原创文章,转载请注明原文章地址,谢谢!
根据公司业务需要实现发送短信验证码的功能,于是开通了阿里的短信功能,单条短信0.045元左右,可以购买套餐包,相对比较优惠。
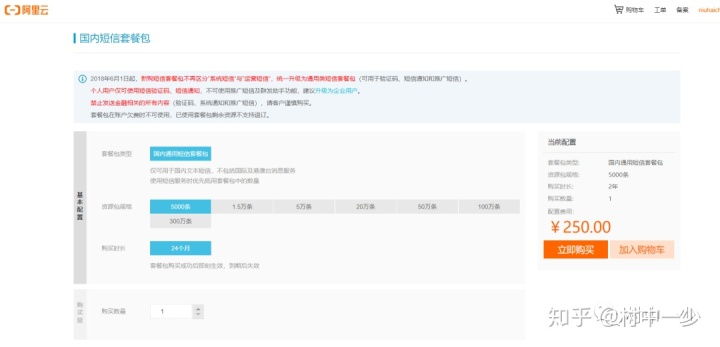
第一步、短信配置
1)购买成功后,进入短信服务控制台进行参数配置
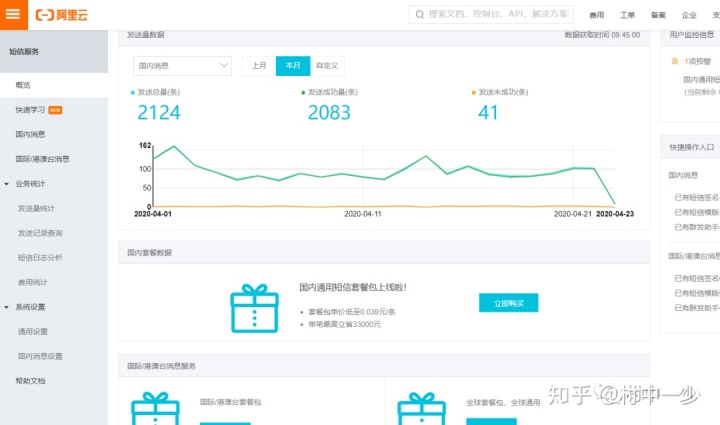
2)点击左侧“国内信息”
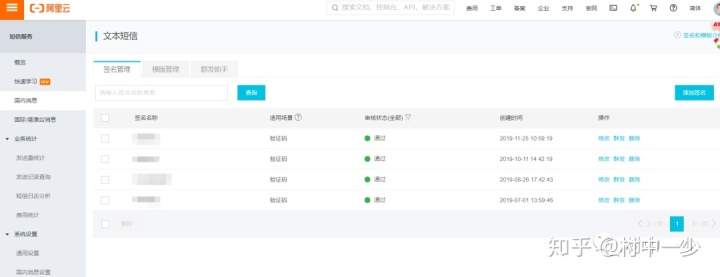
3)签名配置
说明:签名为接收短信时显示的发送方,一般为app名称或公司名,其它按提示填写完等待审核就行。
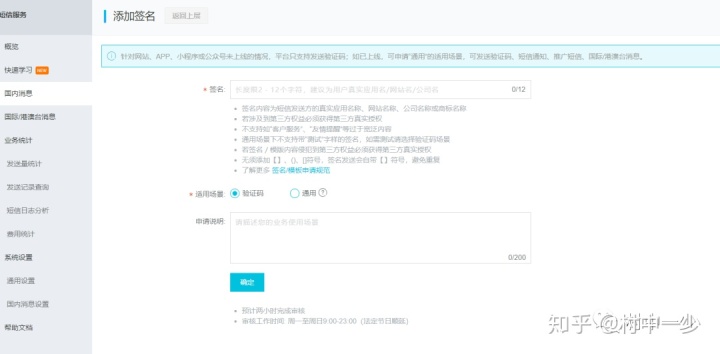
4)模板配置
模板配置为短信内容的配置,模板名称一般与签名相同就可,模板内容可灵活设置,如 “您的 验证码为:${code},2分钟内有效。如非本人操作,请忽略本短信!”
