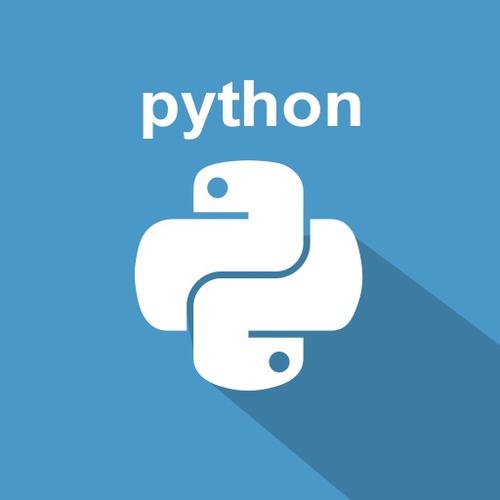
作为存储变量的容器,在Python中存在多种数据类型。
整数 Int、浮点数 Float、布尔值 Bool、字符串 Str、列表 List、元组 Tuple、字典 Dict、集合 Set,除了前三个都是容器类型。
按对象存放在地址的值是否可以被改变分为可变对象和不可变对象
可变对象:字典 Dict, 集合 Set, 列表 List
不可变对象:整型 Int, 字符串 String, 浮点型 Float, 元组 Tuple
按是否可索引分为有序和无序
有序对象:列表 List, 字符串 String, 元组 Tuple
无序对象:字典 Dict, 集合 Set, 整型 Int, 浮点型 Float
知道这些区别对理解不同类型的不同操作方式有帮助。
一、整数 Int、浮点数 Float、布尔值 Bool
- 整数、浮点数和布尔值之间的转换通过int() float() Bool()进行
num1 = 1.5
print(num1, type(num1))
num2 = int(num1)
print(num2, type(num2))
num3 = float(num2)
print(num3, type(num3))
num4 = bool(num3)
print(num4, type(num4))
#输出
# 1.5 <class 'float'>
# 1 <class 'int'>
# 1.0 <class 'float'>
# True <class 'bool'>
二、字符串 Str
字符串类型是有序的容器类型,可以通过下标获取元素或者进行增删改查等操作
1、获取字符个数,len()对于字符串、列表、元组、集合都适用
first_str = "hello world"
#获取字符个数
num = len(first_str)
print(num)
#输出
#11
2、获取指定元素下标
index()和find()两种方法区别在于当元素不存在时使用index()会报错,而find()方法会返回-1
first_str = "hello world"
index_1 = first_str.index("l")
print(index_1)
index_2 = first_str.find("w")
print(index_2)
index_2 = first_str.find("a")
print(index_2)
index_1 = first_str.index("a")
print(index_1)
#输出
# 2
# 6
# -1
# 报错ValueError: substring not found
3、字符串替换、分割
主要使用到replace()进行替换或删除操作,后续应该可以结合正则表达式进行使用
split()实现按指定元素分割字符串,下面的例子中是根据空格分割
first_str = "hello world"
first_str = first_str.replace("world", "python")
print(first_str)
split_str = first_str.split(" ")
print(split_str)
#输出
#hello python
#['hello', 'python']
4、根据下标获取元素
first_str = "hello world"
print(first_str[0])
print(first_str[11])
#输出
#h
#报错 string index out of range
5、字符串遍历和拆包
first_str = "abc"
#遍历
for value in first_str:
print(value)
for index,value in enumerate(first_str):
print(index,value)
#拆包
a, b, c = first_str
print(a, b, c)
#输出
# a
# b
# c
# 0 a
# 1 b
# 2 c
# a b c
6、其他常用字符串方法
first_str = "hello world"
#判断是否以某元素开头或结尾
print(first_str.startswith("h"))
print(first_str.startswith("w"))
print(first_str.endswith("d"))
print(first_str.endswith("o"))
#去除空格
second_str = " a b c "
#去除字符串开头和结尾的空格
print(second_str.strip())
#去除字符串开头的空格
print(second_str.lstrip())
#去除字符串结尾的空格
print(second_str.rstrip())
#使用replace去除所有空格
print(second_str.replace(" ",""))
三、列表 List
列表可以混合存储多种数据类型,以中括号[]表示。
1、获取值和下标,判断值是否在列表中,元素个数
first_list = [1.5, 2, True, "hello python"]
#通过正数下标获取值,从左向右依次为0、1、2 ……
print(first_list[2])
#获取指定元素下标
print(first_list.index(True))
#判断元素是否存在于列表
print(1.5 in first_list)
print(1 in first_list) #理想状态应该返回Flase,不知道为什么这种情况会被判断成True
print(1.1 in first_list)
#通过负数下标获取值,从右向左依次为-1、-2、-3 ……
print(first_list[-2])
#判断元素个数
first_list = [1, 2, True, "hello python", 2, 2]
print(first_list.count(2))
#输出
# True
# 2
# True
# True
# False
# True
# 3
2、增加元素
first_list = [1, 3, True, "hello python"]
#往list最后插入元素
first_list.append(False)
print(first_list)
#插入指定位置,根据下标
first_list.insert(1, 2)
print(first_list)
new_list = ["a", "b"]
#将整个list作为元素插入
first_list = [1, 3, True, "hello python"]
first_list.append(new_list)
print(first_list)
#将list中每个元素当作新元素插入
first_list = [1, 3, True, "hello python"]
first_list.extend(new_list)
print(first_list)
#输出
#[1, 3, True, 'hello python', False]
# [1, 2, 3, True, 'hello python', False]
# [1, 3, True, 'hello python', ['a', 'b']]
# [1, 3, True, 'hello python', 'a', 'b']
3、删除元素
first_list = [1, 3, True, "hello python"]
print(first_list, len())
first_list.remove(3)
print(first_list)
#删除最后一个元素的同时,返回删除的值
item = first_list.pop()
print(first_list, item)
del first_list[0]
print(first_list)
#输出
# [1, 3, True, 'hello python']
# [1, True, 'hello python']
# [1, True] hello python
# [True]
4、修改元素
first_list = [1, 3, True, "hello python"]
print(first_list)
first_list[0] = 2
print(first_list)
#输出
# [1, 3, True, 'hello python']
# [2, 3, True, 'hello python']
5、遍历和拆包
#遍历和拆包
first_list = [1, 3, True, "hello python"]
#遍历
for value in first_list:
print(value)
for index, value in enumerate(first_list):
print(index,value)
#拆包
a, b, c, d = first_list
print(a, b, c, d)1
#输出
# 3
# True
# hello python
# 0 1
# 1 3
# 2 True
# 3 hello python
# 1 3 True hello python
四、元组 Tuple
元组是以小括号()表示的数据集,也可以混合存储多种数据类型,是有序的,可以根据下标获取数据,但是不能通过对其进行修改。元组不支持在原有基础上增加和删除元素。(tuple元组的不可变是指元素对象的引用不可变,不能对其再次赋值,但是在其中可变元素对象的引用不被修改前提下,仍旧可以对可变元素对象修改。引自:https://blog.csdn.net/lzw2016/article/details/85012814)
可变对象:字典 Dict, 集合 Set, 列表 List
不可变对象:整型 Int, 字符串 String, 浮点型 Float, 元组 Tuple
#可变对象和不可变对象
first_list = [1, 3, True, "hello python"]
print(first_list)
first_str = "hello python"
first_list[0] = 2
print(first_list)
first_str[0] = "H"
#输出
# [1, 3, True, 'hello python']
# [2, 3, True, 'hello python']
# 直接修改字符串报错TypeError: 'str' object does not support item assignment
1、根据下标取值、获取元素下标
first_tuple = (1, 2, True, "hello python")
print(first_tuple[1])
print(first_tuple.index(2))
#输出
#2
#1
2、元组中的列表等可变元素可以修改,元组列表的转换
first_tuple = (1, 2, True, "hello python", [1, 2, 3, 4, 5])
print(first_tuple, len(first_tuple))
first_list = list(first_tuple)
print(first_list)
second_tuple = tuple(first_list)
print(second_tuple)
second_tuple[-1][1] = 0
print("修改元组中列表中的元素是可以的:", first_tuple, first_list, second_tuple, sep="n")
#输出
# (1, 2, True, 'hello python', [1, 2, 3, 4, 5]) 5
# [1, 2, True, 'hello python', [1, 2, 3, 4, 5]]
# (1, 2, True, 'hello python', [1, 2, 3, 4, 5])
# 修改元组中列表中的元素是可以的:
# (1, 2, True, 'hello python', [1, 0, 3, 4, 5])
# [1, 2, True, 'hello python', [1, 0, 3, 4, 5]]
# (1, 2, True, 'hello python', [1, 0, 3, 4, 5])
3、遍历和拆包
first_tuple = (1, 2, True, "hello python", [1, 2, 3, 4, 5])
for value in first_tuple:
print(value)
for index, value in enumerate(first_tuple):
print(index, value)
a, b, c, d, e = first_tuple
print(a,b, c, d, e)
#输出
# 1
# 2
# True
# hello python
# [1, 2, 3, 4, 5]
# 0 1
# 1 2
# 2 True
# 3 hello python
# 4 [1, 2, 3, 4, 5]
# 1 2 True hello python [1, 2, 3, 4, 5]
五、字典 Dict
以键值对形式存储的{key: value}, 以大括号表示的
1、增加键值对
first_dict = {"name": "zhangsan", "age": 18, "gender": "male"}
print(first_dict, len(first_dict ))
#增加新的键值对
first_dict["height"] = 175
print(first_dict)
2、删除键值对
first_dict = {"name": "zhangsan", "age": 18, "gender": "male"}
print(first_dict)
#指定删除
del first_dict["age"]
print(first_dict)
first_dict.pop("name")
print(first_dict)
first_dict = {"name": "zhangsan", "age": 18, "gender": "male"}
#随机删除,同时返回删除的值
first_dict.popitem()
print(first_dict)
#输出
# {'name': 'zhangsan', 'age': 18, 'gender': 'male'}
# {'name': 'zhangsan', 'gender': 'male'}
# {'gender': 'male'}
# {'name': 'zhangsan', 'age': 18}
3、修改键值对
first_dict = {"name": "zhangsan", "age": 18, "gender": "male"}
print(first_dict)
first_dict["age"] = 20
print(first_dict)
#输出
# {'name': 'zhangsan', 'age': 18, 'gender': 'male'}
# {'name': 'zhangsan', 'age': 20, 'gender': 'male'}
4、查找键值对
first_dict = {"name": "zhangsan", "age": 18, "gender": "male"}
#通过中括号的方式获取值,如果key不存在程序会报错
print(first_dict["name"])
#通过.get()方式获取值,如果key不存在会返回None,但可以指定不存在时的默认值
print(first_dict.get("name"))
print(first_dict.get("height"))
print(first_dict.get("height", "178"))
#通过中括号的方式获取值,如果key不存在会报错
print(first_dict["height"])
# 输出
# zhangsan
# zhangsan
# None
# 178
# 报错 KeyError: 'height'
5、遍历和拆包
first_dict = {"name": "zhangsan", "age": 18, "gender": "male"}
print(first_dict)
#获取所有key
first_keys = first_dict.keys()
print(first_keys)
#获取所有值
first_values = first_dict.values()
print(first_values)
#遍历key
for key in first_dict:
print(key)
#遍历value
for value in first_dict.values():
print(value)
#同时遍历key, value
for key, value in first_dict.items():
print(key, value)
#拆包
a, b, c = first_dict
print(a, b, c)
a, b, c = first_dict.values()
print(a, b, c)
#输出
# {'name': 'zhangsan', 'age': 18, 'gender': 'male'}
# dict_keys(['name', 'age', 'gender'])
# dict_values(['zhangsan', 18, 'male'])
# name
# age
# gender
# zhangsan
# 18
# male
# name zhangsan
# age 18
# gender male
# name age gender
# zhangsan 18 male
五、集合 Set
以大括号形式表现的数据集合,可存储多种数据类型,元素不可重复。是无序的,没有下标。
1、增加元素
first_set = {1, 3, True, "hello python"}
print(first_set)
first_set.add("hello world")
print(first_set)
#update()参数可以是列表,元组,字典
first_list = [1, 3, False, "hello world"]
print(first_list)
first_set.update(first_list)
print(first_set)
#输出
# {1, 3, 'hello python'}
# {1, 3, 'hello world', 'hello python'}
# [1, 3, False, 'hello world']
# {False, 1, 3, 'hello world', 'hello python'}
2、删除元素
first_set = {1, 3, True, "hello python", "hello world"}
print(first_set)
#随机删除
first_set.pop()
print(first_set)
# 不存在不会报错
first_set.discard("hello python")
print(first_set)
first_set.discard("hello python")
print(first_set)
# 不存在会报错
first_set.remove("hello world")
print(first_set)
first_set.remove("hello world")
#输出
# {1, 3, 'hello world', 'hello python'}
# {3, 'hello world', 'hello python'}
# {3, 'hello world'}
# {3, 'hello world'}
# {3}
# KeyError: 'hello world'
3、判断是否存在
first_set = {1, 3, True, "hello python", "hello world"}
print(first_set)
#判断集合中是否存在某某元素
print( "hello python" in first_set)
4、遍历和拆包
first_set = {1, 3, True, "hello python"}
#遍历
for value in first_set:
print(value)
for index,value in enumerate(first_set):
print(index, value)
#拆包
a, b, c= first_set
print(a, b, c)
#输出
# 1
# 3
# hello python
# 0 1
# 1 3
# 2 hello python
# 1 3 hello python