前言
C#调用ZXing.Dll实现图像编码生成-二维码、条码生成。
案例演示
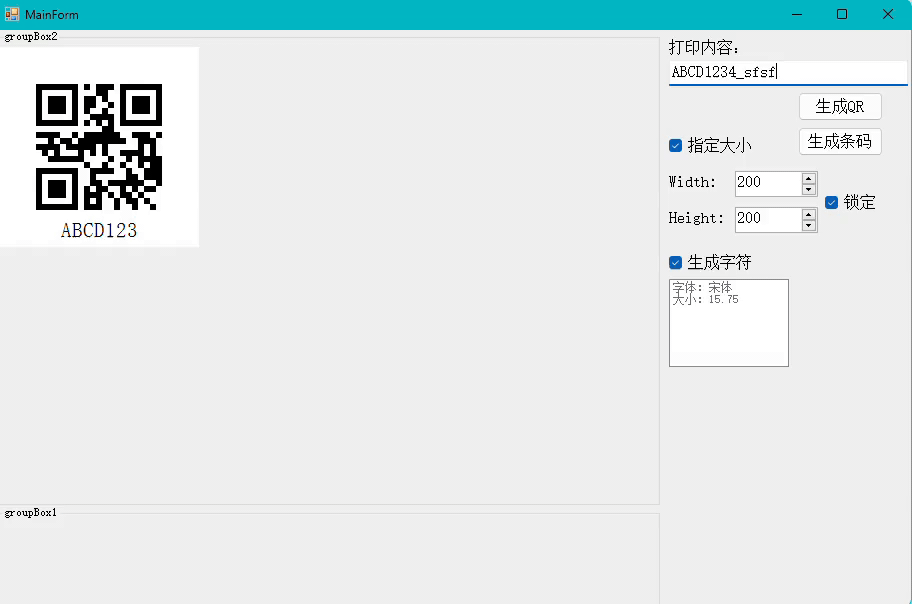
代码
图像编码工具类
public class ImageCodeTool
{
public void GenQRCode(string context, out Bitmap bitmap)
{
string qrCodeText = context;
var qrCodeWriter = new BarcodeWriter
{
Format = BarcodeFormat.QR_CODE,
Options = new ZXing.Common.EncodingOptions
{
Width = 300,
Height = 300
}
};
Bitmap bmp = qrCodeWriter.Write(qrCodeText);
bitmap = new Bitmap(bmp);
bmp.Dispose();
Console.WriteLine("二维码生成成功。");
}
public void GenQRCode(string context, out Bitmap bitmap, int width, int height)
{
string qrCodeText = context;
var qrCodeWriter = new BarcodeWriter
{
Format = BarcodeFormat.QR_CODE,
Options = new ZXing.Common.EncodingOptions
{
Width = width,
Height = height
}
};
Bitmap bmp = qrCodeWriter.Write(qrCodeText);
bitmap = new Bitmap(bmp);
bmp.Dispose();
Console.WriteLine("二维码生成成功。");
}
public void GenBarCode(string context, out Bitmap bitmap)
{
string barcodeText = context;
var barcodeWriter = new BarcodeWriter
{
Format = BarcodeFormat.CODE_128,
Options = new ZXing.Common.EncodingOptions
{
Width = 300,
Height = 150
}
};
Bitmap bmp = barcodeWriter.Write(barcodeText);
bitmap = new Bitmap(bmp);
Console.WriteLine("条码生成成功。");
}
public void GenBarCode(string context, out Bitmap bitmap, int width, int height)
{
string barcodeText = context;
var barcodeWriter = new BarcodeWriter
{
Format = BarcodeFormat.CODE_128,
Options = new ZXing.Common.EncodingOptions
{
Width = width,
Height = height
}
};
Bitmap bmp = barcodeWriter.Write(barcodeText);
bitmap = new Bitmap(bmp);
Console.WriteLine("条码生成成功。");
}
public void AddTextToBitmap(ref Bitmap bitmap, string text,Font contextFont)
{
using (Graphics g = Graphics.FromImage(bitmap))
{
SizeF textSize = g.MeasureString(text, contextFont);
int textX = (bitmap.Width - (int)textSize.Width) / 2;
int textY = bitmap.Height - (int)textSize.Height - 5;
g.DrawString(text, contextFont, Brushes.Black, new PointF(textX, textY));
}
}
}
Form窗体类
public partial class MainForm : Form
{
private Bitmap bitmap;
private int width = 300;
private int height = 300;
private Font ContextFont = new Font("Arial", 12);
private float zoomFactor = 1.0f;
private ImageCodeTool imageCodeTool;
public MainForm()
{
InitializeComponent();
pictureBox1.Location = new Point(10, 10);
this.pictureBox1.MouseWheel += CustomMouseWheel;
}
private void MainForm_Load(object sender, EventArgs e)
{
lbx_FontParams.Items.Clear();
lbx_FontParams.Items.Add($"字体:{ContextFont.Name}");
lbx_FontParams.Items.Add($"大小:{ContextFont.Size}");
imageCodeTool = new ImageCodeTool();
}
private void CustomMouseWheel(object sender, MouseEventArgs e)
{
if (e.Delta > 0)
{
zoomFactor += 0.1f;
}
else if (e.Delta < 0)
{
zoomFactor -= 0.1f;
if (zoomFactor < 0.1f) zoomFactor = 0.1f;
}
pictureBox1.Image = new Bitmap(bitmap, new Size((int)(bitmap.Width * zoomFactor), (int)(bitmap.Height * zoomFactor)));
}
private void btn_QRCode_Click(object sender, EventArgs e)
{
bitmap = null;
if (ckbx_SpecifySize.Checked)
{
width = int.Parse(nudx_Width.Value.ToString());
height = int.Parse(nudx_Height.Value.ToString());
imageCodeTool.GenQRCode(tbx_OCR.Text, out bitmap, width, height);
}
else
{
imageCodeTool.GenQRCode(tbx_OCR.Text, out bitmap);
}
if (ckbx_GenContext.Checked)
{
imageCodeTool.AddTextToBitmap(ref bitmap, tbx_OCR.Text,ContextFont);
}
pictureBox1.Image = bitmap;
}
private void btn_GenBarCode_Click(object sender, EventArgs e)
{
bitmap = null;
if (ckbx_SpecifySize.Checked)
{
width = int.Parse(nudx_Width.Value.ToString());
height = int.Parse(nudx_Height.Value.ToString());
imageCodeTool.GenBarCode(tbx_OCR.Text, out bitmap, width, height);
}
else
{
imageCodeTool.GenBarCode(tbx_OCR.Text, out bitmap);
}
if (ckbx_GenContext.Checked)
{
}
pictureBox1.Invalidate();
pictureBox1.Image = null;
pictureBox1.Image = bitmap;
}
private void ckbx_GenContext_CheckedChanged(object sender, EventArgs e)
{
if (ckbx_GenContext.Checked)
{
if (fontDialog_Context.ShowDialog()== DialogResult.OK)
{
ContextFont = fontDialog_Context.Font;
lbx_FontParams.Items.Clear();
lbx_FontParams.Items.Add($"字体:{ContextFont.Name}");
lbx_FontParams.Items.Add($"大小:{ContextFont.Size}");
}
}
}
private void nudx_Width_ValueChanged(object sender, EventArgs e)
{
if (ckbx_LockWH.Checked)
{
nudx_Height.Value = nudx_Width.Value;
}
}
private void nudx_Height_ValueChanged(object sender, EventArgs e)
{
if (ckbx_LockWH.Checked)
{
nudx_Width.Value = nudx_Height.Value;
}
}
}