本系列文章旨在记录和总结自己在Java Web开发之路上的知识点、经验、问题和思考,希望能帮助更多(Java)码农和想成为(Java)码农的人。
目录
- 介绍
- 工程结构
- POM文件
- Web部署描述符 - web.xml
- Spring IoC配置元数据 - dispatcher.xml
- Java代码 - 控制器层
- Java代码 - 服务层
- Java代码 - 实体类
- 静态页面 - login.html
- JSP页面
介绍
本篇文章给出截止到上篇文章之时,租房网应用的完整代码。
工程结构
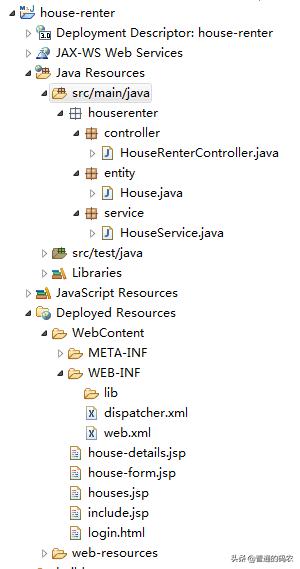
POM文件
4.0.0house-renter house-renter 0.0.1-SNAPSHOTwarorg.springframework spring-context 5.2.1.RELEASEorg.springframework spring-webmvc 5.2.1.RELEASEorg.apache.taglibs taglibs-standard-impl 1.2.5org.apache.taglibs taglibs-standard-spec 1.2.5com.h2database h2 1.4.200org.springframework spring-jdbc 5.2.1.RELEASEcom.alibaba druid 1.1.21 maven-compiler-plugin 3.8.01.81.8 maven-war-plugin 3.2.1WebContent
Web部署描述符 - web.xml
主要是用来配置 Spring MVC 的 DispatcherServlet 。
<?xml version="1.0" encoding="UTF-8"?>house-renterindex.htmlindex.htmindex.jspdefault.htmldefault.htmdefault.jspcharacterEncodingFilterorg.springframework.web.filter.CharacterEncodingFilterencodingUTF-8forceEncodingtruecharacterEncodingFilter/*dispatcherorg.springframework.web.servlet.DispatcherServletcontextConfigLocation/WEB-INF/dispatcher.xml1dispatcher*.action
Spring IoC配置元数据 - dispatcher.xml
主要用来开启组件扫描、配置DataSource和JdbcTemplate这两个Bean。
<?xml version="1.0" encoding="UTF-8"?>
Java代码 - 控制器层
package houserenter.controller;import java.io.UnsupportedEncodingException;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.stereotype.Controller;import org.springframework.web.bind.annotation.GetMapping;import org.springframework.web.bind.annotation.PostMapping;import org.springframework.web.bind.annotation.ResponseBody;import org.springframework.web.servlet.ModelAndView;import houserenter.entity.House;import houserenter.service.HouseService;@Controllerpublic class HouseRenterController {@Autowiredprivate HouseService houseService;@GetMapping("/test.action")@ResponseBodypublic String test() {return "hello";}@PostMapping("/login.action")public ModelAndView postLogin(String userName, String password) {//这里需要验证用户是否已经注册,省略System.out.println("userName: " + userName + ", password: " + password);ModelAndView mv = new ModelAndView();//重定向到查找感兴趣房源列表的动作mv.setViewName("redirect:houses.action?userName=" + userName);return mv;}@GetMapping("/houses.action")public ModelAndView getHouses(String userName) {//这里需要验证用户是否登录,省略ModelAndView mv = new ModelAndView();//查找感兴趣房源并绑定到相应JSP页面,然后将请求转发到该页面mv.addObject("mockHouses", houseService.findHousesInterested(userName));mv.setViewName("houses.jsp?userName=" + userName);return mv;}@GetMapping("/house-details.action")public ModelAndView getHouseDetails(String userName, String houseId) {// 这里需要验证用户是否登录,省略ModelAndView mv = new ModelAndView();//查找房源详情并绑定到相应JSP页面,然后将请求转发到该页面mv.addObject("target", houseService.findHouseById(houseId));mv.setViewName("house-details.jsp?userName=" + userName);return mv;}@GetMapping("/house-form.action")public ModelAndView getHouseForm(String userName, String houseId) {// 这里需要验证用户是否登录,省略ModelAndView mv = new ModelAndView();//查找房源详情并绑定到相应JSP页面,然后将请求转发到该页面mv.addObject("target", houseService.findHouseById(houseId));mv.setViewName("house-form.jsp?userName=" + userName);return mv;}@PostMapping("/house-form.action")public ModelAndView postHouseForm(String userName, House house) throws UnsupportedEncodingException {// 这里需要验证用户是否登录,省略//更新指定房源的详情houseService.updateHouseById(house);//将请求转发到查找房源详情的动作ModelAndView mv = new ModelAndView();mv.setViewName("redirect:house-details.action?userName=" + userName + "&houseId=" + house.getId());return mv;}}
Java代码 - 服务层
package houserenter.service;import java.sql.ResultSet;import java.sql.SQLException;import java.util.List;import javax.annotation.PostConstruct;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.jdbc.core.JdbcTemplate;import org.springframework.jdbc.core.RowMapper;import org.springframework.stereotype.Service;import houserenter.entity.House;@Servicepublic class HouseService {@Autowiredprivate JdbcTemplate jdbcTemplate;@PostConstructpublic void generateMockHouses() {jdbcTemplate.execute("drop table if exists house");jdbcTemplate.execute("create table house(id varchar(20) primary key, name varchar(100), detail varchar(500))");jdbcTemplate.update("insert into house values(?, ?, ?)", "1", "金科嘉苑3-2-1201", "详细信息");jdbcTemplate.update("insert into house values(?, ?, ?)", "2", "万科橙9-1-501", "详细信息");}public List findHousesInterested(String userName) {// 这里查找该用户感兴趣的房源,省略,改为用模拟数据return jdbcTemplate.query("select id,name,detail from house", new HouseMapper());}public House findHouseById(String houseId) {return jdbcTemplate.queryForObject("select id,name,detail from house where id = ?",new Object[]{houseId},new HouseMapper());}public void updateHouseById(House house) {jdbcTemplate.update("update house set id=?, name=?, detail=? where id=?",house.getId(), house.getName(), house.getDetail(), house.getId());}private static final class HouseMapper implements RowMapper {@Overridepublic House mapRow(ResultSet rs, int rowNum) throws SQLException {return new House(rs.getString("id"), rs.getString("name"), rs.getString("detail"));}}}
Java代码 - 实体类
package houserenter.entity;public class House {private String id;private String name;private String detail;public House(String id, String name, String detail) {super();this.id = id;this.name = name;this.detail = detail;}public String getId() {return id;}public void setId(String id) {this.id = id;}public String getName() {return name;}public void setName(String name) {this.name = name;}public String getDetail() {return detail;}public void setDetail(String detail) {this.detail = detail;}@Overridepublic String toString() {return "House [id=" + id + ", name=" + name + ", detail=" + detail + "]";}}
静态页面 - login.html
租房网 - 登录
用户名密码
JSP页面
include.jsp:
租房网
你好,${param.userName}!欢迎来到租房网! 退出
houses.jsp:
共找到你感兴趣的房源 ${mockHouses.size()} 条
${house.name}
house-details.jsp:
${target.name}编辑
${target.detail}
回到列表