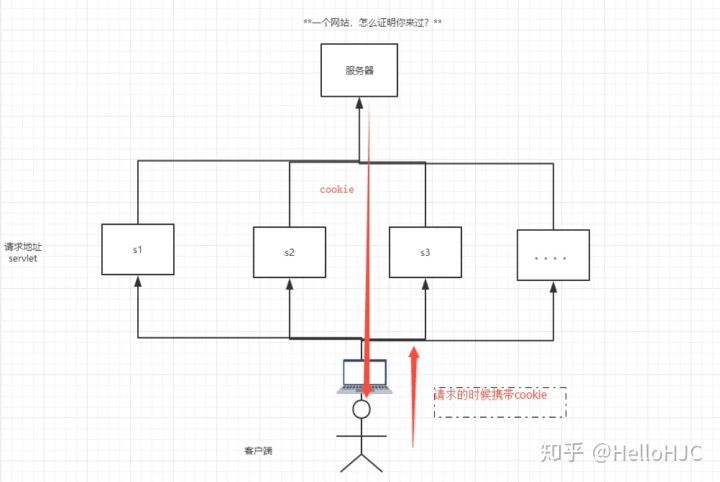
Session:只把id给客户端,存的内容在服务器,根据id在服务器中获取
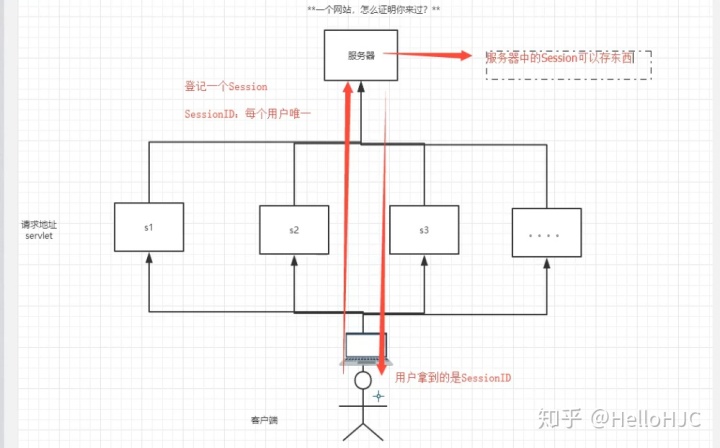
四.JSP
1.JSP原理
在IDEA有一个work目录,发现把index.jsp文件变成了java程序

浏览器不管访问什么资源,其实是都是在访问Servlet!
HttpJspBase继承HttpServlet!
JSP本质上就是一个Servlet


- 生命周期
//初始化
public void _jspInit() {
}
//销毁
public void _jspDestroy() {
}
//Service
public void _jspService(final javax.servlet.http.HttpServletRequest request, final javax.servlet.http.HttpServletResponse response)
throws java.io.IOException, javax.servlet.ServletException {
- 内置对象
final javax.servlet.jsp.PageContext pageContext; //页面上下文
javax.servlet.http.HttpSession session = null; //session
final javax.servlet.ServletContext application; //applicationContext
final javax.servlet.ServletConfig config; //config
javax.servlet.jsp.JspWriter out = null; //out
final java.lang.Object page = this; //page:当前
javax.servlet.jsp.JspWriter _jspx_out = null; //请求
javax.servlet.jsp.PageContext _jspx_page_context = null;//响应
- 输出页面
response.setContentType("text/html");
pageContext = _jspxFactory.getPageContext(this, request, response,
null, true, 8192, true);
_jspx_page_context = pageContext;
application = pageContext.getServletContext();
config = pageContext.getServletConfig();
session = pageContext.getSession();
out = pageContext.getOut();
_jspx_out = out;
out.write("<html>n");
out.write("<body>n");
out.write("<h2>Hello World!</h2>n");
out.write("</body>n");
out.write("</html>n");
- 以上这些对象可以在jsp中直接使用!!
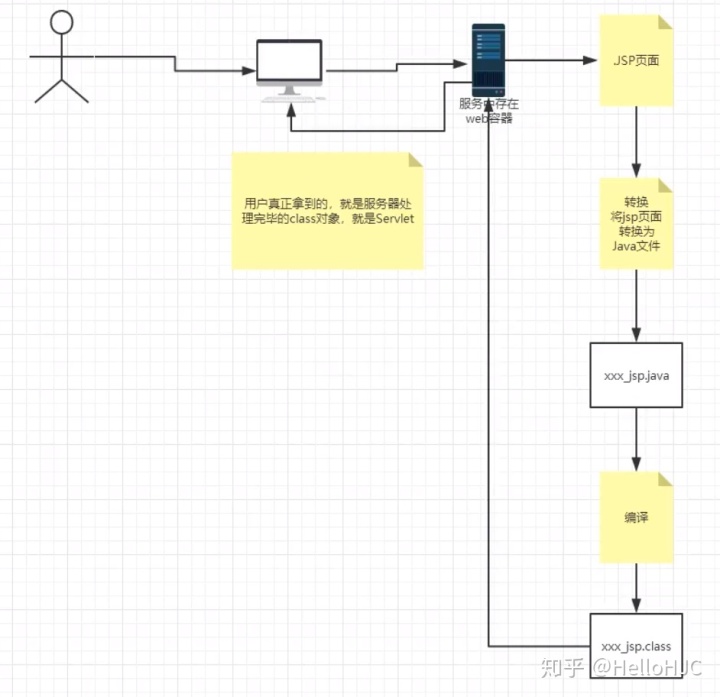
在JSP页面中,
只要是JAVA代码,就会原封不动的输出
如果是HTML代码,就会被转换为
out.write("");
这样的格式
比如:
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%>
<html>
<body>
<h2>Hello World!</h2>
</body>
<% String name = "世界"; %>
<%=name%>
</html>
response.setContentType("text/html; charset=utf-8");
pageContext = _jspxFactory.getPageContext(this, request, response,
null, true, 8192, true);
_jspx_page_context = pageContext;
application = pageContext.getServletContext();
config = pageContext.getServletConfig();
session = pageContext.getSession();
out = pageContext.getOut();
_jspx_out = out;
out.write("n");
out.write("<html>n");
out.write("<body>n");
out.write("<h2>Hello World!</h2>n");
out.write("</body>n");
String name = "世界";
out.write('n');
out.print(name);
out.write("n");
out.write("</html>n");
2.JSP语法
- jsp表达式
<%-- 作用 :用来将程序的输出,输出到客户端 <%= 变量或者表达式%>--%> <%= new Date()%> - jsp脚本片段
<%--jsp脚本片段--%>
<%
int sum = 0;
for (int i = 1; i <= 100 ; i++) {
sum+=i;
}
out.println("<h1>sum="+sum+"</h1>");
%>
<%--在代码中插入html元素--%>
<% for (int i = 0; i < 5; i++) {
%>
<h1>Hello,World<%=i%></h1>
<%
}
%>
- jsp声明
<%-- jsp声明--%>
<%!
static
{
System.out.println("Loading Servlet");
}
private int globalVar = 0;
public void test()
{
System.out.println("进入了方法test()");
}
%>
jsp的声明会被编译到生成的java类中,其它的会被编译到_jspService方法中
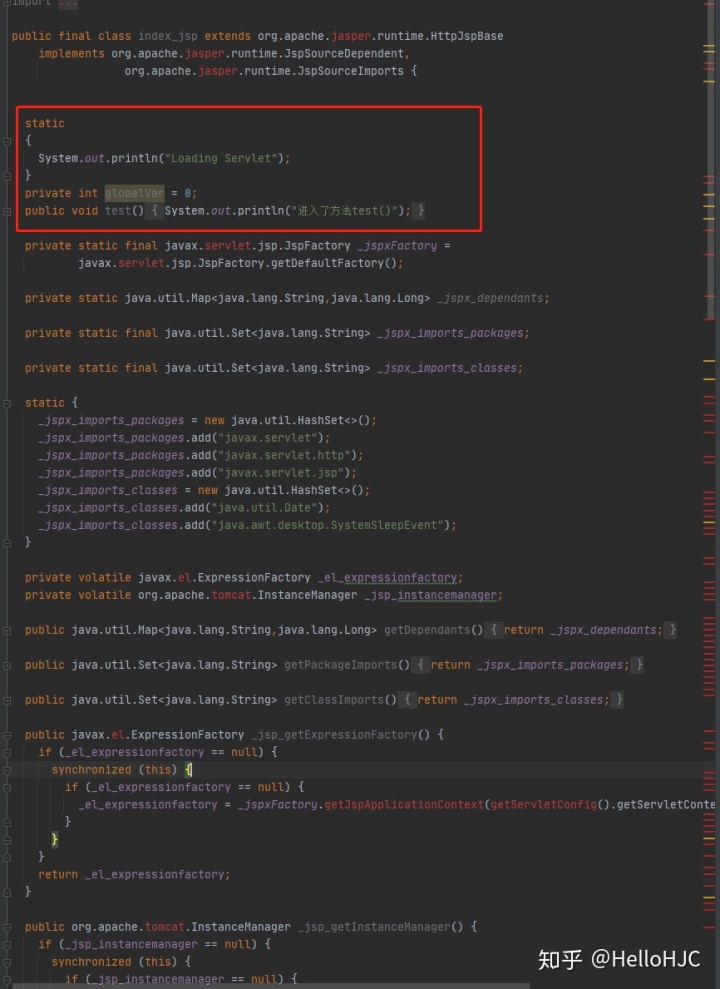
- 注释
<!--我是HTML注释--> <%--我是jsp注释--%> - el表达式
${i} i算是个变量
3.JSP内置对象
- PageContext 存东西
- Request 存东西
- Response
- Sesssion 存东西
- Application【ServletContext】 存东西
- config【ServletConfig】
- out
- page
- exception
存取数据:
<%
pageContext.setAttribute("name1","hjc1");
//保存的数据只在一个页面中有效
request.setAttribute("name2","hjc2");
//保存的是数据只在一个请求中有效,请求转发会携带这个数据
session.setAttribute("name3","hjc3");
//保存的数据只在一次会话中有效,从打开浏览器到关闭浏览器
application.setAttribute("name4","hjc4");
//保存的数据只在服务器中有效,从打开服务器到关闭服务器
%>
<%
//从pageContext中取出,我们通过寻找的方式来
///从底层到高层(作用域):page->request->session->application
//JVM:双亲委派机制
String name1 = (String) pageContext.findAttribute("name1");
String name2 = (String) pageContext.findAttribute("name2");
String name3 = (String) pageContext.findAttribute("name3");
String name4 = (String) pageContext.findAttribute("name4");
String name5 = (String) pageContext.findAttribute("name5");//不存在
%>
<%--使用el表达式输出 el表达式为null则不会输出 <%=%>则会输出 --%>
<h1>取出的值为:</h1>
<h3>${name1}</h3> //打印hjc1
<h3>${name2}</h3> //打印hjc2
<h3>${name3}</h3> //打印hjc3
<h3>${name4}</h3> //打印hjc4
<h3><%=name5%></h3> //打印null
</body>
拓展:JVM双亲委派机制
寻找类从rt.jar->拓展类->应用
比如你自己建了一个类叫String,这个类在java.lang下,但是这个类是不生效的,你只能使用系统的java.lang下的String类
在另外一个页面显示显示数据:
<%
//从pageContext中输出
String name1 = (String) pageContext.findAttribute("name1");
String name2 = (String) pageContext.findAttribute("name2");
String name3 = (String) pageContext.findAttribute("name3");
String name4 = (String) pageContext.findAttribute("name4");
String name5 = (String) pageContext.findAttribute("name5");//不存在
%>
<%--使用el表达式输出 el表达式为null则不会输出 <%=%>则会输出 --%>
<h1>取出的值为:</h1>
<h3>${name1}</h3>
<h3>${name2}</h3>
<h3>${name3}</h3>
<h3>${name4}</h3>
<h3><%=name5%></h3>
name3和name4会被显示
如果是转发到这个页面,name2也会被显示
转发页面的代码:
pageContext.forward("/pageContextDemo02.jsp");
总结
request:客户端向服务器发送请求,产生的数据,用户看完没用了,比如:新闻
session:客户端向服务器发送请求,产生的数据,与用户看完一会还有用,比如:购物车
application:客户端向服务器发送请求,产生的数据,与用户看完一会还有用,其他用户还可能使用,比如:聊天数据