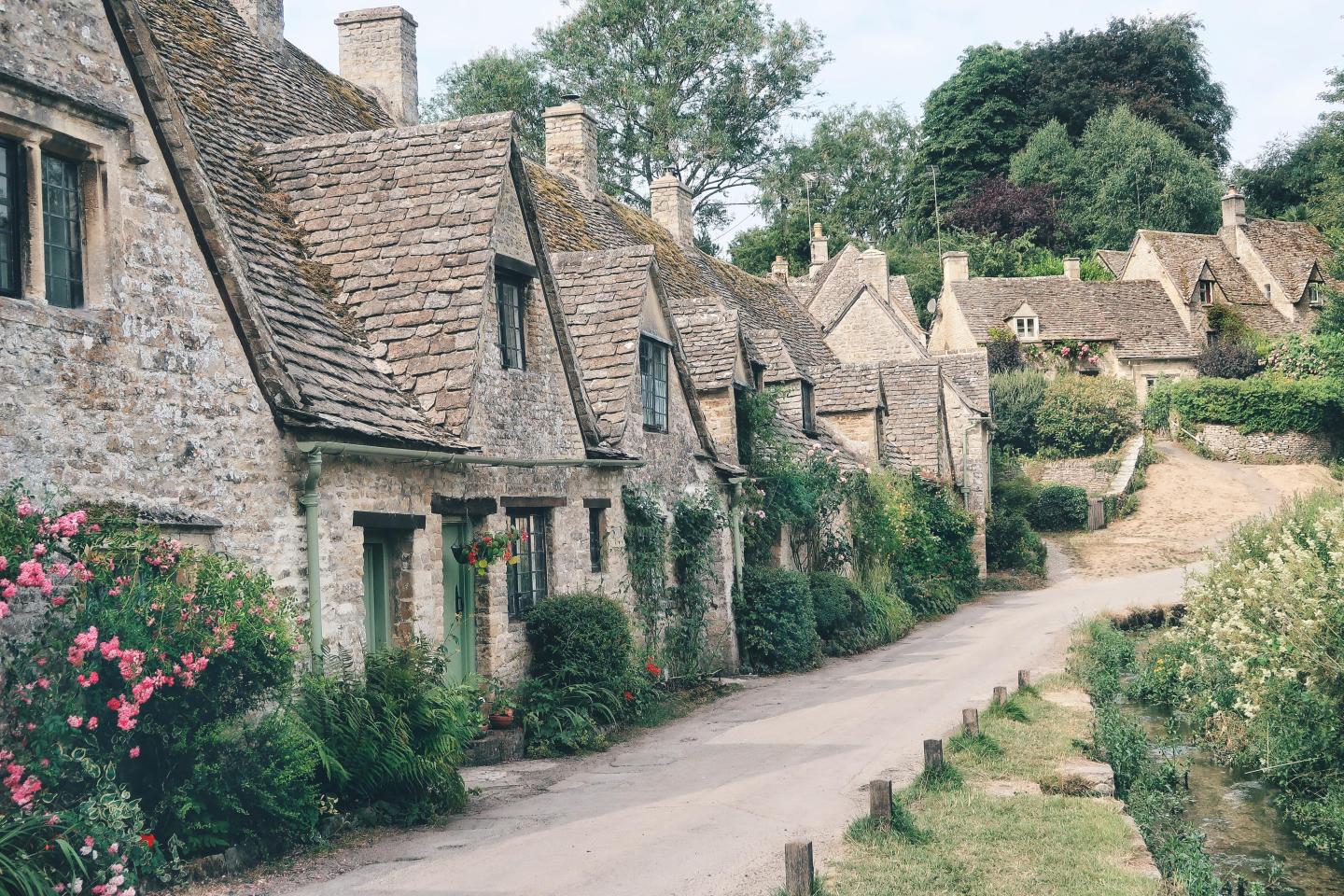
1.前言
JaveEE要学好,主要包括这么几个大部分:
- java基础编程 基础编程 面向对象 集合 IO 线程
- MySQL或者其他数据库
- Web前端
- JavaWeb jsp+servlet
- SSH Struts Hibernate Springs
- SSM Mybatis Spring SpringMvc
- 项目实战
2.说明:本篇整理了基础java的13个重要知识点,详细内容请参考其他网站
话不多说,开始第一部分:
@13个基础的重点知识点
- 推荐使用的编辑工具:IDEA
1.java中的数据类型:数据的单位
基本数据类型:程序里面自带的数据类型
布尔类型:boolean *:true/false
字符类型:char *: Unciode编码 -》 不区分中英文
统一的都是2个字节 = 16个位
取值范围:0-65535
char x = 'a';
char y = '男';
char z = 55;//范围:0-65535
整数类型:byte short int long -> int*
浮点类型:float double -> 小数 double*
重点:boolean char int/long double
boolean -> 判断
char -> 一个字、一个字母
int/long -> 整数
double -> 小数
2.基本数据类型之间的转换:
取值范围:小 -》 大
char
byte short int long float double
小的变大的 直接转变
int x = 45;
double y = x;
大的变小的 需要强转:
double x = 45.5;
int y = (int)x;
3.引用数据类型 程序员自己开发的数据类型
main:
创建对象:
Student stu = new Student();
对属性赋值:
stu.name = "张三";
stu.gender = '男';
stu.stuId = 12345;
stu.study();//学习
//char x = stu.showGender();
System.out.println(stu.showGender());
main:
Student stu = new Student();
System.out.println(stu.name.length());
class Student{
String name;//null
char gender;//
int stuId;//0
public void study(){
System.out.println("学习");
}
public char showGender(){
int x = 45;
return gender;
}
}
4.java中的变量: 分类: 成员变量 属性 实例变量:定义在类体里面 局部变量 自动变量:定义在方法体里面
区别:
1:定义的位置不同
2:作用范围不同
成员变量:依赖于对象存在
Student stu = new Student();//name gender stuId创建
局部变量:依赖于方法存在
stu.XXX();里面的局部变量创建
3:默认值不同:
成员变量:即使不赋值 也有默认值
局部变量:没有默认值 要求在使用之前必须先赋值
5.java中的分支/判断: if else:*
if(boolean判断){
执行语句;
}else if(boolean判断){
执行语句;
}else if(boolean判断){
执行语句;
}
if(x % 2 == 0){
return "偶数";
}else{
return "奇数";
}
-》
if(x % 2 == 0)
return "偶数";
return "奇数";
if(x % 2 == 0){
return true;
}else{
return false;
}
->
return x % 2 == 0;
只要两个学生的姓名一样就是为相等对象
public boolean equals(Object obj){
if(obj == null)return false;
if(!(obj instanceof Student))return false;
if(obj == this)return true;
return this.name.equals(((Student)obj).name));
}
=====================================
switch case:
switch(参数){
case XX : 执行语句;
case YY : 执行语句;
default : 执行语句;
}
参数只能传某几种数据类型:
jdk1.0 char byte short int
jdk5.0 enum
jdk7.0 String
System.out.println("请选择A/B/C/D");
Scanner sc = new Scanner(System.in);
String ABCD = sc.next();
switch(ABCD){
case "A" : 语法操作1;break;
case "B" : 语法操作1;break;
case "C" : 语法操作1;break;
case "D" : 语法操作1;break;
default : 语法操作1;
}
6.java中的循环:
for:
for(变量初始化;循环条件;循环之后的变化){
循环执行的代码
}
for(int x = 1;x <= 100;x++){
if(x % 8 == 0){
System.out.println(x);
}
}
while:
变量初始化;
while(循环条件){
执行语句;
循环之后的变化;
}
int x = 1;
while(x <= 100){
if(x % 8 == 0){
System.out.println(x);
}
x++;
}
do while:
int x = 1;
do{
if(x % 8 == 0){
System.out.println(x);
}
x++;
}while(x <= 100);
7.数组: 基本概念:数组是容器 装元素 类型相同 存储空间连续
int[] data = new int[5];//0 0 0 0 0
int[] data = new int[]{45,77,82,19,90};
int[] data = {56,77,28,99};
得到某一个元素:data[下标] 从0开始
得到数组大小:data.length
str.length()
list.size()
遍历数组:
for(int x = 0;x < data.length - 1;x++){
System.out.println(data[x]);
}
for(int x : data){
System.out.println(x);
}
数组的复制1:
int[] data = {56,77,28,99};
往数组里面添加元素:
int[] temp = new int[data.length+1];//0 0 0 0 0 0
System.arraycopy(data,0,temp,0,4);//56 77 28 99 0
temp[temp.length - 1] = 66;
data = temp;
数组的复制2:
int[] data = {44,55,66,77,88};
data = Arrays.copyOf(data,data.length+1);
数组排序:
自动排序:Arrays.sort(数组对象);
只能升序 import java.util.*;
手动排序 冒泡排序:
int[] data = {56,88,37,40,99};
for(int x = 0;x < data.length - 1;x++){//次数
for(int y = 0;y < data.length - 1;y++){//下标
if(data[y] > daya[y + 1]){
int z = data[y];
data[y] = data[y + 1];
data[y + 1] = z;
}
}
}
8.封装:用private修饰属性和方法
main:
Student stu = new Student();
//stu.name = "";
stu.setName("张三");
System.out.println(stu.getName());
class Student{
private String name;
//setter方法修改值
public void setName(String name){
this.name = name;
}
//getter方法得到值:
public String getName(){
return name;
}
}
