从此章开始,遇到前面的知识点,不再解释。
QUESTION 71
Given the records from the
Employee
table:
eid | ename |
111 | Tom |
112 | Jerry |
113 | Donald |
and given the code fragment:
try {
Connection conn = DriverManager.getConnection (URL, userName,
passWord);
Statement st = conn.createStatement(ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_UPDATABLE);
st.execute(“SELECT*FROM Employee”);
ResultSet rs = st.getResultSet();
while (rs.next()) {
if (rs.getInt(1) ==112) {
rs.updateString(2, “Jack”);
}
}
rs.absolute(2);
System.out.println(rs.getInt(1) + “ “ + rs.getString(2));
} catch (SQLException ex) {
System.out.println(“Exception is raised”);
}
Assume that:
The required database driver is configured in the classpath.
The appropriate database accessible with the
URL
,
userName
, and
passWord
exists.
What is the result?
A. The Employee table is updated with the row:
112 Jack
and the program prints:
112 Jerry
B. The Employee table is updated with the row:
112 Jack
and the program prints:
112 Jack
C. The Employee table is not updated and the program prints:
112 Jerry
D. The program prints
Exception is raised
.
Correct Answer:
C
Section: (none)
Explanation
Explanation/Reference:
QUESTION 72
Given:
class RateOfInterest {
public static void main (String[] args) {
int rateOfInterest = 0;
String accountType = "LOAN";
switch (accountType) {
case"RD":
rateOfInterest = 5;
break;
case"FD":
rateOfInterest = 10;
break;
default:
assert false: "No interest for this account"; //line n1
}
System.out.println ("Rate of interest:" + rateOfInterest);
}
and the command:
java –ea RateOfInterest
What is the result?
A.
Rate of interest: 0
B. An AssertionError is thrown.
C.
No interest for this account
D. A compilation error occurs at
line n1
.
Correct Answer: B
Section: (none)
Explanation
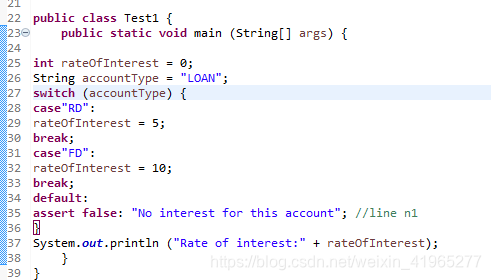

Explanation/Reference:
QUESTION 73
Given the code fragment:
class CallerThread implements Callable<String> {
String str;
public CallerThread(String s) {this.str=s;}
public String call() throws Exception {
return str.concat(“Call”);
}
}
and
public static void main (String[] args) throws InterruptedException,
ExecutionException
{
ExecutorService es = Executors.newFixedThreadPool(4); //line n1
Future f1 = es.submit (new CallerThread("Call"));
String str = f1.get().toString();
System.out.println(str);
}
Which statement is true?
A. The program prints
Call Call
and terminates.
B. The program prints
Call Call
and does not terminate.
C. A compilation error occurs at
line n1
.
D. An
ExecutionException
is thrown at run time.
Correct Answer:
B
Section: (none)
Explanation
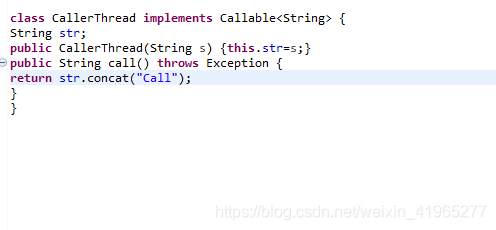
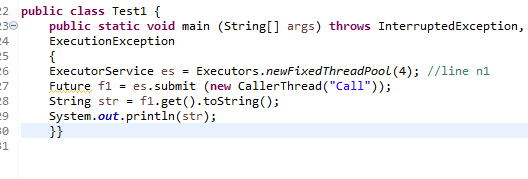
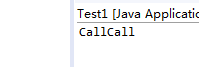
Explanation/Reference:
ExecutionException API说明:
https://docs.oracle.com/javase/9/docs/api/java/util/concurrent/ExecutionException.html
QUESTION 74
Given the code fragment:
String fName;
public FileThread(String fName) { this.fName = fName; }
public void run () System.out.println(fName);}
public static void main (String[] args) throws IOException,
InterruptedException {
ExecutorService executor = Executors.newCachedThreadPool();
Stream<Path> listOfFiles = Files.walk(Paths.get(“Java Projects”));
listOfFiles.forEach(line -> {
executor.execute(new FileThread(line.getFileName().toString()));
//
line n1
});
executor.shutdown();
executor.awaitTermination(5, TimeUnit.DAYS); //
line n2
}
}
The
Java Projects
directory exists and contains a list of files.
What is the result?
A. The program throws a runtime exception at
line n2
.
B. The program prints files names concurrently.
C. The program prints files names sequentially.
D. A compilation error occurs at
line n1
.
Correct Answer:
B
Section: (none)
Explanation
找buging待定...
Explanation/Reference:
QUESTION 75
Given:
class CheckClass {
public static int checkValue (String s1, String s2) {
return s1.length()-s2.length();
}
}
and the code fragment:
String[] strArray = new String [] {“Tiger”, “Rat”, “Cat”, “Lion”}
//line n1
for (String s : strArray) {
System.out.print (s + “ “);
}
Which code fragment should be inserted at line n1 to enable the code to print
Rat Cat Lion Tiger
?
A.
Arrays.sort(strArray, CheckClass : : checkValue);
B.
Arrays.sort(strArray, (CheckClass : : new) : : checkValue);
C.
Arrays.sort(strArray, (CheckClass : : new).checkValue);
D.
Arrays.sort(strArray, CheckClass : : new : : checkValue);
Correct Answer:
A
Section: (none)
Explanation
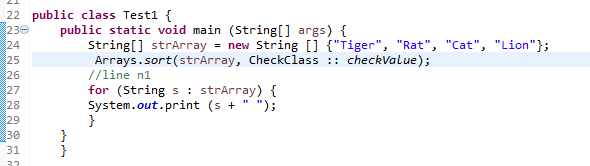
A
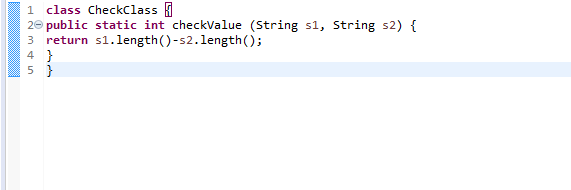
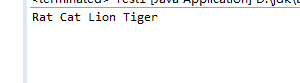
B:
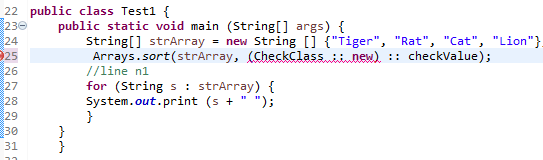
error:The target type of this expression must be a functional interface
C:
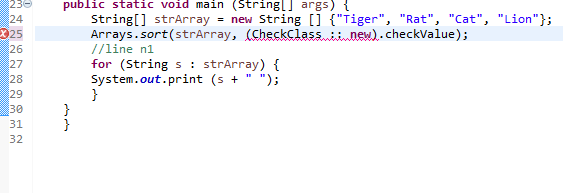
error:The target type of this expression must be a functional interface
D:
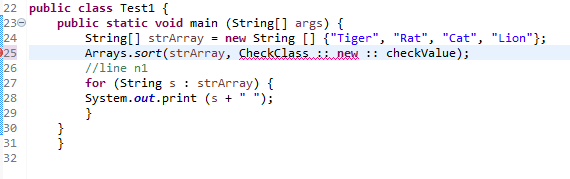
error:The target type of this expression must be a functional interface
Explanation/Reference:
Arrays.sort()的几种用法:https://blog.csdn.net/qq_41763225/article/details/82890122
QUESTION 76
Given the code fragments:
class TechName {
String techName;
TechName (String techName) {
this.techName=techName;
}
}
and
List<TechName> tech = Arrays.asList (
new TechName(“Java-“),
new TechName(“Oracle DB-“),
new TechName(“J2EE-“)
);
Stream<TechName> stre = tech.stream();
//line n1
Which should be inserted at line n1 to print Java-Oracle DB-J2EE-?
A.
stre.forEach(System.out::print);
B.
stre.map(a-> a.techName).forEach(System.out::print);
C.
stre.map(a-> a).forEachOrdered(System.out::print);
D.
stre.forEachOrdered(System.out::print);
Correct Answer:
B
Section: (none)
Explanation
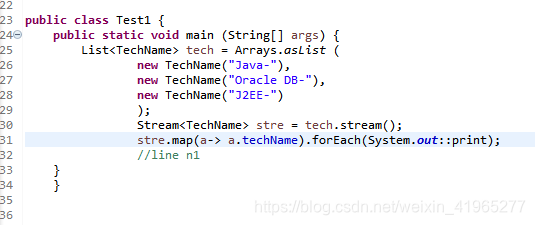
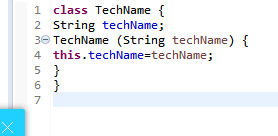
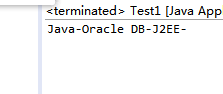
Explanation/Reference:
QUESTION 77
Given that
/green.txt
and
/colors/yellow.txt
are accessible, and the code fragment:
Path source = Paths.get(“/green.txt);
Path target = Paths.get(“/colors/yellow.txt);
Files.move(source, target, StandardCopyOption.ATOMIC_MOVE);
Files.delete(source);
Which statement is true?
A. The
green.txt
file content is replaced by the
yellow.txt
file content and the
yellow.txt
file is
deleted.
B. The
yellow.txt
file content is replaced by the
green.txt
file content and an exception is thrown.
C. The file
green.txt
is moved to the
/colors
directory.
D. A
FileAlreadyExistsException
is thrown at runtime.
Correct Answer:
D
Section: (none)
Explanation
Explanation/Reference:
QUESTION 78
Given:
interface Doable {
public void doSomething (String s);
}
Which two class definitions compile? (Choose two.)
A.
public abstract class Task implements Doable {
public void doSomethingElse(String s) { }
}
B.
public abstract class Work implements Doable {
public abstract void doSomething(String s) { }
public void doYourThing(Boolean b) { }
}
C.
public class Job implements Doable {
public void doSomething(Integer i) { }
}
D.
public class Action implements Doable {
public void doSomething(Integer i) { }
public String doThis(Integer j) { }
}
E.
public class Do implements Doable {
public void doSomething(Integer i) { }
public void doSomething(String s) { }
public void doThat (String s) { }
}
Correct Answer:
AE
Section: (none)
Explanation
Explanation/Reference:
QUESTION 79
Given the code fragment:
List<Integer> list1 = Arrays.asList(10, 20);
List<Integer> list2 = Arrays.asList(15, 30);
//line n1
Which code fragment, when inserted at line n1, prints 10 20 15 30?
A.
Stream.of(list1, list2)
.flatMap(list -> list.stream())
.forEach(s -> System.out.print(s + “ “));
B.
Stream.of(list1, list2)
.flatMap(list -> list.intStream())
.forEach(s -> System.out.print(s + “ “));
C.
list1.stream()
.flatMap(list2.stream().flatMap(e1 -> e1.stream())
.forEach(s -> System.out.println(s + “ “));
D.
Stream.of(list1, list2)
.flatMapToInt(list -> list.stream())
.forEach(s -> System.out.print(s + “ “));
Correct Answer:
A
Section: (none)
Explanation
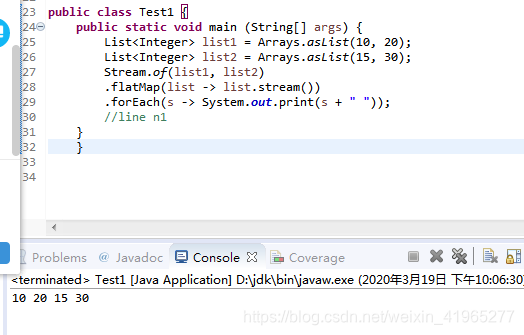
Explanation/Reference:
QUESTION 80
Given the code fragment:
public static void main (String[] args) throws IOException {
BufferedReader brCopy = null;
try (BufferedReader br = new BufferedReader (new FileReader
(“employee.txt”))) { //
line n1
br.lines().forEach(c -> System.out.println(c));
brCopy = br; //line n2
}
brCopy.ready(); //line n3;
}
Assume that the ready method of the BufferedReader, when called on a closed BufferedReader, throws an
exception, and employee.txt is accessible and contains valid text.
What is the result?
A. A compilation error occurs at
line n3
.
B. A compilation error occurs at
line n1
.
C. A compilation error occurs at
line n2
.
D. The code prints the content of the
employee.txt
file and throws an exception at
line n3
.
Correct Answer:
D
Section: (none)
Explanation
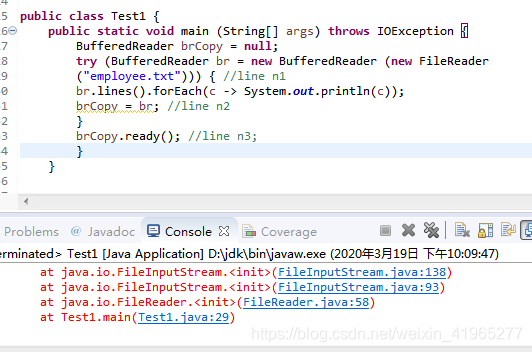
Explanation/Reference: