C语言的字符串库函数定义在头文件string.h中,在使用字符串库函数之前应该先使用#include 包含在源文件中。
字符串复制
字符串的复制,主要是将一个字符串复制到另外一个字符串中,因为字符串常量默认是以’0‘字符结尾,因此复制时包含'0'。
C语言提供了strcpy()函数完成字符串的复制,参数传递两个字符串的地址即可。
#define _CRT_SECURE_NO_WARNINGS#include #include #include // 包含string.h 该头文件包含了常用字符串操作的函数*字符串复制strcpy(char str1[],char str2[])将str2的字符数组拷贝到str1字符数组中,会将0复制到str1中需要注意的是str1的大小不能比str2小*/void strcpy_test() {//字符串都是以0结尾char str1[128] = "";char str2[128] = "world";//将str2的内容复制到str1中,str2遇到0停止复制,会将str2的0复制到str1//将str2复制到str1strcpy(str1, str2);//str1 = worldprintf("str1 = %s",str1);//如果str3内容不为空,str2的0会复制到str3中,0之后的内容都不会被读取char str3[] = "hello again";//将str2复制到str3strcpy(str3,str2);//打印输出str3 = worldprintf("str3 = %s",str3);}/*字符串常用函数strcpy()@author liuguanglei 18601767221@163.com@wechat 18601767221@website ittimeline.net@version 2020/11/26*/int main(int argc, char* argv[]){strcpy_test();system("pause");return 0;}
程序运行效果
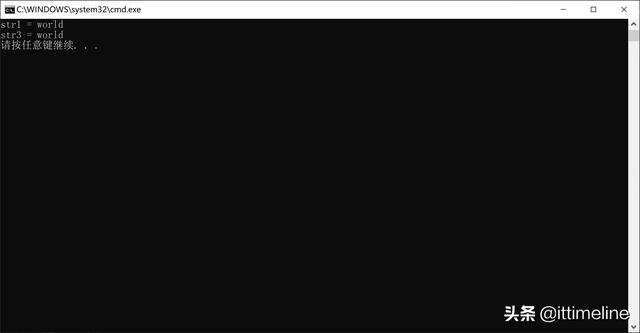
C语言提供了标准库函数strcncpy()用于将复制指定数量的字符串,其参数是需要两个字符串的地址以及指定的数量。
#define _CRT_SECURE_NO_WARNINGS#include #include #include // 包含string.h 该头文件包含了常用字符串操作的函数/*复制n个字符 strncpy(char str1[],char str2[],int size)将str2中指定数量的字符拷贝到str1中,如果不足size,遇到0复制结束*/void strncpy_test() {char str1[128] = "";char str2[128] = "world";//从str2中复制前2个字符到str1中,如果复制时不足2个,遇到0停止复制strncpy(str1, str2,2);//str1 = woprintf("str1 = %s", str1);char str3[128] = "w0orld";//从str3中复制前2个字符到str1中,如果复制时不足2个,遇到0停止复制strncpy(str1, str3, 2);//str1 = wprintf("str1 = %s", str1);}/*字符串常用函数strncpy()@author liuguanglei 18601767221@163.com@wechat 18601767221@website ittimeline.net@version 2020/11/26*/int main(int argc, char* argv[]){strncpy_test();system("pause");return 0;}
程序运行效果
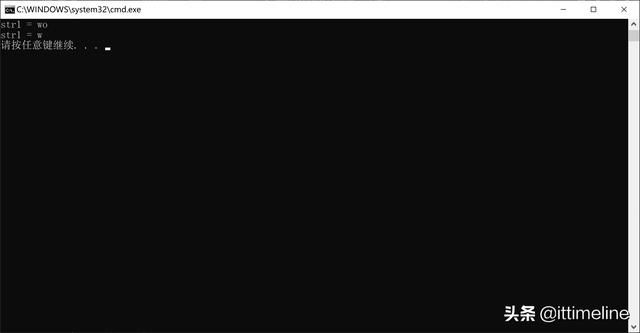
字符串连接
C语言提供了标准库函数strcat()用于两个字符串的连接,其参数传递两个字符串的首地址即可。
