创建
工作目录下
安装angular工具
npm i @angular/cli --save-dev
创建项目
npx ng new first-demo
启动
cd
npx ng serve
http://localhost:4200 打开
--------------
创建组件
npx ng generate component sub
app.component.ts
import { Component } from '@angular/core';
// enum IMG = {
// A=qq,
// B=wx
// }
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'app';
// myclass="div2";
myclass= {
div2:true,
div1:false
};
mystyle={
"background-color":"yellow",
"border":"1px solid black"
};
// imgType:IMG = IMG.A;
myimgsrc = "../assets/qq.jpg";
// changeImg(){
// this.imgType = this.imgType === IMG.A ?IMG.B :IMG.A;
// this.myimgsrc = `../assets/${this.imgType}.jpg`;
// };
myhandle(){
this.title +="-----";
}
}
.html
<div [ngClass]="myclass"> {{ title }} </div>
<app-sub (myclick)="myhandle()"></app-sub>
<h2 [ngStyle]="mystyle">my style</h2>
<img [src]="myimgsrc" alt="" (click)= "changeImg()">
.css
.div2{
color:red;
}
.div1{
color:blue;
}
app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
import { SubComponent } from './sub/sub.component';
@NgModule({
declarations: [
AppComponent,
SubComponent
],
imports: [
BrowserModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
子组件
sub....ts
import { Component, OnInit, EventEmitter } from '@angular/core';
@Component({
selector: 'app-sub',
templateUrl: './sub.component.html',
styleUrls: ['./sub.component.css'],
inputs:["mybody"],
outputs:["myclick"]
})
export class SubComponent{
mybody = "wss";
myjson = {name:"wss",age:"22",group:"node.js"}
myclick = new EventEmitter();
mydate = new Date("2008-10-10");
constructor() { }
add(){
this.mybody += "x";
this.myclick.emit();
}
}
.html
<p>
sub works!
</p>
<button (click)="add()"> add</button>
<h1>{{ mybody | uppercase }}</h1>
<h2>{{ myjson | json }}</h2>
<h3>{{ mydate | date:shortDate }}</h3>
<!-- 管道 -->
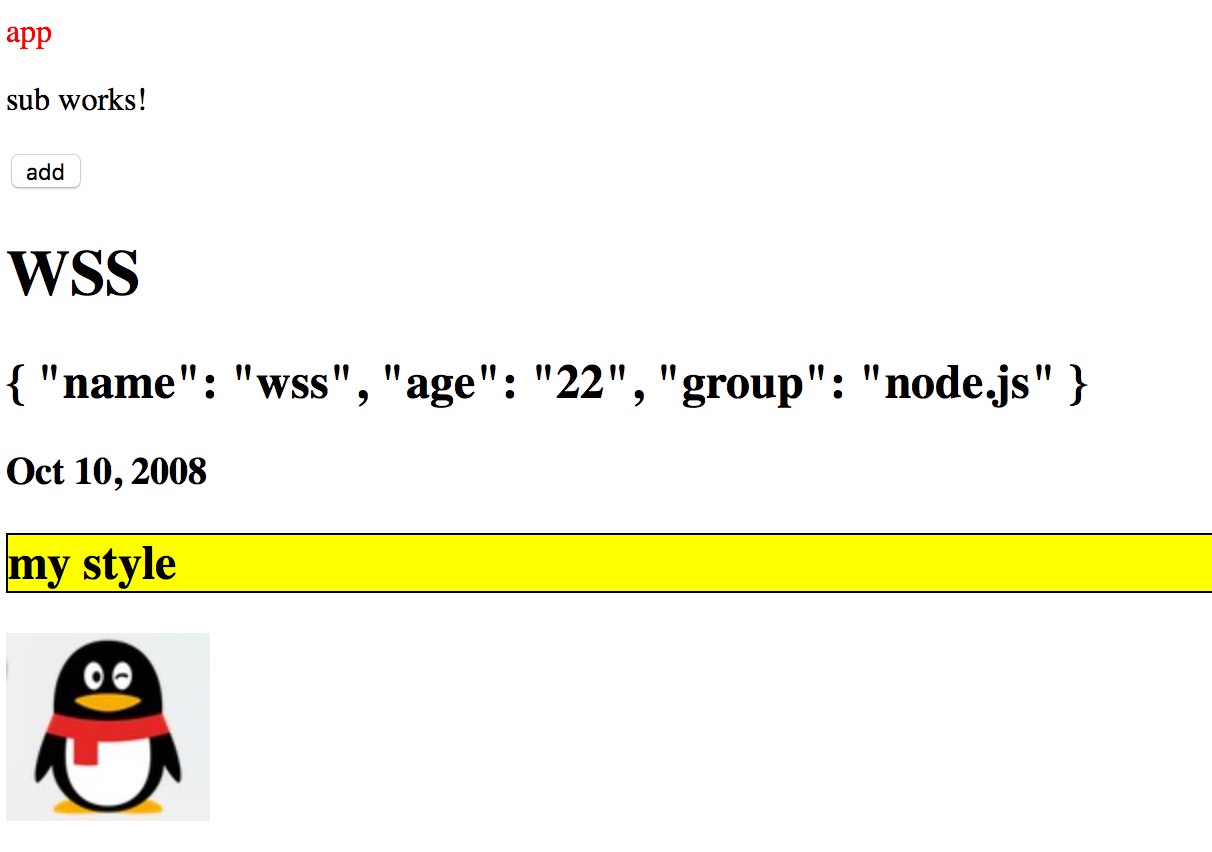
if for switch用法例子
<p>
sub works!
</p>
<!-- 单选项 []属性指令 *是结构性指令 -->
<div [ngSwitch]="abc">
<p *ngSwitchCase="'a'">aaa</p>
<p *ngSwitchCase="'b'">bbb</p>
<p *ngSwitchCase="'c'">ccc</p>
<p *ngSwitchDefault>default</p>
</div>
<p>开关</p>
<h4 *ngIf = "showTitle"> mytitle </h4>
<button type="button" name="button" (click)="showTitle = !showTitle">toggle</button>
<p>for</p>
<ul>
<li *ngFor="let item of list;let i = index;" [ngStyle]="{'background-color':i===1?'red':none}">{{ i }}-{{ item.name }}</li>
</ul>
<div *ngIf="s1">
<div *ngIf="s2">
@#$%^111
</div>
</div>
<button (click)="add()"> add</button>
<h1>{{ mybody | uppercase }}</h1>
<h2>{{ myjson | json }}</h2>
<h3>{{ mydate | date:shortDate }}</h3>
<!-- 管道 -->
import { Component, OnInit, EventEmitter } from '@angular/core';
@Component({
selector: 'app-sub',
templateUrl: './sub.component.html',
styleUrls: ['./sub.component.css'],
inputs:["mybody"],
outputs:["myclick"]
})
export class SubComponent{
abc = "b";
showTitle = true;
// mytitle = "sub title";
s1=true;
s2= true;
mybody = "wss";
myjson = {name:"wss",age:"22",group:"node.js"};
list=[
{name:"ww"},
{name:"ss"},
{name:"xx"}
]
myclick = new EventEmitter();
mydate = new Date("2008-10-10");
constructor() { }
add(){
this.mybody += "x";
this.myclick.emit();
}
}
http
app.module.ts
import { HttpClientModule } from "@angular/common/http";
imports: [
BrowserModule,
FormsModule,
HttpClientModule
],
app.component.ts:
import{HttpClient} from '@angular/common/http';
使用json数据
assets/mydata.json
{
"name":"hahahha"
}
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import {FormsModule}from "@angular/forms";
import { AppComponent } from './app.component';
import { SubComponent } from './sub/sub.component';
import { MyformComponent } from './myform/myform.component';
import { HttpClientModule } from "@angular/common/http";
@NgModule({
declarations: [
AppComponent,
SubComponent,
MyformComponent
],
imports: [
BrowserModule,
FormsModule,
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
<!--The content below is only a placeholder and can be replaced.-->
<app-myform></app-myform>
<hr>
<button type="button" name="button" (click)="getData()"> {{buttonName}}</button>
<hr>
<div [ngClass]="myclass"> {{ title }} </div>
<app-sub (myclick)="myhandle()"></app-sub>
<h2 [ngStyle]="mystyle">my style</h2>
<img [src]="myimgsrc" alt="" (click)= "changeImg()">
<!-- <div style="text-align:center">
<h1>
Welcome to {{ title }}!
