1. 创建maven
项目
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-aspects</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
</dependencies>
2. 创建普通类接口并实现该类
package org.example;
public interface SomeService {
public void doSome(String name, int age);
}
package org.example.impl;
import org.example.SomeService;
import org.springframework.stereotype.Component;
@Component("mySomeServiceImpl")
public class SomeServiceImpl implements SomeService {
@Override
public void doSome(String name, int age) {
System.out.println("doSome is used....");
}
}
3. 实现切面(用于增强业务功能)
package org.example;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.springframework.stereotype.Component;
@Component("myAspectj")
@Aspect
public class MyAspectJ {
@Before("execution(public void org.example.impl.SomeServiceImpl.doSome(String,int))")
public void doLog() {
System.out.println("日志信息.....");
}
}
4. 测试功能
package org.example;
import static org.junit.Assert.assertTrue;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class TestAspectJBefore {
@Test
public void testAspectjBefore() {
String config = "aspectj_before/aspectj_before.xml";
ApplicationContext ac = new ClassPathXmlApplicationContext(config);
SomeService proxy = (SomeService) ac.getBean("mySomeServiceImpl");
proxy.doSome("coder-itl", 18);
}
}
5. 配置文件信息
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/aop https://www.springframework.org/schema/aop/spring-aop.xsd">
<context:component-scan base-package="org.example"/>
<aop:aspectj-autoproxy/>
</beans>
6. 测试输出
AspectJ Before |
---|
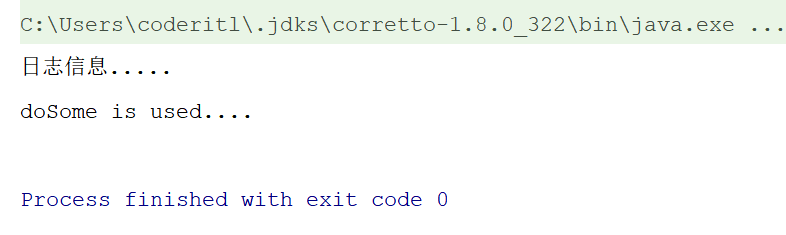 |
|