常用类
Object类
getClass方法
hashCode方法
toString方法
equals方法
finalize方法
package com.ljh.usualClass;
public class Application {
public static void main(String[] args) {
//1.getClass方法
Student s1 = new Student();
Student s2= new Student();
//判断s1和s2是不是同一个类型
Class class1 = s1.getClass();
Class class2 = s2.getClass();
if(class1 == class2){
System.out.println("s2和s2属于同一个类型");
}else{
System.out.println("s2和s2不属于同一个类型");
}
System.out.println("-------------2hashCode--------------");
//2.hashCode方法
System.out.println(s1.hashCode());
System.out.println(s2.hashCode());
Student s3 = s1;
System.out.println(s3.hashCode());
System.out.println("-------------3toString--------------");
System.out.println(s1.toString());
System.out.println(s2.toString());
//4equals方法,判断两个对象是否相等
System.out.println("-------------4equals--------------");
System.out.println(s1.equals(s2));
//判断对象是不是相等,可以用getClass方法比较两个对象,
// 也可以用instanceof
//5finalize方法用于垃圾回收
}
}
包装类
类型转换与装箱和拆箱
基础类型在栈,引用类型在堆里面
-
基础类型–>引用类型-装箱
-
引用类型–>基础类型-拆箱
package com.ljh.usualClass;
public class Demo1 {
public static void main(String[] args) {
//类型转换:装箱,基本类型转换成引用类型的过程
//基本类型
int num = 10;
//使用Integer类型创建对象
Integer integer1 = new Integer(num);
Integer integer2 = Integer.valueOf(num);
//类型转换:拆箱,引用类型转换成基本类型的过程
Integer integer3 = new Integer(100);
int num2 = integer3.intValue();
//JDK1.5之后,提供自动装箱和拆箱
int age = 30;
//自动装箱
Integer integer4 = age;
//自动拆箱
int age2 = integer4;
//基本类型和字符串之间转换
//1.基本类型转成字符串
int n1 = 100;
//1.1使用+号
String s1 = n1 + "";
//1.2使用Integer中的toString()方法
String s2 = Integer.toString(n1,16);//f
System.out.println(s1);
System.out.println(s2);
//2字符串转成基本类型
String str = "150";
//使用Integer.parsexxx();
int n2 = Integer.parseInt(str);
System.out.println(n2);
//boolean字符串形式转成基本类型,"true"--->true 非"true"--->false
String str2 = "false";
boolean b1 = Boolean.parseBoolean(str2);
System.out.println(b1);
}
}
Integer整数缓冲区
package com.ljh.usualClass;
public class Demo2 {
public static void main(String[] args) {
//面试题
Integer integer1 = new Integer(100);
Integer integer2 = new Integer(100);
System.out.println(integer1 == integer2);//false
Integer integer3 = 100;//自动装箱 装箱就是 Integer integer3 = Integer.valueof(100);
Integer integer4 = 100;
System.out.println(integer3 == integer4);//true
Integer integer5= 200;//自动装箱Integer integer5 = Integer.valueof(200);
Integer integer6 = 200;
System.out.println(integer5 == integer6);//false
//在Integer类中我们看到valueOf()方法中,能够知道缓冲区中范围是-128~127
// public static Integer valueOf(int i) {
// if (i >= Integer.IntegerCache.low && i <= Integer.IntegerCache.high)
// return Integer.IntegerCache.cache[i + (-Integer.IntegerCache.low)];
// return new Integer(i);
// }
// private static class IntegerCache {
// static final int low = -128;
// static final int high;
// static final Integer cache[];
//
// static {
// // high value may be configured by property
// int h = 127;
// String integerCacheHighPropValue =
// sun.misc.VM.getSavedProperty("java.lang.Integer.IntegerCache.high");
// if (integerCacheHighPropValue != null) {
// try {
// int i = parseInt(integerCacheHighPropValue);
// i = Math.max(i, 127);
// // Maximum array size is Integer.MAX_VALUE
// h = Math.min(i, Integer.MAX_VALUE - (-low) -1);
// } catch( NumberFormatException nfe) {
// // If the property cannot be parsed into an int, ignore it.
// }
// }
// high = h;
//
// cache = new Integer[(high - low) + 1];
// int j = low;
// for(int k = 0; k < cache.length; k++)
// cache[k] = new Integer(j++);
//
// // range [-128, 127] must be interned (JLS7 5.1.7)
// assert Integer.IntegerCache.high >= 127;
// }
//
// private IntegerCache() {}
// }
}
}
String类概述
package com.ljh.usualClass;
public class Demo3 {
public static void main(String[] args) {
String name = "hello";//"hello"常量存储在字符串池中
name = "zhangsan";//“张三”赋值给name变量,给字符串赋值时,并没有修改数据,而是重新开辟一个空间
String name2 = "zhangsan";
System.out.println(name == name2);
//演示字符串的另一种创建方式
String str1 = new String("java");//浪费空间
String str2 = new String("java");//浪费空间
System.out.println(str1 == str2);
System.out.println(str1.equals(str2));
//字符串方法的使用
//1.length();返回字符串的长度
//2.charAt(int index);返回某个位置的字符
//3.cantains(String str);判断是否包含某个字符串
String content = "java是世界上最好的语言";
System.out.println(content.length());
System.out.println(content.charAt(content.length()-1));
System.out.println(content.contains("java"));
//4.toCharArray()将字符转换为数组
//5.indexOf();返回字符串首次出现的位置
//6.lastIdexOf();返回字符串最后一次出现的位置
System.out.println(content.toCharArray());
System.out.println(content.indexOf("java"));
System.out.println(content.indexOf("java",4));
System.out.println(content.lastIndexOf("java"));
//7.trim();去掉字符串前后的空格
//8.toUpperCase();//把小写转成大写toLowerCase();把大写转成小写
//9.endWith(str);判断是否以str结尾,startWith(str);判断是否以str开头
//10.replace();用心的字符或字符串替换旧的字符或字符串
//11.split();对字符串进行拆分
//补充两个方法equals,compare();比较大小
System.out.println("---------------------");
String s1 = "hello";
String s2 = "HELLO";
System.out.println(s1.equalsIgnoreCase(s2));
String s3 = "abc";//97
String s4 = "ayzawe";
System.out.println(s3.compareTo(s4));
}
}
StringBuffer和StringBuilder
package com.ljh.usualClass;
/**
* StringBuffer和StringBuilder的使用
* 和String区别(1)效率比String高(2)比String节省内存
*/
public class Demo4 {
public static void main(String[] args) {
StringBuffer sb = new StringBuffer();
// StringBuilder sb = new StringBuilder();
//1.appened();追加
sb.append("java");
System.out.println(sb.toString());
sb.append("世界");
System.out.println(sb.toString());
//insert();添加
sb.insert(0,"我在前面");
//3.replace();替换
sb.replace(0,5,"hello");
//4.delete();删除
sb.delete(0,5);
System.out.println(sb.toString());
//清空
sb.delete(0,sb.length());
System.out.println(sb);
}
}
package com.ljh.usualClass;
/**
* 验证StringBuilder效率高于String
*/
public class Demo5 {
public static void main(String[] args) {
//开始时间
long start = System.currentTimeMillis();
// String string = "";
// for (int i = 0; i < 99999; i++) {
// string +=i;
//
// }
StringBuilder string = new StringBuilder();
for (int i = 0; i < 99999; i++) {
string.append(i);
}
System.out.println(string);
long end = System.currentTimeMillis();
System.out.println("用时:"+(end-start));
}
}
Date
