showwidget
showwidget.h
#ifndef SHOWWIDGET_H
#define SHOWWIDGET_H
#include <QWidget>
#include <QLabel>
#include <QTextEdit>
#include <QImage>
class showwidget : public QWidget
{
Q_OBJECT
public:
showwidget(QWidget *parent = nullptr);
~showwidget();
QImage* Img;
QLabel* ImageLabel;
QTextEdit* Text;
};
#endif
}
showwidget::~showwidget() {}
showwidget.cpp
#include "showwidget.h"
#include <QHBoxLayout>
showwidget::showwidget(QWidget *parent)
: QWidget(parent)
{
ImageLabel = new QLabel;
ImageLabel->setScaledContents(true);
Text = new QTextEdit;
QHBoxLayout* MainLayout = new QHBoxLayout(this);
MainLayout->addWidget(ImageLabel);
MainLayout->addWidget(Text);
}
showwidget::~showwidget() {}
processor
processor.h
#ifndef PROCESSOR_H
#define PROCESSOR_H
#include <QMainWindow>
#include <QImage>
#include <QLabel>
#include <QMenu>
#include <QMenuBar>
#include <QAction>
#include <QComboBox>
#include <QSpinBox>
#include <QFontComboBox>
#include <QToolButton>
#include <QTextCharFormat>
#include <QFileDialog>
#include <QTextStream>
#include <QFile>
#include <QDebug>
#include "showwidget.h"
#include <QPrintDialog>
#include <QPrinter>
#include <QPainter>
#include <QTransform>
class Processor : public QMainWindow
{
Q_OBJECT
public:
Processor(QWidget *parent = nullptr);
~Processor();
void CreateActions();
void CreateMenus();
void CreateToolBars();
void LoadFile(QString tFileName);
void MergeFormat(QTextCharFormat);
private:
showwidget* ShowWidget;
QMenu* FileMenu;
QMenu* ZoomMenu;
QMenu* RotateMenu;
QMenu* MirrorMenu;
QImage Img;
QString FileName;
QAction* OpenFileAction;
QAction* NewFileAction;
QAction* ExitAction;
QAction* PrintTextAction;
QAction* PrintImageAction;
QAction* EditAction;
QAction* CopyAction;
QAction* CutAction;
QAction* PastAction;
QAction* AboutAction;
QAction* ZoomInAction;
QAction* ZoomOutAction;
QAction* Rotate90Action;
QAction* Rotate180Action;
QAction* Rotate270Action;
QAction* MirrorVerticalAction;
QAction* MirrorHorizontalAction;
QAction* UndoAction;
QAction* RedoAction;
QToolBar* FileTool;
QToolBar* ZoomTool;
QToolBar* RotateTool;
QToolBar* MirrorTool;
QToolBar* DoToolBar;
signals:
protected slots:
void ShowNewFile();
void ShowOpenFile();
void ShowPrintImage();
void ShowZoomIn();
void ShowZoomOut();
void ShowRotate90();
void ShowRotate180();
void ShowRotate270();
void ShowMirrorVertical();
void ShowMirrorHorizontal();
};
#endif
processor.cpp
#include "processor.h"
#include <QApplication>
#include <QToolBar>
Processor::Processor(QWidget *parent)
: QMainWindow{parent}
{
setWindowTitle(tr("Easy Word"));
ShowWidget = new showwidget(this);
setCentralWidget(ShowWidget);
CreateActions();
CreateMenus();
CreateToolBars();
if(Img.load("text.png"))
{
ShowWidget->ImageLabel->setPixmap(QPixmap::fromImage(Img));
}
}
Processor::~Processor()
{
}
void Processor::CreateActions()
{
OpenFileAction = new QAction(QApplication::style()->standardIcon(QStyle::StandardPixmap(42))
,tr("打开"),this);
OpenFileAction->setShortcut(tr("Ctrl+O"));
OpenFileAction->setStatusTip(tr("打开一个文件"));
connect(OpenFileAction,&QAction::triggered,this,&Processor::ShowOpenFile);
NewFileAction = new QAction(QApplication::style()->standardIcon(QStyle::StandardPixmap(32))
,tr("新建"),this);
NewFileAction->setShortcut(tr("Ctrl+N"));
NewFileAction->setStatusTip(tr("新建一个文件"));
connect(NewFileAction,SIGNAL(triggered()),this,SLOT(ShowNewFile()));
