分页技术
分页效果图:

思路:
前台需要展示的是一页一页的数据其他的事情前台不用关心,它的目的只是展示数据。而数据的来源在后台,前台告诉后台需要哪一页的数据以及这一页有多少条数据,pageSize(每页记录数)是可以设定的,所以pageIndex(当前页)是前台和后台交互的核心。那么后台接收到前台的pageIndex,要去识别它,根据这个参数到数据库取得数据返回给前台。
后台需要的前台传送过来的数据有pageIndex(当前页)、pageSize(每页展示的记录数),totalCount(总记录数)通过查询数据库获取,totalPageCount(总页数)通过计算进行获得.
totalPageCount(总页数)计算公式
totalPageCount=totalCount%pageSize==0?totalCount/pageSize?totalCount/pageSize+1
oracle数据库分页语句
select * from (select e.*, rownum rn from emp e where rownum<=3) e2 where e2.rn>0 --第一页数据
select * from (select e.*, rownum rn from emp e where rownum<=6) e2 where e2.rn>3 --第二页数据
select * from (select e.*, rownum rn from emp e where rownum<=9) e2 where e2.rn>6 --第三页数据
--分页单位:每页展示的条数 --->pageSize
--当前页: 当前展示的页数 ---->curPage
---结束位置:分页单位*当前页
--起始位置: (当前页-1)*分页单位
select * from (select * from (select e.*, rownum rn from emp e where rownum<=结束位置) e2 where e2.rn>起始位置
mysql分页语句
-- mysql分页语句使用limit关键字
-- 起始位置:(当前页-1)*分页单位
SELECT * FROM systemdictionaryitem LIMIT 起始位置,分页单位
-- 分页单位:3
-- 第一页:展示前三条
SELECT * FROM systemdictionaryitem LIMIT 0,3
-- 第二页:展示4-6条
SELECT * FROM systemdictionaryitem LIMIT 3,3
-- 第三页:展示7-9条
SELECT * FROM systemdictionaryitem LIMIT 6,3
分页的作用:
①数据量大,一页容不下
②后台查询的是部分数据而不是全部数据、
③降低带宽提高访问速度
工具类:
在开发中分页思路是通用的,可以把分页写成一个工具类,放在util包中,它与业务逻辑是没有关系的
分页工具类代码例子:
import java.util.Arrays;
import java.util.List;
/**
* 分页的三个基本属性
* 1.每页几条记录size 可以有默认值5
* 2.当前页号 index 可以有默认值1
* 3.记录总数totalCount:不可能有默认值,需要查询数据库获取真正的记录总数
*
* 4.一共多少页 :totalPageCount=totalCount/size+1
* 5 30 31 32 33 34 35
* 5.上一页 index-1 当前页1,上一页1
* 6.下一页 index+1 当前页是最后一页 下一页:还是最后一页
*
* 扩展
* 分页Bean还可以放要查询的数据 protected List<T> list;
* 分页Bean还可以放页码列表 [1] 2 3 4 5 private int[] numbers;
*
* @author Administrator
*
* @param <T>
*/
public class PageBean<T> {
private int size = 5;//每页显示记录 //
private int index = 1;// 当前页号
private int totalCount = 0;// 记录总数 ok
private int totalPageCount = 1;// 总页数 ok
private int[] numbers;//展示页数集合 //ok
protected List<T> list;//要显示到页面的数据集
@Override
public String toString() {
return "PageBean [size=" + size + ", index=" + index + ", totalCount=" + totalCount + ", totalPageCount="
+ totalPageCount + ", numbers=" + Arrays.toString(numbers) + ", list=" + list + "]";
}
/**
* 得到开始记录
* @return
*/
public int getStartRow() {
return (index - 1) * size;
}
/**
* 得到结束记录
* @return
*/
public int getEndRow() {
return index * size;
}
/**
* @return Returns the size.
*/
public int getSize() {
return size;
}
/**
* @param size
* The size to set.
*/
public void setSize(int size) {
if (size > 0) {
this.size = size;
}
}
/**
* @return Returns the currentPageNo.
*/
public int getIndex() {
if (totalPageCount == 0) {
return 0;
}
return index;
}
/**
* @param currentPageNo
* The currentPageNo to set.
*/
public void setIndex(int index) {
if (index > 0) {
this.index = index;
}
}
/**
* @return Returns the totalCount.