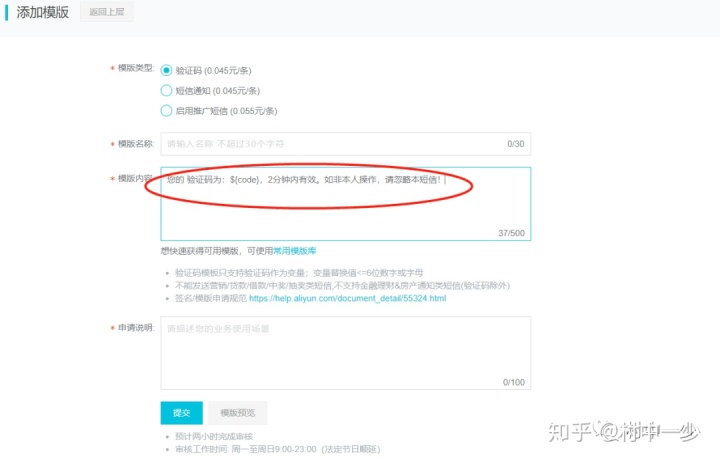
审核通过后,会生成模板CODE,这个需要用到。

第二步、springboot对接
1)引入依赖
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>aliyun-java-sdk-core</artifactId>
<version>4.1.0</version>
</dependency>
2)service
import com.alibaba.fastjson.JSONObject;
import com.aliyuncs.CommonRequest;
import com.aliyuncs.CommonResponse;
import com.aliyuncs.DefaultAcsClient;
import com.aliyuncs.IAcsClient;
import com.aliyuncs.exceptions.ClientException;
import com.aliyuncs.exceptions.ServerException;
import com.aliyuncs.http.MethodType;
import com.aliyuncs.profile.DefaultProfile;
import com.qh.app.repository.AppConfigRepository;
import com.qh.app.service.SmsConfigService;
import com.qh.common.entity.ConfigEntity;
import com.qh.common.exception.BizException;
import com.qh.common.exception.ResultEnum;
import com.qh.common.response.SmsResponse;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class SmsConfigServiceImpl implements SmsConfigService {
public final static Logger logger = LoggerFactory.getLogger(SmsConfigServiceImpl.class);
@Autowired
private AppConfigRepository appConfigRepository;
/**
* 发送校验码
*/
@Override
public String send(String phone,String companyNum) {
ConfigEntity configEntity = getConfigEntity(companyNum);
DefaultProfile profile = DefaultProfile.getProfile("default", configEntity.getSmsAkId(), configEntity.getSmsAkSecret());
IAcsClient client = new DefaultAcsClient(profile);
CommonRequest request = new CommonRequest();
//request.setProtocol(ProtocolType.HTTPS);
request.setMethod(MethodType.POST);
request.setDomain("dysmsapi.aliyuncs.com");
request.setVersion("2017-05-25");
request.setAction("SendSms");
request.putQueryParameter("PhoneNumbers", phone);
request.putQueryParameter("SignName", configEntity.getSmsSignName());
request.putQueryParameter("TemplateCode", configEntity.getSmsTemplateCode());
String verifyCode =getCode();
request.putQueryParameter("TemplateParam", "{"code":"" + verifyCode + ""}");
try {
CommonResponse response = client.getCommonResponse(request);
logger.info("发送校验码返回结果:{}",response.getData());
SmsResponse smsResponse = JSONObject.parseObject(response.getData(),SmsResponse.class);
if(!"OK".equals(smsResponse.getCode())){
throw new BizException(ResultEnum.VERIFY_CODE_SEND_ERR);
}
return verifyCode;
} catch (ServerException e) {
e.printStackTrace();
throw new BizException(ResultEnum.VERIFY_CODE_SEND_ERR);
} catch (ClientException e) {
e.printStackTrace();
throw new BizException(ResultEnum.VERIFY_CODE_SEND_ERR);
}
}
private ConfigEntity getConfigEntity(String companyNum){
ConfigEntity configEntity = appConfigRepository.queryByCompanyNum(companyNum);
if(configEntity==null){
throw new BizException(ResultEnum.COMPANYNUM_NO_CONFIG);
}
return configEntity;
}
private static String getCode() {
return ((int) ((Math.random() * 9 + 1) * 100000))+"";
}
}
短信发送需要四个参数:AkId、AkSecret、signName、templateCode,其中AkId、AkSecret是阿里云账号的AkId、AkSecret,signName是上面设置的短信签名名称,templateCode是上面设置的短信模板code。这四个参数我配置在了数据库中,通过ConfigEntity获取。
getCode方法为随机生成的短信验证码的方法。
3)演示
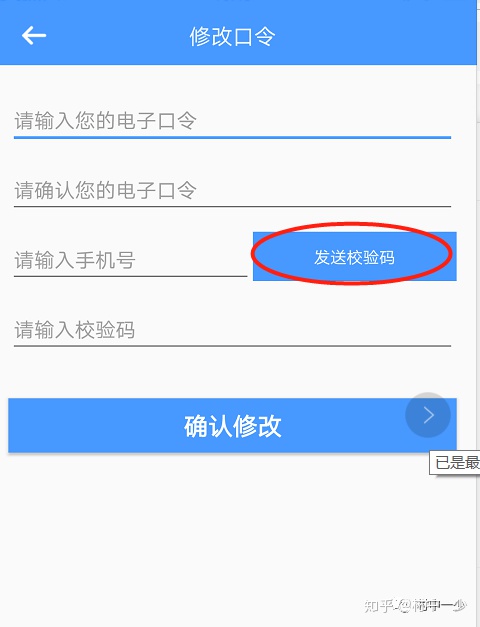
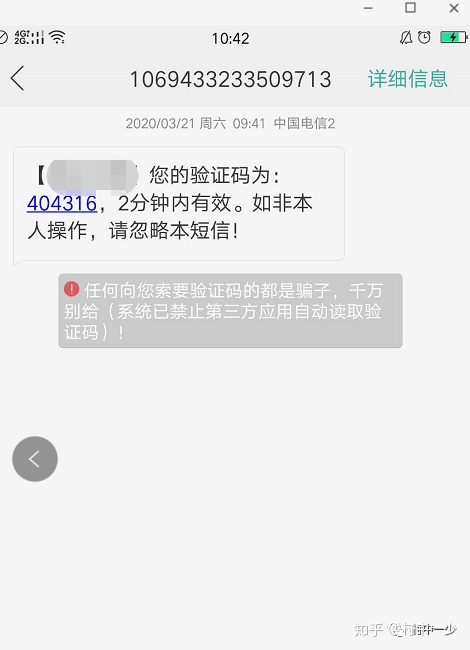
点击"验证码"后,收到短信如上,至此,对接成功。
坑:用了一段时间后发现阿里云短信的一个问题,通知信息里没有显示图标和公司app名称,咨询客服后回复说是还没有实现此功能。好吧!!!
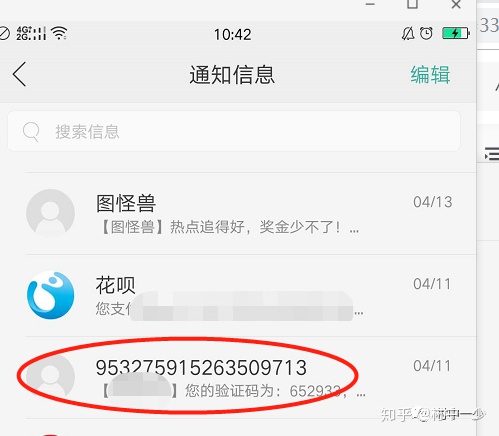