QUESTION 11
Given the code fragment:
Stream<List<String>> iStr= Stream.of (
Arrays.asList (“1”, “John”),
Arrays.asList (“2”, null)0;
Stream<<String> nInSt = iStr.flatMapToInt ((x) -> x.stream ());
nInSt.forEach (System.out :: print);
What is the result?
A.
1John2null
B.
12
C. A
NullPointerException
is thrown at run time.
D. A compilation error occurs.
Correct Answer:
D
Section: (none)
Explanation
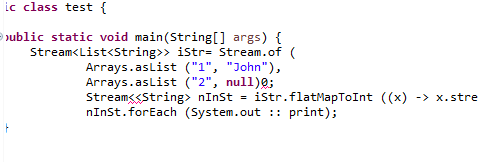
Explanation/Reference:
QUESTION 12
Given the code fragment:
Path file = Paths.get (“courses.txt”);
// line n1
//Assume the courses.txt is accessible.
Which code fragment can be inserted at line n1 to enable the code to print the content of the courses.txt file?
A.
List<String> fc = Files.list(file);
fc.stream().forEach (s - > System.out.println(s));
B.
Stream<String> fc = Files.readAllLines (file);
fc.forEach (s - > System.out.println(s));
C.
List<String> fc = readAllLines(file);
fc.stream().forEach (s - > System.out.println(s));
D.
Stream<String> fc = Files.lines (file);
fc.forEach (s - > System.out.println(s));
Correct Answer:
D
Section: (none)
Explanation
Explanation/Reference:
Path和Files用法:
https://www.cnblogs.com/devilwind/p/8623098.html
QUESTION 13
Given the code fragment:
public void recDelete (String dirName) throws IOException {
File [ ] listOfFiles = new File (dirName) .listFiles();
if (listOfFiles ! = null && listOfFiles.length >0) {
for (File aFile : listOfFiles) {
if (aFile.isDirectory ()) {
recDelete (aFile.getAbsolutePath ());
} else {
if (aFile.getName ().endsWith (“.class”))
aFile.delete ();
}
}
}
}
Assume that
Projects
contains subdirectories that contain
.class
files and is passed as an argument to
the
recDelete ()
method when it is invoked.
What is the result?
A. The method deletes all the
.class
files in the
Projects
directory and its subdirectories.
B. The method deletes the
.class
files of the
Projects
directory only.
C. The method executes and does not make any changes to the
Projects
directory.
D. The method throws an IOException.
Correct Answer:
A
Section: (none)
Explanation
Explanation/Reference:
QUESTION 14
Given the code fragments:
4. void doStuff() throws ArithmeticException, NumberFormatException, Exception
{
5. if (Math.random() >-1 throw new Exception (“Try again”);
6. }
and
24. try {
25. doStuff ( ):
26. } catch (ArithmeticException | NumberFormatException | Exception e) {
27. System.out.println (e.getMessage()); }
28. catch (Exception e) {
29. System.out.println (e.getMessage()); }
30. }
Which modification enables the code to print
Try again
?
A. Comment the lines 28, 29 and 30.
B. Replace line 26 with:
} catch (Exception | ArithmeticException | NumberFormatException e) {
C. Replace line 26 with:
} catch (ArithmeticException | NumberFormatException e) {
D. Replace line 27 with:
throw e;
Correct Answer:
C
Section: (none)
Explanation
Explanation/Reference:
java.lang.ArithmeticException
这个异常的解释是"数学运算异常",比如程序中出现了除以零这样的运算就会出这样的异常。
java.lang.NumberFormatException
是数据格式在转化的时候,出现错误。
QUESTION 15
Given the definition of the Country class:
public class country {
public enum Continent {ASIA, EUROPE}
String name;
Continent region;
public Country (String na, Continent reg) {
name = na; region = reg;
}
public String getName () {return name;}
public Continent getRegion () {return region;}
}
and the code fragment:
List<Country> couList = Arrays.asList (
new Country (“Japan”, Country.Continent.ASIA),
new Country (“Italy”, Country.Continent.EUROPE),
new Country (“Germany”, Country.Continent.EUROPE));
Map<Country.Continent, List<String>> regionNames = couList.stream ()
.collect(Collectors.groupingBy (Country ::getRegion,
Collectors.mapping(Country::getName, Collectors.toList())));
System.out.println(regionNames);
A.
{EUROPE = [Italy, Germany], ASIA = [Japan]}
B.
{ASIA = [Japan], EUROPE = [Italy, Germany]}
C.
{EUROPE = [Germany, Italy], ASIA = [Japan]}
D.
{EUROPE = [Germany], EUROPE = [Italy], ASIA = [Japan]}
Correct Answer:
B
Section: (none)
Explanation
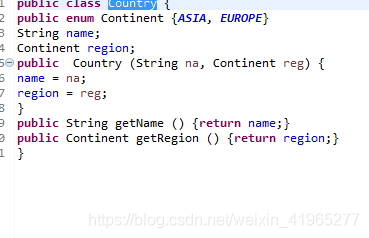
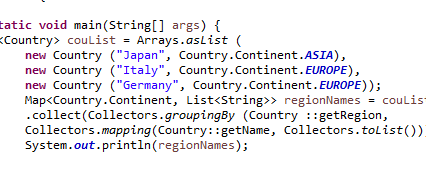

Explanation/Reference:
Collectors API:
https://blog.csdn.net/M_Joes_17/article/details/82805208
QUESTION 16
Given the code fragment:
Map<Integer, String> books = new TreeMap<>();
books.put (1007, “A”);
books.put (1002, “C”);
books.put (1001, “B”);
books.put (1003, “B”);
System.out.println (books);
What is the result?
A.
{1007 = A, 1002 = C, 1001 = B, 1003 = B}
B.
{1001 = B, 1002 = C, 1003 = B, 1007 = A
}
C.
{1002 = C, 1003 = B, 1007 = A}
D.
{1007 = A, 1001 = B, 1003 = B, 1002 = C}
Correct Answer:
B
Section: (none)
Explanation
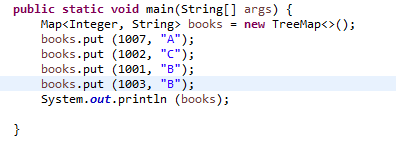
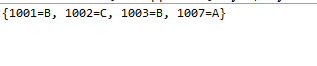
Explanation/Reference:
Reference: TreeMap inherits SortedMap and automatically sorts the element's key
QUESTION 17
Given:
class Book {
int id;
String name;
public Book (int id, String name) {
this.id = id;
this.name = name;
}
public boolean equals (Object obj) { //line n1
boolean output = false;
Book b = (Book) obj;
if (this.name.equals(b name)){
output = true;
}
return output;
}
}
and the code fragment:
Book b1 = new Book (101, “Java Programing”);
Book b2 = new Book (102, “Java Programing”);
System.out.println (b1.equals(b2)); //line n2
Which statement is true?
A. The program prints
true
.
B. The program prints
false
.
C. A compilation error occurs. To ensure successful compilation, replace
line n1
with:
boolean equals (Book obj) {
D. A compilation error occurs. To ensure successful compilation, replace
line n2
with:
System.out.println (b1.equals((Object) b2));
Correct Answer:
A
Section: (none)
Explanation
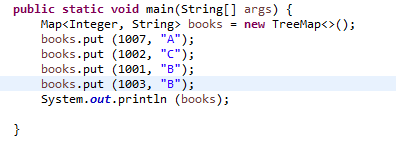
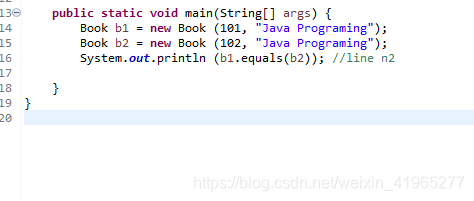
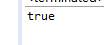
Explanation/Reference:
QUESTION 18
Given the content of
/resourses/Message.properties
:
welcome1=”Good day!”
and given the code fragment:
Properties prop = new Properties ();
FileInputStream fis = new FileInputStream (“/resources/Message.properties”);
prop.load(fis);
System.out.println(prop.getProperty(“welcome1”));
System.out.println(prop.getProperty(“welcome2”, “Test”));//line n1
System.out.println(prop.getProperty(“welcome3”));
What is the result?
A.
Good day!
Test
followed by an
Exception
stack trace
B.
Good day!
followed by an
Exception
stack trace
C.
Good day!
Test
null
D. A compilation error occurs at
line n1
.
Correct Answer:
C
Section: (none)
Explanation
Explanation/Reference:
FileInputStream类的使用:
https://blog.csdn.net/chentiefeng521/article/details/51776218
QUESTION 19
Which action can be used to load a database driver by using JDBC3.0?
A. Add the driver class to the META-INF/services folder of the JAR file.
B. Include the JDBC driver class in a
jdbc.properties
file.
C. Use the
java.lang.Class.forName
method to load the driver class.
D. Use the
DriverManager.getDriver
method to load the driver class.
Correct Answer:
C
Section: (none)
Explanation
Explanation/Reference:
QUESTION 20
Given the code fragment:
Path p1 = Paths.get(“/Pics/MyPic.jpeg”);
System.out.println (p1.getNameCount() +
“:” + p1.getName(1) +
“:” + p1.getFileName());
Assume that the
Pics
directory does NOT exist.
What is the result?
A. An exception is thrown at run time.
B.
2:MyPic.jpeg: MyPic.jpeg
C.
1:Pics:/Pics/ MyPic.jpeg
D.
2:Pics: MyPic.jpeg
Correct Answer:
B
Section: (none)
Explanation
Explanation/Reference: