iOS模仿微信滑块动态设置字体大小的功能
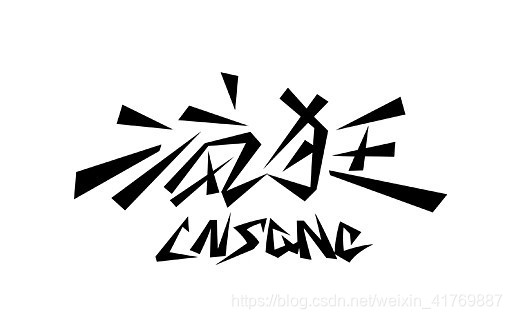
首先说一下实现这一功能的核心类别,UITableViewCell+FSAutoCountHeight。这个类其实是利用运行时将每个cell高度通过子控件的约束动态加以控制进而返回一个值,这个值就是我们要在tableView:(UITableView*)tableView cellForRowAtIndexPath:(NSIndexPath*)indexPath中要返回的值,那么有朋友要问了这个数据肯定是从后台来的,如果数据很多的时候在cell自身重用机制的因素下会不会受到影响?当然这层是考虑到的,FSCellHeightForTableView:(UITableView*)tableView indexPath:(NSIndexPath*)indexPath cacheKey:(NSString*)cacheKey cellContentViewWidth:(CGFloat)contentViewWidth bottomOffset:(CGFloat)bottomOffset这个方法其实就是通过cacheKey进而进行对cell高度缓存的策略,cacheKey如何取呢,举个栗子,比如接口上返回的是有两个字段,一个productId 一个productName, 显而易见productId意思就是每个产品不同的ID。很简单这里的cacheKey就可以取这里的productId。
#import "ViewController.h"
#import "WeiChatTableViewCell.h"
#import "Masonry.h"
#import "UITableViewCell+FSAutoCountHeight.h"
#define SCREEN_WIDTH [[UIScreen mainScreen] bounds].size.width
#define SCREEN_HEIGHT [[UIScreen mainScreen] bounds].size.height
#define COLOR_White [UIColor whiteColor]
@interface ViewController ()
@property (nonatomic, strong) UITableView *tableView;
@property (nonatomic, strong) NSMutableArray *dataArr;
@property (nonatomic, strong) UISlider *slider;
@property (nonatomic, assign) CGFloat fontSize;
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
self.dataArr = [NSMutableArray array];
[self.dataArr addObjectsFromArray:@[@"你好1",@"你好2",@"你好3",@"你好4",@"你好5",@"你好6",@"你好7"]];
[self setUpTableView];
[self setUpMyControlView];
//设置默认字体大小为16px
self.fontSize=16;
}
- (void)setUpTableView{
self.tableView = [[UITableView alloc]initWithFrame:CGRectMake(0, 0, SCREEN_WIDTH, SCREEN_HEIGHT - 100)];
self.tableView.backgroundColor = [UIColor greenColor];
self.tableView.dataSource = self;
self.tableView.delegate=self;
[self.view addSubview:self.tableView];
self.tableView.separatorStyle = UITableViewCellSeparatorStyleNone;
}
- (void)setUpMyControlView{
UIView*smallView = [[UIViewalloc]init];
smallView.backgroundColor = [UIColor yellowColor];
[self.viewaddSubview:smallView];
smallView.frame=CGRectMake(0,SCREEN_HEIGHT-100,SCREEN_WIDTH,100);
// [smallView mas_makeConstraints:^(MASConstraintMaker *make) {
// make.top.equalTo(self.tableView.mas_bottom);
// make.left.with.equalTo(self.view);
// make.height.mas_equalTo(100);
// }];
UISlider *slider = [[UISlider alloc] init];
self.slider= slider;
slider.minimumValue=9;
slider.maximumValue=32;
slider.value=16;
[smallViewaddSubview:slider];
[slidermas_makeConstraints:^(MASConstraintMaker *make) {
make.center.equalTo(smallView);
make.size.mas_equalTo(CGSizeMake(SCREEN_WIDTH, 30));
}];
slider.continuous=YES;
//滑轮左边颜色,如果设置了左边的图片就不会显示
slider.minimumTrackTintColor = [UIColor greenColor];
//滑轮右边颜色,如果设置了右边的图片就不会显示
slider.maximumTrackTintColor = [UIColor redColor];
[slideraddTarget:self action:@selector(sliderValueChanged:) forControlEvents:UIControlEventValueChanged];
}
- (void)sliderValueChanged:(UISlider*)slider{
for (WeiChatTableViewCell *cell in [self.tableView visibleCells]) {
cell.fontSize= slider.value;
