思想:
1.了解“”假溢出“”现象
2.利用循环队列解决
注:循环队列判满时,要利用一个没有元素的空间。所以分配空间时要在多申请一个空间,即使用空间+1。(代码注释里有)
可以借鉴一下别人写的,思路很清稀。
现世安稳:循环队列(C语言)zhuanlan.zhihu.com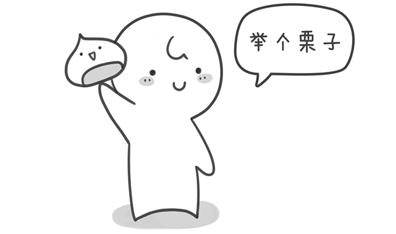
代码:
#include<stdio.h>
#include<stdlib.h>
typedef struct QueueRecord {
int Capacity;
int Front;
int Rear;
int* array;
}*Queue;
int IsEmpty(Queue Q);//判断队是否为空
int IsFull(Queue Q);//判断队是否已满
Queue InitQueue(int ElementDigits);//初始化队列
void Destroy(Queue* Q);//销毁队列
void Enqueue(Queue Q);//入队
int Dequeue(Queue Q);//出队
//判断队列是否为空
int IsEmpty(Queue Q) {
return Q->Rear == Q->Front;
}
//判断队列是否已满
int IsFull(Queue Q) {
return (Q->Rear+1)%Q->Capacity == Q->Front;
}
//初始化队列
Queue InitQueue(int Size) {
Queue Q;
Q = (Queue)malloc(sizeof(struct QueueRecord));
if (Q == NULL) {
printf("out of space");
return 0;
}
Q->Capacity = Size + 1;//多申请一个空间用来判断队列是否已满
Q->array = (int*)malloc(sizeof(int) * Q->Capacity);
if (Q->array == NULL) {
printf("out of space");
return 0;
}
Q->Rear = 0;
Q->Front = 0;
return Q;
}
//入队
void Enqueue(Queue Q,int element) {
if (IsFull(Q)) {
printf("stack is full");
}
else {
Q->array[Q->Rear] = element;
Q->Rear = (Q->Rear++) % Q->Capacity;
}
}
//出队
int DeQueue(Queue Q) {
int temp;
if (IsEmpty(Q)) {
printf("队列以空");
return 0;
}
else {
temp = Q->array[Q->Front];
Q->Front = (Q->Front++) % Q->Capacity;
return temp;
}
}
//销毁队列
void Destroy(Queue* Q)
{
free(Q);
printf("队列已销毁!n");
}
int main() {
Queue Q;
int num;
int e;
printf("输入入队的个数:");
scanf_s("%d", &num);
Q = InitQueue(num);
printf("输入入队元素:");
for (int i = 0; i < num; i++) {
scanf_s("%d",&e);
Enqueue(Q, e);
if (IsFull(Q))
printf("队列已满n");
}
for (int j = 0; j < num; j++) {
printf("%d出队n",DeQueue(Q));
if (IsEmpty(Q))
printf("队列以空n");
}
Destroy(Q);
return 0;
}
结果:
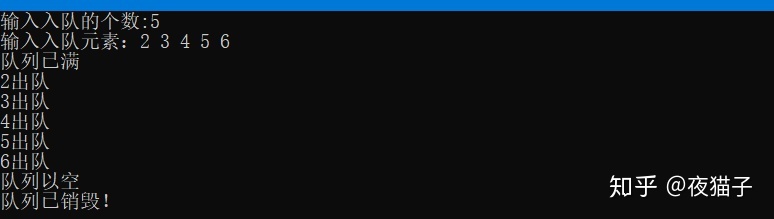