import sqlite3fromQueue import Queuefromthreading import ThreadclassSqliteMultithread(Thread):''' Wrap sqlite connection in a way that allows concurrent requests frommultiple threads. Thisisdone by internally queueing the requests and processing them sequentiallyin a separate thread (inthe same order they arrived).'''def __init__(self, filename, autocommit, journal_mode): super(SqliteMultithread, self).__init__() self.filename=filename self.autocommit=autocommit self.journal_mode=journal_mode self.reqs=Queue() # use request queue of unlimited size self.setDaemon(True) # python2.5-compatible self.start() def run(self):ifself.autocommit: conn= sqlite3.connect(self.filename, isolation_level=None, check_same_thread=False)else: conn= sqlite3.connect(self.filename, check_same_thread=False) conn.execute('PRAGMA journal_mode = %s' %self.journal_mode) conn.text_factory=str cursor=conn.cursor() cursor.execute('PRAGMA synchronous=OFF')whileTrue: req, arg, res= self.reqs.get()if req == '--close--':breakelif req== '--commit--': conn.commit()else: cursor.execute(req, arg)ifres:for rec incursor: res.put(rec) res.put('--no more--')ifself.autocommit: conn.commit() conn.close() def execute(self, req, arg=None, res=None):''' `execute` calls are non-blocking: just queue up the request and returnimmediately.'''self.reqs.put((req, arg or tuple(), res)) def executemany(self, req, items):for item initems: self.execute(req, item) defselect(self, req, arg=None):''' Unlike sqlite's native select, this select doesn't handle iteration efficiently. The result of `select` starts filling up with values as soon asthe requestisdequeued, and although you can iterate over the result normally (`for res in self.select(): ...`), the entire result will be inmemory.''' res = Queue() # results of the select will appear as items in thisqueue self.execute(req, arg, res)whileTrue: rec= res.get()if rec == '--no more--':break yieldrec def select_one(self, req, arg=None):'''Return only the first row of the SELECT, or None if there are no matching rows.''' try:return iter(self.select(req, arg)).next() except StopIteration:returnNone def commit(self): self.execute('--commit--') def close(self): self.execute('--close--')#endclass SqliteMultithread
python多线程访问sqlite3_python操作sqlite示例(支持多进程/线程同时操作) - 学步园...
最新推荐文章于 2024-08-24 22:04:32 发布
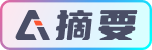