原文:https://www.cnblogs.com/wanmeishenghuo/p/9651363.html
参考:
https://www.jb51.net/article/41331.htm
https://blog.csdn.net/windgs_yf/article/details/81146817
https://www.cnblogs.com/c-slmax/p/5948662.html
https://blog.csdn.net/lc_910927/article/details/29829811
https://blog.csdn.net/zhangxinrun/article/details/5940019
https://blog.csdn.net/linuxheik/article/details/80449059
https://www.cnblogs.com/zhoug2020/archive/2012/04/06/2434245.html
https://blog.csdn.net/qq_26822029/article/details/81213537
在全局重“定义”operator new。
#include <iostream>
#include <cstdlib>
using namespace std;
class Test
{
public:
Test()
{
cout << "Test()" << endl;
}
};
void* operator new(unsigned int size)
{
void* ret = malloc(sizeof(int) * size);
cout << "normal new" << endl;
return ret;
}
int main()
{
Test* t = new Test();
Test t2;
return 0;
}
结果如下:
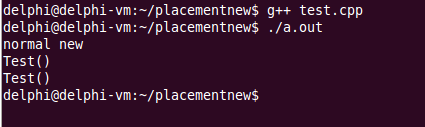
在全局重载placement new
#include <iostream>
#include <cstdlib>
using namespace std;
class Test
{
public:
Test()
{
cout << "Test()" << endl;
}
};
void* operator new(unsigned int size, void* loc)
{
cout << "placement new" << endl;
return loc;
}
int main()
{
char mem[100];
mem[0] = 'A';
mem[1] = '\0';
mem[2] = '\0';
mem[3] = '\0';
Test* t1 = new((void*)mem)Test();
return 0;
}
结果如下:

可以看出,不能在全局重“定义”placement new,参考https://blog.csdn.net/windgs_yf/article/details/81146817
对于自定义对象,我们可以重载普通new操作符,这时候使用new Test()时就会调用到我们重载的普通new操作符。
示例程序:
#include <iostream>
#include <cstdlib>
using namespace std;
class Test
{
public:
Test()
{
cout << "Test()" << endl;
}
void* operator new(unsigned int size)
{
void* ret = malloc(sizeof(int) * size);
cout << "normal new" << endl;
return ret;
}
};
int main()
{
Test* t = new Test();
Test t2;
return 0;
}
执行结果如下:
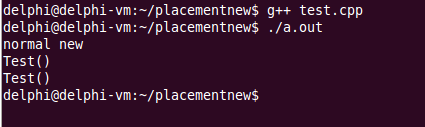
调用placement new,程序如下:
#include <iostream>
#include <cstdlib>
using namespace std;
class Test
{
public:
Test()
{
cout << "Test()" << endl;
}
void* operator new(unsigned int size)
{
void* ret = malloc(sizeof(int) * size);
cout << "normal new" << endl;
return ret;
}
};
int main()
{
Test* t = new Test();
Test* t1 = new((void*)t)Test();
Test t2;
return 0;
}
编译结果如下:

提示我们没有对应的函数,这是因为在类test中定义了operator new, 全局作用域中的new函数的实现就被隐藏了,重载都必须在同一作用域,所以在类test这个作用域中,没有实现placement new。
若此时,删除类中的operator new的定义,也是可以编译通过的,默认使用了全局的operator new和placement new。
在这里,我们在类中加入placement new,更改程序:
#include <iostream>
#include <cstdlib>
using namespace std;
class Test
{
public:
Test()
{
cout << "Test()" << endl;
}
void* operator new(unsigned int size)
{
void* ret = malloc(sizeof(int) * size);
cout << "normal new" << endl;
return ret;
}
void* operator new(unsigned int size, void* loc)
{
cout << "placement new" << endl;
return loc;
}
};
int main()
{
Test* t = new Test();
Test* t1 = new((void*)t)Test();
Test t2;
return 0;
}
结果如下:
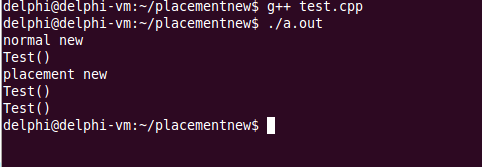
再次给出一个测试程序
#include <iostream>
#include <cstdlib>
using namespace std;
class Test
{
public:
Test()
{
cout << "Test()" << endl;
}
void* operator new(unsigned int size)
{
void* ret = malloc(sizeof(int) * size);
cout << "normal new" << endl;
return ret;
}
void* operator new(unsigned int size, void* loc)
{
cout << "placement new" << endl;
return loc;
}
};
int main()
{
Test* t = new Test();
Test* t1 = new((void*)t)Test();
Test t2;
Test* t3 = new((void*)&t2)Test();
int a = 3;
int* p = new((void*)&a)int(10);
cout << "a = " << a << endl;
return 0;
}
运行结果如下:
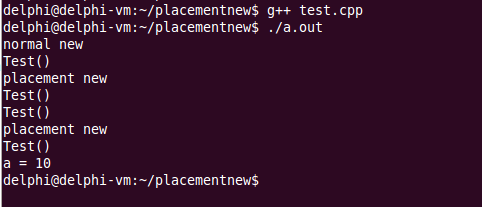
可以看到普通内置类型可以直接使用placement new。
总结:
1、在全局中可以可重复定义operator new;
2、在全局中不能重复定义placement new;
3、一旦在类中定义了operator new或placement new,全局中的所有new函数将被隐藏(函数的重载必须发生在同一作用域)