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-XOsuGqWr-1620664517933)(C:\Users\86136\AppData\Roaming\Typora\typora-user-images\image-20210511002706890.png)]
package com.ljh.usualClass;
import java.util.Date;
public class Demo7 {
public static void main(String[] args) {
//1.创建Date对象
//今天
Date date1 = new Date();
System.out.println(date1.toString());
System.out.println(date1.toLocaleString());
//昨天
Date date2 = new Date(date1.getTime() - (60 * 60 * 24 * 1000));
System.out.println(date2.toLocaleString());
//2.方法after before
boolean after = date1.after(date2);
System.out.println(after);
boolean before = date1.before(date2);
System.out.println(before);
//比较compareTo();
int d = date2.compareTo(date1);
System.out.println(d);
//比较是否相等equals
boolean equals = date1.equals(date2);
System.out.println(equals);
}
}
Calendar
package com.ljh.usualClass;
import java.util.Calendar;
public class Demo8 {
public static void main(String[] args) {
//1.创建Calendar对象
Calendar calendar = Calendar.getInstance();
System.out.println(calendar.getTime().toLocaleString());
System.out.println(calendar.getTimeInMillis());
//2.获取时间信息
//获取年
int year = calendar.get(Calendar.YEAR);
//月
int month = calendar.get(Calendar.MONTH);
//日
int day = calendar.get(Calendar.DAY_OF_MONTH);
//小时
int hour = calendar.get(Calendar.HOUR_OF_DAY);
//分
int minute = calendar.get(Calendar.MINUTE);
//秒
int second = calendar.get(Calendar.SECOND);
//3.修改时间
Calendar calendar1 = Calendar.getInstance();
calendar1.set(Calendar.DAY_OF_MONTH,5);
System.out.println(calendar1.getTime().toLocaleString());
//4add方法修改时间
calendar1.add(Calendar.HOUR,-1);
System.out.println(calendar1.getTime().toLocaleString());
//5补充方法
int actualMaximum = calendar1.getActualMaximum(Calendar.DAY_OF_MONTH);
int actualMinimum = calendar1.getActualMinimum(Calendar.DAY_OF_MONTH);
System.out.println(actualMaximum);
System.out.println(actualMinimum);
}
}
SimpleDateFormat
package com.ljh.usualClass;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Demo9 {
public static void main(String[] args) throws ParseException {
//1.创建simpleDateFormat对象y年M月
SimpleDateFormat sdf = new SimpleDateFormat("yyy年MM月dd日 HH:mm:ss");
//2.创建Date
Date date = new Date();
//格式化date(把日期转换为字符串)
String str = sdf.format(date);
System.out.println(str);
//解析(把字符串转换为日期)
Date date2 = sdf.parse("1990年05月01日 12:01:01");
System.out.println(date2);
}
}
System类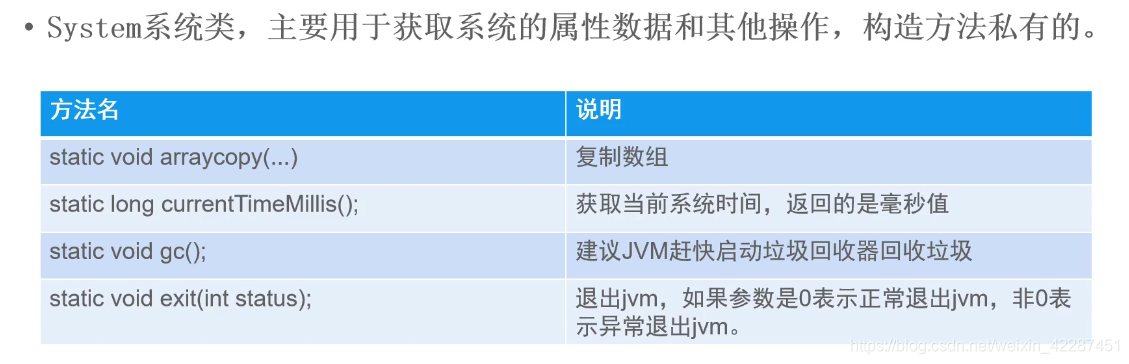
package com.ljh.usualClass;
public class Demo10 {
public static void main(String[] args) {
//arraycopy 数组的复制
//src :源数组
//srcPos:从哪个位置开始复制0
//dest:目标数组
//destPos:目标数组的位置
int[] arr = {20,10,9,22,12,323,32,22};
int[] dest = new int[8];
System.arraycopy(arr,4,dest,4,4);
long start = System.currentTimeMillis();
for (int i = 0; i < 999999; i++) {
}
long end = System.currentTimeMillis();
System.out.println("用时"+(end-start));
new Student("ljh");
//3垃圾回收
System.gc();//告诉垃圾回收期回收
//4.退出jvm
System.exit(0);
System.out.println("程序结束了");
}
}