1.读取properties配置文件
2.读取yml配置文件
3.对于多模块中的读取配置文件的问题
4.将配置文件注入到spring容器中,通过获取对象的方式进行获取
4.1 ConfigurationProperties方式加载配置文件(yml)
4.2ConfigurationProperties(指定配置文件前缀)+PropertySource(指定配置文件类加载路径)
4.3使用@PropertySource加载yml使用方法参考
5.补充: 读取yml中的字符串数组
6.补充:如何从自定义工具类(未受springring容器管理)中读取加载到spring容器中的配置类的信息
7.补充:读取yml中带有上下级关系的配置信息
8.补充:读取resource下文件pem文件内容信息
web项目中有properties配置文件以及yml配置文件,这里整理一下读取配置文件的方式,参考了两位大神的博客.这里表示感谢!
1.读取properties配置文件
public class LoadApplicationUtil {
private static final Logger logger = LoggerFactory.getLogger(LoadApplicationUtil.class);
private static Properties props;
static {
// 初始化加载properties文件进内存
//loadProps();
}
// 加载properties配置文件
synchronized static private void loadProps() {
logger.info("start to load properties.......");
props = new Properties();
InputStream in = null;
try {
in = LoadApplicationUtil.class.getClassLoader().
getResourceAsStream("application-dev.yml");
props.load(in);
logger.info("load propeties success");
} catch (FileNotFoundException e) {
logger.error("properties not found!");
} catch (IOException e) {
logger.error("IOException");
} finally {
try {
if (null != in) {
in.close();
}
} catch (IOException e) {
logger.error("properties close Exception!");
}
}
logger.info("load properties over...........");
}
public static String getProperty(String key) {
if (null == props) {
loadProps();
}
return props.getProperty(key);
}
public static void main(String[] args) {
String property = LoadApplicationUtil.getProperty("personal");
System.out.println(property);
}
}
2.读取yml配置文件
public class LoadApplicationUtil {
private static final Logger logger = LoggerFactory.getLogger(LoadApplicationUtil.class);
// 直接输入配置文件内容进行获取,比如说:aliyun.oss.endpoint
public static String getYmlNew(String key){
Resource resource = new ClassPathResource("application-dev.yml");
Properties properties = null;
try {
YamlPropertiesFactoryBean yamlFactory = new YamlPropertiesFactoryBean();
yamlFactory.setResources(resource);
properties = yamlFactory.getObject();
} catch (Exception e) {
e.printStackTrace();
return null;
}
return (String) properties.get(key);
}
public static void main(String[] args) {
// 读取yml配置文件
String ymlNew = getYmlNew("aliyun.oss.endpoint");
System.out.println(ymlNew);
}
}
3.对于多模块中的读取配置文件的问题
场景:api模块中需要读取配置文件中的自定义配置,公共的配置模块是config,但是api所在模块中是读取不到其他模块中的内容,解决方法是从api模块中自己创建两个文件application-dev.yml,application-prod.yml,然后重写一下相关内容,就能获取到自定义配置文件中的内容.
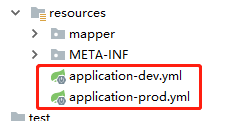
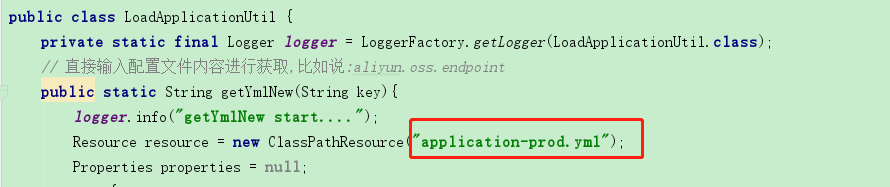
补充: 可以从指定的模块下面添加此工具类来获取指定模块下面的指定配置文件.ClassPathResource只能读取含有ClassPathResource的类所在的模块的根目录下面的所有配置文件,保证Resource resource = new ClassPathResource(“配置文件名”)中的配置文件名与该工具类所在模块下面resource下面的配置文件名一致即可.
4.将配置文件注入到spring容器中,通过获取对象的方式进行获取
4.1 ConfigurationProperties方式加载配置文件(yml)
使用说明:ConfigurationProperties中声明配置文件中的前缀,定义的javabean中的成员变量为配置文件中的属性.注意:ConfigurationProperties注解默认只能加载application.yml配置文件.
配置文件:
配置类:
4.2ConfigurationProperties(指定配置文件前缀)+PropertySource(指定配置文件类加载路径)
配置文件:
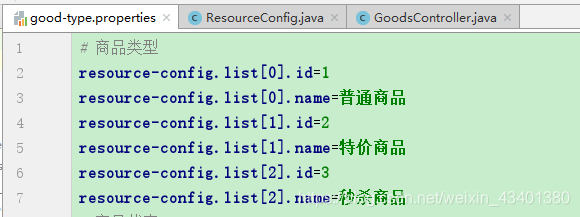
配置类:
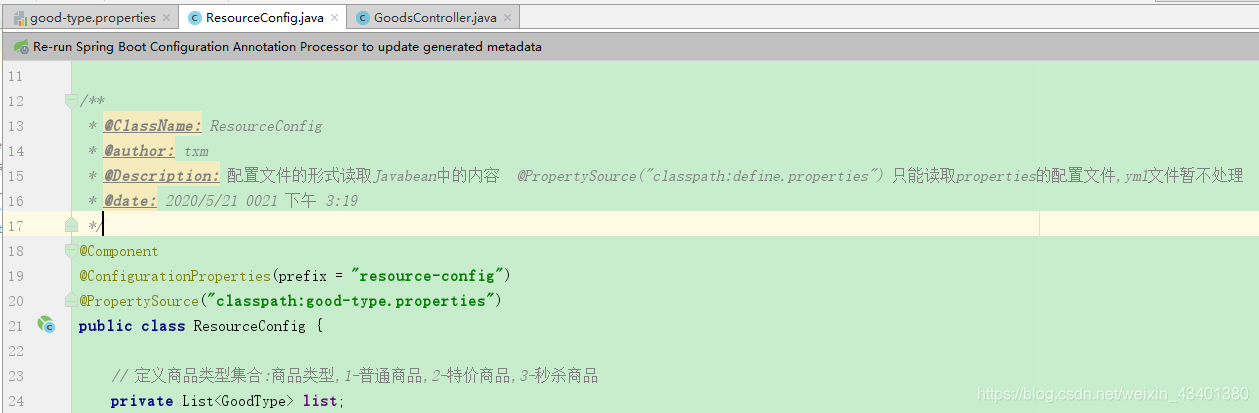
使用:

4.3使用@PropertySource加载yml使用方法参考
使用@PropertySource加载yml使用方法参考:https://blog.csdn.net/weixin_43401380/article/details/113869006
参考:https://blog.csdn.net/weixin_39721052/article/details/85783709
https://blog.csdn.net/u011958281/article/details/81531676
5.补充: 读取yml中的字符串数组
读取内容:
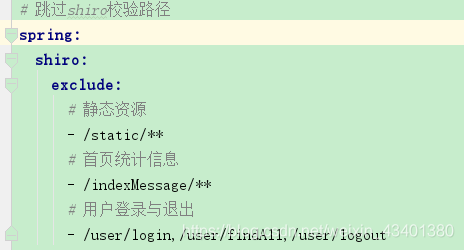
配置文件:
/**
* @ClassName: ShiroAnnoProperty
* @Desc: shiro中跳过校验配置信息
* @Author: txm
* @Date: 2021/5/21 14:17
**/
@Component
@ConfigurationProperties(prefix = "spring.shiro")
public class ShiroAnnoProperty {
private List<String[]> exclude;
public List<String[]> getExclude() {
return exclude;
}
public void setExclude(List<String[]> exclude) {
this.exclude = exclude;
}
}
读取配置文件信息方式:
注入ShiroAnnoProperty对象,然后ShiroAnnoProperty.exclude()
6.补充:如何从自定义工具类(未受springring容器管理)中读取加载到spring容器中的配置类的信息
使用背景说明:自定义的配置类一般都是添加到spring容器进行管理,具体的使用场景是在加载到容器的bean对象中使用@Autowire进行注入,然后获取配置属性;有的时候自定义的工具类,一般是不用spring进行管理,所以不能使用@Autowire进行注入配置类获取配置文件,这里提供一种使用方法,根据bean对象名称或是beanClass对象类型从springring器中进行获取配置对象,然后获取配置对象中的属性.
相关代码如下:
配置类:
工具类:
spring工具类中根据bean名称获取对象方法:
7.补充:读取yml中带有上下级关系的配置信息
配置文件如下:
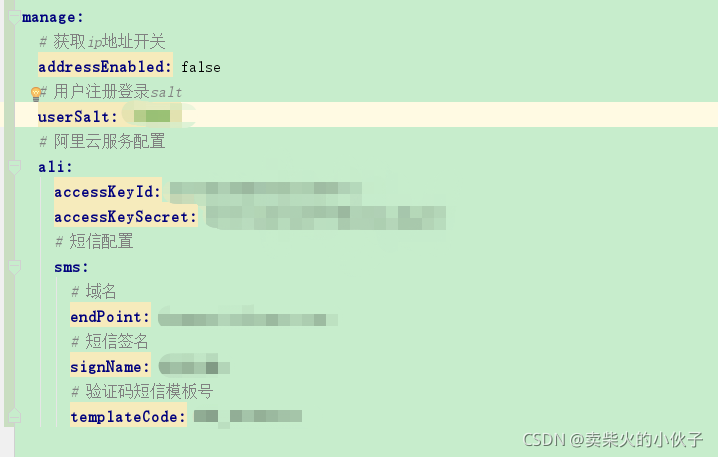
配置类读取方式:
8.补充:读取resource下pem文件内容信息
springboot中使用ResourceLoader对象读取, 这也是官方推荐的读取文件方式.
配置文件目录:
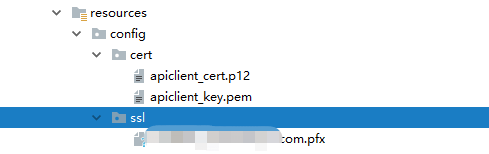
首先进行注册ResourceLoader对象()
// import org.springframework.core.io.ResourceLoader;
@Autowired
private ResourceLoader resourceLoader;
然后可以读取配置文件获取文件相关内容:
Resource resource = resourceLoader.getResource(h5Config.getApiClientKeyPath());
InputStream inputStream = resource.getInputStream();
File file = resource.getFile();
URL url = resource.getURL();
URI uri = resource.getURI();