}
}
#pragma mark - tableViewDataSource
- (NSInteger) numberOfSectionsInTableView:(UITableView*)tableView{
return 1;
}
- (NSInteger)tableView:(UITableView*)tableView numberOfRowsInSection:(NSInteger)section{
return self.dataArr.count;
}
- (UITableViewCell*)tableView:(UITableView*)tableView cellForRowAtIndexPath:(NSIndexPath*)indexPath{
WeiChatTableViewCell *cell = [WeiChatTableViewCell cellWithTableView:tableView];
cell.fontSize = self.fontSize;
if(self.dataArr.count> indexPath.row){
cell.mainLabel.text=self.dataArr[indexPath.row];
}
returncell;
}
- (CGFloat) tableView:(UITableView*)tableView heightForRowAtIndexPath:(NSIndexPath*)indexPath{
if(self.dataArr.count> indexPath.row) {
NSString*titleString =self.dataArr[indexPath.row];
return [WeiChatTableViewCell FSCellHeightForTableView:tableView indexPath:indexPath cacheKey:titleString cellContentViewWidth:0 bottomOffset:0];
}
return 0.01f;
}
@end
这个是自定义的cell
(.h)
#import
@interfaceWeiChatTableViewCell :UITableViewCell
@property (nonatomic, strong) UILabel *mainLabel;
//外界改变label大小方式的键
@property (nonatomic, assign) CGFloat fontSize;
+ (instancetype)cellWithTableView:(UITableView*)tableView;
@end
(.m)
#import "WeiChatTableViewCell.h"
#import "Masonry.h"
#import "UITableViewCell+FSAutoCountHeight.h"
@implementationWeiChatTableViewCell
+ (instancetype)cellWithTableView:(UITableView*)tableView{
static NSString * cellId=@"PersonCentreCell";
WeiChatTableViewCell *cell=[tableView dequeueReusableCellWithIdentifier:cellId];
if(cell==nil) {
cell=[[WeiChatTableViewCell alloc]initWithStyle:UITableViewCellStyleValue1 reuseIdentifier:cellId];
}
returncell;
}
- (instancetype)initWithStyle:(UITableViewCellStyle)style reuseIdentifier:(NSString*)reuseIdentifier{
self= [superinitWithStyle:stylereuseIdentifier:reuseIdentifier];
if(self) {
for(UIView *sub in self.contentView.subviews)
{
[subremoveFromSuperview];
}
[selfcreatUI];
}
return self;
}
- (void)creatUI{
self.mainLabel = [[UILabel alloc] init];
self.mainLabel.textColor = [UIColor grayColor];
self.mainLabel.backgroundColor = [UIColor blueColor];
self.mainLabel.textAlignment = NSTextAlignmentLeft;
self.mainLabel.translatesAutoresizingMaskIntoConstraints = NO;
if(self.fontSize&&self.fontSize>0) {
self.mainLabel.font = [UIFont systemFontOfSize:self.fontSize];
}else{
//默认是16
self.mainLabel.font = [UIFont systemFontOfSize:16];
}
[self.contentView addSubview:self.mainLabel];
[self.mainLabel mas_makeConstraints:^(MASConstraintMaker *make) {
make.top.equalTo(self.contentView.mas_top).offset(25);
// make.top.mas_equalTo(SCREEN_AUTO_HIGHT * 30/1271);
make.left.mas_equalTo(12);
make.right.lessThanOrEqualTo(self.contentView.mas_right).with.offset(- 12);
}];
UIView*smallView = [[UIViewalloc]init];
[self.contentView addSubview:smallView];
[smallViewmas_makeConstraints:^(MASConstraintMaker *make) {
make.top.equalTo(self.mainLabel.mas_bottom);
make.left.with.equalTo(self.contentView);
make.height.mas_equalTo(20);
}];
self.FS_cellBottomView= smallView;
}
- (void)setFontSize:(CGFloat)fontSize{
_fontSize= fontSize;
self.mainLabel.font = [UIFont systemFontOfSize:fontSize];
[self layoutIfNeeded];
}
@end
最后上一下提到的核心(分)类
(.h)
#import
@interfaceUITableViewCell (FSAutoCountHeightCell)
/**
cell底视图(提高计算效率,能传则传)
*/
@property(nonatomic,strong)UIView* FS_cellBottomView;
/**
cell底视图数组(在不确定最下面的视图关系时,可以传入一个视图数组)
*/
@property(nonatomic,strong)NSArray* FS_cellBottomViews;
/**
cell自动计算行高
@param tableView tableView
@param indexPath indexPath
@param contentViewWidth cell内容宽度,不确定可传0
@return cell高度
*/
+ (CGFloat)FSCellHeightForTableView:(UITableView*)tableView indexPath:(NSIndexPath*)indexPath cellContentViewWidth:(CGFloat)contentViewWidth bottomOffset:(CGFloat)bottomOffset;
/**
cell自动计算行高优化版
@param tableView tableView
@param indexPath indexPath
@param cacheKey 当前cell唯一标识符
@param contentViewWidth cell内容宽度,不确定可传0
@return cell高度
*/
+ (CGFloat)FSCellHeightForTableView:(UITableView*)tableView indexPath:(NSIndexPath*)indexPath cacheKey:(NSString*)cacheKey cellContentViewWidth:(CGFloat)contentViewWidth bottomOffset:(CGFloat)bottomOffset;
@end
(.m)
#import "UITableViewCell+FSAutoCountHeight.h"
#import
#define ScreenScale ([[UIScreen mainScreen] scale])
CG_INLINE CGFloat
flatSpecificScale(CGFloatfloatValue,CGFloatscale) {
scale = scale ==0?ScreenScale: scale;
CGFloatflattedValue =ceil(floatValue * scale) / scale;
returnflattedValue;
}
CG_INLINE CGFloat
flat(CGFloatfloatValue) {
returnflatSpecificScale(floatValue,0);
}
CG_INLINE CGRect
CGRectSetWidth(CGRectrect,CGFloatwidth) {
rect.size.width=flat(width);
returnrect;
}
CG_INLINE void
ReplaceMethod(Class_class,SEL_originSelector,SEL_newSelector) {
MethodoriMethod =class_getInstanceMethod(_class, _originSelector);
MethodnewMethod =class_getInstanceMethod(_class, _newSelector);
BOOLisAddedMethod =class_addMethod(_class, _originSelector,method_getImplementation(newMethod),method_getTypeEncoding(newMethod));
if(isAddedMethod) {
class_replaceMethod(_class, _newSelector, method_getImplementation(oriMethod), method_getTypeEncoding(oriMethod));
}else{
method_exchangeImplementations(oriMethod, newMethod);
}
}
@interfaceUITableView (FSAutoCountHeight)
@property(nonatomic,strong)NSMutableDictionary*keyCacheDic_Portrait;
@property(nonatomic,strong)NSMutableDictionary*keyCacheDic_Landscape;
@property(nonatomic,strong)NSMutableArray*indexCacheArr_Portrait;
@property(nonatomic,strong)NSMutableArray*indexCacheArr_Landscape;
@property (nonatomic, assign) BOOL isIndexPath;
@end
@implementationUITableView (FSAutoCountHeight)
+ (void)load
{
SELselectors[] = {
@selector(reloadData),
@selector(insertSections:withRowAnimation:),
@selector(deleteSections:withRowAnimation:),
@selector(reloadSections:withRowAnimation:),
@selector(moveSection:toSection:),
@selector(insertRowsAtIndexPaths:withRowAnimation:),
@selector(deleteRowsAtIndexPaths:withRowAnimation:),
@selector(reloadRowsAtIndexPaths:withRowAnimation:),
@selector(moveRowAtIndexPath:toIndexPath:)
};
for(NSUIntegerindex =0; index
SELoriginalSelector = selectors[index];
SEL swizzledSelector = NSSelectorFromString([@"FS_" stringByAppendingString:NSStringFromSelector(originalSelector)]);
ReplaceMethod(self, originalSelector, swizzledSelector);
}
}
#pragma mark setter/getter
- (NSMutableDictionary*)keyCacheDicForCurrentOrientation
{
return UIDeviceOrientationIsPortrait([UIDevice currentDevice].orientation) ? self.keyCacheDic_Portrait: self.keyCacheDic_Landscape;
}
- (void)setKeyCacheDic_Portrait:(NSMutableDictionary*)keyCacheDic_Portrait
{
objc_setAssociatedObject(self, @selector(keyCacheDic_Portrait), keyCacheDic_Portrait, OBJC_ASSOCIATION_RETAIN_NONATOMIC);
}
- (NSMutableDictionary*)keyCacheDic_Portrait
{
return objc_getAssociatedObject(self, _cmd);
}
- (void)setKeyCacheDic_Landscape:(NSMutableDictionary*)keyCacheDic_Landscape
{
objc_setAssociatedObject(self, @selector(keyCacheDic_Landscape), keyCacheDic_Landscape, OBJC_ASSOCIATION_RETAIN_NONATOMIC);
}
- (NSMutableDictionary*)keyCacheDic_Landscape
{
return objc_getAssociatedObject(self, _cmd);
}
- (NSMutableArray*)indexCacheArrForCurrentOrientation
{
return UIDeviceOrientationIsPortrait([UIDevice currentDevice].orientation) ? self.indexCacheArr_Portrait: self.indexCacheArr_Landscape;
}
- (void)setIndexCacheArr_Portrait:(NSMutableArray*)indexCacheArr_Portrait
{
objc_setAssociatedObject(self,@selector(indexCacheArr_Portrait), indexCacheArr_Portrait,OBJC_ASSOCIATION_RETAIN_NONATOMIC);
}
- (NSMutableArray*)indexCacheArr_Portrait
{
return objc_getAssociatedObject(self, _cmd);
}
- (void)setIndexCacheArr_Landscape:(NSMutableArray*)indexCacheArr_Landscape
{
objc_setAssociatedObject(self,@selector(indexCacheArr_Landscape), indexCacheArr_Landscape,OBJC_ASSOCIATION_RETAIN_NONATOMIC);
}
- (NSMutableArray*)indexCacheArr_Landscape
{
return objc_getAssociatedObject(self, _cmd);
}
- (void)setIsIndexPath:(BOOL)isIndexPath
{
objc_setAssociatedObject(self, @selector(isIndexPath), @(isIndexPath), OBJC_ASSOCIATION_ASSIGN);
}
- (BOOL)isIndexPath
{
return [objc_getAssociatedObject(self, _cmd) boolValue];
}
#pragma mark exchangeVoid
- (void)FS_reloadData
{
if (self.indexCacheArrForCurrentOrientation&&self.isIndexPath) {
[self.indexCacheArrForCurrentOrientation removeAllObjects];
}
[self FS_reloadData];
}
- (void)FS_reloadSections:(NSIndexSet*)sections withRowAnimation:(UITableViewRowAnimation)animation
{
if (self.indexCacheArrForCurrentOrientation&§ions&&self.isIndexPath) {
[sectionsenumerateIndexesUsingBlock:^(NSUIntegeridx,BOOL*_Nonnullstop) {
if (idx < self.indexCacheArrForCurrentOrientation.count) {
[self.indexCacheArrForCurrentOrientation[idx] removeAllObjects];
}
}];
}
[self FS_reloadSections:sections withRowAnimation:animation];
}
- (void)FS_reloadRowsAtIndexPaths:(NSArray *)indexPaths withRowAnimation:(UITableViewRowAnimation)animation
{
if (self.indexCacheArrForCurrentOrientation&&indexPaths&&self.isIndexPath) {
[indexPathsenumerateObjectsUsingBlock:^(NSIndexPath*_Nonnullobj,NSUIntegeridx,BOOL*_Nonnullstop) {
if (obj.section < self.indexCacheArrForCurrentOrientation.count) {
NSMutableArray*rowCacheArr =self.indexCacheArrForCurrentOrientation[obj.section];
if(obj.row< rowCacheArr.count) {
rowCacheArr[obj.row] = @-1;
}
}
}];
}
[self FS_reloadRowsAtIndexPaths:indexPaths withRowAnimation:animation];
}
- (void)FS_moveSection:(NSInteger)section toSection:(NSInteger)newSection
{
if (self.isIndexPath && self.indexCacheArrForCurrentOrientation) {
if (section < self.indexCacheArrForCurrentOrientation.count && newSection < self.indexCacheArrForCurrentOrientation.count) {
[self.indexCacheArrForCurrentOrientation exchangeObjectAtIndex:section withObjectAtIndex:newSection];
}
}
[selfFS_moveSection:sectiontoSection:newSection];
}
- (void)FS_moveRowAtIndexPath:(NSIndexPath*)indexPath toIndexPath:(NSIndexPath*)newIndexPath
{
if (self.isIndexPath && self.indexCacheArrForCurrentOrientation) {
if (indexPath.section < self.indexCacheArrForCurrentOrientation.count && newIndexPath.section < self.indexCacheArrForCurrentOrientation.count) {
NSMutableArray *indexPathRows =self.indexCacheArrForCurrentOrientation[indexPath.section];
NSMutableArray *newIndexPathRows =self.indexCacheArrForCurrentOrientation[newIndexPath.section];
if(indexPath.row< indexPathRows.count&& newIndexPath.row< newIndexPathRows.count) {
NSNumber*indexValue = indexPathRows[indexPath.row];
NSNumber*newIndexValue = newIndexPathRows[newIndexPath.row];
indexPathRows[indexPath.row] = newIndexValue;
newIndexPathRows[newIndexPath.row] = indexValue;
}
}
}
[self FS_moveRowAtIndexPath:indexPath toIndexPath:newIndexPath];
}
- (void)FS_insertSections:(NSIndexSet*)sections withRowAnimation:(UITableViewRowAnimation)animation
{
if (self.isIndexPath && self.indexCacheArrForCurrentOrientation) {
[sectionsenumerateIndexesUsingBlock:^(NSUIntegeridx,BOOL*_Nonnullstop) {
if (idx <= self.indexCacheArrForCurrentOrientation.count) {
[self.indexCacheArrForCurrentOrientation insertObject:[NSMutableArray array] atIndex:idx];
}
}];
}
[self FS_insertSections:sections withRowAnimation:animation];
}
- (void)FS_insertRowsAtIndexPaths:(NSArray *)indexPaths withRowAnimation:(UITableViewRowAnimation)animation
{
if (self.isIndexPath && self.indexCacheArrForCurrentOrientation) {
[indexPathsenumerateObjectsUsingBlock:^(NSIndexPath*_Nonnullobj,NSUIntegeridx,BOOL*_Nonnullstop) {
if (obj.section < self.indexCacheArrForCurrentOrientation.count) {
NSMutableArray *rowCacheArr =self.indexCacheArrForCurrentOrientation[obj.section];
if(obj.row<= rowCacheArr.count) {
[rowCacheArrinsertObject:@-1atIndex:obj.row];
}
}
}];
}
[self FS_insertRowsAtIndexPaths:indexPaths withRowAnimation:animation];
}
- (void)FS_deleteSections:(NSIndexSet*)sections withRowAnimation:(UITableViewRowAnimation)animation
{
if (self.isIndexPath && self.indexCacheArrForCurrentOrientation) {
[sectionsenumerateIndexesUsingBlock:^(NSUIntegeridx,BOOL*_Nonnullstop) {
if (idx < self.indexCacheArrForCurrentOrientation.count) {
[self.indexCacheArrForCurrentOrientation removeObjectAtIndex:idx];
}
}];
}
[self FS_deleteSections:sections withRowAnimation:animation];
}
- (void)FS_deleteRowsAtIndexPaths:(NSArray *)indexPaths withRowAnimation:(UITableViewRowAnimation)animation
{
if (self.isIndexPath && self.indexCacheArrForCurrentOrientation) {
[indexPathsenumerateObjectsUsingBlock:^(NSIndexPath*_Nonnullobj,NSUIntegeridx,BOOL*_Nonnullstop) {
if (obj.section < self.indexCacheArrForCurrentOrientation.count) {
NSMutableArray *rowCacheArr =self.indexCacheArrForCurrentOrientation[obj.section];
if(obj.row< rowCacheArr.count) {
[rowCacheArrremoveObjectAtIndex:obj.row];
}
}
}];
}
[self FS_deleteRowsAtIndexPaths:indexPaths withRowAnimation:animation];
}
@end
@implementationUITableViewCell (FSAutoCountHeightCell)
- (void)setFS_cellBottomViews:(NSArray*)FS_cellBottomViews {
objc_setAssociatedObject(self,
@selector(FS_cellBottomViews),
FS_cellBottomViews,
OBJC_ASSOCIATION_COPY);
}
- (NSArray*)FS_cellBottomViews {
return objc_getAssociatedObject(self, _cmd);
}
- (void)setFS_cellBottomView:(UIView*)FS_cellBottomView {
objc_setAssociatedObject(self,
@selector(FS_cellBottomView),
FS_cellBottomView,
OBJC_ASSOCIATION_RETAIN_NONATOMIC);
}
- (UIView*)FS_cellBottomView {
return objc_getAssociatedObject(self, _cmd);
}
+ (CGFloat)FSCellHeightForTableView:(UITableView*)tableView indexPath:(NSIndexPath*)indexPath cellContentViewWidth:(CGFloat)contentViewWidth bottomOffset:(CGFloat)bottomOffset
{
if(!indexPath) {
return0;
}
tableView.isIndexPath=YES;
if (!tableView.indexCacheArr_Portrait) {
tableView.indexCacheArr_Portrait= [NSMutableArrayarray];
}
if (!tableView.indexCacheArr_Landscape) {
tableView.indexCacheArr_Landscape= [NSMutableArrayarray];
}
for(NSIntegersectionIndex =0; sectionIndex <= indexPath.section; sectionIndex++) {
if(sectionIndex >= tableView.indexCacheArrForCurrentOrientation.count) {
tableView.indexCacheArrForCurrentOrientation[sectionIndex] = [NSMutableArrayarray];
}
}
NSMutableArray*rowCacheArr = tableView.indexCacheArrForCurrentOrientation[indexPath.section];
for(NSIntegerrowIndex =0; rowIndex <= indexPath.row; rowIndex++) {
if(rowIndex >= rowCacheArr.count) {
rowCacheArr[rowIndex] = @-1;
}
}
if(![tableView.indexCacheArrForCurrentOrientation[indexPath.section][indexPath.row]isEqualToNumber:@-1]) {
CGFloatcellHeight =0;
NSNumber*heightNumber = tableView.indexCacheArrForCurrentOrientation[indexPath.section][indexPath.row];
cellHeight = heightNumber.floatValue;
returncellHeight;
}
UITableViewCell *cell = [tableView.dataSource tableView:tableView cellForRowAtIndexPath:indexPath];
if(contentViewWidth ==0) {
[tableViewlayoutIfNeeded];
contentViewWidth =CGRectGetWidth(tableView.frame);
}
if(contentViewWidth ==0) {
return0;
}
cell.frame=CGRectSetWidth(cell.frame, contentViewWidth);
cell.contentView.frame = CGRectSetWidth(cell.contentView.frame, CGRectGetWidth(tableView.frame));