#define _CRT_SECURE_NO_WARNINGS#include #include #include // 包含string.h 该头文件包含了常用字符串操作的函数/*字符串连接strcat(char str1[],char str2[])*/void strcat_test() {char str1[128] = "hello";char str2[128] = "world";//将str2的内容连接到str1的后面strcat(str1,str2);// str1 = helloworldprintf("str1 = %s ",str1);char str3[128] = "world0again";strcat(str1,str3);// str1 = helloworldworldprintf("str1 = %s ", str1);}/*字符串常用函数strcat()@author liuguanglei 18601767221@163.com@wechat 18601767221@website ittimeline.net@version 2020/11/26*/int main(int argc, char* argv[]){strcat_test();system("pause");return 0;}
程序运行结果
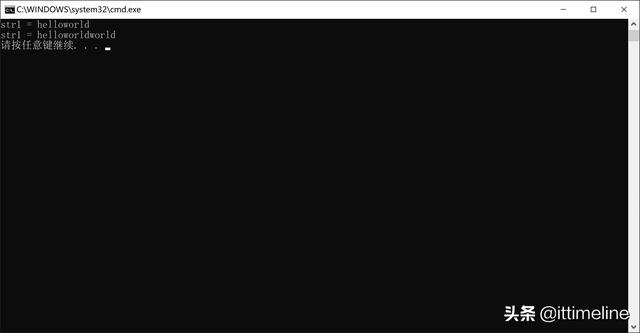
除了strncat()函数外,C语言还提供了标准库函数strncat(),该函数用于连接指定字符个数的字符串
#define _CRT_SECURE_NO_WARNINGS#include #include #include // 包含string.h 该头文件包含了常用字符串操作的函数/*连接n个字符串strncat(char str1[],char str2[],int size)*/void strncat_test() {char str1[128] = "hello";char str2[128] = "world";//将str2的2个字符连接到str1strncat(str1, str2,2);//str1=hellowoprintf("str1 = %s ", str1);}/*字符串常用函数strncat()@author liuguanglei 18601767221@163.com@wechat 18601767221@website ittimeline.net@version 2020/11/26*/int main(int argc, char* argv[]){strncat_test();system("pause");return 0;}
程序运行效果
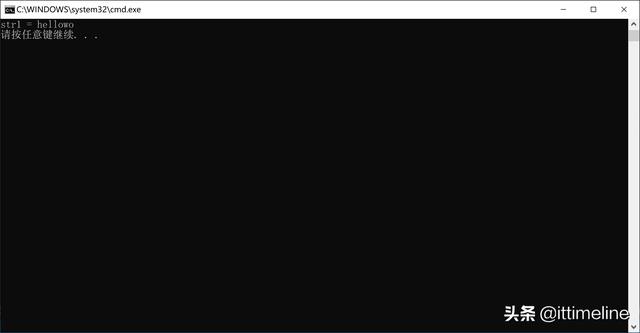
字符串比较
C语言提供了标准库函数strcmp(),用于比较两个字符串的大小,其实现是循环比较的字符的ASCII值,传递的参数也是两个字符串的地址。
如果str1>str2,则返回1
如果str1==str2则返回0
如果str1
#define _CRT_SECURE_NO_WARNINGS#include #include #include // 包含string.h 该头文件包含了常用字符串操作的函数/*字符串的比较strcmp(char str1[],char str2[])//将str1数组和str2数组中拿一个元素进行比较,相等则继续往后比较,比较的是字符的ASCII值,遇到0结束比较//str1>str2 返回1// str1==str2 返回0// str1str2 返回1// str1==str2 返回0// str1
除了strcmp()函数外,C语言还提供了标准库函数strncmp(),该函数可以实现比较指定数量的字符大小
#define _CRT_SECURE_NO_WARNINGS#include #include #include // 包含string.h 该头文件包含了常用字符串操作的函数/*指定大小的字符串的比较strncmp(char str1[],char str2[],int size)*/void strncmp_test() {char str1[128] = "hello";char str2[128] = "world";//str1>str2 返回1// str1==str2 返回0// str1
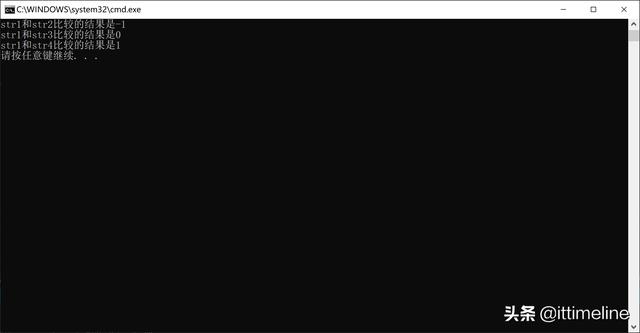
程序运行结果
组包拆包函数
组包函数sprintf()
sprintf(buf,"格式","数据"); 将数据按照指定的格式组包后写入buf,返回值是int,即有效字符的个数,等价于strlen()函数
#define _CRT_SECURE_NO_WARNINGS#include #include /*sprintf()组包函数的使用sprintf(buf,"格式","数据"); 将数据按照指定的格式组包后写入buf,返回值是int,即有效字符的个数,等价于strlen()函数@author liuguanglei 18601767221@163.com@wechat 18601767221@website ittimeline.net@version 2020/11/26*/int main(int argc, char* argv[]){int year = 2020;int month = 11;int day = 26;//格式化打印输出当前年月日printf("将当前年月日格式化输出到终端 year = %d month = %d day = %d ",year,month,day);char buf[128] = "";int length = sprintf(buf, "year=%d month=%d day=%d", year, month, day);//将字符串年月日输出到bufprintf("buf= %s", buf);//获取buf字符的数量printf("length = %d ", length);system("pause");return 0;}
程序运行效果
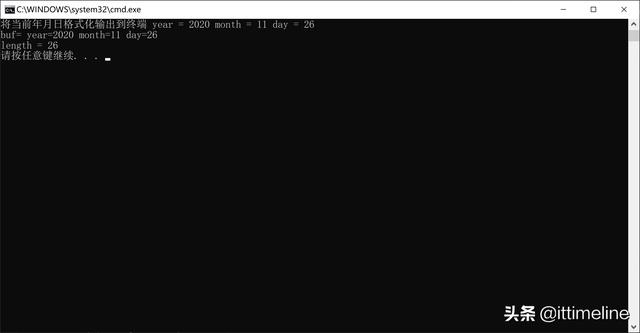
拆包函数sscanf()
sscanf(buf,"格式","数据"); 将buf的内容格式化输出到指定的数据
#define _CRT_SECURE_NO_WARNINGS#include #include /*拆包函数sscanf()的使用 sscanf(buf,"格式","数据"); 将buf的内容格式化输出到指定的数据@author liuguanglei 18601767221@163.com@wechat 18601767221@website ittimeline.net@version 2020/11/26*/int main(int argc, char* argv[]){int year = 0;int month = 0;int day = 0;char buf[128] = "2020-11-26";// 将buf的内容格式化输出到指定的数据int result=sscanf(buf,"%d-%d-%d",&year,&month,&day);if (result!=-1) {//失败返回-1printf("year = %d month = %d day = %d ", year, month, day);}system("pause");return 0;}
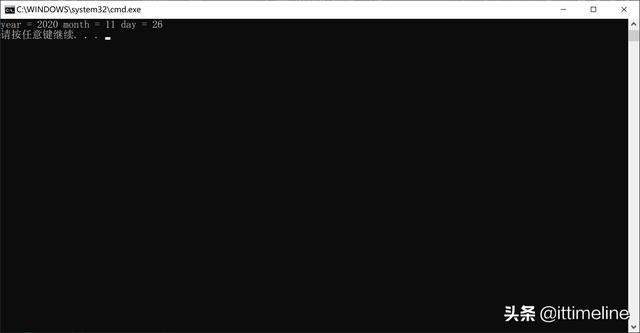
拆包函数
查找字符串
查找字符串的指定字符
C语言提供了标准库函数用于查找指定字符串的字符strchr()函数,该函数需要两个参数,字符串的首地址和查找的字符。
自己实现一个strchr()函数
/*自己实现strchr()函数如果从字符串中找到指定的字符,返回字符的地址,如果没有找到返回NULL*/char* my_str_chr( char* p_char_array, char target_element) {int i = 0;// p_char_array[i]等价于*(p_char_array+i)while (p_char_array[i] != 0) {if (p_char_array[i] == target_element) {//返回出现字符的地址return &p_char_array[i];}i++;}//没有找到指定的字符则返回NULL if(p_char_array[i]==0){ return NULL; }}
自定义实现strchr()函数和库函数strchr()的使用
#define _CRT_SECURE_NO_WARNINGS#include #include #include /*查找字符串中指定字符的位置@author liuguanglei 18601767221@163.com@wechat 18601767221@website ittimeline.net@version 2020/11/26*/int main(int argc, char* argv[]){char str[] = "SeeYourAgain";char target_element = 'o';//使用自己实现的my_str_chr()函数查找字符串中指定的字符char *p_char_my_str_chr = my_str_chr(str, target_element);//使用库函数strchr()函数查找字符串中指定的字符char *p_char_str_chr = strchr(str, target_element);printf("目标字符%c的地址是%d", target_element, p_char_my_str_chr);printf("目标字符%c的地址是%d", target_element, p_char_str_chr);//根据地址取内容printf("%d地址的内容是%c", p_char_str_chr,*p_char_str_chr);system("pause");return 0;}
程序运行结果
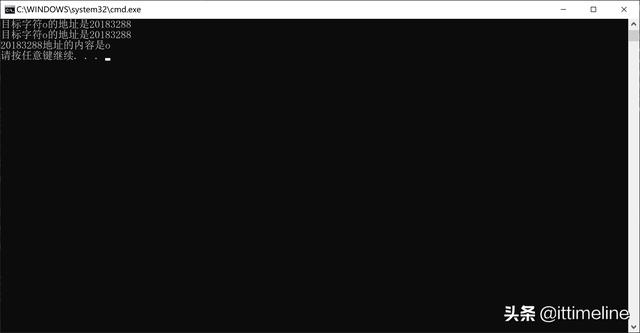
查找字符串的指定子字符串
C语言提供了库函数strstr()用于查找字符串中指定子串的次数,例如查找helloworldhelloworld中helloworld出现的次数
自己实现strstr()库函数
*自己实现strstr()函数查找字符串中子串出现的位置并返回*/char* my_str_str(char * source,char *target) {int i = 0;while (source[i]!=0) {//如果souce字符串中有和target字符串的第一个地址相等if (source[i]= target[0]) {//如果source和target 指定长度相等if (0==strncmp(source+i,target,strlen(target))) {return source+i;}}i++;}if (source[i]==0) {return NULL;}}
自己实现的strstr()函数和库函数strstr()库函数的调用
/*strstr()函数的使用找一个字符串中子串出现的位置@author liuguanglei 18601767221@163.com@wechat 18601767221@website ittimeline.net@version 2020/11/26*/int main(int argc, char* argv[]){//字符串char str1[] = "helloworld";//子串char str2[] = "hello";printf("使用自己实现的strstr()函数实现查找指定子串在字符串中出现的位置");char* p_str1_my_str_str = my_str_str(str1,str2);printf("%s在%s的内存地址是%d",str2,str1, p_str1_my_str_str);printf("内存地址%d的内容是%s", p_str1_my_str_str, p_str1_my_str_str);printf("使用库函数strstr()实现查找指定子串在字符串中出现的位置");char* p_str1_strstr = strstr(str1,str2);printf("%s在%s的内存地址是%d", str2, str1, p_str1_strstr);printf("内存地址%d的内容是%s", p_str1_strstr, p_str1_strstr);system("pause");return 0;}
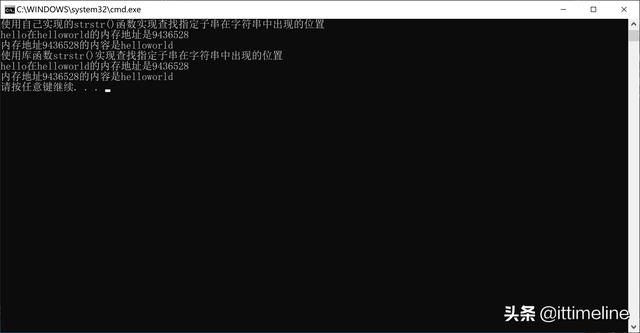
程序运行结果
字符串切割
C语言提供了库函数strtok()用于按照指定的规则切割字符串,该函数需要两个参数,切割字符串的地址以及切割字符串的分隔符
#define _CRT_SECURE_NO_WARNINGS#include #include #include /*strtok()函数实现字符串按照指定的格式切割@author liuguanglei 18601767221@163.com@wechat 18601767221@website ittimeline.net@version 2020/11/26*/int main(int argc, char* argv[]){char str[128] = "18601767221#678901#1860176722@163.com";//在str中将#切割,返回#前面的字符串char *p_mobile_phone=strtok(str,"#");printf("%s", p_mobile_phone);//从NULL之后开始切char *p_code=strtok(NULL,"#");printf("%s", p_code);char* p_email = strtok(NULL, "#");printf("%s", p_email);printf("使用字符指针数组+循环+strtok函数实现切割");//初始化char* 指针数组 为NULLchar *p_info[4] = {NULL};char char_array[] = "18601767221#678901#1860176722@163.com&shanghai";int size =sizeof(p_info) / sizeof(p_info[0]);for (int i = 0; i < size;i++) {if (i==0) {p_info[i]=strtok(char_array,"#&");}else {p_info[i] = strtok(NULL,"#&");}}printf("【for循环切割】for循环遍历切割之后的元素内容");for (int i = 0; i < size; i++) {printf("p_info[%d] = %s ",i,p_info[i]);}//使用do/while循环实现char char_array_data[] = "18601767221#678901#1860176722@163.com&shanghai";char *p_custom_info[4] = {NULL};int i = 0;do {if (i==0) {p_custom_info[i]=strtok(char_array_data,"#");}else {p_custom_info[i] = strtok(NULL, "#&");}i++;} while (char_array_data[i]!=NULL);printf("【do/while循环切割】for循环遍历切割之后的元素内容");for (int i = 0; i < sizeof(p_custom_info) / sizeof(p_custom_info[0]);i++) {printf("p_custom_info[%d] = %s ",i, p_custom_info[i]);}system("pause");return 0;}
程序运行效果
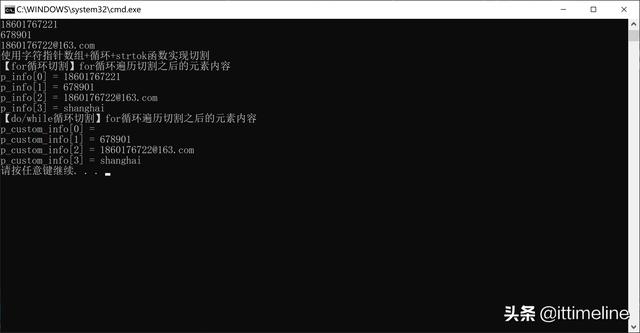
字符串转数值
C语言提供了标准库函数atoi()和atof()函数用于将字符串转换为整数和浮点数
自己实现atoi()函数
/*自己实现将字符串转换为整数*/int my_atoi(char* str) {char* tmp = str;// 0代表正数,1代表负数int flag = 0;while (*tmp==' ' || *tmp == '' || *tmp == '' || *tmp == '') {tmp++;}//先处理正负数if (*tmp == '-') {flag = 1;//从第二个字符开始转换tmp = tmp + 1;}else if (*tmp == '+') {tmp = tmp + 1;}int number = 0;while (*tmp != '0') {number = number * 10 + (*tmp - '0');tmp++;}if (0 == flag) {return number;}else {return -number;}}
atoi()和atof()函数的使用
#define _CRT_SECURE_NO_WARNINGS#include #include /*atoi()函数:将字符转换为整数atof()函数:将字符转换为小数@author liuguanglei 18601767221@163.com@wechat 18601767221@website ittimeline.net@version 2020/11/26*/int main(int argc, char* argv[]){//0-9之间的数字以及正号(+)负号(-)开始转换,否则就结束转换// 如果前面有空格就跳过char int_str[128] = "1234";int atoi_result = atoi(int_str);printf("atoi_result = %d ", atoi_result);int my_atoi_result = my_atoi(int_str);printf("my_atoi_result = %d ", my_atoi_result);char float_str[128] = "3.14";float flt_result = atof(float_str);printf("flt_result = %.2f",flt_result);system("pause");return 0;}
程序运行结果
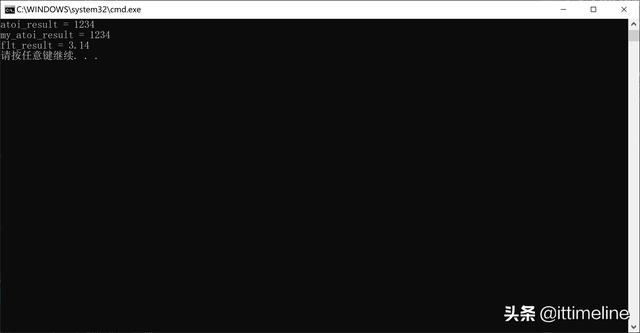
字符串应用
获取非空字符
#define _CRT_SECURE_NO_WARNINGS#include #include #include /*获取非空字符的个数@author liuguanglei 18601767221@163.com@wechat 18601767221@website ittimeline.net@version*/int main(int argc, char* argv[]){//定义一个包含空字符的字符串char buf[] = " hello world ";char not_null_buf[128] = "";if (buf[0]==0) {return;}//指向第一个字符的地址char* start = buf;//指向最后一个字符的地址char* end = &buf[strlen(buf) - 1];//为空格,并且不为0while (*start==' '&& *start!=0 ) {start++;}while (*end == ' '&&end!=start) {end--;}int char_size = end - start + 1;printf("%s非空字符的个数为%d ", buf,char_size);strncpy(not_null_buf, start,char_size);printf("not_null_buf =%s ", not_null_buf);system("pause");return 0;}
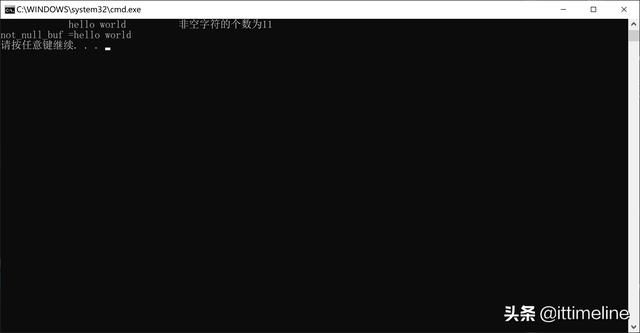
程序运行结果
字符串反转
#define _CRT_SECURE_NO_WARNINGS#include #include #include /*获取非空字符的个数@author liuguanglei 18601767221@163.com@wechat 18601767221@website ittimeline.net@version*/int main(int argc, char* argv[]){//定义一个包含空字符的字符串char buf[] = " hello world ";char not_null_buf[128] = "";if (buf[0]==0) {return;}//指向第一个字符的地址char* start = buf;//指向最后一个字符的地址char* end = &buf[strlen(buf) - 1];//为空格,并且不为0while (*start==' '&& *start!=0 ) {start++;}while (*end == ' '&&end!=start) {end--;}int char_size = end - start + 1;printf("%s非空字符的个数为%d ", buf,char_size);strncpy(not_null_buf, start,char_size);printf("not_null_buf =%s ", not_null_buf);system("pause");return 0;}
程序运行结果
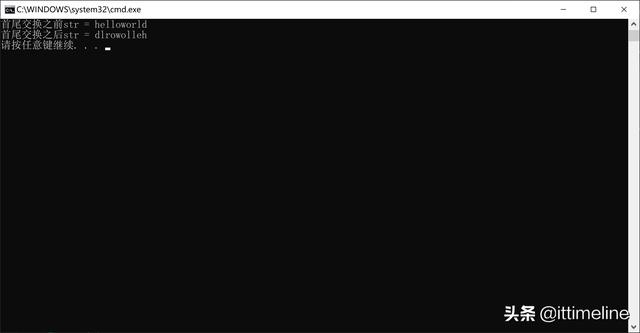
字符串排序
#define _CRT_SECURE_NO_WARNINGS#include #include #include /*通过for循环遍历指定大小的字符数组指针指向的元素内容*/void print_element_list(char* cities[],int size,int flag) {if (flag==0) {printf("【未进行冒泡排序之前】通过for循环遍历获取指字符指针数组指向的内容");}else if (flag==1) {printf("【进行冒泡排序之后】通过for循环遍历获取指字符指针数组指向的内容");}for (int i = 0; i < size; i++) {printf("cities[%d] = %s ", i, cities[i]);}}/*字符指针数组的冒泡排序实现*/void bubble_sort(char* cities[],int size) {for (int i = 0; i < size-1;i++) {for (int j = 0; j < size-i - 1;j++) {char* tmp = NULL;int result = strcmp(cities[j], cities[j+1] );//str1>str2 返回1// str1==str2 返回0// str1
程序运行效果
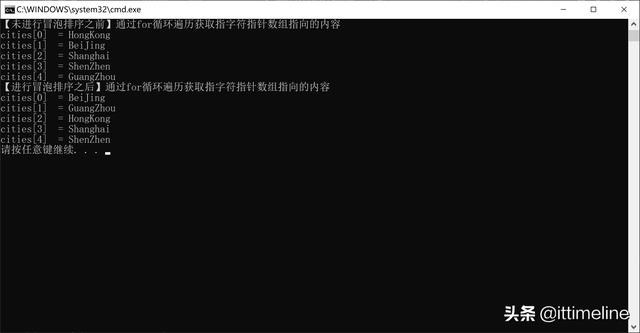
删除指定字符
#define _CRT_SECURE_NO_WARNINGS#include #include /*字符串删除@author liuguanglei 18601767221@163.com@wechat 18601767221@website ittimeline.net@version 2020/11/26*/int str_delete_main(int argc, char* argv[]){char str[] = "I will see ,you ,,again";printf("删除之前:str = %s",str);char* p = str;//删除str中的逗号字符int i = 0;while (*p!=0) {if (*p==',') {char* q = p;while (*q!=0) {*q = *(q + 1);q++;}}else {p++;}}printf("删除之后:str = %s", str);system("pause");return 0;}
程序运行效果
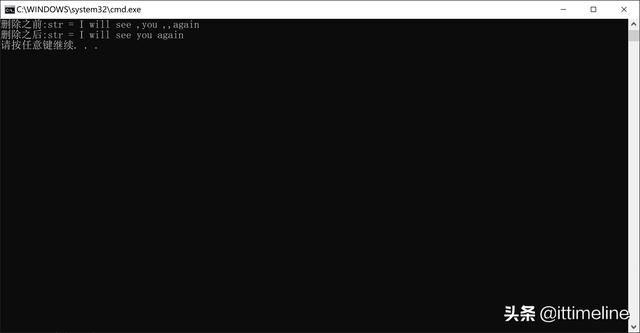