*/
public int getTotalCount() {
return totalCount;
}
/**
* @param totalCount
* The totalCount to set.
*/
public void setTotalCount(int totalCount) {
if (totalCount >= 0) {
this.totalCount = totalCount;
setTotalPageCountByRs();//根据总记录数计算总页
}
}
public int getTotalPageCount() {
return this.totalPageCount;
}
/**
* 根据总记录数计算总页
* 5
* 20 4
* 23 5
*/
private void setTotalPageCountByRs() {
if (this.size > 0 && this.totalCount > 0 && this.totalCount % this.size == 0) {
this.totalPageCount = this.totalCount / this.size;
} else if (this.size > 0 && this.totalCount > 0 && this.totalCount % this.size > 0) {
this.totalPageCount = (this.totalCount / this.size) + 1;
} else {
this.totalPageCount = 0;
}
setNumbers(totalPageCount);//获取展示页数集合
}
public int[] getNumbers() {
return numbers;
}
/**
* 设置显示页数集合
*
* 默认显示10个页码
* 41 42 43 44 [45 ] 46 47 48 49 50
*
*
* [1] 2 3 4 5 6 7 8 9 10
*
* 41 42 43 44 45 46 47 [48] 49 50
* @param totalPageCount
*/
public void setNumbers(int totalPageCount) {
if(totalPageCount>0){
//!.当前数组的长度
int[] numbers = new int[totalPageCount>10?10:totalPageCount];//页面要显示的页数集合
int k =0;
//
//1.数组长度<10 1 2 3 4 .... 7
//2.数组长度>=10
// 当前页<=6 1 2 3 4 10
// 当前页>=总页数-5 ......12 13 14 15
// 其他 5 6 7 8 9 当前页(10) 10 11 12 13
for(int i = 0;i < totalPageCount;i++){
//保证当前页为集合的中
if((i>=index- (numbers.length/2+1) || i >= totalPageCount-numbers.length) && k<numbers.length){
numbers[k] = i+1;
k++;
}else if(k>=numbers.length){
break;
}
}
this.numbers = numbers;
}
}
public void setNumbers(int[] numbers) {
this.numbers = numbers;
}
public List<T> getList() {
return list;
}
public void setList(List<T> list) {
this.list = list;
}
public static int getTotalPageCount(int iTotalRecordCount, int iPageSize) {
if (iPageSize == 0) {
return 0;
} else {
return (iTotalRecordCount % iPageSize) == 0 ? (iTotalRecordCount / iPageSize) : (iTotalRecordCount / iPageSize) + 1;
}
}
}
文件上传:
上传效果图:
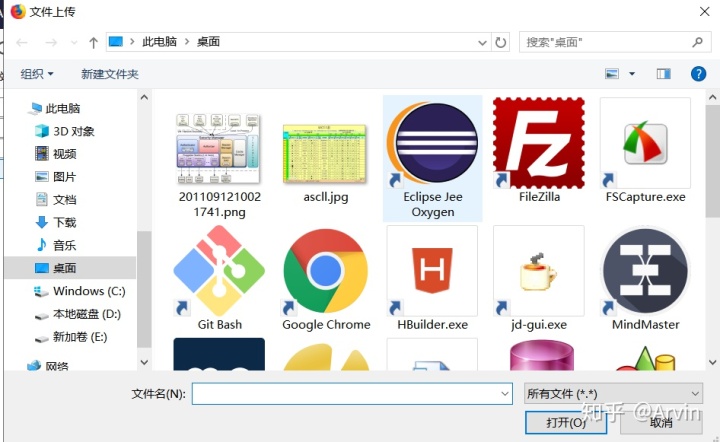
在实现上一般使用第三方jar包,在使用上先导入相关jar包
commons-fileupload.jar //文件上传jar包
commons-io.jar //依赖包
使用代码示例:
/**
* System.out.println(item.isFormField()); //是否是file表单项 是file false 不是file true
System.out.println(item.getFieldName());//表单项的name属性的值
System.out.println(item.getString());//对于非file表单项,value属性的值;对于file表单项,是文件内容
System.out.println(item.getName());//对于file表单项,上传文件的名称;对于非file表单项,返回null
System.out.println(item.getContentType());//对于file表单项,上传文件mime类型 ;对于非file表单项,返回null
System.out.println(item.getSize());//对于file表单项,上传文件的大小 ;对于非file表单项,value值的宽度
*
*
* @author Administrator
*
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
//1.创建FileItemFactory对象
FileItemFactory factory = new DiskFileItemFactory();
//2.创建ServletFileUpload对象
ServletFileUpload upload = new ServletFileUpload(factory);
upload.setHeaderEncoding("utf-8");//解决file表单项的文件名中文乱码问题
//完善5:限制上传的单个和所有文件的大小。建议使用该方式
upload.setFileSizeMax(16*1024);//单个文件的上限
upload.setSizeMax(5*16*1024);//一次上传的所有文件的总大小的上限
//3.通过ServletFileUpload对象实现上传操作,将客户端一个个表单项封装到一个个FileItem中
List<FileItem> itemList = null;
try {
itemList = upload.parseRequest(request);
} catch (FileUploadException e) {
e.printStackTrace();
request.setAttribute("mess", "文件不能超过16kb....");
request.getRequestDispatcher("/add.jsp").forward(request, response);
return;
}
//4.遍历各个FileItem(相当于对各个表单项进行处理)
//System.out.println(itemList.size());
String name = null;
int age = 0;
double score = 0;
String realName =null;
String photoName = null;
String photoType = null;
for(int i=0;i<itemList.size();i++){
//取出第i个表单项
FileItem item = itemList.get(i);
String fieldName = item.getFieldName();
//对各个表单项进行处理(普通表单项和文件表单项要分开处理)
if(item.isFormField()){//普通表单项
//name
if("name".equals(fieldName)){
name = item.getString("utf-8");
}
//age
if("age".equals(fieldName)){
age = Integer.parseInt(item.getString());
}
//score
if("score".equals(fieldName)){
score = Double.parseDouble(item.getString());
}
}else{//文件表单项
//photo
if("photo".equals(fieldName)){
//完善5:限制上传文件大小 16kb(在此处,采用此种方法限制文件大小,是不合适的)
// long size = item.getSize();
// if(size > 16*1024){
// request.setAttribute("mess", "文件不能超过16kb");
// request.getRequestDispatcher("/add.jsp").forward(request, response);
// return;
// }
//完善4:只上传jpg、jpeg和gif文件
String contentType = item.getContentType();//images/jpg
photoType = item.getContentType();
if(!"image/jpeg".equals(contentType) && !"image/gif".equals(contentType)){
request.setAttribute("mess", "只能上传jpg和gif文件");
request.getRequestDispatcher("/add.jsp").forward(request, response);
return;
}
//指定上传的文件夹(Tomcat的webApps目录下,Tomcat的webApps目录之外)
//File dir = new File("d:/upload");//Servlet是服务器技术,此处只服务端路径
//直接使用物理路径,不灵活
//完善3:由逻辑路径得到物理路径,提高灵活性
//File dir = new File("D:Javaapache-tomcat-7.0.79webappsupdownload1/upload");
//String path = "upload";//逻辑路径
///upload-----------C:Javaapache-tomcat-7.0.79webappsupdownload1/upload
String realPath = this.getServletContext().getRealPath("/upload");
File dir = new File(realPath);
//完善1:如果文件夹不存在,就创建
if(!dir.exists()){
dir.mkdirs();
}
//指定上传的文件名
realName = item.getName(); //adfad.fadf.yi.jpg
//指定长传的文件夹和文件名
//完善2:为了防止文件的同名覆盖,上传到服务器端的文件重新命名
UUID uuid = UUID.randomUUID();
String extName = realName.substring(realName.lastIndexOf("."));
photoName = uuid.toString()+extName; //535bc231-935a-427b-a1d7-b3e6d8b8293e.jpg
File file = new File(dir, photoName);
//上传该照片到指定位置
try {
item.write(file);
} catch (Exception e) {
e.printStackTrace();
}
}
//other
}
}
Student stu = new Student(name, age, score, realName, photoName, photoType);
StudentService stuService = new StudentServiceImpl();
int n = stuService.save(stu);
// 页面跳转
if (n != 0) {
// 重定向:/后面要跟上下文路径 /stumgr /stumgr2
response.sendRedirect(request.getContextPath()
+ "/servlet/ShowAllServlet");
} else {
request.setAttribute("mess", "添加失败!");
request.getRequestDispatcher("/add.jsp").forward(request, response);
}
}