[celllayoutIfNeeded];
UIView*cellBottomView =nil;
if (cell.FS_cellBottomView) {
cellBottomView = cell.FS_cellBottomView;
}else if (cell.FS_cellBottomViews && cell.FS_cellBottomViews.count > 0) {
cellBottomView = cell.FS_cellBottomViews[0];
for(UIView*viewincell.FS_cellBottomViews) {
if(CGRectGetMaxY(view.frame) >CGRectGetMaxY(cellBottomView.frame)) {
cellBottomView = view;
}
}
}else{
NSArray*contentViewSubViews = cell.contentView.subviews;
if(contentViewSubViews.count==0) {
cellBottomView = cell.contentView;
}else{
cellBottomView = contentViewSubViews[0];
for(UIView*viewincontentViewSubViews) {
if(CGRectGetMaxY(view.frame) >CGRectGetMaxY(cellBottomView.frame)) {
cellBottomView = view;
}
}
}
}
CGFloatcellHeight =CGRectGetMaxY(cellBottomView.frame) + bottomOffset;
tableView.indexCacheArrForCurrentOrientation[indexPath.section][indexPath.row] =@(cellHeight);
returncellHeight;
}
+ (CGFloat)FSCellHeightForTableView:(UITableView*)tableView indexPath:(NSIndexPath*)indexPath cacheKey:(NSString*)cacheKey cellContentViewWidth:(CGFloat)contentViewWidth bottomOffset:(CGFloat)bottomOffset
{
if(!indexPath || !cacheKey) {
return0;
}
tableView.isIndexPath=NO;
if (!tableView.keyCacheDic_Portrait) {
tableView.keyCacheDic_Portrait = [NSMutableDictionary dictionary];
}
if (!tableView.keyCacheDic_Landscape) {
tableView.keyCacheDic_Landscape = [NSMutableDictionary dictionary];
}
NSNumber*cacheHeight = tableView.keyCacheDicForCurrentOrientation[cacheKey];
if(cacheHeight !=nil) {
CGFloatcellHeight =0;
cellHeight = cacheHeight.floatValue;
returncellHeight;
}
UITableViewCell *cell = [tableView.dataSource tableView:tableView cellForRowAtIndexPath:indexPath];
if(contentViewWidth ==0) {
[tableViewlayoutIfNeeded];
contentViewWidth =CGRectGetWidth(tableView.frame);
}
if(contentViewWidth ==0) {
return0;
}
cell.frame=CGRectSetWidth(cell.frame, contentViewWidth);
cell.contentView.frame = CGRectSetWidth(cell.contentView.frame, CGRectGetWidth(tableView.frame));
[celllayoutIfNeeded];
UIView*cellBottomView =nil;
if (cell.FS_cellBottomView) {
cellBottomView = cell.FS_cellBottomView;
}else if (cell.FS_cellBottomViews && cell.FS_cellBottomViews.count > 0) {
cellBottomView = cell.FS_cellBottomViews[0];
for(UIView*viewincell.FS_cellBottomViews) {
if(CGRectGetMaxY(view.frame) >CGRectGetMaxY(cellBottomView.frame)) {
cellBottomView = view;
}
}
}else{
NSArray*contentViewSubViews = cell.contentView.subviews;
if(contentViewSubViews.count==0) {
cellBottomView = cell.contentView;
}else{
cellBottomView = contentViewSubViews[0];
for(UIView*viewincontentViewSubViews) {
if(CGRectGetMaxY(view.frame) >CGRectGetMaxY(cellBottomView.frame)) {
cellBottomView = view;
}
}
}
}
CGFloatcellHeight =CGRectGetMaxY(cellBottomView.frame) + bottomOffset;
[tableView.keyCacheDicForCurrentOrientationsetValue:@(cellHeight)forKey:cacheKey];
returncellHeight;
}
@end
内容有点多,其实我这边还有压缩版的,可以添加我的微信(Xpc1314520xpc),直接发给你们。这个是干货,感觉用的上的小伙伴可以赞一个,不一定要打赏嘛☺!