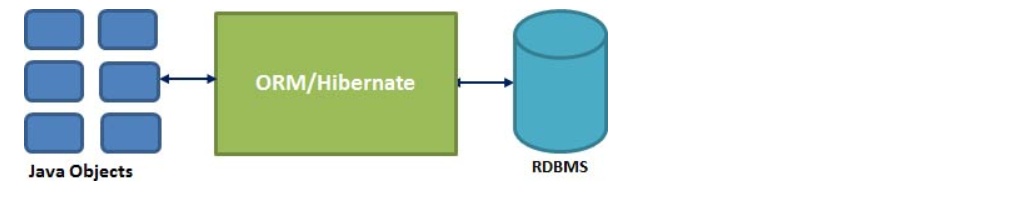
Hibernate 是传统 Java 对象和数据库服务器之间的桥梁,用来处理基于 O/R 映射机制和模式的那些对象。本文介绍如何使用idea构建hibernate项目,并实现对数据库的简单操作。
1、new project 勾选 java Application和hibernate、并配置hibernate版本。
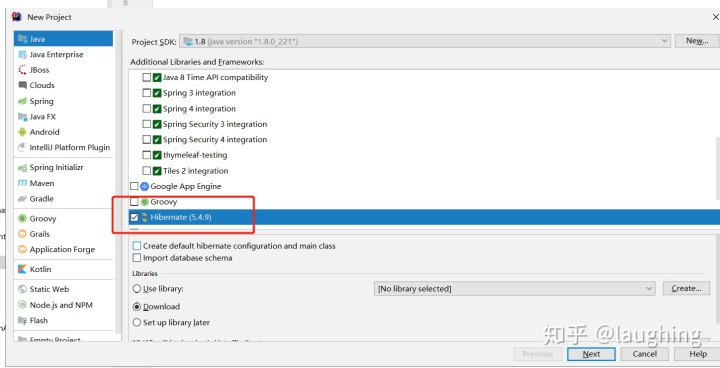
2、连接mysql并添加jdbc驱动包。
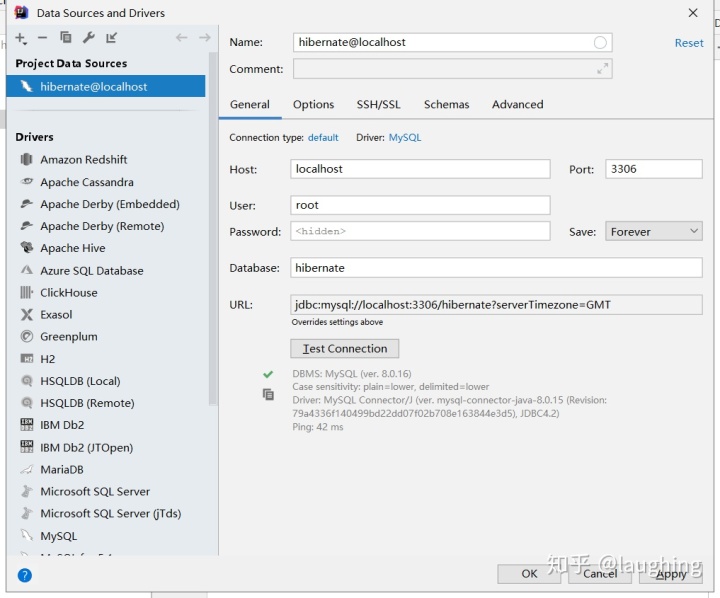

3、配置hibernate.cfg.xml
hibernate.cfg.xml为Hibernate的主配置文件,配置内容包括:数据库连接信息,参数,映射信息等
在project structure - facets中配置hibernate可直接生成
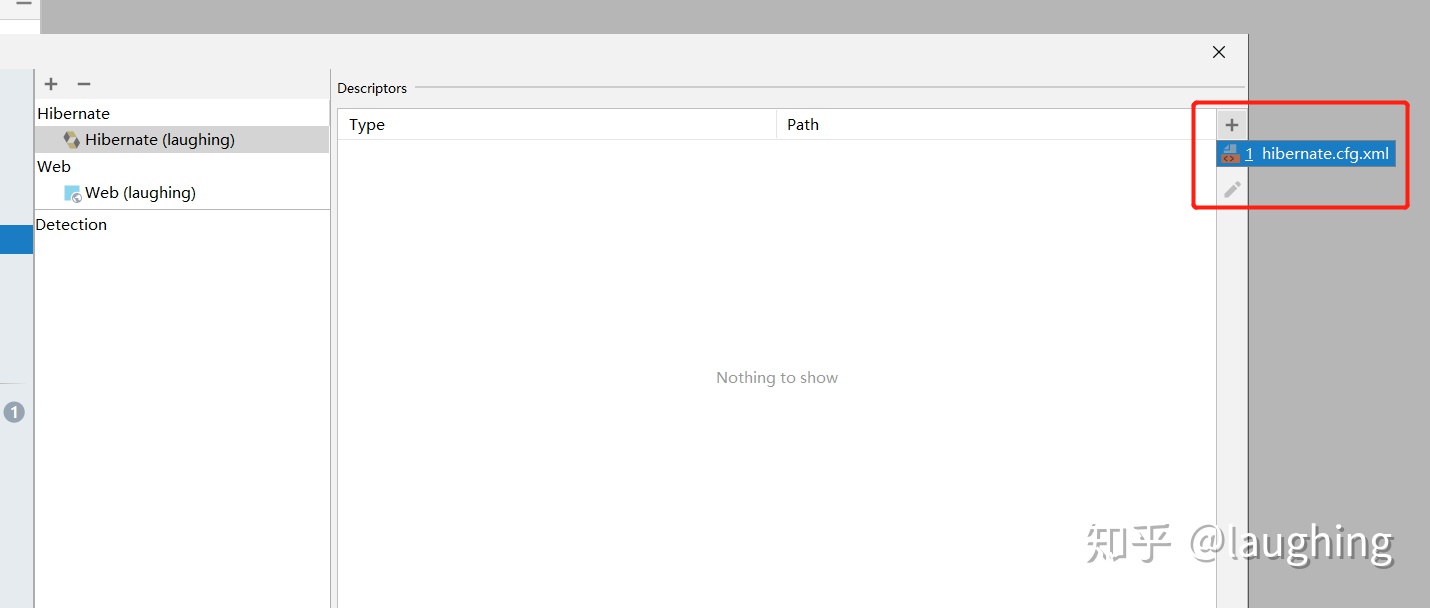
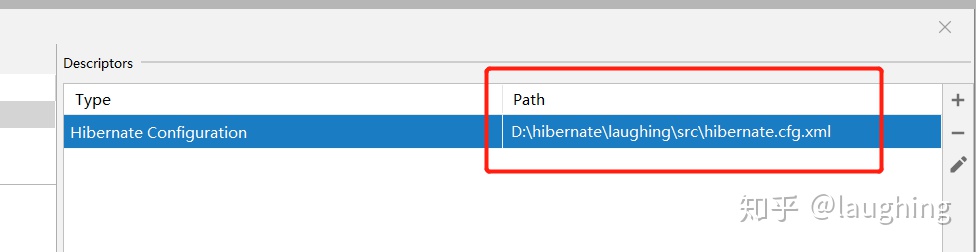
hibernate.cfg.xml配置数据库信息
<?xml version='1.0' encoding='utf-8'?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD//EN"
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!-- 数据连接配置 -->
<property name="hibernate.connection.driver_class">com.mysql.cj.jdbc.Driver</property>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/hibernate?serverTimezone=GMT</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password">*******</property>
<!-- 其他相关配置 -->
<!-- show_sql显示hibernate运行时候执行的sql语句 -->
