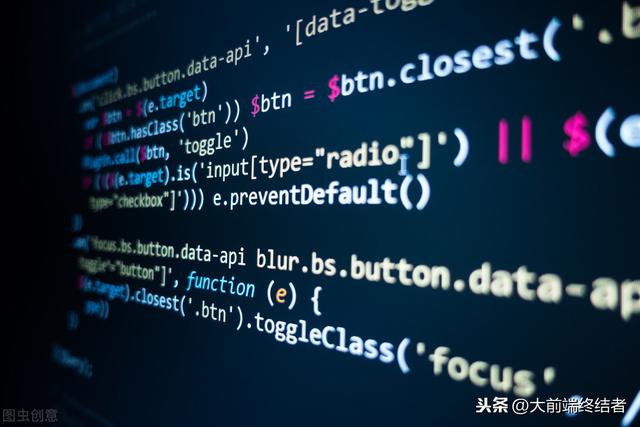
一、NDK和JNI
- NDK是什么
NDK是Native Development Kit 的缩写,是 Android 的工具开发包。
作用是快速开发 C/C++ 的动态库,并自动将动态库与应用一起打包到 apk。
NDK 是属于 Android 的,与 Java 无直接关系。
- JNI是什么
JNI 是 Java Native Interface 的缩写,即 Java 的本地接口。
目的是使得 Java 与本地其他语言(如 C/C++)进行交互。
JNI 是属于 Java 的,与 Android 无直接关系。
- JNI与NDK的关系
JNI 是实现的目的,NDK 是 Android 中实现 JNI 的手段。
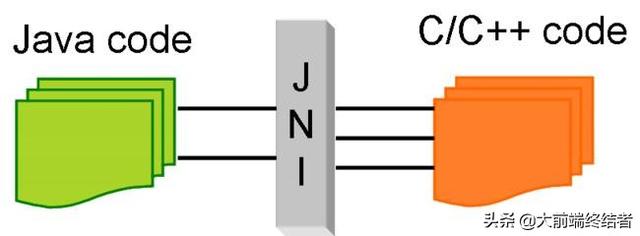
二、Android Studio 调用流程
在AndroidStudio里新建工程,并选择包含 C++ support,会自动帮我们生成一个可执行的 Hello World 程序。相比平常的Android工程,这里主要是多了cpp目录下的native-lib.cpp和CMakeList.txt文件。其中cpp目录就是我们的 C/C++ 代码、预编译库的默认路径,而 CMakeList.txt 就是编译的脚本文件。
MainActivity代码如下:
public class MainActivity extends AppCompatActivity { // Used to load the 'native-lib' library on application startup. static { System.loadLibrary("native-lib"); } @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // Example of a call to a native method TextView tv = (TextView) findViewById(R.id.sample_text); tv.setText(stringFromJNI()); } /** * A native method that is implemented by the 'native-lib' native library, * which is packaged with this application. */ public native String stringFromJNI();}
Java 调用本地方法,是使用 native 关键字。而本例中,本地方法的实现是在一个叫做 “native-lib” 的动态库里(动态库的名称是在 CMakeList.txt 中指定的),要想使用这个动态库,就必须先加载这个库,即 System.loadLibrary(native-lib) 。这些都是 Java 的语法定义。
native-lib.cpp
#include #include extern "C" JNIEXPORT jstring JNICALLJava_com_qq_jnidemo_MainActivity_stringFromJNI( JNIEnv *env, jobject /* this */) { std::string hello = "Hello from C++"; return env->NewStringUTF(hello.c_str());}
