Collection集合
集合概述
集合是Java提供的一种存储多个引用数据类型的容器,如果想用存储基本数据类型,需要存储对应的包装类型。
集合相比数组而言
- 数组的长度是固定的,而集合的长度是可变的
- 数组中存储的是同一个类型的数据,而集合中可以存储任意类型的数据
集合分为单列集合和多列集合两种。
其中单列集合的顶层接口是java.util.Collection,多列集合的顶层接口是java.util.Map
Collection集合的继承体系
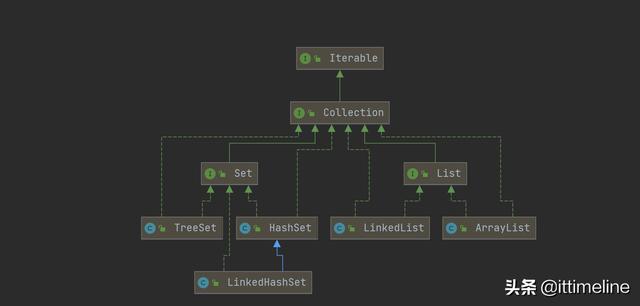
Collection集合的继承体系
Collection接口有两个常用的子接口,java.util.List和java.util.Set
- List集合中的元素是有顺序,而且可以重复
- Set集合的元素是无序的,但是不可以重复(也就是唯一)
List集合常用的实现类有java.util.ArrayList和java.util.LinkedList
Set集合常用的实现类有java.util.HashSet、java,util,LinkedHashSet、java.util.TreeSet
Collection集合的常用方法
java.util.Collection集合是所有单列集合的顶层接口,因此所有单列集合的实现类(ArrayList,LinkedList,HashSet,LinkedHashSet,TreeSet)都会实现该接口的方法,这里以ArrayList作为Collection接口的实现类来创建Collection对象后使用其方法。
集合操作的都是引用数据类型,这里还是引用之前使用过的Employee JavaBean作为集合的元素
package net.ittimeline.java.core.jdk.api.lang.bean;import java.util.Objects;/** * 员工类 默认会继承java.lang.Object * * @author tony [email protected] * @version 2020/12/16 12:58 * @since JDK11 */public class Employee extends Object { /*************************私有化成员变量*****************************************/ /** * 员工编号 */ private String employeeNo; /** * 姓名 */ private String name; /** * 年龄 */ private Integer age; /** * 默认无参数构造器 */ public Employee(){} /** * 全参数构造器 * @param employeeNo * @param name * @param age */ public Employee(String employeeNo, String name, Integer age) { this.employeeNo = employeeNo; this.name = name; this.age = age; } /**************************get/set方法*****************************************/ public String getEmployeeNo() { return employeeNo; } public void setEmployeeNo(String employeeNo) { this.employeeNo = employeeNo; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Integer getAge() { return age; } public void setAge(Integer age) { this.age = age; } /** * 重写父类java.lang.Object的toString()方法 * @return 对象的属性信息的字符串 * @see Object#toString() */ @Override public String toString() { return "Employee{" + "employeeNo='" + employeeNo + ''' + ", name='" + name + ''' + ", age=" + age + '}'; } /** * 重写java.lang.Object的equals()方法 * @param object * @return * @see Object#equals(Object) */ @Override public boolean equals(Object object) { //如果两个对象的地址相等则返回true if(this==object){ return true; } //方法的参数为空或者类型不一样返回false if(null==object||this.getClass()!