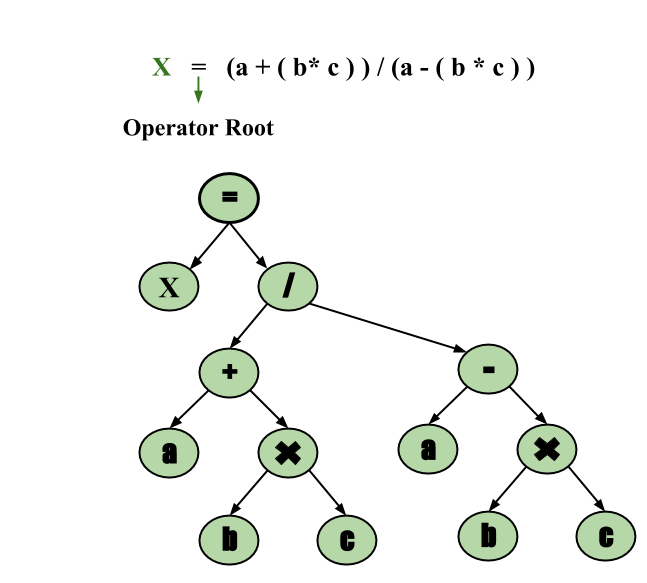
前言
Presto引擎中引入动态代码生成技术主要应用于表达式以及部分算子的局部逻辑代码生成,通过动态代码生成可以产生灵活、高效的执行代码实现。本文主要介绍Presto引擎中coalesce函数从sql解析到生成物理java代码的整个过程。
coalesce函数
coalesce函数语义:
coalesce(value1,value2 [,...])
返回参数列表中的第一个非null值。 与CASE表达式一样,仅在必要时才会计算参数。
语义等价于:
CASE WHEN (value1 IS NOT NULL) THEN expression1
...
WHEN (valueN IS NOT NULL) THEN valueN ELSE NULLEND
以下面这个sql查询为例子来介绍coalesce的实现:
SELECT coalesce(name, comment) FROM tpch.tiny.region
首先从sql解析开始,我们知道Presto支持标准sql语法,它使用ANTLR4生成的SQL语法解析器,查看其语法文件SqlBase.g4
,我们可以知道上面这个查询的中的coalesce(name, comment)
表达式会被解析成FunctionCall
节点(在Presto的AST树中)。
Presto的sql语法解析是一个递归遍历解析树的过程,通过AstBuilder.visit(tree)
从树的根节点开始处理,我们重点看到AstBuilder
中的对FunctionCall
节点的处理逻辑visitFunctionCall
:
// AstBuilder.java
public Node visitFunctionCall(SqlBaseParser.FunctionCallContext context)
{
...
if (name.toString().equalsIgnoreCase("coalesce")) {
check(context.expression().size() >= 2, "The 'coalesce' function must have at least two arguments", context);
check(!window.isPresent(), "OVER clause not valid for 'coalesce' function", context);
check(!distinct, "DISTINCT not valid for 'coalesce' function", context);
check(!filter.isPresent(), "FILTER not valid for 'coalesce' function", context);
return new CoalesceExpression(getLocation(context), visit(context.expression(), Expression.class));
}
...
return new FunctionCall(
getLocation(context),
getQualifiedName(context.qualifiedName()),
window,
filter,
orderBy,
distinct,
visit(context.expression(), Expression.class));
}
```
我们可以看到这个过程中对coalesce函数进行了一些语法限制(至少有两个参数、不能包含开窗子句等