(一)Vue 的基础介绍
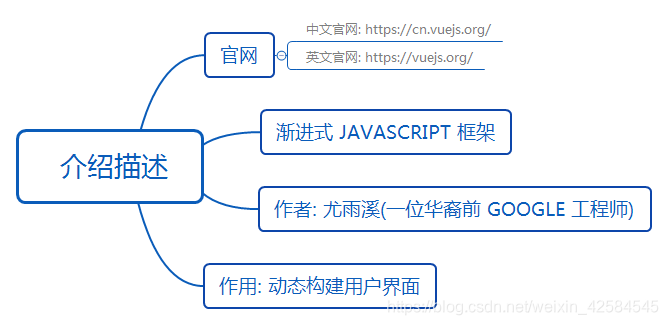
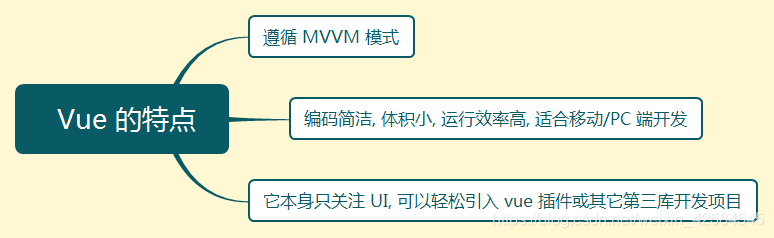
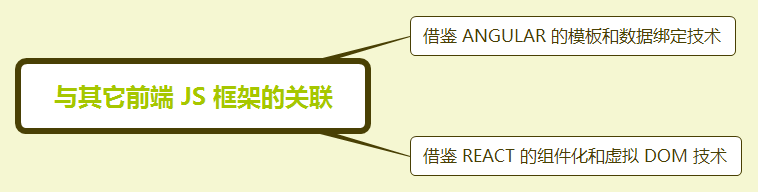
(二)Vue 的基本使用
1.vue入门案例
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<!-- <script src="https://cdn.jsdelivr.net/npm/vue@2.6.10/dist/vue.js"></script> -->
<script type="text/javascript">
window.onload=function(){
//创建vue对象
new Vue({
el:'#box',
data:{
msg:'Hello Vue'
}
});
}
</script>
</head>
<body>
<div id="box">
<h1>{{msg}}</h1>
</div>
</body>
</html>
2.属性绑定
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
window.onload=function(){
new Vue({
el:'#box',
data:{
msg:100,
mydata:true,
imgurl:'https://cn.vuejs.org/images/dcloud.gif',
wz:'http://www.sina.com'
}
});
}
</script>
</head>
<body>
<!-- 指令: v-bind 属性绑定 -->
<div id="box">
<img src="https://cn.vuejs.org/images/dcloud.gif"/>
<img v-bind:src="imgurl"/>
<a href="http://www.baidu.com">进入百度</a>
<a v-bind:href="wz">进入新浪</a>
<!-- 简写 -->
<a :href="wz">进入新浪</a>
</div>
</body>
</html>
3.vue的事件绑定
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
window.onload=function(){
new Vue({
el:'#box',
data:{
msg:'Hello Vue'
},
//事件的处理函数
methods:{
show:function(){
alert(this.msg);
},
show2:function(){
alert("haha");
},
//ES6语法
show3(){
alert("show3333");
}
}
})
}
</script>
</head>
<body>
<div id="box">
<!-- 采用 指令 v-on:事件名 -->
<button v-on:click="show()">一个按钮</button>
<button v-on:click="show2()">一个按钮2</button>
<button v-on:click="show3()">一个按钮3</button>
</div>
</body>
</html>
4.事件对象
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
#nei{
width: 200px;
height: 200px;
background: red;
}
</style>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
window.onload=function(){
new Vue({
el:'#box',
data:{
msg:'Hello Vue'
},
//事件的处理函数
methods:{
show:function(num){
alert(this.msg+"---"+num);
},
show2(event){
//阻止默认行为
//event.preventDefault()
//或
//阻止冒泡
//event.stopPropagation()
},
show4(event){
//阻止默认行为
//event.preventDefault()
//或
//阻止冒泡
//event.stopPropagation()
alert(this.msg);
},
show5(){
alert(this.msg);
},
test(){
alert("abc");
},
test2(){
alert("wai")
},
test3(){
alert("btn")
}
}
})
}
</script>
</head>
<body>
<div id="box">
<!-- 采用 指令 v-on:事件名 -->
<button v-on:click="show()">一个按钮</button>
<!-- 绑定事件的简写方式 采用 @事件名-->
<button @click="show(100)">一个按钮</button>
<!-- 如何传递事件对象 $event -->
<button @click="show2($event)">一个按钮2</button>
<!-- vue阻止默认行为 @click.prevent -->
<a href="http://www.baidu.com" @click.prevent="test()">进入百度</a>
<!--vue 阻止冒泡行为 @click.stop -->
<div id="nei" @click.stop="test2()">
<button type="button" @click.stop="test3()">按钮</button>
</div>
<!-- 事件只执行一次 @click.once -->
<button @click.once="show4(100)">一个按钮</button>
<!--
为了在必要的情况下支持旧浏览器,Vue 提供了绝大多数常用的按键码的别名:
.enter
.tab
.delete (捕获“删除”和“退格”键)
.esc
.space
.up
.down
.left
.right
.ctrl
.alt
.shift
.meta
-->
<!-- 键盘事件 -->
<input type="text" id="" value="" @keypress.enter="show5()"/>
</div>
</body>
</html>
5.模板语法
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
#nei{
width: 200px;
height: 200px;
background: red;
}
</style>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
window.onload=function(){
new Vue({
el:'#box',
data:{
msg:'Hello Vue',
num:100,
mymsg:'<b>加粗文字</b>'
}
});
}
</script>
</head>
<body>
<div id="box">
<!-- 模板语法 可以在页面取data 对象中数据 -->
<h1>{{msg}}<h1>
<!-- 模板语法 会支持一些 JS 简单的运算符和函数 -->
<h1>{{msg.length}}<h1>
<h1>{{msg.toUpperCase()}}<h1>
<h1>{{num*2}}<h1>
<h1>{{num==100?'abc':'ccc'}}<h1>
<!--v-text 取数据 -->
<h1 v-text="msg"></h1>
{{mymsg}}
<span v-text="mymsg"></span>
<!-- v-html 可以转意 html 标签 -->
<span v-html="mymsg"></span>
</div>
</body>
</html>
6.数据绑定
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
#nei {
width: 200px;
height: 200px;
background: red;
}
</style>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
window.onload = function() {
new Vue({
el: '#box',
data: {
msg: 'Hello Vue',
}
});
}
</script>
</head>
<body>
<div id="box">
<!-- v-model 表单的数据绑定-->
<input type="text" id="" value="" v-model="msg" />
<h1>{{msg}}</h1>
<input type="text" id="" value="" v-model="msg" />
</div>
</body>
</html>
7.隐藏和显示
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
#nei {
width: 200px;
height: 200px;
background: red;
}
</style>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
window.onload = function() {
new Vue({
el: '#box',
data: {
msg: 'Hello Vue',
flag:true
},
methods:{
isShow(){
//this 代表vue对象
this.flag=!this.flag
}
}
});
}
</script>
</head>
<body>
<div id="box">
<!-- v-show="true" true 元素显示 false 元素隐藏-->
<div id="nei" v-show="flag">
</div>
<button type="button" @click="isShow()">显示/隐藏</button>
<button type="button" @click="flag=!flag">显示/隐藏2</button>
</div>
</body>
</html>
8.计算属性
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
#nei {
width: 200px;
height: 200px;
background: red;
}
</style>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
window.onload = function() {
new Vue({
el: '#box',
data: {
msg: 'Hello Vue',
xing:'王',
name:'老虎',
},
computed:{ //计算属性
//fullname 什么时候调用 第一次初始化的时候调用,
// fullname 函数中 相关的属性,发送变化时就会调用
fullname:function(){
console.log("fullname 调用了")
return this.xing+" "+this.name;
}
}
});
}
</script>
</head>
<body>
<div id="box">
姓:<input type="text" id="" value="" placeholder="请输入你的姓" v-model="xing"/><br>
名:<input type="text" id="" value="" placeholder="请输入你的名" v-model="name"/><br>
全名:<input type="text" id="" value="" placeholder="你的全名"/ v-model="fullname"><br>
<!-- 全名:<input type="text" id="" value="" placeholder="你的全名"/ v-model="xing.concat(name)"><br> -->
</div>
</body>
</html>
9.计算属性2
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
#nei {
width: 200px;
height: 200px;
background: red;
}
</style>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
window.onload = function() {
new Vue({
el: '#box',
data: {
msg: 'Hello Vue',
xing:'王',
name:'老虎',
},
computed:{ //计算属性
fullname:{
get:function () {
console.log("get");
return this.xing+" "+this.name;
},
//监听fullname 属性的变化
set:function(value){
//value 就是fullname 属性的值
console.log("set fullname 变化了"+value);
var text=value.split(' ');
this.xing=text[0];
this.name=text[1];
}
}
}
});
}
</script>
</head>
<body>
<div id="box">
姓:<input type="text" id="" value="" placeholder="请输入你的姓" v-model="xing"/><br>
名:<input type="text" id="" value="" placeholder="请输入你的名" v-model="name"/><br>
全名:<input type="text" id="" value="" placeholder="你的全名"/ v-model="fullname"><br>
<!-- 全名:<input type="text" id="" value="" placeholder="你的全名"/ v-model="xing.concat(name)"><br> -->
</div>
</body>
</html>
10.属性值的变化的监听
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
#nei {
width: 200px;
height: 200px;
background: red;
}
</style>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
window.onload = function() {
new Vue({
el: '#box',
data: {
msg: 'Hello Vue',
xing:'王',
name:'老虎',
fullname:'王 老虎'
},
//watch 监听属性值的变化
watch:{
xing:function(newValue,oldValue){
//alert("调用了 新值"+newValue+" 旧值"+oldValue);
},
name:function(newValue,oldValue){
//alert("调用了 新值"+newValue+" 旧值"+oldValue);
},
fullname:function(newValue){
var text=newValue.split(' ');
this.xing=text[0];
this.name=text[1];
}
}
});
}
</script>
</head>
<body>
<div id="box">
姓:<input type="text" id="" value="" placeholder="请输入你的姓" v-model="xing"/><br>
名:<input type="text" id="" value="" placeholder="请输入你的名" v-model="name"/><br>
全名:<input type="text" id="" value="" placeholder="你的全名"/ v-model="fullname"><br>
<!-- 全名:<input type="text" id="" value="" placeholder="你的全名"/ v-model="xing.concat(name)"><br> -->
</div>
</body>
</html>
(三)属性绑定
1.class属性绑定
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<style type="text/css">
.aClass {
color: red;
font-size: 100px;
}
.bClass {
font-size: 200px;
color: blue;
}
</style>
<script type="text/javascript">
window.onload = function() {
new Vue({
el: '#box',
data: {
msg: 'abc',
url: 'http://www.baidu.com',
myClass: 'bClass',
myClass2: 'aClass',
isA: true,
isB: false,
myjson: {
'aClass': true,
'bClass': false,
}
},
methods: {
change() {
this.isA = !this.isA;
this.isB = !this.isB;
}
}
});
}
</script>
</head>
<body>
<div id="box">
<a v-bind:href="url">百度</a>
<!-- -->
<h1 class="aClass">11111111111111111</h1>
<!-- vue 绑定这个class 属性 -->
<!-- 方式1:绑定一个class选择器的名称,注意 选择器的名称用单引号引起来 -->
<h1 :class="'bClass'">222222222222</h1>
<!-- -->
<!-- 绑定多个选择器的名称 -->
<h1 :class="['bClass','aClass']">222222222222</h1>
<!-- 绑定的值是一个变量 -->
<h1 :class="myClass">3333333333333333333</h1>
<!-- 多个数据,可以使用[] 括起来 -->
<h1 :class="[myClass,myClass2]">444444</h1>
<!-- 选择器要不要生效 true 生效,false 不生效 -->
<h1 :class="{'aClass':true,'bClass':false}">5555555555555555555</h1>
<h1 :class="{'aClass':isA,'bClass':isB}">5555555555555555555</h1>
<button type="button" @click="change()">切换</button>
<h1 :class="myjson">5555555555555555555</h1>
</div>
</body>
</html>
2.style属性绑定.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<style type="text/css">
div:{
font-style: italic;
}
</style>
<script type="text/javascript">
window.onload = function() {
new Vue({
el: '#box',
data: {
a: 'yellow',
b: '100px',
flag: true,
myjson: {
color:'red',
fontSize:'200px'
},
myjson2: {
fontStyle:'italic'
}
},
methods: {
change(f) {
if (f) {
this.a = 'blue'
this.b = '50px'
} else {
this.a = 'pink'
this.b = '150px'
}
}
}
});
}
</script>
</head>
<body>
<div id="box">
<h1 style="color:red;font-size: 100px;">11111111111111111111</h1>
<!-- vue 绑定style属性 值 要一个json对象
值要使用 单引号 引起来
css样式名 如果有 "-" 连接 把"-"去掉,"-"后面的第一个字母变大写
-->
<h1 :style="{color:'red',fontSize:'100px'}">2222222</h1>
<!-- 值 作为变量 -->
<h1 :style="{color:a,fontSize:b}">2222222</h1>
<button type="button" @click="change(flag=!flag)">按钮</button>
<h1 :style="myjson">2222222</h1>
<h1 :style="[myjson,myjson2]">2222222</h1>
</div>
</body>
</html>
3.if条件指令
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<style type="text/css">
div:{
font-style: italic;
}
</style>
<script type="text/javascript">
window.onload = function() {
new Vue({
el: '#box',
data: {
flag:true
},
methods: {
change(){
this.flag=!this.flag;
}
}
});
}
</script>
</head>
<body>
<div id="box">
<h1 v-if="flag">正确</h1>
<h1 v-else>错误</h1>
<button type="button" @click="change()">切换</button>
</div>
</body>
</html>
4.多重条件选择.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
window.onload = function() {
new Vue({
el: '#box',
data: {
score: 0
},
methods: {
change() {
this.flag = !this.flag;
}
}
});
}
</script>
</head>
<body>
<div id="box">
<input type="text" name="" id="" value="" placeholder="请输入你的成绩0---100" v-model="score" />
<h1 v-if="score>=0&&score<60">
不及格
</h1>
<h1 v-else-if="score>=60&&score<80">
及格
</h1>
<h1 v-else-if="score>=80&&score<90">
优秀
</h1>
<h1 v-else-if="score>=90&&score<100">
非常优秀
</h1>
<h1 v-else-if="score==100">
满分
</h1>
<h1 v-else>
成绩乱输
</h1>
</div>
</body>
</html>
5.for循环指令
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<style type="text/css">
div: {
font-style: italic;
}
</style>
<script type="text/javascript">
window.onload = function() {
new Vue({
el: '#box',
data: {
myArr: [10, 20, 30, 40, 50, 60, 70, 80, 80],
myJson: {
username: 'zhangsan',
age: 100,
phone: '13962052305'
},
jsonArry: [{
username: 'lisi',
age: 200,
phone: '13962052305'
}, {
username: 'wangwu',
age: 300,
phone: '13962052305'
}, {
username: '赵六',
age: 400,
phone: '13962052305'
}]
}
});
}
</script>
</head>
<body>
<div id="box">
<!-- {{myArr[0]}} -->
<ul>
<!-- 为了给 Vue 一个提示,以便它能跟踪每个节点的身份,从而重用和重新排序现有元素,你需要为每项提供一个唯一 key 属性: -->
<li v-for="(ele,index) in myArr" :key="index">
{{ele}}----{{index}}
</li>
</ul>
<!-- 遍历JSON对象 -->
<ul>
<li v-for="(value,key,index) in myJson" :key="key">
{{value}}-----{{key}}----{{index}}
</li>
</ul>
<!-- 遍历 JSON数组 -->
<ul>
<li v-for="(obj,index) in jsonArry" :key="index">
{{obj.username}}----{{obj.age}}----{{obj.phone}}----{{index}}
</li>
</ul>
</div>
</body>
</html>
6.for循环案例
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<style type="text/css">
div: {
font-style: italic;
}
</style>
<script type="text/javascript">
window.onload = function() {
new Vue({
el: '#box',
data: {
jsonArr: [{
'name': '张三',
'age': 24,
'sex': '男'
}, {
'name': '李四',
'age': 25,
'sex': '男'
}, {
'name': '王五',
'age': 26,
'sex': '男'
}]
},
methods: {
deleteObj(index) {
//alert('删除')
//根据索引删除
//splice() 删除元素,并向数组添加新元素。
this.jsonArr.splice(index, 1);
},
//更新
updateObj:function(index,obj){
// alert(index);
// alert(obj);
// this.jsonArr[index]=obj;
// this.jsonArr.reverse().reverse();
this.jsonArr.splice(index,1,obj);
}
}
});
}
</script>
</head>
<body>
<div id="box">
<ul>
<li v-for="(obj,index) in jsonArr" :key="index">
{{index}}---{{obj.name}}----{{obj.age}}---{{obj.sex}}--<button @click="deleteObj(index)">删除</button>---<button
type="button" @click="updateObj(index,{
'name': '赵六',
'age': 26,
'sex': '男'
})">更新</button>
</li>
</ul>
</div>
</body>
</html>
7.for循环案例2
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<style type="text/css">
</style>
<script type="text/javascript">
window.onload = function() {
new Vue({
el: '#box',
data: {
name: '',
age: '',
jsonArray: []
},
methods: {
addObj() {
//alert("添加");
//把输入框中的数据拿出来 ,封装成JSON对象,把这个对象再添加到数组中
var name = this.name.trim();
var age = this.age.trim();
//对表单做非空校验 //自动类型转换
if (!name || !age) {
alert("用户名或年龄不能为空")
return;
}
var json = {
'name': name,
'age': age
};
//添加到数组里面去
// unshift()
// push()
this.jsonArray.push(json);
//加完数据后,清空输入框中的就内容
this.name = '';
this.age = '';
},
//全部删除
delAll() {
if (window.confirm("要删除全部数据吗?")) {
this.jsonArray = [];
}
}
}
});
}
</script>
<!-- <script type="text/javascript">
var name=window.prompt("请输入新的名字");
document.write(name);
</script> -->
</head>
<body>
<div id="box">
<center>
<h1>添加数据</h1>
<input type="text" id="" value="" placeholder="请输入姓名" v-model="name" />
<input type="text" id="" value="" placeholder="请输入年龄" v-model="age" />
<button type="button" @click="addObj()">添加</button>
<br>
<br>
<br>
<br>
<br>
<table border="1" cellspacing="0" cellpadding="" width="500px">
<caption>
<h3>用户信息</h3>
</caption>
<tr>
<th>序号</th>
<th>姓名</th>
<th>年龄</th>
<th>操作</th>
</tr>
<tr v-for="(obj,index) in jsonArray" align="center">
<td>
{{index}}
</td>
<td>
{{obj.name}} <a href="#" @click..prevent="updateName()">修改</a>
</td>
<td>
{{obj.age}} <a href="#" @click..prevent="updateAge()">修改</a>
</td>
<td>
<button type="button" @mousedown.left="delObj()">删除</button>
</td>
</tr>
<tr>
<td colspan="4" align="center"><button type="button" @click="delAll()">全部删除</button></td>
</tr>
</table>
</center>
</div>
</body>
</html>
8.过滤搜索排序案例
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<style type="text/css">
</style>
<script type="text/javascript">
window.onload = function() {
new Vue({
el: '#box',
data: {
content: '',
jsonArr: [{
'name': 'zhangsan',
'age': 24,
'sex': '男'
}, {
'name': 'lisi',
'age': 23,
'sex': '男'
}, {
'name': 'wangwu',
'age': 22,
'sex': '男'
}, {
'name': 'zhaoliu',
'age': 28,
'sex': '男'
}, {
'name': 'tianqi',
'age': 27,
'sex': '男'
}]
},
//计算属性
computed: {
newArray: function() {
//过滤原来的数组
//filter() 检测数值元素,并返回符合条件所有元素的数组。
//var name=this.content; //注意this
//alert(this);
/* var arr=this.jsonArr.filter(function(ele){
if(ele.name.indexOf(name)!=-1){
return true;
}else{
return false;
}
}); */
//采用箭头函数 this 就代表了Vue
var arr = this.jsonArr.filter((ele) => ele.name.indexOf(this.content) != -1);
return arr;
}
},
methods: {
sortObj(num) {
if (num == 1) { //升序
this.jsonArr.sort(function(a, b) {
return a.age - b.age;
});
} else if (num == 2) { //降序
this.jsonArr.sort(function(a, b) {
return b.age - a.age;
});
} else if (num == 3) { //默认顺序
}
}
}
});
}
</script>
<!-- <script type="text/javascript">
var name=window.prompt("请输入新的名字");
document.write(name);
</script> -->
</head>
<body>
<div id="box">
<center>
<input type="" name="" id="" value="" placeholder="请输入搜索内容" v-model="content" />
<ul style="list-style: none;">
<li v-for="(obj,index) in newArray" :key="index">
{{index}}---{{obj.name}}---{{obj.age}}---{{obj.sex}}
</li>
</ul>
<button type="button" @click="sortObj(1)">年龄升序</button>
<button type="button" @click="sortObj(2)">年龄降序</button>
<button type="button" @click="sortObj(3)">默认顺序</button>
</center>
</div>
</body>
</html>
calss属性的绑定
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script src="js/vue.js" type="text/javascript" charset="utf-8"></script>
<style type="text/css">
.aClass{
color: red;
font-size:100px;
}
.bClass{
font-size:200px;
color:blue;
}
</style>
<script type="text/javascript">
window.onload=function(){
new Vue({
el:'#box',
data:{
msg:'abc',
url:'http://www.baidu.com',
myClass:'bClass',
myClass2:'aClass',
isA:true,
isB:false,
myjson:{
'aClass':true,
'bClass':false,
}
},
methods:{
change(){
this.isA=!this.isA;
this.isB=!this.isB;
}
}
});
}
</script>
</head>
<body>
<div id="box">
<a v-bind:href="url" >百度</a>
<!-- -->
<h1 class="aClass">11111111111111111</h1>
<!-- vue 绑定这个class 属性 -->
<!-- 方式1:一个class选择器的名称 -->
<h1 :class="'bClass'">222222222222</h1>
<!-- -->
<h1 :class="['bClass','aClass']">222222222222</h1>
<!-- 是一个变量 -->
<h1 :class="myClass">3333333333333333333</h1>
<!-- 多个数据,可以使用[] 括起来 -->
<h1 :class="[myClass,myClass2]">444444</h1>
<!-- 选择器要不要生效 true 生效,false 不生效 -->
<h1 :class="{'aClass':true,'bClass':false}">5555555555555555555</h1>
<h1 :class="{'aClass':isA,'bClass':isB}">5555555555555555555</h1>
<button type="button" @click="change()">切换</button>
<h1 :class="myjson">5555555555555555555</h1>
</div>
</body>
</html>