=object.getClass()){ return false; } Employee targetEmployee= (Employee) object; //判断如果员工编号一样就表示同一个员工 return Objects.equals(this.getEmployeeNo(),targetEmployee.getEmployeeNo()); } @Override public int hashCode() { return Objects.hash(employeeNo, name, age); }}
- 集合元素的添加和清除
- add()方法是往集合中添加元素
- clear()方法是清除集合中所有的元素
/** * add(E) 往集合中添加指定的元素 * clear() 清除集合中指定的元素 * @see ArrayList#add(Object) * @see ArrayList#clear() */ @Test public void testCollectionAddClear(){ //创建一个Collection对象,实现类是ArrayList // 表示限制集合中的元素只能是Employee类型 Collection employees=new ArrayList<>(); Employee ittimeline00001=new Employee(); ittimeline00001.setEmployeeNo("ittimeline00001"); ittimeline00001.setName("tony"); ittimeline00001.setAge(28); Employee ittimeline00002=new Employee(); ittimeline00002.setEmployeeNo("ittimeline00002"); ittimeline00002.setName("jack"); ittimeline00002.setAge(32); Employee ittimeline00003=new Employee(); ittimeline00003.setEmployeeNo("ittimeline00003"); ittimeline00003.setName("tom"); ittimeline00003.setAge(26); //往集合中添加3个Employee对象 //add 方法是往集合中添加添加指定的对象 employees.add(ittimeline00001); employees.add(ittimeline00002); employees.add(ittimeline00003); System.out.println("往集合中添加三个元素"+employees); //清空集合的元素 employees.clear(); System.out.println("清空集合的元素"+employees); }
- 集合元素的删除和包含
- remove() 删除指定的元素
- contains() 集合中是否包含指定的元素
/** * 集合元素的删除和包含 * remove()删除指定的集合元素 * contains()是否包含指定的元素 * @see ArrayList#remove(Object) * @see ArrayList#contains(Object) */ @Test public void testCollectionRemoveContains(){ Collection employees=new ArrayList<>(); Employee ittimeline00001=new Employee(); ittimeline00001.setEmployeeNo("ittimeline00001"); ittimeline00001.setName("tony"); ittimeline00001.setAge(28); employees.add(ittimeline00001); System.out.println("添加元素之后的集合"+employees); boolean removeSuccess = employees.remove(ittimeline00001); System.out.println("删除元素的返回值:"+removeSuccess); System.out.println("删除元素之后的集合"+employees); boolean contains = employees.contains(ittimeline00001); System.out.println("删除元素之后的集合是否包含指定元素返回值"+contains); }
程序运行结果
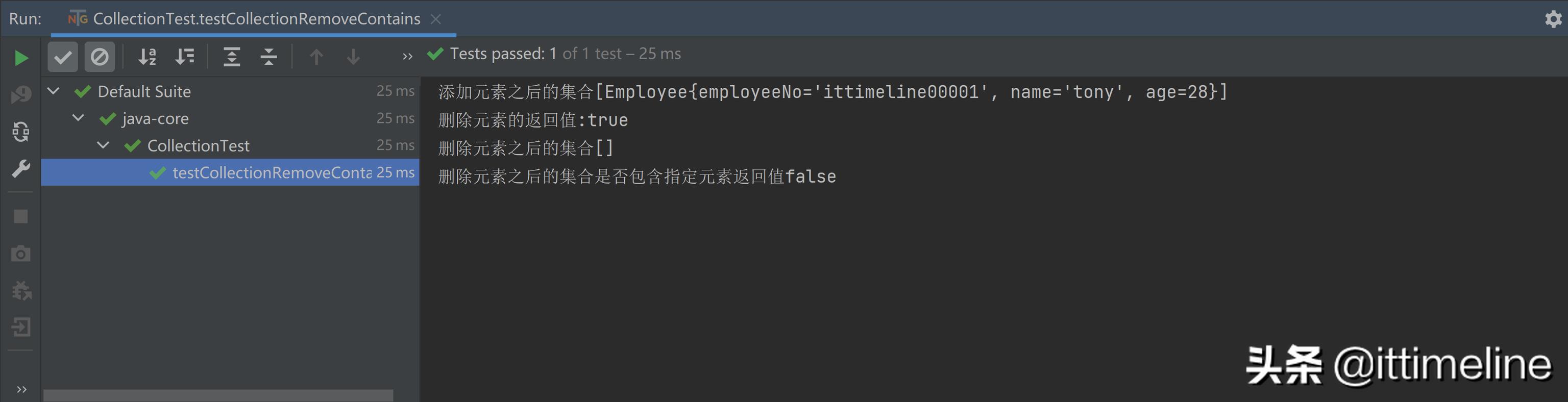
- 集合元素的非空判断以及集合的个数
- isEmpty() 判断当前集合是否为空
- size()获取元素的个数
/** * 集合的非空判断以及获取元素个数 * isEmpty() 判断集合是否为空 * size() 获取集合的元素个数 * @see ArrayList#isEmpty() * @see ArrayList#size() */ @Test public void testCollectionIsEmptySize(){ Collection employees=new ArrayList<>(); Employee ittimeline00001=new Employee(); ittimeline00001.setEmployeeNo("ittimeline00001"); ittimeline00001.setName("tony"); ittimeline00001.setAge(28); employees.add(ittimeline00001); System.out.println("添加元素之后的集合"+employees); System.out.println("添加元素之后的集合元素个数是"+employees.size()); System.out.println("当前集合元素否为空:"+employees.isEmpty()); employees.clear(); System.out.println("清空集合元素后当前集合元素否为空:"+employees.isEmpty()); }
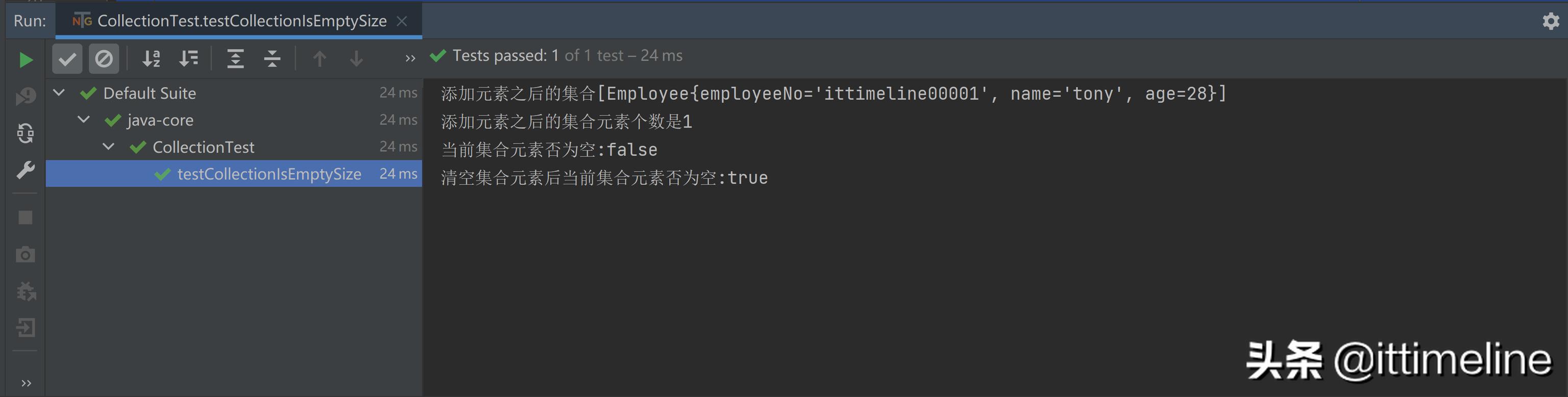
程序运行结果
- 集合和数组的相互转换
- toArray() 方法将集合转换为数组
- Arrays.asList(Object[]) 将数组转换为集合
/** * 集合和数组元素的相互转换 * @see ArrayList#toArray() * @see Arrays#asList(Object[]) */ @Test public void testCollectionToArray(){ Collection employees=new ArrayList<>(); Employee ittimeline00001=new Employee(); ittimeline00001.setEmployeeNo("ittimeline00001"); ittimeline00001.setName("tony"); ittimeline00001.setAge(28); Employee ittimeline00002=new Employee(); ittimeline00002.setEmployeeNo("ittimeline00002"); ittimeline00002.setName("jack"); ittimeline00002.setAge(32); employees.add(ittimeline00001); employees.add(ittimeline00002); System.out.println("添加元素之后的集合"+employees); //将employees转换为数组 Employee[] employeeArray = employees.toArray(new Employee[employees.size()]); System.out.println("employees集合转换为数组后" + Arrays.toString(employeeArray)); //将employeeArray转换为集合 List employeeList = Arrays.asList(employeeArray); System.out.println("employeeArray数组转换为集合后" +employeeList); }
程序运行结果
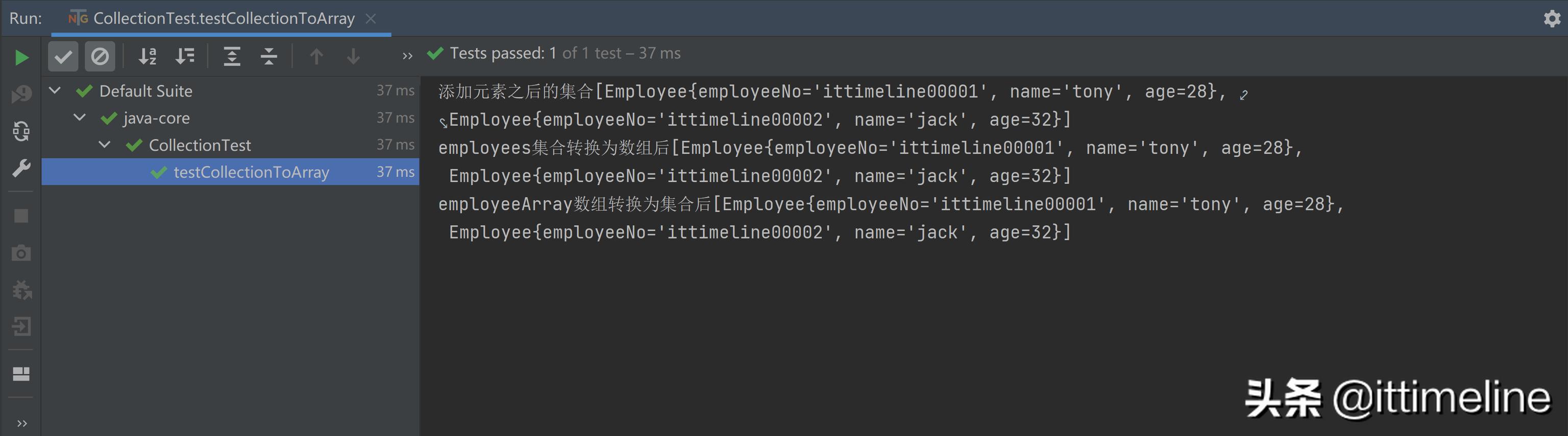
迭代器
迭代器概述
迭代指的是Collection集合获取集合元素的通用方式