03-结构化程式与自定义函数
一、MATLAB Script(脚本)
1、A file containing a series of MATLAB commands(一个包含系列指令的文件)
2、Pretty much like a C/C++ program(与C/C++程式语言相似)
3、Scripts need to be save to a <file>.m file before they can run(在执行前需要保存为脚本文件)
练习
for i=1:10
x = linspace(0,10,101);
plot(x,sin(x + i));
print(gcf,'-deps',strcat('plot',num2str(i),'.ps'));
end
答案注解
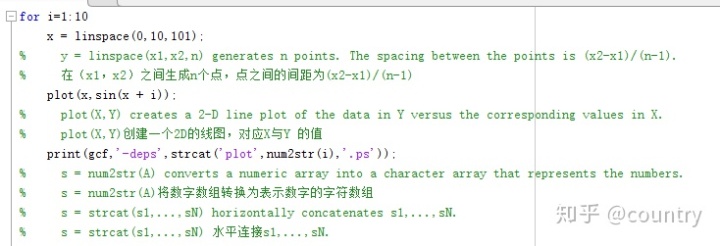
二、Script FLow(脚本执行流)
1、Typically scripts run from the first line to the last(通常脚本从第一行执行到最后一行)
2、Structured programming techniques(subroutine,loop,condition,etc)are applied to make the program looks neat(应用结构化编程技术可以让程序看起来更简洁,如子程序、循环、条件等)
3、Flow Control(执行流控制)
1)if,elseif,else:Execute statements if condition is true(条件为真时执行语句)
2)for:Execute statements specified number of times(执行指定次数的语句)
3)switch,case,otherwise:Execute one of several groups of statements(执行几组语句之一)
4)try,catch:Execute statements and catch resulting errors(执行语句并捕获产生的错误)
5)while:Repeat execution of statements while condition is true(条件为真时重复执行语句)
6)break:Terminate execution of for or while loop(终止for或while循环的执行)