上面提到的 public native String stringFromJNI() 方法的实现就是在这里,那怎么知道 Java 中的某个 native 方法是对应的 cpp 中的哪个方法呢?这就和 JNI 的注册有关了,本例中使用的是静态注册,即 “Java包名类名_方法名” 的形式,其中包名也是用下划线替代点号。
整个调用流程就是:
- Gradle 调用您的外部构建脚本 CMakeLists.txt。
- CMake 按照构建脚本中的命令将 C++ 源文件 native-lib.cpp 编译到共享的对象库中,并命名为 libnative-lib.so,Gradle 随后会将其打包到 APK 中。
- 运行时,应用的 MainActivity 会使用 System.loadLibrary() 加载原生库。现在,应用可以使用库的原生函数 stringFromJNI()。
- MainActivity.onCreate() 调用 stringFromJNI(),这将返回“Hello from C++”并使用这些文字更新 TextView。
CMakeLists.txt 文件内容简单分析
# For more information about using CMake with Android Studio, read the# documentation: https://d.android.com/studio/projects/add-native-code.html # Sets the minimum version of CMake required to build the native library. cmake_minimum_required(VERSION 3.4.1) # Creates and names a library, sets it as either STATIC# or SHARED, and provides the relative paths to its source code.# You can define multiple libraries, and CMake builds them for you.# Gradle automatically packages shared libraries with your APK. add_library( # Sets the name of the library. native-lib # Sets the library as a shared library. SHARED # Provides a relative path to your source file(s). src/main/cpp/native-lib.cpp ) # Searches for a specified prebuilt library and stores the path as a# variable. Because CMake includes system libraries in the search path by# default, you only need to specify the name of the public NDK library# you want to add. CMake verifies that the library exists before# completing its build. find_library( # Sets the name of the path variable. log-lib # Specifies the name of the NDK library that # you want CMake to locate. log ) # Specifies libraries CMake should link to your target library. You# can link multiple libraries, such as libraries you define in this# build script, prebuilt third-party libraries, or system libraries. target_link_libraries( # Specifies the target library. native-lib # Links the target library to the log library # included in the NDK. ${log-lib} )
- cmake_minimum_required
指定 Cmake 需要的最低版本
- add_library
创建和命名该库,第一个参数是库的名字,例如取名为 native-lib,将会生成一个命名为 libnative-lib.so 的库。
第二个参数是指定库的类型,一般为 SHARED,即动态库(以 .so 为后缀),还有一种是静态库 STATIC,即静态库(以 .a 为后缀)。
第三个参数是指定该库使用的源文件路径。
使用多个 add_library() 命令,您可以为 CMake 定义要从其他源文件构建的更多库。
- find_library
找到一个 NDK 的库,并且将这个库的路径存储在一个变量中。例如上例中是找到 NDK 中的 log 库(Android 特定的日志支持库),并将其路径存储在 “log-lib” 变量中,在后面你就可以通过 “${log-lib}” 命令取出变量中的值了。
- target_link_libraries
关联库。将指定的库关联起来 。
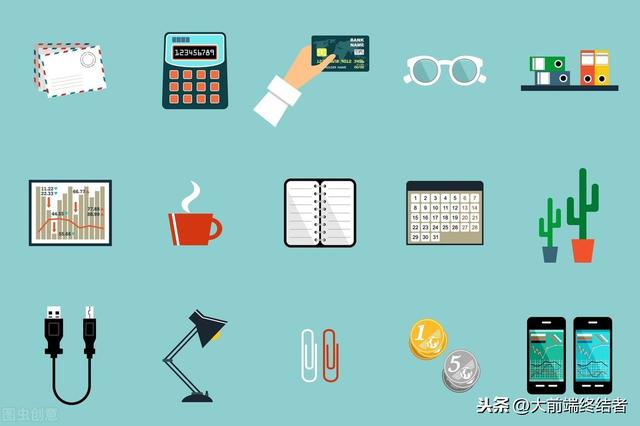
三、JNI数据类型和类型描述符
- 基本数据类型
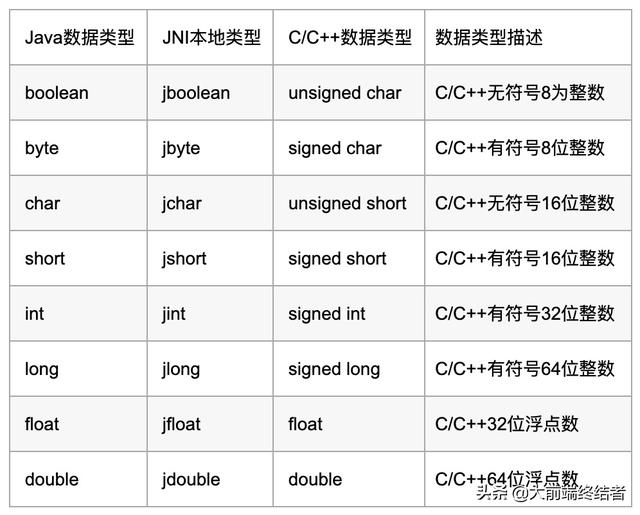
- 引用数据类型
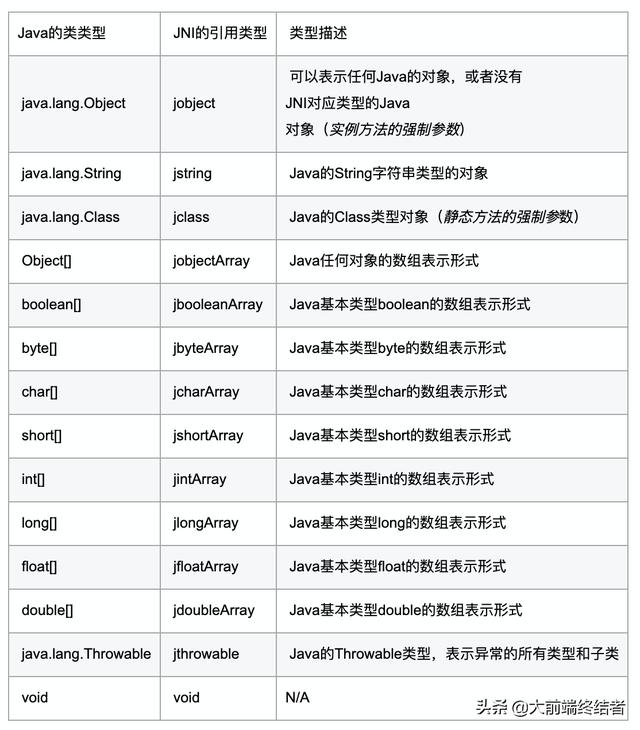
- 数据类型描述符
在JVM虚拟机中,存储数据类型的名称时,是使用指定的描述符来存储,而不是我们习惯的 int,float 等
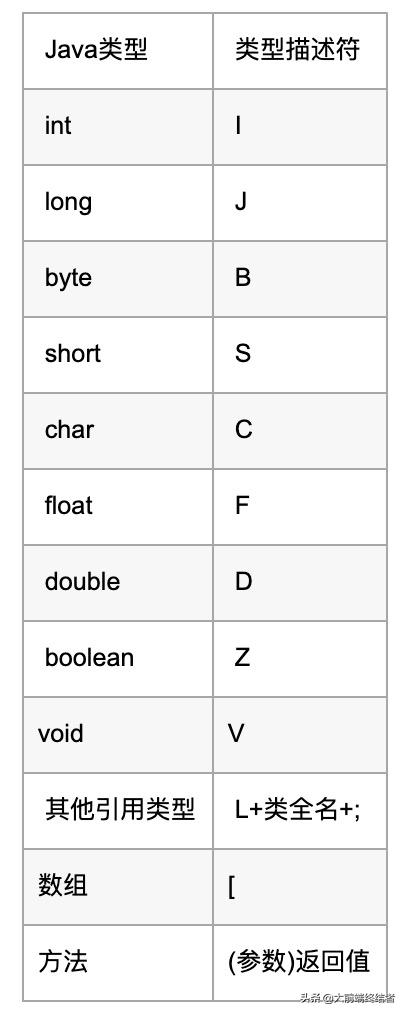
- 例如我们要表示一个 String 类
Java 类型:java.lang.String
JNI 描述符:Ljava/lang/String;
即一个Java类对应的描述符,就是L加上类的全名,其中.要换成/,最后不要忘掉末尾的分号。
- 假如我们想要表示数组的话
Java 类型:String[]
JNI 描述符:[Ljava/lang/String;
Java 类型:int[][]
JNI 描述符:[[I
数组就是简单的在类型描述符前加 [ 即可,二维数组就是两个 [ ,以此类推。
- 方法
Java 方法:long f (int n, String s, int[] arr);
JNI 描述符:(ILjava/lang/String;[I)J
Java 方法:void f ();
JNI 描述符:()V
括号内是每个参数的类型符,括号外就是返回值的类型符。