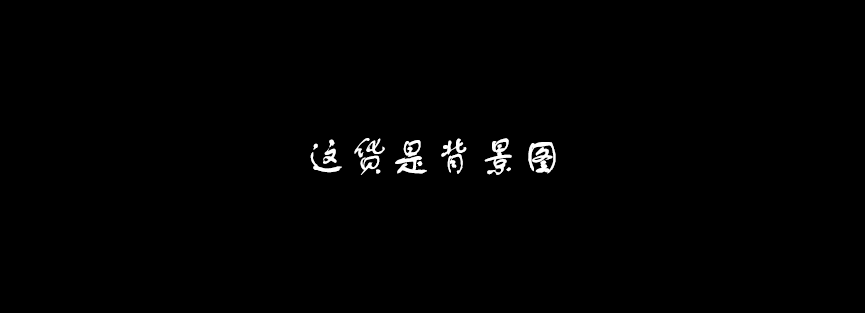
a)
注解是用于描述代码的代码. 例如: @Test(用于描述方法进行junit测试), @Override(用于描述方法的重写), @Param(用于描述属性的名称)
b)
注解的使用风格: @xxx(属性), 使用前必须先导包
c)
使用注解一般用于简化配置文件. 但是, 注解有时候也不是很友好(有时候反而更麻烦), 例如动态SQL.1.在MyBatis中如何解决列名和属性不一致的问题
如果我们在使用查询select标签时使用的时resultType属性时,MyBatis会自动使用Auto_Mapping的映射机制,即相同的类名和属性会自动去匹配,当列名和表明不一致时将会出现查不到数据的情况,此时有两种方法可以去解决这个问题
- 列别名:在查询时我们可以在sql语句中设置列别名与类的属性名保持一致,继续使用自动映射时就可以解决这个问题。
- 可以使用resultMap属性:resultMap是用于自定义映射关系,可以由程序员自定义列名和属性名的映射机制,一旦使用resultMap, 表示不再采用自动映射机制.
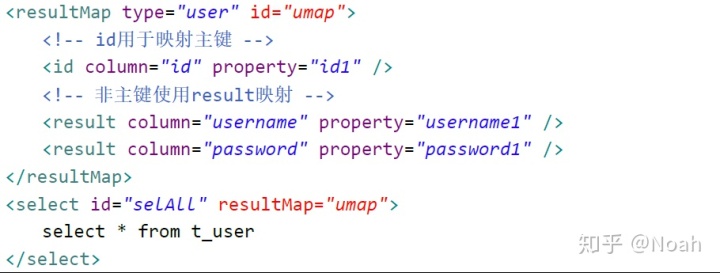
2.MyBatis的优点
- 易于掌握与上手
- sql与源码分开写在xml文件中,便于统一管理和优化
- 降低sql语句与程序的耦合度
- 提供映射标签,支持对象与数据库ORM关系的映射
- 提供d对象映射标签,支持对象关系组建维护
- 提供xml标签,支持编写动态的sql语句
3.多表连接查询,单个对象关联查询
多表连接查询,单个对象,简单来说就是进行一个多对一的关系查询,假设现在有一个t_student 与t_class表,其中t_student 包含着学生的id,姓名name,性别gender,与班级号cid,然后t_class中包含班级信息,班级的id,班级名称与班级的地点。然后要查询每个学生的全部信息就可以使用N+1查询。步骤如下:
- 在StudentMapper.xml中定义多表连接查询SQL语句, 一次性查到需要的所有数据, 包括对应班级的信息.
- 通过<resultMap>定义映射关系, 并通过<association>指定对象属性的映射关系. 可以把<association>看成一个<resultMap>使用. javaType属性表示当前对象, 可以写全限定路径或别名.mapper的xml文件可以这样写:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.bjsxt.mapper.StudentMapper">
<resultMap type="student" id="smp">
<id property="id" column="cid"/>
<result property="name" column="tname"/>
<result property="age" column="age"/>
<result property="gender" column="gender"/>
<result property="cid" column="cid"/>
<association property="clazz" javaType="clazz">
<id property="id" column="cid"/>
<result property="name" column="cname"/>
<result property="room" column="room"/>
</association>
</resultMap>
<select id="selAll" resultMap="smp">
select t.id sid,t.name tname,t.age,t.gender,t.cid cid,
c.name cname,c.room from t_student t left join t_clazz c
on t.cid=c.id
</select>
</mapper>
这样就配置好了映射的xml文件,就可以实现多表进行查询。
4.resultMap的关联方式实现多表查询(一对多)
就是我们进行着查询每个班级都有哪些学生的一对多的多表联合操作,我们可以使用resultMap属性来实现
a)在ClazzMapper.xml中定义多表连接查询SQL语句, 一次性查到需要的所有数据, 包括对应学生的信息.
b)通过<resultMap>定义映射关系, 并通过<collection>指定集合属性泛型的映射关系. 可以把<collection>看成一个<resultMap>使用. ofType属性表示集合的泛型, 可以写全限定路径或别名.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace = "com.bjsxt.mapper.ClazzMapper">
<resultMap type="Clazz" id="cmp">
<id property="id" column="cid"/>
<result property="name" column="cname"/>
<result property="room" column="room"/>
<collection property="stus" javaType="list" ofType="Student">
<id property="id" column="sid"/>
<result property="name" column="tname"/>
<result property="age" column="age"/>
<result property="gender" column="gender"/>
</collection>
</resultMap>
<select id="selAll" resultMap="cmp">
select t.id sid,t.name tname,t.age,t.gender,t.cid cid,c.name cname,c.room from t_clazz c
left join t_student t on t.cid=c.id order by cid
</select>
</mapper>
这样就配置好了xml文件,就可以实现多对一的操作。
5.注解
- a)注解是用于描述代码的代码. 例如: @Test(用于描述方法进行junit测试), @Override(用于描述方法的重写), @Param(用于描述属性的名称)
- b)注解的使用风格: @xxx(属性), 使用前必须先导包
- c)使用注解一般用于简化配置文件. 但是, 注解有时候也不是很友好(有时候反而更麻烦), 例如动态SQL
- d)注解的属性的设定方式是: 属性名=属性值
- e)关于属性值的类型:基本类型和String, 可以直接使用双引号的形式、数组类型, name={值1, 值2, ...}; 如果数组元素只有一个, 可以省略大括号对象类型, name=@对象名(属性)。如果属性是该注解的默认属性, 而且该注解只配置这一个属性, 可以将属性名省略
- f)注解和配置文件可以配合使用
注解可以大大的简化代码量,当进行简单的操作的时候甚至不用配置映射mapperxml文件。
我们可以直接在mapper'包下创建一个要操作表的mapper接口加上注解就可以实现增删改查的操作
package com.bjsxt.mapper;
import java.util.List;
import org.apache.ibatis.annotations.Delete;
import org.apache.ibatis.annotations.Insert;
import org.apache.ibatis.annotations.One;
import org.apache.ibatis.annotations.Result;
import org.apache.ibatis.annotations.Results;
import org.apache.ibatis.annotations.Select;
import org.apache.ibatis.annotations.Update;
import com.bjsxt.pojo.Student;
public interface StudentMapper {
@Select(value= {"select * from t_student"})
List<Student> selAll();
@Insert("insert into t_student values(default,#{name},#{age},#{gender},#{cid})")
int intStu(Student stu);
@Update("update t_student set gender=#{1} where id=#{0}")
int upStu(int id,String gender);
@Delete("delete from t_student where id=#{0}")
int delById(int id);
@Select("select * from t_student")
@Results(value= {
@Result(column="id",property="id",id=true),
@Result(column ="naem",property="name"),
@Result(column ="gender",property="gender"),
@Result(column ="cid",property="cid"),
@Result(property="clazz",one=@One(select="com.bjsxt.mapper.ClazzMapper.selById"), column="cid")
})
List<Student> sel();
}
在实现绑定属性的时候用到的clazzMapper方法
public interface ClazzMapper {
@Select("select * from t_class where id=#{0}")
Clazz selById(int id);
}
6.MyBatis的运行原理:
- 当Mybatis运行开始的时候,先要使用Resources加载类的核心配置文件,之后使用xmlconfigbuilder对配置文件进行解析,将解析的结果封装为configration对象,接着使用configration对象构建一个defaultsqlSessionfactory对象,至此sqlsession对象就构造完成。
- 接下来,通过工厂对象调用openSession方法构建sqlSession对象,在这个过程中,需要通过transactionfactory生成transaction对象,并且需要创建核心执行器executor对象,之后通过这个对象来创建defaultssqlsession对象,至此sqlsession对象创建完成,之后,通过sqlsession对象执行相应的操作,如果执行成功,调用commit方法提交事务;如果失败调用rollback方法进行事务回滚,最后调用close方法关闭session资源,这就是mybatis’的运行原理。
7.MyBatis使用到的接口及其作用:
- Resources(C):用于读取MyBatis核心配置的类
- XMLConfigBuilder(C)用于解析xml核心配置文件
- Configuration(C)用于存放xml解析后的结果
- DefaultSqlSessionFactory(C)时sqlsessionfactory的实现类,创建时需要使用comfiguration对象
- Sqlsession对象,Mybatis操作的核心
- DefaultSqlSession(C)时sqlsession的实现类
- TransactionFactory(I)用于生产Transaction对象,
- Transaction(I)用于操作数据库事务的对象,
- Executor(I)是mybatis的核心执行器,类似于jdbc的statement常用的实现类是simplexecutor