7)continue:Pass control to next iteration of for or while loop(将控制传递到for或while循环的下一次迭代)
8)end:Terminate block of code,or indicate last array index(终止代码块,或指示最后一个数组索引)
9)pause:Halt execution temporarily(暂时停止执行)
10)return:Return control to invoking function(返回控件值到调用的函数)
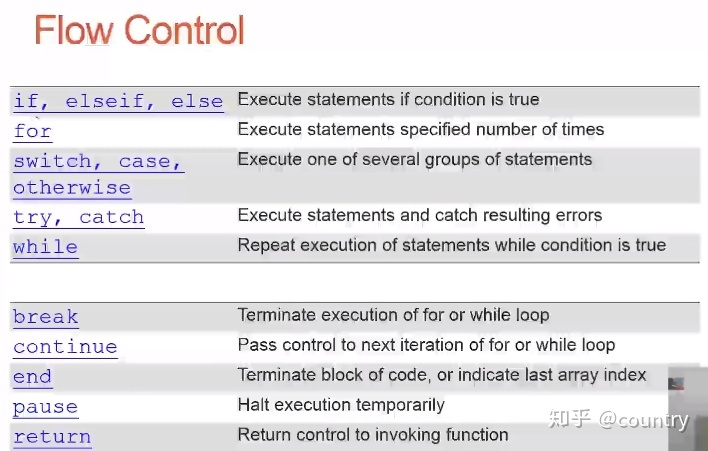
4、Relational(Logical)Operators(关系/逻辑运算符)
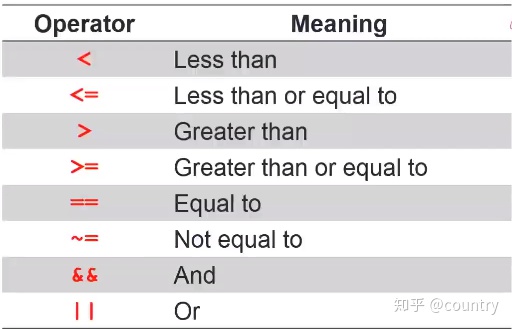
5、几个常用语法
1)if elseif else
例题代码:
a = 3;
if rem(a,2) == 0
disp('a is even')
else
disp('a is odd')
end
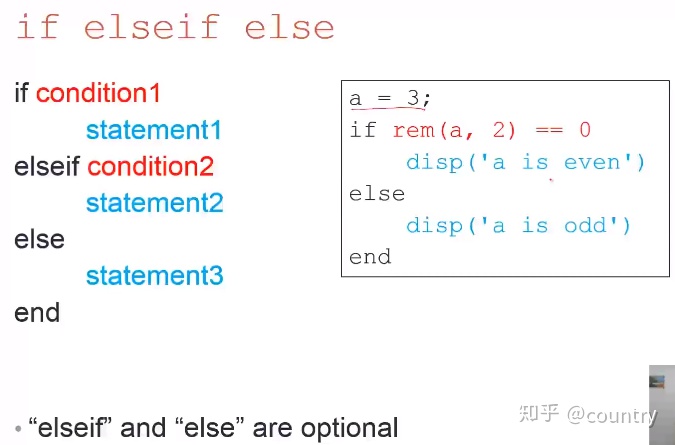
注意:
·"elseif" and "else" are optional(“elseif”和“else”是可选的)
·r = rem(a,b)
returns the remainder after division ofa
byb
, wherea
is the dividend andb
is the divisor.
r=rem(a,b)返回a除以b后的余数,其中a是被除数,b是除数。
2)switch
例题代码:
input_num = 1;
switch input_num
case -1
disp('negative 1');
case 0
disp('zero');
case 1
disp('positive 1');
otherwise
disp('other value');
end
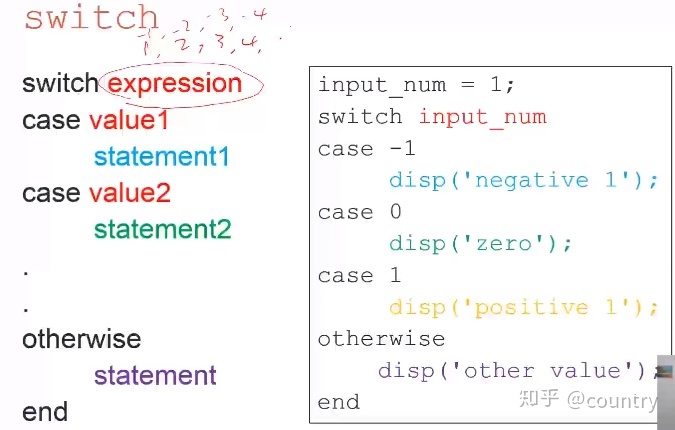
3)while
例题代码:
n = 1;
while prod(1 : n) < 1e100
n = n + 1;
end
disp(n)
%disp(n)用于显示n的数值
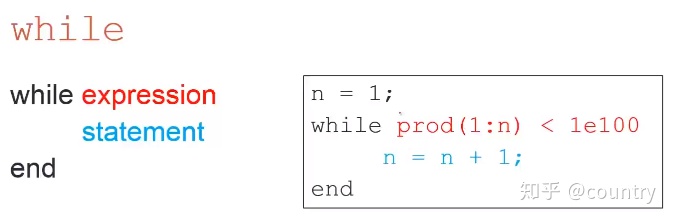
注意:
·B= prod(A)
returns the product of the array elements ofA
.
B=prod(A)返回A的数组元素的乘积。prod(1 : n)即1*2*3...*n(n!);
·1e100,即
4)Excise:Use while loop to calculate the summation of the series 1+2+3+...+999(使用while循环计算序列1+2+3+…+999的总和)
答案代码
n = 1;
s = 0;
while n < 1000
s = s + n;
n = n + 1;
end
disp(n);
disp(s);
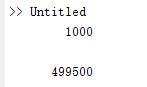
5)for
例题代码:
for n = 1 : 10
a(n) = 2 ^ n;
end
disp(a)
结果
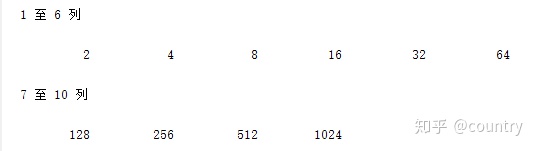
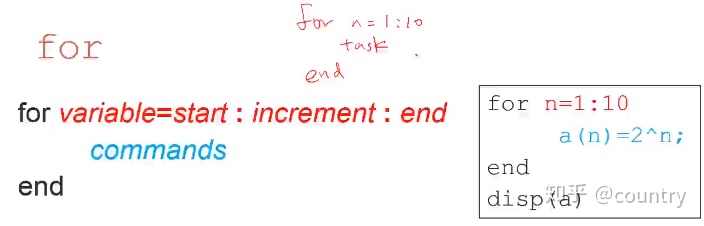
6)break
·Terminate the execution of for or while loops(终止for或while循环的执行)
·Used in iteration where convergence is not guaranteed(用于不保证收敛的迭代)
示例代码:
x = 2;k = 0;error = inf;
error_threshold = 1e-32;
while error > error_threshold
if k > 100
break
end
x = x - sin(x) / cos(x);
error = abs(x - pi);
k = k + 1;
end
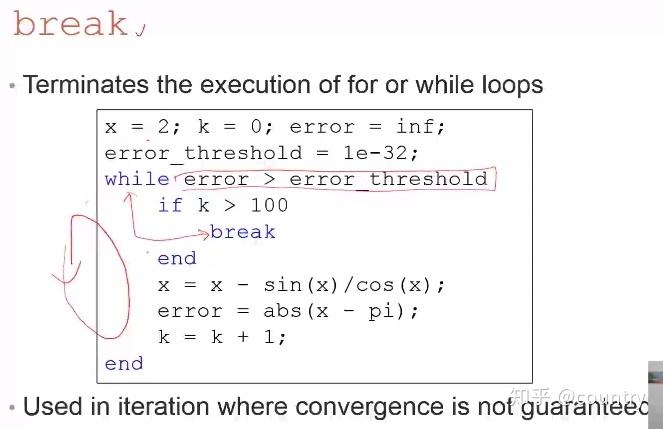
6、Pre-allocation Space to Variables(变量预分配空间)
1、In the previous example,we do not pre-allocate space to vector a rather than letting MATLAB resize it on every iteration(在前面的例子中,我们没有预先分配空间给向量a,而是让MATLAB在每次迭代时调整它的大小)
例如,以下两个例子,B代码执行比A代码快10倍!
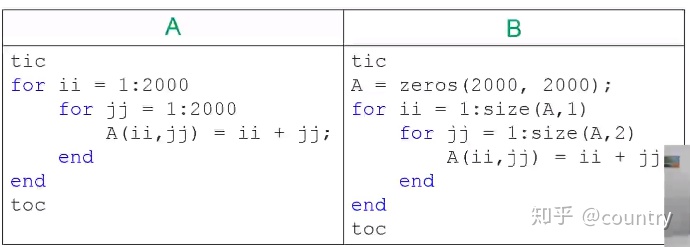
答案:
A的代码及运行时间:(Not pre-allocating)
tic
for ii = 1:2000
for jj = 1:2000
A(ii,jj) = ii + jj;
end
end
toc
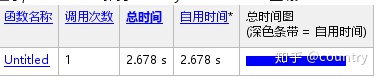
B的代码及运行时间:(Pre-allocating)
tic
A = zeros(2000,2000);
for ii = 1:size(A,1)
for jj = 1:size(A,2)
A(ii,jj) = ii + jj;
end
end
toc

结论:有预宣告空间的,在迭代中可以大大节省运算时间
注意:tic toc,用于测量两个指令之间所执行的时间,并输出结果
8、练习
Use structured programming to:
1)Copy entries in matrix A to matrix B(将矩阵A元素复制到矩阵B)
2)Change the value in matrix B if their corresponding entries in matrix A is negative.(如果矩阵B中的对应项为负,则更改矩阵B中的值,随便什么数值都可以)
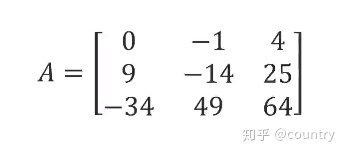
练习答案:
A = [0 -1 4 ; 9 -14 25 ; -34 49 64];
B = zeros(3,3);
for i = 1:9
B(i) = A(i);
end
disp(A)
disp(B)
%将A矩阵复制到B矩阵
for j = 1:9
if B(j) < 0
B(j) = unidrnd(100);
end
end
disp(B)
%将B矩阵小于0的值,随机赋值一个(0,100)的正整数
9、Tips for Script Writing(脚本编写技巧)
1)At the beginning of your script,use command(在脚本的开头,使用命令)
· clear all to remove previous variables(删除之前的变量)
· close all to close all figures(关闭所有图形)
· clc to Clear Command Window(清空命令窗口)
2)Use semicolon‘ ;’ at the end of commands to inhibit unwanted output(在命令末尾使用分号“;”以禁止不需要的结果输出)
3)Use ellipsis ... to make scripts more readable:(使用省略号‘...’,可使脚本更具可读性,即换行号)
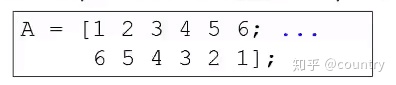
4)Press Ctrl + C to terminate the script before conclusion(按Ctrl+C在结束前终止脚本,左下角会显示busy,即宕机的情况使用)
10、Scripts vs. Functions(脚本和功能)
1)Script and functions are both .m files that contain MATLAB commands(脚本和函数都是包含MATLAB命令的.m文件)
2)Functions are written when we need to perform routines(函数是在我们需要执行例程时编写的)

3)Contenet of MATLAB built-in Functions(MATLAB内置函数的内容)
edit(which('mean.m'))------------用于打开‘mean’的代码
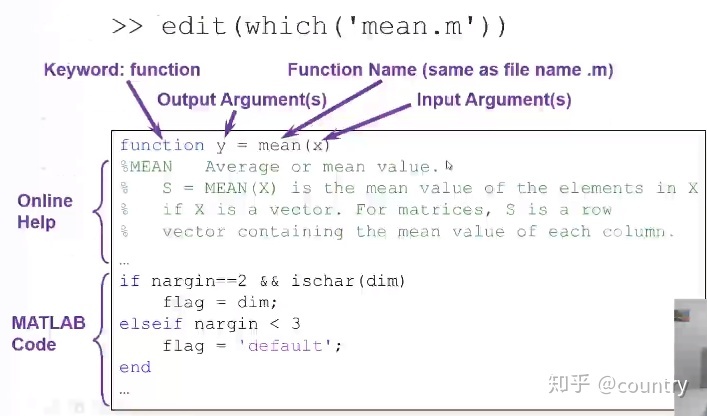
function y = mean(x)
其中,function为keyword,y为output,mean为function name,x为input 。function与script最大的区别就在于function有这个表头
11、User Define Functions(自定义功能)
1)Write a function that calculate the displacement of free falling for given initial displacement
示例代码
function x = freebody(x0,v0,t)
%calculation of free falling
%x0:initial displacement in m
%initial velocity in m/sec
%the elapsed time in sec
%the depth of falling in m
x = x0 + v0 .* t + 1/2 * 9.8 * t .* t;
输入
freebody(0,0,10)
freebody([0 1],[0 1],[10 20])
输出结果为
490
490 1981
注意:
C =A.*B
multiplies arraysA
andB
element by element and returns the result inC
.(C=A.*B将数组A和B逐元素相乘,并以C返回结果。)
所以,用 '.*' 可以同时算多组解
2)Functions with Multiple Inputs and Outputs(多输入和输出功能)
The acceleration of a particle and the force acting on it are as follows:(质点的加速度和作用在质点上的力如下:)
示例代码
function [a F] = acc(v2,v1,t2,t1,m)
a = (v2 - v1) ./ (t2 - t1);
F = m.* a;
注意,保存的文件名称需要与功能块的名称相同!
