73.Set Matrix Zeroes
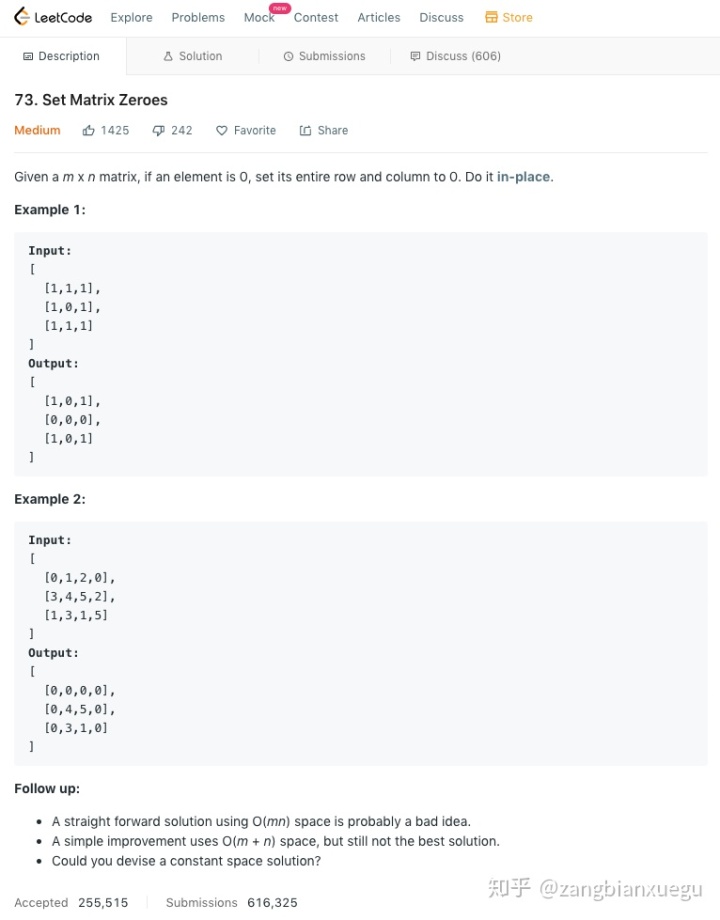
这是一道相对简单的问题,但是如果考虑解决问题的空间复杂度,值得作为一道典型进行研究。
一、复制矩阵。空间复杂度 O(m*n)。
这道题很容易理解,直觉上也让人觉得好做。循环遍历整个二维数组,遇到 0 就将当前行和列的所有元素都变成 0。问题是如果直接改变当前行和列的值,变为 0 之后会影响后续其他元素的判断。
复制这个二维数组就没有问题了。循环复制的二维数组,遇到 0,改变原来的数组行列。
二、记录行和列。空间复杂度 O(m+n)。
循环时,为0,记录当前行和列,两个集合分别记录行和列。然后再根据集合记录改变二维数组。
/**
* @param {number[][]} matrix
* @return {void} Do not return anything, modify matrix in-place instead.
*/
const setZeroes = (matrix) => {
if (matrix && matrix.length) {
let m = matrix.length
let n = matrix[0].length
let rows = new Set()
let cols = new Set()
for (let i = 0; i < m; i++) {
for (let j = 0; j < n; j++) {
if (matrix[i][j] === 0) {
rows.add(i)
cols.add(j)
}
}
}
for (let i = 0; i < m; i++) {
for (let j = 0; j < n; j++) {
if (rows.has(i) || cols.has(j)) {
matrix[i][j] = 0
}
}
}
}
return matrix
}
三、记录列。空间复杂度 O(n)
/**
* @param {number[][]} matrix
* @return {void} Do not return anything, modify matrix in-place instead.
*/
const setZeroes = (matrix) => {
if (matrix && matrix.length) {
let m = matrix.length
let n = matrix[0].length
let cols = []
let flag = false
for (let i = 0; i < m; i++) {
for (let j = 0; j < n; j++) {
if (matrix[i][j] === 0) {
if (cols.indexOf(j) === -1) {
cols.push(j)
}
flag = true
}
}
if (flag) {
for (let k = 0; k < n; k++) {
matrix[i][k] = 0
}
flag = false
}
}
for (let l = 0; l < cols.length; l++) {
for (let i = 0; i < m; i++) {
matrix[i][cols[l]] = 0
}
}
}
return matrix
}
这是我最早想到的方法,循环,用一个数组记录为 0 的元素所在的列,然后先将当前行全部变为 0,循环完毕。再根据记录的列改变矩阵相应的列为 0。
一个问题是需要循环完当前行,记录所有的为 0 的列,再将当前行改为 0。还需要一个变量记录是否要改变当前行。
四、用矩阵本身来记录行和列。空间复杂度 O(1)
用第一行和第一列来记录。从左上开始循环,如果一个元素 matrix[i][j] 为 0,就把 matrix[0][j] 和 matrix[i][0] 改为 0。从右下开始循环,如果当前元素所在的行的第一个元素或者列的第一个元素为 0,则当前元素改为 0。循环一遍就能将所有元素改完。
需要注意的是,[0][0] 元素既表示行又表示列,例如第一行的非第一个元素为 0,如果将第一行第一个元素改为 0,则第二遍循环时,第一列元素将因为第一个元素为 0 而改为 0。所以循环时除去第一列,单独处理。
// https://leetcode.com/problems/set-matrix-zeroes/discuss/26014/Any-shorter-O(1)-space-solution
/**
* @param {number[][]} matrix
* @return {void} Do not return anything, modify matrix in-place instead.
*/
const setZeroes = (matrix) => {
if (matrix && matrix.length) {
let m = matrix.length
let n = matrix[0].length
let col0 = 1
for (let i = 0; i < m; i++) {
if (matrix[i][0] === 0) {
col0 = 0
}
for (let j = 1; j < n; j++) {
if (matrix[i][j] === 0) {
matrix[i][0] = matrix[0][j] = 0
}
}
}
for (let i = m - 1; i >= 0; i--) {
for (let j = n - 1; j >= 1; j--) {
if (matrix[i][0] === 0 || matrix[0][j] === 0) {
matrix[i][j] = 0
}
}
if (col0 === 0) {
matrix[i][0] = 0
}
}
}
}
LeetCode 上另一个解法是设置一个特殊的值,尽量使其不在矩阵中。循环遍历,如果当前元素为 0,则设置所在行和所在列不为 0 的元素为那个值,标记之后需要改变。之后循环矩阵,根据标记改变为 0。
class Solution {
public void setZeroes(int[][] matrix) {
int MODIFIED = -1000000;
int R = matrix.length;
int C = matrix[0].length;
for (int r = 0; r < R; r++) {
for (int c = 0; c < C; c++) {
if (matrix[r][c] == 0) {
// We modify the corresponding rows and column elements in place.
// Note, we only change the non zeroes to MODIFIED
for (int k = 0; k < C; k++) {
if (matrix[r][k] != 0) {
matrix[r][k] = MODIFIED;
}
}
for (int k = 0; k < R; k++) {
if (matrix[k][c] != 0) {
matrix[k][c] = MODIFIED;
}
}
}
}
}
for (int r = 0; r < R; r++) {
for (int c = 0; c < C; c++) {
// Make a second pass and change all MODIFIED elements to 0 """
if (matrix[r][c] == MODIFIED) {
matrix[r][c] = 0;
}
}
}
}
}
但是这种解法存在风险,因为矩阵中可能存在那个标记的值。
五、奇技淫巧。空间复杂度 O(0)
根据Object.is(-0, 0)
为 false,将为 0 的元素所在的行和列元素改为 -0。根据0 && -0 = 0
和-0 && 0 = -0
区分原来为 0 的元素和后来被改为 -0 的元素。因为-0 === 0
,所以不影响结果。不需要额外的存储空间。
// https://leetcode.com/problems/set-matrix-zeroes/discuss/26047/Quiet-simple-answer-'hacking'-with-javascript
/**
* @param {number[][]} matrix
* @return {void} Do not return anything, modify matrix in-place instead.
*/
const setZeroes = matrix => {
let m = matrix.length
let n = matrix[0].length
for (let i = 0; i < m; i++) {
for (let j = 0; j < n; j++) {
if (!Object.is(matrix[i][j], 0)) {
continue
}
for (let x = 0; x < m; x++) {
matrix[x][j] = matrix[x][j] && -0
}
for (let y = 0; y < n; y++) {
matrix[i][y] = matrix[i][y] && -0
}
}
}
}
参考
https://leetcode.com/problems/set-matrix-zeroes/
https://leetcode.com/problems/set-matrix-zeroes/solution/
https://leetcode.com/problems/set-matrix-zeroes/discuss/26014/Any-shorter-O(1)-space-solution
https://leetcode.com/problems/set-matrix-zeroes/discuss/26047/Quiet-simple-answer-'hacking'-with-javascript
https://leetcode.wang/leetcode-73-Set-Matrix-Zeroes.html