ExitAction = new QAction(QApplication::style()->standardIcon(QStyle::StandardPixmap(11))
,tr("退出"),this);
ExitAction->setShortcut(tr("Ctrl+Q"));
ExitAction->setStatusTip(tr("退出程序"));
connect(ExitAction,SIGNAL(triggered()),this,SLOT(close()));
CopyAction = new QAction(QApplication::style()->standardIcon(QStyle::StandardPixmap(21))
,tr("复制"),this);
CopyAction->setShortcut(tr("Ctrl+C"));
CopyAction->setStatusTip(tr("复制文件"));
CutAction = new QAction(QApplication::style()->standardIcon(QStyle::StandardPixmap(22))
,tr("剪切"),this);
CutAction->setShortcut(tr("Ctrl+X"));
CutAction->setStatusTip(tr("剪切文件"));
PastAction = new QAction(QApplication::style()->standardIcon(QStyle::StandardPixmap(22))
,tr("粘贴"),this);
PastAction->setShortcut(tr("Ctrl+V"));
PastAction->setStatusTip(tr("粘贴文件"));
AboutAction = new QAction(tr("关于"),this);
connect(AboutAction,&QAction::triggered,this,[=]() { QApplication::aboutQt(); });
PrintTextAction = new QAction(tr("打印文本"),this);
PrintTextAction->setStatusTip(tr("打印一个文本"));
PrintImageAction = new QAction(tr("打印图片"),this);
PrintImageAction->setStatusTip(tr("打印一个图片"));
connect(PrintImageAction,&QAction::triggered,this,&Processor::ShowPrintImage);
ZoomInAction = new QAction(tr("放大动作"),this);
ZoomInAction->setStatusTip(tr("放大一幅图片"));
connect(ZoomInAction,&QAction::triggered,this,&Processor::ShowZoomIn);
ZoomOutAction = new QAction(tr("缩小动作"),this);
ZoomOutAction->setStatusTip(tr("缩小一幅图片"));
connect(ZoomOutAction,&QAction::triggered,this,&Processor::ShowZoomOut);
Rotate90Action = new QAction(tr("旋转90"),this);
Rotate90Action->setStatusTip(tr("将一幅图片旋转90"));
connect(Rotate90Action,&QAction::triggered,this,&Processor::ShowRotate90);
Rotate180Action = new QAction(tr("旋转180"),this);
Rotate180Action->setStatusTip(tr("将一幅图片旋转180"));
connect(Rotate180Action,&QAction::triggered,this,&Processor::ShowRotate180);
Rotate270Action = new QAction(tr("旋转270"),this);
Rotate270Action->setStatusTip(tr("将一幅图片旋转270"));
connect(Rotate270Action,&QAction::triggered,this,&Processor::ShowRotate270);
MirrorVerticalAction = new QAction(tr("纵向图片"),this);
MirrorVerticalAction->setStatusTip(tr("将一幅图片纵向"));
connect(MirrorVerticalAction,&QAction::triggered,this,&Processor::ShowMirrorVertical);
MirrorHorizontalAction = new QAction(tr("横向图片"),this);
MirrorHorizontalAction->setStatusTip(tr("将一幅图片横向"));
connect(MirrorHorizontalAction,&QAction::triggered,this,&Processor::ShowMirrorHorizontal);
UndoAction =new QAction(tr("撤销"),this);
connect(UndoAction,SIGNAL(triggered()),ShowWidget->Text,SLOT(undo()));
RedoAction =new QAction(tr("重做"),this);
connect(RedoAction,SIGNAL(triggered()),ShowWidget->Text,SLOT(redo()));
}
void Processor::CreateMenus()
{
FileMenu = menuBar()->addMenu(tr("文件"));
FileMenu->addAction(OpenFileAction);
FileMenu->addAction(NewFileAction);
FileMenu->addAction(PrintTextAction);
FileMenu->addAction(PrintImageAction);
FileMenu->addSeparator();
FileMenu->addAction(ExitAction);
ZoomMenu = menuBar()->addMenu(tr("编辑"));
ZoomMenu->addAction(CopyAction);
ZoomMenu->addAction(CutAction);
ZoomMenu->addAction(PastAction);
ZoomMenu->addAction(AboutAction);
ZoomMenu->addSeparator();
ZoomMenu->addAction(ZoomInAction);
ZoomMenu->addAction(ZoomOutAction);
RotateMenu = menuBar()->addMenu(tr("旋转"));
RotateMenu->addAction(Rotate90Action);
RotateMenu->addAction(Rotate180Action);
RotateMenu->addAction(Rotate270Action);
MirrorMenu = menuBar()->addMenu(tr("镜像"));
MirrorMenu->addAction(MirrorVerticalAction);