</h1>
<img width="300" alt="Angular Logo" src="data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHZpZXdCb3g9IjAgMCAyNTAgMjUwIj4KICAgIDxwYXRoIGZpbGw9IiNERDAwMzEiIGQ9Ik0xMjUgMzBMMzEuOSA2My4ybDE0LjIgMTIzLjFMMTI1IDIzMGw3OC45LTQzLjcgMTQuMi0xMjMuMXoiIC8+CiAgICA8cGF0aCBmaWxsPSIjQzMwMDJGIiBkPSJNMTI1IDMwdjIyLjItLjFWMjMwbDc4LjktNDMuNyAxNC4yLTEyMy4xTDEyNSAzMHoiIC8+CiAgICA8cGF0aCAgZmlsbD0iI0ZGRkZGRiIgZD0iTTEyNSA1Mi4xTDY2LjggMTgyLjZoMjEuN2wxMS43LTI5LjJoNDkuNGwxMS43IDI5LjJIMTgzTDEyNSA1Mi4xem0xNyA4My4zaC0zNGwxNy00MC45IDE3IDQwLjl6IiAvPgogIDwvc3ZnPg==">
</div>
<h2>Here are some links to help you start: </h2>
<ul>
<li>
<h2><a target="_blank" rel="noopener" href="https://angular.io/tutorial">Tour of Heroes</a></h2>
</li>
<li>
<h2><a target="_blank" rel="noopener" href="https://github.com/angular/angular-cli/wiki">CLI Documentation</a></h2>
</li>
<li>
<h2><a target="_blank" rel="noopener" href="https://blog.angular.io/">Angular blog</a></h2>
</li>
</ul> -->
import { Component } from '@angular/core';
import{HttpClient} from '@angular/common/http';
// enum IMG = {
// A=qq,
// B=wx
// }
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private http:HttpClient){
}
getData(){
this.http.get("assets/mydata.json").subscribe((result:any)=>this.buttonName=result.name)
}
buttonName ="更改button名称";
title = 'app';
// myclass="div2";
myclass= {
div2:true,
div1:false
};
mystyle={
"background-color":"yellow",
"border":"1px solid black"
};
// imgType:IMG = IMG.A;
myimgsrc = "../assets/qq.jpg";
// changeImg(){
// this.imgType = this.imgType === IMG.A ?IMG.B :IMG.A;
// this.myimgsrc = `../assets/${this.imgType}.jpg`;
// };
myhandle(){
this.title +="-----";
}
ngOnInit(){}
}
route
import{RouterModule,Routes}from '@angular/router';
const appRoutes:Routes=[{path, component},...];
import :[RouterModule.forRoot(appRoutes)] 加入模块
固定用法
程序中
<router-outlet></router-outlet>
路由链接
<a routerLink="/a">aa</a>
app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import{RouterModule,Routes}from '@angular/router';
import {FormsModule}from "@angular/forms";
import { AppComponent } from './app.component';
import { SubComponent } from './sub/sub.component';
import { MyformComponent } from './myform/myform.component';
import { HttpClientModule } from "@angular/common/http";
import { AComponent } from './a/a.component';
import { BComponent } from './b/b.component';
import { CComponent } from './c/c.component';
const routes:Routes =[
{path:"a",component:AComponent},
{path:"b",component:BComponent},
{path:"c",component:CComponent}
]
@NgModule({
declarations: [
AppComponent,
SubComponent,
MyformComponent,
AComponent,
BComponent,
CComponent
],
imports: [
BrowserModule,
FormsModule,
HttpClientModule,
RouterModule.forRoot(routes)
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
<a routerLink="/a">aaa</a>
<a routerLink="/b">bbb</a>
<a routerLink="/c">ccc</a>
<router-outlet></router-outlet>