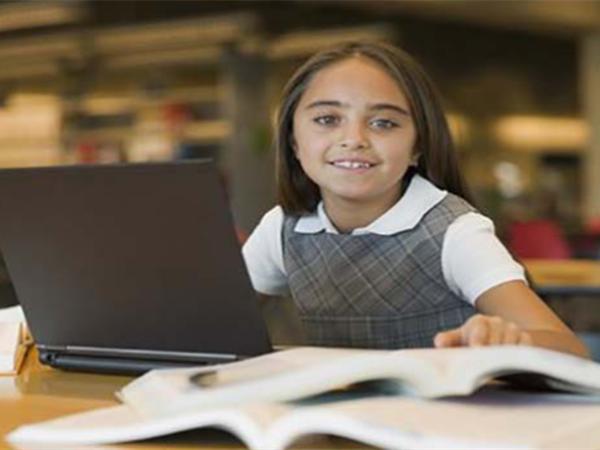
在Python中,字符串是表示Unicode字符的字节数组。但是,Python没有字符数据类型,单个字符只是长度为1的字符串。方括号可用于访问字符串的元素。
创建字符串
Python中的字符串可以使用单引号、双引号甚至三引号创建。
# Python Program for # Creation of String # Creating a String # with single Quotes String1 = 'Welcome to the Geeks World'print("String with the use of Single Quotes: ") print(String1) # Creating a String # with double Quotes String1 = "I'm a Geek"print("String with the use of Double Quotes: ") print(String1) # Creating a String # with triple Quotes String1 = '''I'm a Geek and I live in a world of "Geeks"'''print("String with the use of Triple Quotes: ") print(String1) # Creating String with triple # Quotes allows multiple lines String1 = '''Geeks For Life'''print("Creating a multiline String: ") print(String1)
输出:
String with the use of Single Quotes: Welcome to the Geeks WorldString with the use of Double Quotes: I'm a GeekString with the use of Triple Quotes: I'm a Geek and I live in a world of "Geeks"Creating a multiline String: Geeks For Life
访问Python中的字符
在Python中,可以使用索引方法访问字符串的各个字符。索引允许负地址引用访问字符串后面的字符,例如,-1表示最后一个字符,-2表示倒数第二个字符,依此类推。
访问超出范围的索引将导致IndexError。仅允许将整数作为索引。浮点数或其他类型传递将导致TypeError。
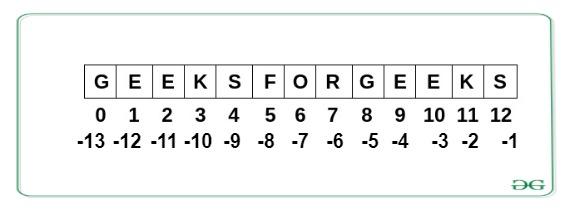
# Python Program to Access # characters of String String1 = "GeeksForGeeks"print("Initial String: ") print(String1) # Printing First character print("First character of String is: ") print(String1[0]) # Printing Last character print("Last character of String is: ") print(String1[-1])
输出:
Initial String: GeeksForGeeksFirst character of String is: GLast character of String is: s
字符串切片(Slicing)
要访问字符串中的一系列字符,请使用切片方法。字符串中的切片是通过使用切片运算符(冒号)完成的。
# Python Program to # demonstrate String slicing # Creating a String String1 = "GeeksForGeeks"print("Initial String: ") print(String1) # Printing 3rd to 12th character print("Slicing characters from 3-12: ") print(String1[3:12]) # Printing characters between # 3rd and 2nd last character print("Slicing characters between " + "3rd and 2nd last character: ") print(String1[3:-2])
输出:
Initial String: GeeksForGeeksSlicing characters from 3-12: ksForGeekSlicing characters between 3rd and 2nd last character: ksForGee
从字符串中删除/更新
在Python中,不允许从字符串中更新或删除字符。这将导致错误,因为不支持从字符串中分配项或删除项。尽管使用内置的del关键字可以删除整个字符串。这是因为字符串是不可变的,因此字符串的元素一旦被分配就不能更改。只能将新字符串重新分配给同一名称。
字符更新:
# Python Program to Update # character of a String String1 = "Hello, I'm a Geek"print("Initial String: ") print(String1) # Updating a character # of the String String1[2] = 'p'print("Updating character at 2nd Index: ") print(String1)
错误:
Traceback (most recent call last):File “/home/360bb1830c83a918fc78aa8979195653.py”, line 10, inString1[2] = ‘p’TypeError: ‘str’ object does not support item assignment
更新整个字符串:
# Python Program to Update # entire String String1 = "Hello, I'm a Geek"print("Initial String: ") print(String1) # Updating a String String1 = "Welcome to the Geek World"print("Updated String: ") print(String1)
输出:
Initial String: Hello, I'm a GeekUpdated String: Welcome to the Geek World
删除字符:
# Python Program to Delete # characters from a String String1 = "Hello, I'm a Geek"print("Initial String: ") print(String1) # Deleting a character # of the String del String1[2] print("Deleting character at 2nd Index: ") print(String1)
错误:
Traceback (most recent call last):File “/home/499e96a61e19944e7e45b7a6e1276742.py”, line 10, indel String1[2]TypeError: ‘str’ object doesn’t support item deletion
删除整个字符串:
使用del关键字可以删除整个字符串。此外,如果我们尝试打印字符串,这将产生一个错误,因为字符串已被删除,无法打印。
# Python Program to Delete # entire String String1 = "Hello, I'm a Geek"print("Initial String: ") print(String1) # Deleting a String # with the use of del del String1 print("Deleting entire String: ") print(String1)
错误:
Traceback (most recent call last):File “/home/e4b8f2170f140da99d2fe57d9d8c6a94.py”, line 12, inprint(String1)NameError: name ‘String1’ is not defined
Python中的转义序列
当打印包含单引号和双引号的字符串时,会导致语法错误,因为字符串已经包含单引号和双引号,因此使用这两种引号都无法打印。因此,要打印这样的字符串,要么使用三个引号,要么使用转义序列来打印这样的字符串。
转义序列以反斜杠开头,可以有不同的解释。如果单引号用于表示字符串,则字符串中的所有单引号都必须转义,双引号也必须转义。
# Python Program for # Escape Sequencing # of String # Initial String String1 = '''I'm a "Geek"'''print("Initial String with use of Triple Quotes: ") print(String1) # Escaping Single Quote String1 = 'I'm a "Geek"'print("Escaping Single Quote: ") print(String1) # Escaping Doule Quotes String1 = "I'm a "Geek""print("Escaping Double Quotes: ") print(String1) # Printing Paths with the # use of Escape Sequences String1 = "C:PythonGeeks"print("Escaping Backslashes: ") print(String1)
输出:
Initial String with use of Triple Quotes: I'm a "Geek"Escaping Single Quote: I'm a "Geek"Escaping Double Quotes: I'm a "Geek"Escaping Backslashes: C:PythonGeeks
要忽略字符串中的转义序列,请使用r或R,这意味着该字符串是原始字符串,并且其内部的转义序列将被忽略。
# Printing Geeks in HEX String1 = "This is x47x65x65x6bx73 in x48x45x58"print("Printing in HEX with the use of Escape Sequences: ") print(String1) # Using raw String to # ignore Escape Sequences String1 = r"This is x47x65x65x6bx73 in x48x45x58"print("Printing Raw String in HEX Format: ") print(String1)
输出:
Printing in HEX with the use of Escape Sequences: This is Geeks in HEXPrinting Raw String in HEX Format: This is x47x65x65x6bx73 in x48x45x58
字符串格式化
Python中的字符串可以使用format()方法进行格式化,该方法是一个非常通用和强大的字符串格式化工具。字符串中的Format方法包含大括号{}作为占位符,占位符可以根据位置或关键字保存参数以指定顺序。
# Python Program for # Formatting of Strings # Default order String1 = "{} {} {}".format('Geeks', 'For', 'Life') print("Print String in default order: ") print(String1) # Positional Formatting String1 = "{1} {0} {2}".format('Geeks', 'For', 'Life') print("Print String in Positional order: ") print(String1) # Keyword Formatting String1 = "{l} {f} {g}".format(g = 'Geeks', f = 'For', l = 'Life') print("Print String in order of Keywords: ") print(String1)
输出:
Print String in default order: Geeks For LifePrint String in Positional order: For Geeks LifePrint String in order of Keywords: Life For Geeks
二进制、十六进制等整数和浮点数可以使用格式说明符舍入或以指数形式显示。
# Formatting of Integers String1 = "{0:b}".format(16) print("Binary representation of 16 is ") print(String1) # Formatting of Floats String1 = "{0:e}".format(165.6458) print("Exponent representation of 165.6458 is ") print(String1) # Rounding off Integers String1 = "{0:.2f}".format(1/6) print("one-sixth is : ") print(String1)
输出:
Binary representation of 16 is 10000Exponent representation of 165.6458 is 1.656458e+02one-sixth is : 0.17
字符串可以使用格式说明符左对齐或居中对齐,用冒号(:)分隔。
# String alignment String1 = "|{:<10}|{:^10}|{:>10}|".format('Geeks','for','Geeks') print("Left, center and right alignment with Formatting: ") print(String1) # To demonstrate aligning of spaces String1 = "{0:^16} was founded in {1:<4}!".format("GeeksforGeeks", 2009) print(String1)
输出:
Left, center and right alignment with Formatting: |Geeks | for | Geeks| GeeksforGeeks was founded in 2009 !
使用%运算符在不使用format方法的情况下完成了旧样式的格式化
# Python Program for # Old Style Formatting # of Integers Integer1 = 12.3456789print("Formatting in 3.2f format: ") print('The value of Integer1 is %3.2f' %Integer1) print("Formatting in 3.4f format: ") print('The value of Integer1 is %3.4f' %Integer1)
输出:
Formatting in 3.2f format: The value of Integer1 is 12.35Formatting in 3.4f format: The value of Integer1 is 12.3457
字符串常量
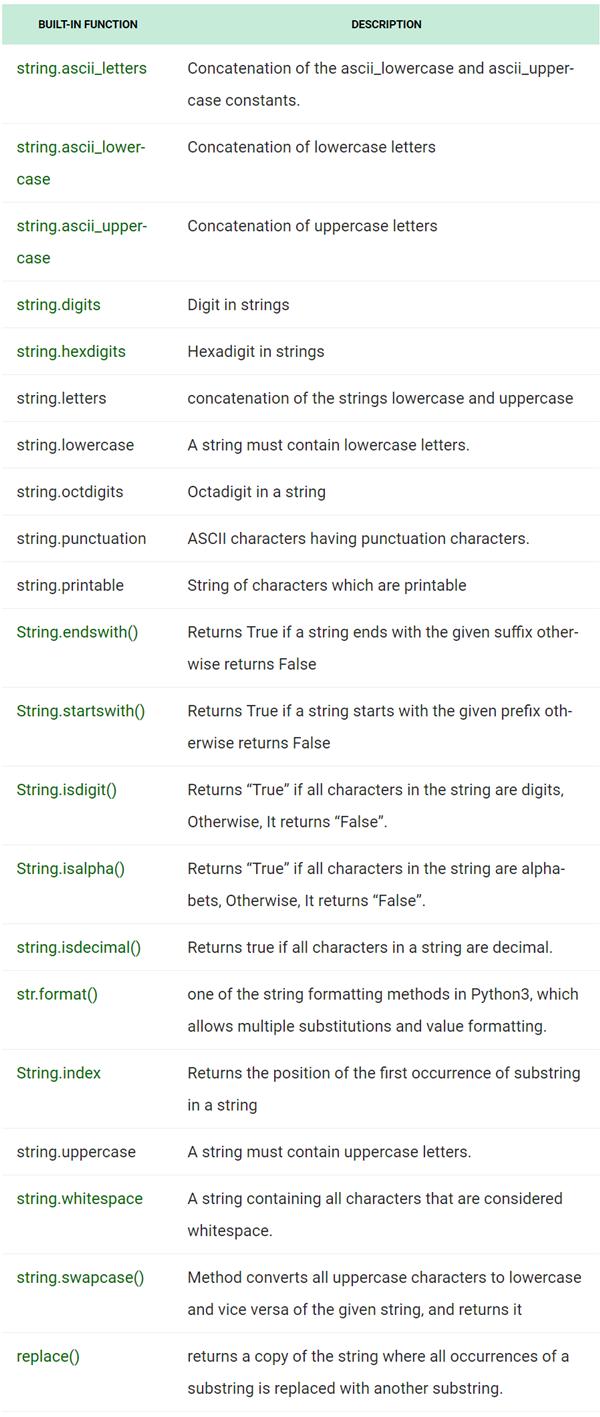
不推荐使用的字符串函数
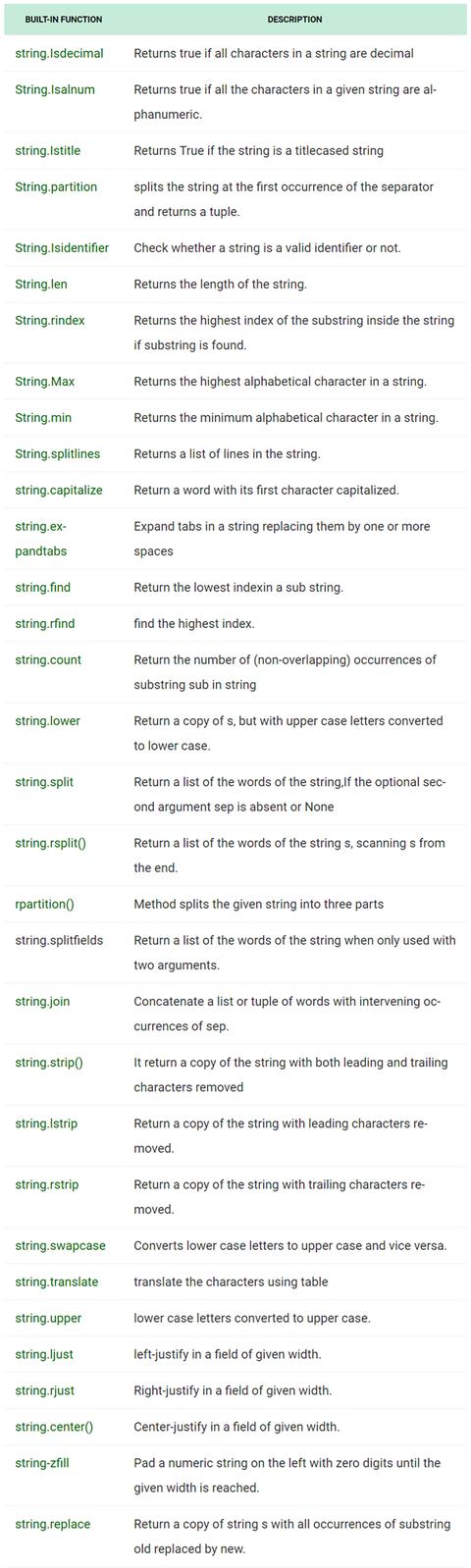