9.继承:用extends实现两个类之间的is a的关系
共享代码
class Person{
String name;
int age;
char gender;
}
class Student extends Person{}
10多态:一个对象总有不同的类型去定义它
1:创建对象
Person x = new Student();
2:放在参数里面解耦
public void wc(Student stu){XXX}
public void wc(Teacher tea){XXX}
public void wc(Doctor x){XXX}
->
public void wc(Person x){}
-> Object类里面有一个方法判断两个对象能不能视为相等对象
1.equals(Object obj)
11.方法重载 Overload:
条件?
是否发生在同一个类体中
方法名是否一模一样
参数是否不同【类型不同 个数不同 顺序不同】
String:
substring(int 下标):该下标截取到最后
substring(int x,int y):从下标x截取到下标y-1
ArrayList:
remove(int 下标);
remove(Object 元素)
作用
同时满足用户的不同需求
12方法覆盖 Override
条件?
子类在继承得到父类的某些方法之后 不满意 在子类里面重新实现一下
子类权限 >= 父类权限
返回类型
jdk5.0之前 一模一样
jdk5.0开始 斜变返回类型
方法签名 一模一样
异常 <= 父类的异常
父类:
protected Object clone(){XXX}
子类:
public Object/Object子类 clone(){}
13.构造方法
每个类都有构造方法 我们不写 系统提供一个默认构造方法
//Student stu = new Student();
Student stu = new Student("张三",34);
stu.setAge(45);
class Student{
String name;
int age;
public Student(String name,int age){
this.name = name;
this.age = age;
}
//public Student(){}
}
构造方法的首行:
super():表示要执行本构造方法之前 先去执行父类的构造方法
class Father{
String name;
int age;
public Father(String name,int age){
this.name = name;
this.age = age;
}
public void showDay(){
System.out.println("吃饭");
System.out.println("看电视");
System.out.println("砍树");
}
}
class Son extends Father{
//name age
int stuId;
public Son(String name,int age,int stuId){
super(name,age);//只能放在构造方法的首行 共享父类构造方法的代码
this.stuId = stuId;
}
@Override
public void showDay(){
super.showDay();//放在普通方法里面任何一行 共享父类普通方法的代码
System.out.println("打游戏");
}
}
this():要执行本构造方法之前 先去执行本类的其他的构造方法
class Student{
String name;
int age;
int stuId;//成员变量
public Student(String name,int age){
this.name = name;
this.age = age;
}
public Student(String name,int age,int stuId){
this(name,age);//放在构造方法首行 共享本类其他构造方法的代码
this.stuId = stuId;
}
public void show(){
int stuId = 12345;//局部变量
System.out.println(stuId);
//this.表示当前调用该方法的对象 没有位置限制
System.out.println(this.stuId);
}
}