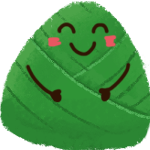
智能图像分析
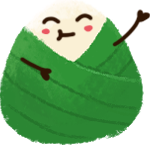

端午节快乐!今天为大家带来一个有趣的小项目,做一个智能图像分析的小程序,一起来看看吧~
知识:
微信前端基础、python基础、django基础
设计思路:
1、前端发送图片(base64格式)
2、后端接收图片,调用腾讯云图像分析接口分析图片,将分析结果返回前端展示。
参数:
image_base64:base64图片
接口:
wx.createCameraContext:创建相机上下文 CameraContext 对象
ctx.takePhoto:拍照
wx.getFileSystemManager().readFile:读取图片base64
wx.showActionSheet:显示操作菜单
wx.showToast:提示信息
wx.navigateTo:保留当前页面,跳转到应用内的某个页面
wx.navigateBack:返回前一页
前端主要文件:
ImgAnalysis:用于发送图片分析请求
msg:用于展示后端返回的数据
后端主要文件:views.py:用于获取请求中的图像ImageAnalysis.py:用于图像分析
前端
首页:ImgAnalysis/ImgAnalysis.wxml
<view class="container">
<camera device-position="back" flash="off" binderror="error" style="width: 100%; height: 250px;{{ display_block }}">camera>
<image src="{{src}}" style="width: 100%; height: 250px;{{ display_none }}">
image>
<view class="ImgOperate" style="">
<button class="ImgAnalysisSendBtn" bindtap="reqImgAnalysis" style="{{ display_none }}">
开始分析
button>
<button class="cancelAnalysisBtn" bindtap="cancelAnalysis" style="{{ display_none }}">
取消
button>
<button type="primary" bindtap="takePhoto" style="width:100%;">
拍照识别
button>
<button type="primary" bindtap="localUpImg" style="width:100%;">
本地上传
button>
view>
view>
图像分析请求:ImgAnalysis/ImgAnalysis.js
逻辑:
点击拍照或本地上传图片>>获取图片base64字符串>>点击图像分析>>向后端发送base64字符串
const app = getApp()
Page({
data: {
avatarUrl: './user-unlogin.png',
userInfo: {},
logged: false,
takeSession: false,
requestResult: '',
},
onLoad: function () {
this.ctx = wx.createCameraContext();
this.setData({
display_none:"display:none;",
display_block:"display:block;"
})
},
//拍照
takePhoto: function () {
this.ctx.takePhoto({
quality: "high",
success: (res) => {
this.setData({
src: res.tempImagePath,
})
var that = this;
wx.getFileSystemManager().readFile({
filePath: that.data.src, //选择图片返回的相对路径
encoding: 'base64', //编码格式
success: res => { //成功的回调
that.setData({
ori_base64src: res.data,
display_none: "display:block;",
display_block: "display:none;"
})
}
})
},
})
},
//请求图像分析
reqImgAnalysis: function () {
var that = this;
wx.showActionSheet({
itemList: ['确认'],
success(res) {
if(res.tapIndex === 0){
wx.showToast({
title: '数据请求中',
icon: 'loading',
duration: 2500
});
wx.request({
url: '图片请求地址',
method: 'POST',
data: {
image_base64: that.data.ori_base64src
},
header: {
'content-type': 'application/json' // 默认值
},
success(res) {
var handle_res = JSON.parse(res.data.data);
wx.showToast({
title: '请求成功',
icon: 'success',
});
that.setData({
returnTabData: handle_res.Labels
})
//跳转
wx.navigateTo({
url: '../msg/msg_success',
success: function (res) {
res.eventChannel.emit('acceptDataFromOpenerPage', { returnTabData: that.data.returnTabData }),
that.setData({
display_none: "display:none;",
display_block: "display:block;",
})
}
})
},
fail:function(res) {
console.log(res)
}
})
}
}
})
},
//本地图片上传
localUpImg:function() {
var that = this;
wx.chooseImage({
count:1,
success: function(res) {
var tempImagePath = res.tempFilePaths[0];
that.setData({
src: tempImagePath,
})
wx.getFileSystemManager().readFile({
filePath: that.data.src, //选择图片返回的相对路径
encoding: 'base64', //编码格式
success: res => { //成功的回调
that.setData({
ori_base64src: res.data,
})
}
}),
that.setData({
display_none: "display:block;",
display_block: "display:none;"
})
},
})
},
//取消图像分析
cancelAnalysis:function() {
var that = this;
that.setData({
display_none: "display:none;",
display_block: "display:block;",
returnTabData:[]
})
}
})
成功页面:msg/msg_success.wxml
<view class="page">
<view class="weui-msg">
<view class="weui-msg__icon-area">
<icon type="success" size="64">icon>
view>
<view class="weui-msg__text-area">
<view class="weui-msg__title">分析结果view>
<view class="weui-msg__desc"><text wx:for="{{returnTabData}}">{{item.Name}}、text>view>
view>
<view class="weui-msg__opr-area">
<view class="weui-btn-area">
<button class="weui-btn" type="primary" bindtap="returnTakePhoto">返回button>
view>
view>
view>
view>
展示后端的数据:msg/msg_success.js
逻辑:
接收后端返回的分析结果>>跳转到成功页面>>展示返回的数据
Page({
data:{},
onLoad: function (option) {
var that = this;
const eventChannel = this.getOpenerEventChannel()
// 监听acceptDataFromOpenerPage事件,获取上一页面通过eventChannel传送到当前页面的数据
eventChannel.on('acceptDataFromOpenerPage', function (data) {
that.setData({
returnTabData: data.returnTabData
})
})
},
returnTakePhoto:function() {
wx.navigateBack({
})
}
});
后端
视图:views.py
逻辑:
获取前端base64字符串>>将字符串传给ImageAnalysis函数得到分析结果>>返回结果
from django.http import JsonResponse
from django.shortcuts import render
from django.views import View
import json
import logging
from util.tengxunyun.ImageAnalysisApi import ImageAnalysis
logger = logging.getLogger('django')
# Create your views here.
class ImgUploadView(View):
def get(self,request):
return JsonResponse({'data':'get'})
def post(self,request):
json_data = request.body
dict_data = json.loads(json_data.decode('utf8'))
image_base64 = dict_data['image_base64']
result = ImageAnalysis(image_base64) #图像分析
return JsonResponse({'data':result})
调用图像分析接口:ImageAnalysis.py
逻辑:
接收base64字符串>>调用腾讯云图像分析接口>>获取并返回分析结果
from tencentcloud.common import credential
from tencentcloud.common.profile.client_profile import ClientProfile
from tencentcloud.common.profile.http_profile import HttpProfile
from tencentcloud.common.exception.tencent_cloud_sdk_exception import TencentCloudSDKException
from tencentcloud.tiia.v20190529 import tiia_client, models
def ImageAnalysis(ImageBase64):
try:
cred = credential.Credential("SecretId", "SecretKey")
httpProfile = HttpProfile()
httpProfile.endpoint = "tiia.tencentcloudapi.com"
clientProfile = ClientProfile()
clientProfile.httpProfile = httpProfile
client = tiia_client.TiiaClient(cred, "ap-guangzhou", clientProfile)
req = models.DetectLabelRequest()
params = '{"ImageBase64":"%s"}'%ImageBase64
req.from_json_string(params)
resp = client.DetectLabel(req)
# print(resp.to_json_string())
return resp.to_json_string()
except TencentCloudSDKException as err:
print(err)
效果图: