在学Java集合框架的集合类的时候偶然看到使用Iterator遍历集合,感觉代码很简单,就去了解了下。
Iterator,迭代器,它提供了对一个容器对象中的各个元素进行访问,而又不暴露该容器对象的内部细节的一种方法。
Java集合框架的集合类,我们也称之为容器。容器有很多种,比如ArrayList,linkedList,HashSet……每种容器都有其特点,ArrayList底层是一个动态数组,LinkedList是双链表结构,HashSet依赖的是哈希表,每种容器都有其特有的数据结构。
而正是由于容器的内部结构不同,我们有时可能并不知道该如何去遍历一个容器中的元素,为了使对容器内元素的操作更为简单,于是就有了迭代器(Iterator)。
一般我们对于容器的遍历:
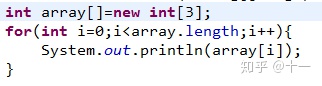
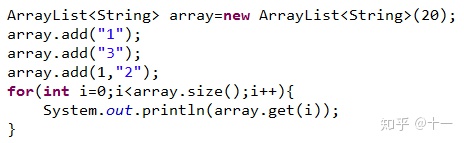
对于这两种遍历方式,我们都知道它的内部结构,访问代码和集合本身是紧密耦合的,无法将访问逻辑从集合类和客户端代码中分离出来。不同的集合会对应不同的遍历方法,客户端代码无法复用。在实际应用中如何将上面两个集合整合是相当麻烦的,所以才有了Iterator,他总是用一种逻辑来遍历集合,使得客户端自身不需要和集合进行打交道,而是控制Iterator向它发送向前向后指令,就可以遍历集合。
1,Iterator:
那么在Java中的Iterator接口是如何实现的呢?
在JDK中它是这样定义的:
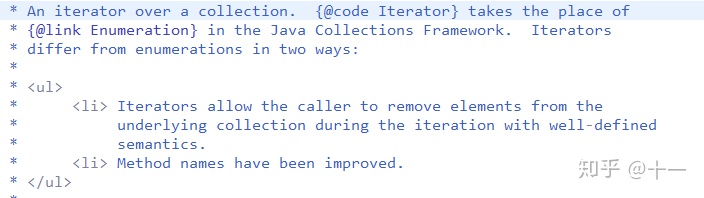
对collection进行迭代的迭代器。迭代器取代了Java Collection Framework中的Enumeration。迭代器和枚举有两点不同:1,迭代器在迭代期间可以从集合中移除元素;2,方法名得到了改进。
Iterator接口定义如下:
public interface Iterator<E> {
boolean hasNext();//判断是否存在下一个对象元素
E next();//获取下一个元素
default void remove() {//移除元素
throw new UnsupportedOperationException("remove");}
default void forEachRemaining(Consumer<? super E> action) {
Objects.requireNonNull(action);
while (hasNext())
action.accept(next());
}
}
2,Iterable:
Java中还提供了一个Iterable接口,该接口实现后返回的是一个迭代器,实现了该接口的子接口有:

实现Iterable接口允许对象称为Foreach语句的目标。就可以通过foreach语句来遍历底层序列。
Iterable接口定义如下:
public interface Iterable<T> {
Iterator<T> iterator();
default void forEach(Consumer<? super T> action) {
Objects.requireNonNull(action);
for (T t : this) {
action.accept(t);
}
}
default Spliterator<T> spliterator() {
return Spliterators.spliteratorUnknownSize(iterator(), 0);
}
}
使用迭代器进行遍历集合的例子:
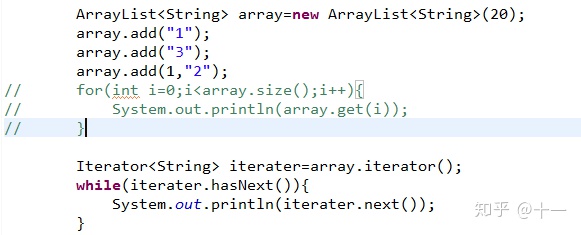
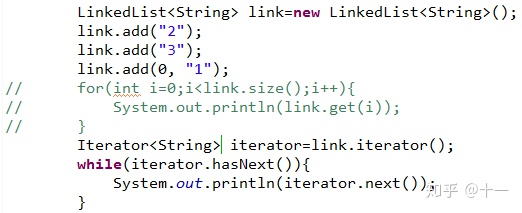
使用foreach遍历集合:
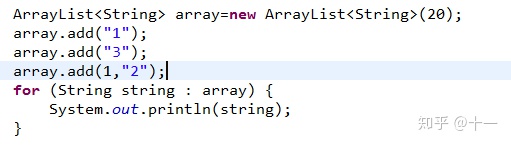
可以看出,使用foreach遍历集合的优势在于代码更加简洁,更不容易出错,不用关心下标的起始值和终止值。
3,Iterator遍历时不可以改变集合问题:
在使用Iterator的时候不能对所遍历的容器进行改变其大小结构的操作。例如在使用Iterator进行迭代时,如果对集合进行add、remove操作就会出现异常,如下:
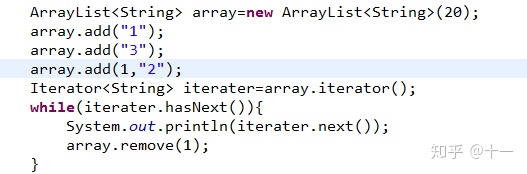

在ArrayList源码中可以看到Iterator的实现源码:
private class Itr implements Iterator<E> {
int cursor; // index of next element to return
int lastRet = -1; // index of last element returned; -1 if no such
int expectedModCount = modCount;
public boolean hasNext() {
return cursor != size;
}
@SuppressWarnings("unchecked")
public E next() {
checkForComodification();
int i = cursor;
if (i >= size)
throw new NoSuchElementException();
Object[] elementData = ArrayList.this.elementData;
if (i >= elementData.length)
throw new ConcurrentModificationException();
cursor = i + 1;
return (E) elementData[lastRet = i];
}
public void remove() {
if (lastRet < 0)
throw new IllegalStateException();
checkForComodification();
try {
ArrayList.this.remove(lastRet);
cursor = lastRet;
lastRet = -1;
expectedModCount = modCount;
} catch (IndexOutOfBoundsException ex) {
throw new ConcurrentModificationException();
}
}
@Override
@SuppressWarnings("unchecked")
public void forEachRemaining(Consumer<? super E> consumer) {
Objects.requireNonNull(consumer);
final int size = ArrayList.this.size;
int i = cursor;
if (i >= size) {
return;
}
final Object[] elementData = ArrayList.this.elementData;
if (i >= elementData.length) {
throw new ConcurrentModificationException();
}
while (i != size && modCount == expectedModCount) {
consumer.accept((E) elementData[i++]);
}
// update once at end of iteration to reduce heap write traffic
cursor = i;
lastRet = i - 1;
checkForComodification();
}
final void checkForComodification() {
if (modCount != expectedModCount)
throw new ConcurrentModificationException();
}
}
通过查看源码可以发现,检查并抛出异常的是checkForComodification() 方法。在ArrayList中,modCount是当前集合的版本号,每次修改集合都会加1;而expectedModCount是当前迭代器的版本号,在迭代器实例化时 int expectedModCount = modCount;,而在checkForComodification() 方法中就是验证modCount和expectedModCount是否相等。当我们调用了ArrayList的add()或remove()时,会更新modCount,而expectedModCount不变,所以下回导致再次调用next()方法的时候抛出异常。而为什么使用Iterator.remove()没有问题呢?我们可以由源代码看出,在Iterator的remove()方法中同步了expectedModCount的值,所以不会抛出异常。