无意中发现一个几篇很nice的Python英文推文,思路、结构都清晰。在此,结合自己的理解,稍作翻译与内容讲解,虽然都是英文的,但是木有想象中的难的,小伙子加油哈!
本节知识点:Python中function的基本概念
idea behind function
A function is a set of statements that take inputs, do some specific computation and produces output. The idea is to put some commonly or repeatedly done task together and make a function, so that instead of writing the same code again and again for different inputs, we can call the function.
译:(1) 函数说白了就是一组code 语句,常用于处理输入、数值计算、生成返回结果; (2)构建函数背后的idea就是为了把我们常用的操作结构化定义起来,减少代码重复,节约代码量
Python provides built-in functions like print(), etc. but we can also create your own functions. These functions are called user-defined functions.
译:Python中包括一系列的built-in functions,同时用户也可以自定义function
关于built-in functions建议查阅我之前的推文:Basic Python —— Built-in Functions
代码示例
# A simple Python function to check
# whether x is even or odd
def evenOdd( x ):
if (x % 2 == 0):
print "even"
else:
print "odd"
# Driver code
evenOdd(2)
evenOdd(3)
even
odd
Pass by Reference or pass by value?
One important thing to note is, in Python every variable name is a reference. When we pass a variable to a function, a new reference to the object is created. Parameter passing in Python is same as reference passing in Java.
译:参数传递,关于自定义function可以查阅我之前的推文:Basic Python —— Built-in Functions
函数中参数传递这个知识点的核心内容包括以下两点:
- Python中的参数传递还是通过赋值符号 = 实现的,例如定义fun(x,y ),并执行调用语句fun( [1,2], {"name":"xiaole","height":165}),调用过程执行了以下赋值操作 x = [1,2] , y = {"name":"xiaole","height":165}
- x 和 y 是指向 [1,2] 和 {"name":"xiaole","height":165} 的label
# Here x is a new reference to same list lst
def myFun(x):
x[0] = 20
# Driver Code (Note that lst is modified
# after function call.
lst = [10, 11, 12, 13, 14, 15]
myFun(lst);
print(lst)
[20, 11, 12, 13, 14, 15]
notes:代码中x 为 [10, 11, 12, 13, 14, 15] 的一个label,指向该lst,因为执行函数内部执行x[0] = 20, 最后lst的元素值发生改变
When we pass a reference and change the received reference to something else, the connection between passed and received parameter is broken. For example, consider below program.
译:如以下代码所示,在function内部,我们重新为参数赋值,效果又怎么样呢?
the connection between passed and received parameter is broken,也就是撕标签贴,然后吧这个标签贴到别的数据内容上
def myFun(x):
# After below line link of x with previous
# object gets broken. A new object is assigned
# to x.
x = [20, 30, 40]
# Driver Code (Note that lst is not modified
# after function call.
lst = [10, 11, 12, 13, 14, 15]
myFun(lst);
print(lst)
[10, 11, 12, 13, 14, 15]
code分析:
Step 1、 在内存中new一个list对象[10, 11, 12, 13, 14, 15] ,并将x这个标签贴在[10, 11, 12, 13, 14, 15]上,
Step 2、调用 myFun, 将输入参数设置为lst,相当于执行x=lst,等价于为[10, 11, 12, 13, 14, 15] 再贴上一个x标签
Step 3、在myFun内部吧x这个标签从[10, 11, 12, 13, 14, 15] 上撕下来,贴到[20,30,40]上面,并结束myFun调用
Step 4、打印lst对应的数据内容,也即[10, 11, 12, 13, 14, 15]
过程理解了,结果你也就理解了,嘿嘿
代码示例、猜一猜下面代码的执行结果
def swap(x, y):
temp = x;
x = y;
y = temp;
# Driver code
x = 2
y = 3
swap(x, y)
print(x)
print(y)
2
3
code分析:猜都不用猜,维持不变
Default arguments
A default argument is a parameter that assumes a default value if a value is not provided in the function call for that argument.The following example illustrates Default arguments.
# Python program to demonstrate
# default arguments
def myFun(x, y=50):
print("x: ", x)
print("y: ", y)
# Driver code (We call myFun() with only
# argument)
myFun(10)
('x: ', 10)
('y: ', 50)
Like C++ default arguments, any number of arguments in a function can have a default value. But once we have a default argument, all the arguments to its right must also have default values.
译:Python中Default arguments(默认参数)的设置就是为了简化调用;同时,在定义参数的过程当中,要求位置参数在左,Default arguments在右
Keyword arguments:
The idea is to allow caller to specify argument name with values so that caller does not need to remember order of parameters.
# Python program to demonstrate Keyword Arguments
def student(firstname, lastname):
print(firstname, lastname)
# Keyword arguments
student(firstname ='Geeks', lastname ='Practice')
student(lastname ='Practice', firstname ='Geeks')
('Geeks', 'Practice')
('Geeks', 'Practice')
译:
- Default arguments是相对于函数定义而言的,Keyword arguments是相对于函数调用而言的
- Keyword arguments的唯一作用就是为了不用满脑子记住参数顺序
Variable length arguments
We can have both normal and keyword variable number of arguments.
# Python program to illustrate
# *args for variable number of arguments
def myFun(*argv):
for arg in argv:
print (arg)
myFun('Hello', 'Welcome', 'to', 'GeeksforGeeks')
Hello
Welcome
to
GeeksforGeeks
# Python program to illustrate
# *kargs for variable number of keyword arguments
def myFun(**kwargs):
for key, value in kwargs.items():
print ("%s == %s" %(key, value))
# Driver code
myFun(first ='Geeks', mid ='for', last='Geeks')
last == Geeks
mid == for
first == Geeks
notes:
在Python中,面对参数不定的情况,我们分别有 *arg 和 **kwargs进行参数接受,具体内容在此不展开,有个映像即可
*arg 用于收集一堆data的,**kwargs收集一度data、同时每个data都拥有一个key(类似于title的意思)。前者方便同类型数据收集,后者方便不同类型的数据收集
你看上面的例子是不是这样子? 嘿嘿嘿
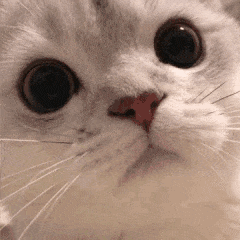
Anonymous functions
In Python, anonymous function means that a function is without a name. As we already know that def keyword is used to define the normal functions and the lambda keyword is used to create anonymous functions.
# Python code to illustrate cube of a number
# using labmda function 大家都在发大家都在发
cube = lambda x: x*x*x
print(cube(7))
343
notes:在Python中,我们使用lambda进行匿名函数定义,后序后进步一作介绍,有个映像即可
References
[1] GeeksforGeeks Functions in Python https://www.geeksforgeeks.org/functions-in-python/?ref=lbp
Motto
日拱一卒,功不唐捐
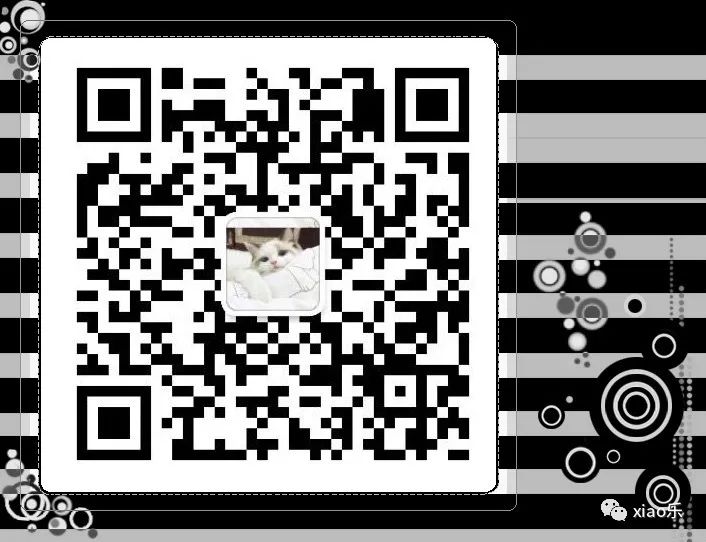