输入
[ Acc Force ] = acc(20,10,5,4,1)
输出结果
Acc = 10
Force = 10
12、Excise
1)Write a function that asks for a temperature in degrees Fahrenheit( 写一个温度以华氏度为单位的函数,数值由用户提供)
2)Compute the equivalent temperature in degrees Celsius(以摄氏度计算等效温度)
3)Show the converted temperature in degrees Celsius(以摄氏度显示转换温度)
4)The function should keep running until no number is provided to convert(函数应该一直运行,直到没有提供要转换的数字)
5)You may want to use these functions:( 您可能需要使用以下功能:)
input,isempty,break,disp,num2str
公式:
答案代码:
主代码块:
while 1
F = input ('Temperature in F = ');
if isempty(F)
break;
else
transform(F);%(此处谢谢 @Humble 提示修正)
disp(['Temperature in C = ',num2str(transform(F))]);
end
end
功能块代码:
function y = transform(x)
y = (x - 32) * 5 / 9;
结果:
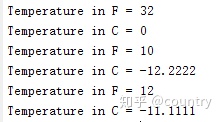
按 CTRL + C 结束程序
注意:
s= num2str(A)
converts a numeric array into a character array that represents the numbers.(将数字数组转换为表示数字的字符数组。)
13、Function Default Variables(函数默认变量)
1)inputname :Variable name of function input(函数输入的变量名)
2)mfilename :File name of currently running function(当前运行函数的文件名)
3)nargin :Number of function input arguments(函数输入参数数目)
4)nargout :Number of function output arguments(函数输出参数数目)
5)varargin :Variable length input argument list(可变长度输入参数列表)
6)varargout :Variable length output argument list(可变长度输出参数列表)
示例代码:
function [volume] = pillar(Do,Di,height)
if nargin == 2;
height = 1;
end
volume = abs(Do .^ 2 - Di .^ 2) .* height * pi / 4;
14、Function Handles(pointer 指针)
1)A way to create anonymous functions,i.e.,one line expression functions that do not have to be defined in .m files(一种创建匿名函数的方法,即不必在.m文件中定义的单行表达式函数)
示例代码:
f = @(x) exp(-2 * x);
x = 0:0.1:2;
plot(x,f(x));
结果
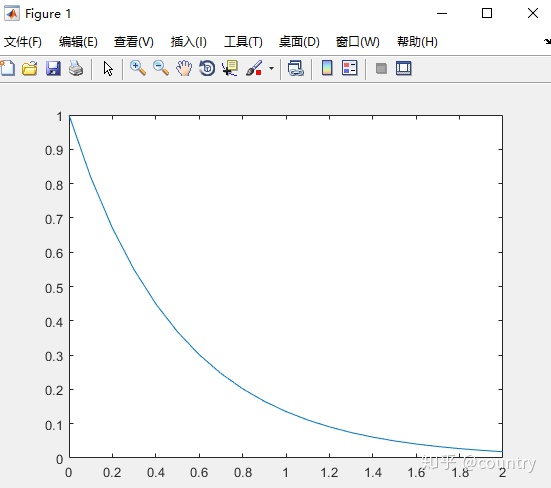
第三节结束