MirrorMenu->addAction(MirrorHorizontalAction);
}
void Processor::CreateToolBars()
{
FileTool = addToolBar("File");
FileTool->addAction(OpenFileAction);
FileTool->addAction(NewFileAction);
FileTool->addAction(PrintTextAction);
FileTool->addAction(PrintImageAction);
ZoomTool = addToolBar("Edit");
ZoomTool->addAction(CopyAction);
ZoomTool->addAction(CutAction);
ZoomTool->addAction(PastAction);
ZoomTool->addSeparator();
ZoomTool->addAction(ZoomInAction);
ZoomTool->addAction(ZoomOutAction);
RotateTool = addToolBar("Rotate");
RotateTool->addAction(Rotate90Action);
RotateTool->addAction(Rotate180Action);
RotateTool->addAction(Rotate270Action);
RotateTool->addSeparator();
RotateTool->addAction(UndoAction);
RotateTool->addAction(RedoAction);
}
void Processor::LoadFile(QString tFileName)
{
qDebug()<< "filename:" << tFileName;
QFile File(tFileName);
if(File.open(QIODevice::ReadOnly|QIODevice::Text))
{
QTextStream TextStream(&File);
while(!TextStream.atEnd())
{
ShowWidget->Text->append(TextStream.readLine());
printf("read line\n");
}
qDebug()<< "end\n";
}
}
void Processor::MergeFormat(QTextCharFormat)
{
}
void Processor::ShowNewFile()
{
Processor* NewProcessor = new Processor;
NewProcessor->show();
}
void Processor::ShowOpenFile()
{
FileName = QFileDialog::getOpenFileName(this);
if(!FileName.isEmpty())
{
if(ShowWidget->Text->document()->isEmpty())
{
LoadFile(FileName);
}
else
{
Processor* NewProcessor = new Processor;
NewProcessor->show();
NewProcessor->LoadFile(FileName);
}
}
}
void Processor::ShowPrintImage()
{
QPrinter Printer;
QPrintDialog PrintDialog(&Printer,this);
if(PrintDialog.exec())
{
QPainter Painter(&Printer);
QRect Rect =Painter.viewport();
QSize Size =Img.size();
Size.scale(Rect.size(),Qt::KeepAspectRatio);
Painter.setViewport(Rect.x(),Rect.y(),Rect.width(),Rect.height());
Painter.setWindow(Img.rect());
Painter.drawImage(0,0,Img);
}
}
void Processor::ShowZoomIn()
{
if(Img.isNull())
{
return;
}
QTransform transform;
transform.scale(2, 2);
Img = Img.transformed(transform);
ShowWidget->ImageLabel->setPixmap(QPixmap::fromImage(Img));
}
void Processor::ShowZoomOut()
{
if(Img.isNull())
{
return;
}
QTransform transform;
transform.scale(0.5, 0.5);
Img = Img.transformed(transform);
ShowWidget->ImageLabel->setPixmap(QPixmap::fromImage(Img));
}
void Processor::ShowRotate90()
{
if(Img.isNull())
{
return;
}
QTransform transform;
transform.rotate(90);
Img = Img.transformed(transform);
ShowWidget->ImageLabel->setPixmap(QPixmap::fromImage(Img));
}
void Processor::ShowRotate180()
{
if(Img.isNull())
{
return;
}
QTransform transform;
transform.rotate(180);
Img = Img.transformed(transform);
ShowWidget->ImageLabel->setPixmap(QPixmap::fromImage(Img));
}
void Processor::ShowRotate270()
{
if(Img.isNull())
{
return;
}
QTransform transform;
transform.rotate(270);
Img = Img.transformed(transform);
ShowWidget->ImageLabel->setPixmap(QPixmap::fromImage(Img));
}
void Processor::ShowMirrorVertical()
{
if(Img.isNull())
{
return;
}
Img = Img.mirrored(false,true);
ShowWidget->ImageLabel->setPixmap(QPixmap::fromImage(Img));
}
void Processor::ShowMirrorHorizontal()
{
if(Img.isNull())
{
return;
}
Img = Img.mirrored(true,false);
ShowWidget->ImageLabel->setPixmap(QPixmap::fromImage(Img));
}
main.cpp
#include "processor.h"
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
Processor w;
w.show();
return a.exec();
}
运行图
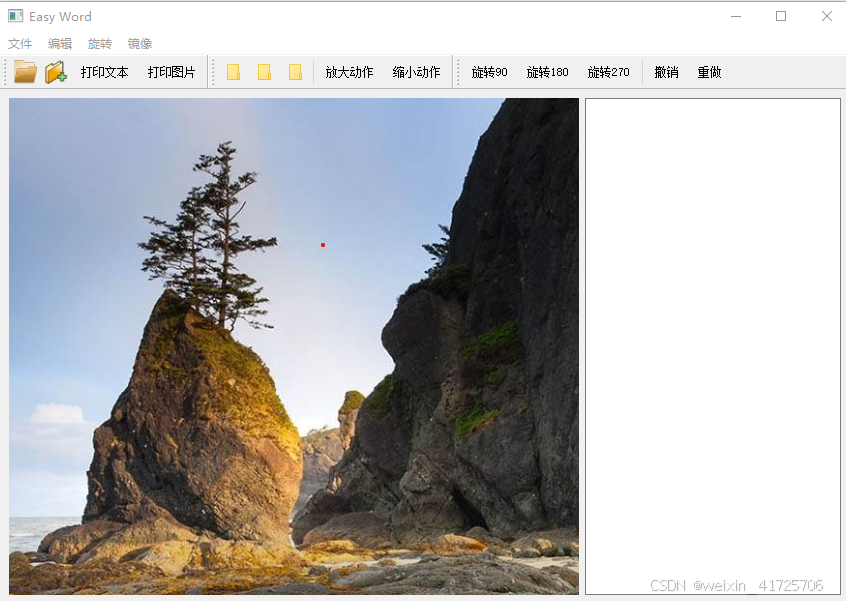