QUESTION 111
Given:
public class Product {
public double applyDiscount(double price)
{
assert(price>0);//line n1
return price*0.50;
}
public static void main(String[ ] args)
{
Product p =new Product();
double newPrice=
p.applyDiscount(Double.parseDouble(args[0]));
System.out.println("New Price: "+newPrice);
}
}
and the command:
java Product 0
What is the result?
A. An
AssertionError
is thrown.
B. A compilation error occurs at
line n1
.
C.
New Price: 0.0
D. A
NumberFormatException
is thrown at run time.
Correct Answer:
C
Section: (none)
Explanation
Explanation/Reference:
QUESTION 112
Given the code fragment:
LocalTime now =LocalTime.now();
long timeToBreakfast=0;
LocalTime office_start =LocalTime.of(7, 30);
if(office_start.isAfter(now)) {
timeToBreakfast=now.until(office_start, MINUTES);
}else {
timeToBreakfast=now.until(office_start, HOURS);
}
System.out.println(timeToBreakfast);
Assume that the value of now is 6:30 in the morning.
What is the result?
A. An exception is thrown at run time.
B.
0
C.
60
D.
1
Correct Answer:
C
Section: (none)
Explanation
Explanation/Reference:
QUESTION 113
Given the code fragments:
class R implements Runnable{
public void run() {System.out.println("Run...");}
}
class C implements Callable<String > {
public String call() throws Exception{return "Call...";}
}
and
ExecutorService es=Executors.newSingleThreadExecutor();
es.execute(new R()); //line n1
Future<String> f1=es.submit(new C()); //line n2
System.out.println(f1.get());
es.shutdown();
What is the result?
A. The program prints
Run…
and throws an exception.
B. A compilation error occurs at
line n1.
C.
Run…
Call…
D. A compilation error occurs at
line n2.
Correct Answer:
C
Section: (none)
Explanation
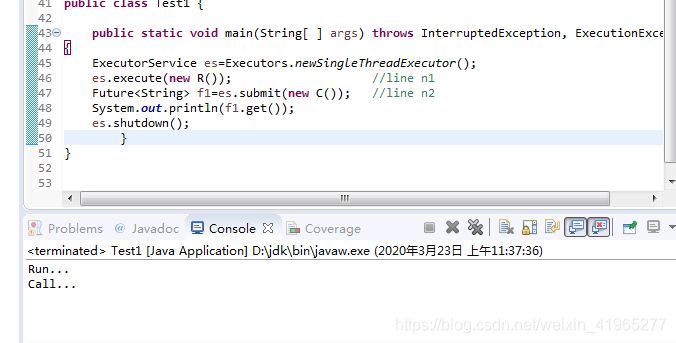
Explanation/Reference:
QUESTION 114
Which two are elements of a singleton class? (Choose two.)
A. a
transient
reference to point to the single instance
B. a
public
method to instantiate the single instance
C. a
public static
method to return a copy of the singleton reference
D. a
private
constructor to the class
E. a
public
reference to point to the single instance
Correct Answer:
BD
Section: (none)
Explanation
Explanation/Reference:
QUESTION 115
Given the code fragment:
Deque<String> queue=new ArrayDeque<>();
queue.add("Susan");
queue.add("Allen");
queue.add("David");
System.out.println(queue.pop());
System.out.println(queue.remove());
System.out.println(queue);
What is the result?
A.
David
David
[Susan, Allen]
B.
Susan
Susan
[Susan, Allen]
C.
Susan
Allen
[David]
D.
David
Allen
[Susan]
E.
Susan
Allen
[Susan, David]
Correct Answer:
C
Section: (none)
Explanation
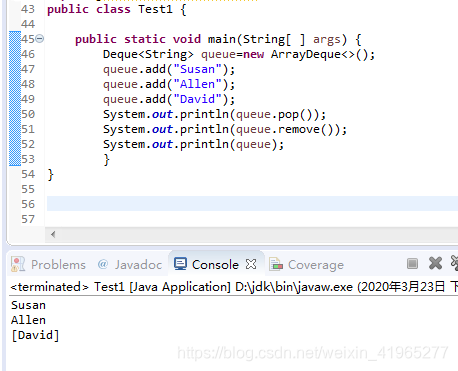
Explanation/Reference:
queue和deque说明:
https://blog.csdn.net/shf4715/article/details/47052385
QUESTION 116
Given:
public class Vehicle {
int vId;
String vName;
public Vehicle(int vIdArg,String vNameArg) {
this.vId=vIdArg;
this.vName=vNameArg;
}
public int getVId() {return vId;}
public String getVName() {return vName;}
public String toString() {
return vName;
}
}
and the code fragment:
List<Vehicle> vehicle=Arrays.asList(
new Vehicle(2,"Car"),
new Vehicle(3,"Bike"),
new Vehicle( 1,"Truck"));
vehicle.stream()
//line n1
.forEach(System.out::print);
Which two code fragments, when inserted at
line n1
independently, enable the code to print
TruckCarBike
?
A.
.sorted ((v1, v2) -> v1.getVId() < v2.getVId())
B.
.sorted (Comparable.comparing (Vehicle: :getVName)).reversed ()
C.
.map (v -> v.getVid())
.sorted ()
D.
.sorted((v1, v2) -> Integer.compare(v1.getVId(), v2.getVId()))
E.
.sorted(Comparator.comparing ((Vehicle v) -> v.getVId()))
Correct Answer:
DE
Section: (none)
Explanation
D:
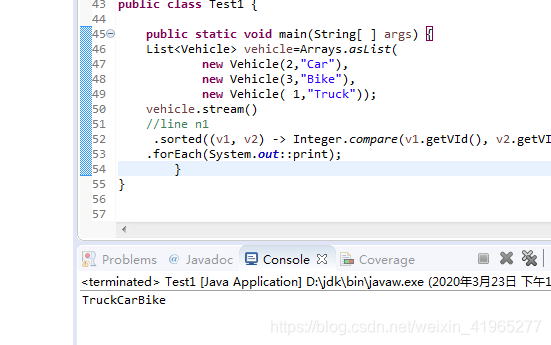
E:
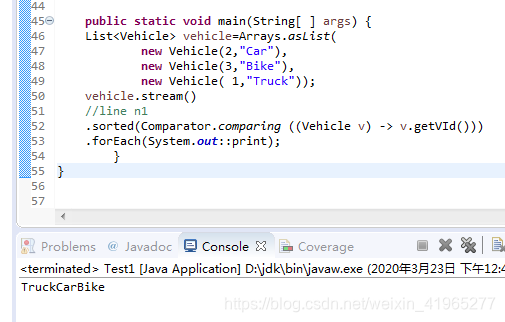
Explanation/Reference:
QUESTION 117
Given the code fragment:
List<String> valList =Arrays.asList("","George","","John","Jim");
Long newVal= valList.stream() //line n1
.filter(x->!x.isEmpty())
.count(); //line n2
System.out.print(newVal);
What is the result?
A. A compilation error occurs at
line n2
.
B.
3
C.
2
D. A compilation error occurs at
line n1.
Correct Answer: B
Section: (none)
Explanation
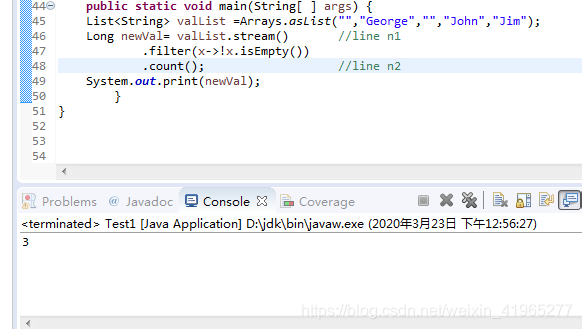
Explanation/Reference:
QUESTION 118
Given the code fragment:
//Login time:2015-01-12T21:58:18.817z
Instant loginTime =Instant.now();
Thread.sleep(1000);
//Logout time:2015-01-12T21:58:19.880z
Instant logoutTime=Instant.now();
loginTime =loginTime.truncatedTo(ChronoUnit.MINUTES); //line n1
logoutTime=logoutTime.truncatedTo(ChronoUnit.MINUTES);
if(logoutTime.isAfter(loginTime))
System.out.println("Logged out at:"+logoutTime);
else
System.out.println("Can't logout");
What is the result?
A. A compilation error occurs at
line n1.
B.
Logged out at: 2015-01-12T21:58:19.880Z
C.
Can’t logout
D.
Logged out at: 2015-01-12T21:58:00Z
Correct Answer:
D
Section: (none)
Explanation
Explanation/Reference:
QUESTION 119
Given the code fragment:
List<String> words =Arrays.asList("win","try","best","luck","do");
Predicate<String> test1=w->{
System.out.println("Checling...");
return w.equals("do"); //line n1
};
Predicate test2=(String w)->w.length()>3; //line n2
words.stream().filter(test2).filter(test1).count();
What is the result?
A. A compilation error occurs at
line n1.
B.
Checking…
C.
Checking…
Checking…
D. A compilation error occurs at
line n2
.
Correct Answer: D
Section: (none)
Explanation
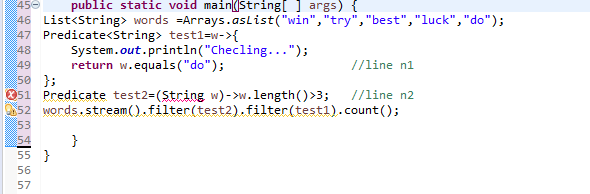
error:
Lambda expression's parameter w is expected to be of type Object
Explanation/Reference:
QUESTION 120
Assume
customers.txt
is accessible and contains multiple lines.
Which code fragment prints the contents of the
customers.txt
file?
A.
Stream<String> stream = Files.find (Paths.get (“customers.txt”));
stream.forEach((String c) -> System.out.println(c));
B.
Stream<Path> stream = Files.find (Paths.get (“customers.txt”));
stream.forEach( c) -> System.out.println(c));
C.
Stream<Path> stream = Files.list (Paths.get (“customers.txt”));
stream.forEach( c) -> System.out.println(c));
D.
Stream<String> lines = Files.lines (Paths.get (“customers.txt”));
lines.forEach( c) -> System.out.println(c));
Correct Answer:
D
Section: (none)
Explanation
Explanation/Reference: