MyBatis-plus初步学习
如何进行基础配置?
看这里
分页查询基础配置
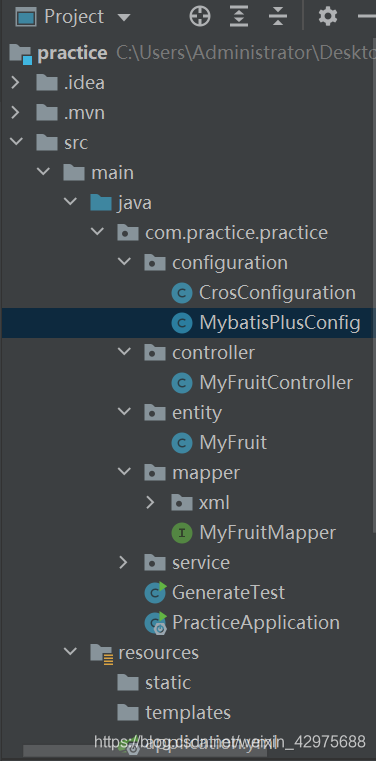
import com.baomidou.mybatisplus.extension.plugins.PaginationInterceptor;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.transaction.annotation.EnableTransactionManagement;
@EnableTransactionManagement
@Configuration
@MapperScan("com.practice.practice.mapper")
public class MybatisPlusConfig {
@Bean
public PaginationInterceptor paginationInterceptor(){
PaginationInterceptor interceptor=new PaginationInterceptor();
return interceptor;
}
}
对于某个表的controller该如何写:
package com.practice.practice.controller;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.practice.practice.entity.MyFruit;
import com.practice.practice.service.MyFruitService;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.ResponseBody;
import java.nio.charset.StandardCharsets;
import java.util.List;
@Controller
@ResponseBody
@RequestMapping("/myFruit")
public class MyFruitController {
@Autowired
private MyFruitService myFruitService;
@GetMapping("/list")
public List<MyFruit> list(){
List<MyFruit> myFruits=this.myFruitService.list();
return myFruits;
}
@GetMapping("/getEq")
public List<MyFruit> getEq(){
QueryWrapper<MyFruit> queryWrapper=new QueryWrapper<>();
queryWrapper.eq("age",20);
return this.myFruitService.list(queryWrapper);
}
@GetMapping("/getOne/{id}")
public MyFruit getOne(@PathVariable("id") Integer id){
return this.myFruitService.getById(id);
}
@GetMapping("/removeOne/{id}")
public void removeOne(@PathVariable("id") Integer id){
this.myFruitService.removeById(id);
}
@GetMapping("/insertOne/test")
public void insertOne(){
MyFruit myFruit=new MyFruit();
myFruit.setAge(4);
myFruit.setName("wocao".getBytes(StandardCharsets.UTF_8));
myFruit.setSize(700L);
System.out.println(myFruit);
this.myFruitService.save(myFruit);
}
@GetMapping("/updataOne/test")
public void updataOne(){
MyFruit myFruit=this.myFruitService.getById(3);
myFruit.setSize(999L);
this.myFruitService.saveOrUpdate(myFruit);
}
@GetMapping("/updateList/test")
public void updateList(){
QueryWrapper<MyFruit> queryWrapper=new QueryWrapper<>();
queryWrapper.eq("size",700L);
MyFruit myFruit=this.myFruitService.getById(3);
myFruit.setSize(10000L);
this.myFruitService.saveOrUpdate(myFruit,queryWrapper);
}
@GetMapping("/findPage/test")
public void findPage(){
IPage<MyFruit> iPage=new Page<>(1,2);
IPage<MyFruit> fruitIPage=this
.myFruitService.page(iPage,null);
Long tot=fruitIPage.getTotal();
System.out.println("tot="+tot);
System.out.println(fruitIPage.getRecords());
}
}