public static void main(String[] args) throws IOException {
//url
String url = "";
//解压的目的文件夹
String path = "";
//下载文件
File zip = downloadFile(url,path);
//解压文件
unZipFiles(zip,path);
}
public static void unZipFiles(File zipFile, String descDir) throws IOException {
File pathFile = new File(descDir);
if (!pathFile.exists()) {
pathFile.mkdirs();
}
//解决zip文件中有中文目录或者中文文件
ZipFile zip = new ZipFile(zipFile, Charset.forName("GBK"));
for (Enumeration entries = zip.entries(); entries.hasMoreElements(); ) {
ZipEntry entry = (ZipEntry) entries.nextElement();
String zipEntryName = entry.getName();
InputStream in = zip.getInputStream(entry);
String outPath = (descDir + zipEntryName).replaceAll("\\*", "/");
;
//判断路径是否存在,不存在则创建文件路径
File file = new File(outPath.substring(0, outPath.lastIndexOf('/')));
if (!file.exists()) {
file.mkdirs();
}
//判断文件全路径是否为文件夹,如果是上面已经上传,不需要解压
if (new File(outPath).isDirectory()) {
continue;
}
//输出文件路径信息
System.out.println(outPath);
OutputStream out = new FileOutputStream(outPath);
byte[] buf1 = new byte[1024];
int len;
while ((len = in.read(buf1)) > 0) {
out.write(buf1, 0, len);
}
in.close();
out.close();
}
System.out.println("******************解压完毕********************");
}
public static File downloadFile(String urlPath,String downloadDir) throws IOException {
File file = null;
try {
// 统一资源
URL url = new URL(urlPath);
// 连接类的父类,抽象类
URLConnection urlConnection = url.openConnection();
// http的连接类
HttpURLConnection httpURLConnection = (HttpURLConnection) urlConnection;
//设置超时
httpURLConnection.setConnectTimeout(1000*5);
//设置请求方式,默认是GET
httpURLConnection.setRequestMethod("GET");
// 设置字符编码
httpURLConnection.setRequestProperty("Charset", "UTF-8");
// 打开到此 URL引用的资源的通信链接(如果尚未建立这样的连接)。
httpURLConnection.connect();
// 文件大小
int fileLength = httpURLConnection.getContentLength();
String fileFullName = ".zip";
String newUrl = httpURLConnection.getURL().getFile();
if (newUrl != null || newUrl.length() >= 0) {
newUrl = java.net.URLDecoder.decode(newUrl, "UTF-8");
int pos = newUrl.indexOf('?');
if (pos > 0) {
newUrl = newUrl.substring(0, pos);
}
pos = newUrl.lastIndexOf('/');
fileFullName = newUrl.substring(pos + 1);
}
// 控制台打印文件大小
System.out.println("您要下载的文件大小为:" + fileLength );
BufferedInputStream bin = new BufferedInputStream(httpURLConnection.getInputStream());
// 指定存放位置(有需求可以自定义)
String path = downloadDir + File.separatorChar + fileFullName;
file = new File(path);
// 校验文件夹目录是否存在,不存在就创建一个目录
if (!file.getParentFile().exists()) {
file.getParentFile().mkdirs();
}
OutputStream out = new FileOutputStream(file);
int size = 0;
int len = 0;
byte[] buf = new byte[2048];
while ((size = bin.read(buf)) != -1) {
len += size;
out.write(buf, 0, size);
// 控制台打印文件下载的百分比情况
System.out.println("下载了-------> " + len * 100 / fileLength + "%\n");
}
// 关闭资源
bin.close();
out.close();
System.out.println("文件下载成功!");
} catch (MalformedURLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
System.out.println("文件下载失败!");
} finally {
return file;
}
}
public static byte[] readInputStream(InputStream inputStream) throws IOException {
byte[] buffer = new byte[1024];
int len = 0;
ByteArrayOutputStream bos = new ByteArrayOutputStream();
while((len = inputStream.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
bos.close();
return bos.toByteArray();
}
通过URL下载文件并解压输出
最新推荐文章于 2024-01-11 17:25:08 发布
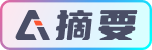