<property name="hibernate.show_sql">true</property>
<!-- 格式化sql -->
<property name="hibernate.format_sql">true</property>
<mapping resource="entity/UserEntity.hbm.xml"/>
<mapping class="entity.UserEntity"/>
<!-- <property name="hibernate.hbm2ddl.auto">update</property>-->
</session-factory>
</hibernate-configuration>
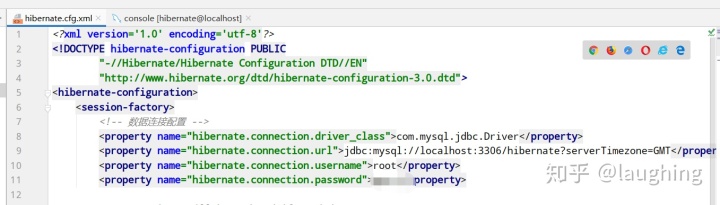
其他相关配置,注释已经写得非常清楚:
配置文件修改hibernate.hbm2ddl.auto可在项目运行时实现自动建表。
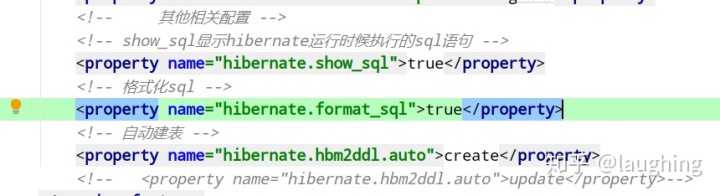
4、生成Hibernate的实体类以及配置文件
在Persistence中右键项目,然后点击Generate Persistence Mapping,选择By Database Schema;
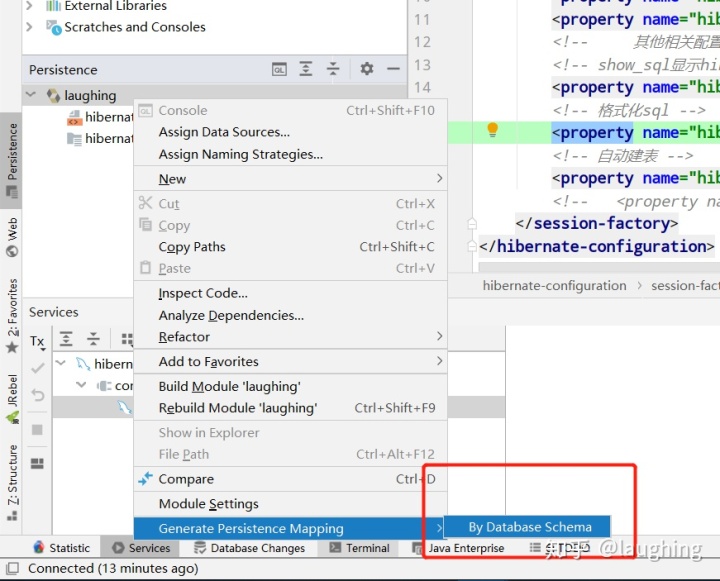
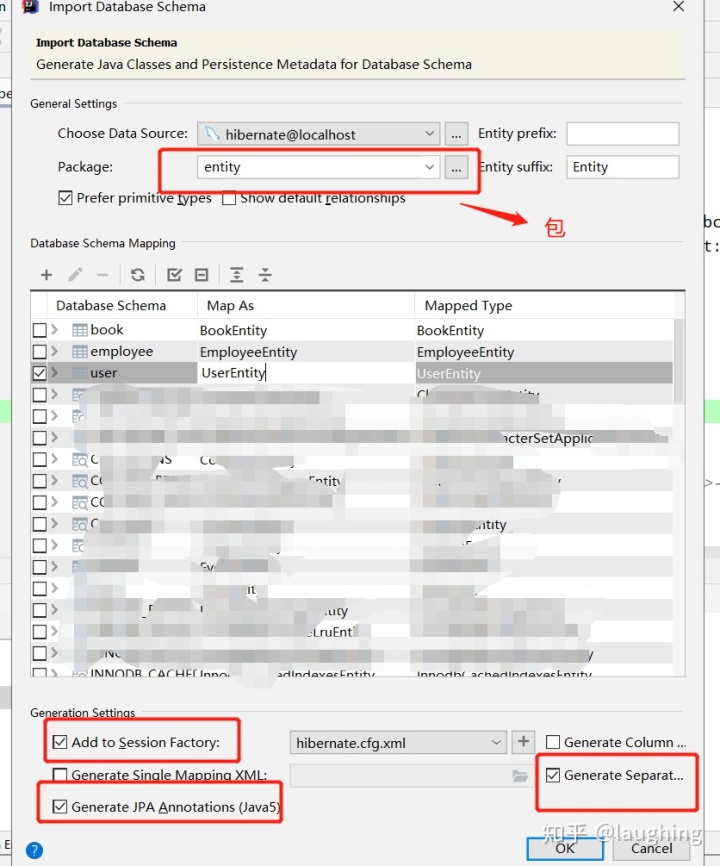
UserEntity :
package entity;
import javax.persistence.*;
import java.util.Objects;
/**
* Created with IntelliJ IDEA
*
* @version 1.0.0
* @author: Laughing
* @create: 2019/12/30 14:12
* @description:
*/
@Entity
@Table(name = "user", schema = "hibernate")
public class UserEntity {
private int id;
private String username;
private String password;
private String email;
@Id
@Column(name = "id")
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
@Basic
@Column(name = "username")
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
@Basic
@Column(name = "password")
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
@Basic
@Column(name = "email")
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
UserEntity that = (UserEntity) o;
return id == that.id &&
Objects.equals(username, that.username) &&
Objects.equals(password, that.password) &&
Objects.equals(email, that.email);
}
@Override
public int hashCode() {
return Objects.hash(id, username, password, email);
}
}
UserEntity.hbn.xml
<?xml version='1.0' encoding='utf-8'?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="entity.UserEntity" table="user" schema="hibernate">
<id name="id" column="id"/>
<property name="username" column="username"/>
<property name="password" column="password"/>
<property name="email" column="email"/>
</class>
</hibernate-mapping>
同时在hibernate.cfg.xml生成加载映射信息:
<mapping resource="entity/UserEntity.hbm.xml"/>
<mapping class="entity.UserEntity"/>
5、编写测试类,这里我们写一个简单的插入。
注释写得非常详细,不再赘述。
package test;
import entity.UserEntity;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.hibernate.cfg.Configuration;
/**
* Created with IntelliJ IDEA
*
* @version 1.0.0
* @author: laughing
* @create: 2019/12/30 14:20
* @description:
*/
public class TestUser {
public static void main(String[] args) {
//创建对象
UserEntity user = new UserEntity();
user.setPassword("*****");
user.setEmail("laughing@163.com");
user.setUsername("laughing");
//获取加载配置管理类
Configuration configuration = new Configuration();
//不给参数就默认加载hibernate.cfg.xml文件,
configuration.configure();
//创建Session工厂对象
SessionFactory factory = configuration.buildSessionFactory();
//得到Session对象
Session session = factory.openSession();
//使用Hibernate操作数据库,都要开启事务,得到事务对象
Transaction transaction = session.getTransaction();
//开启事务
transaction.begin();
//把对象添加到数据库中
session.save(user);
//提交事务
transaction.commit();
//关闭Session
session.close();
}
}
运行,成功插入。非常便捷的就可以实现对业务表的操作。
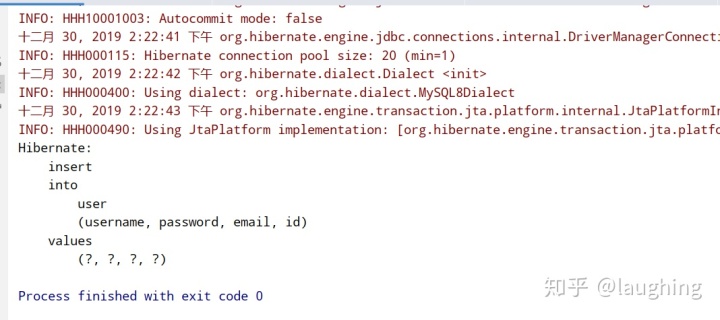