要在Java中实现百度浏览器搜索功能,你可以使用Selenium WebDriver。Selenium是一个用于自动化浏览器的工具,WebDriver是Selenium的一个子项目,它提供了一套API,可以直接与浏览器交互。
依赖:
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>3.141.59</version> <!-- 使用最新的版本 -->
</dependency>
</dependencies>
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class BaiduSearch {
public static void main(String[] args) {
// 设置系统属性,告诉WebDriver使用Chrome浏览器
System.setProperty("webdriver.chrome.driver", "D:\\Java\\chromedriver\\chromedriver-win64\\chromedriver.exe");
// 创建ChromeDriver实例
WebDriver driver = new ChromeDriver();
// 打开百度网页
driver.get("https://www.baidu.com");
// 定位搜索框元素
WebElement searchBox = driver.findElement(By.name("wd"));
// 在搜索框中输入关键词
searchBox.sendKeys("毛泽东");
// 提交搜索表单
searchBox.submit();
// 等待一些时间,以便查看搜索结果
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
// 关闭浏览器
driver.quit();
}
}
chrome驱动下载地址: Chrome for Testing availability
测试结果:
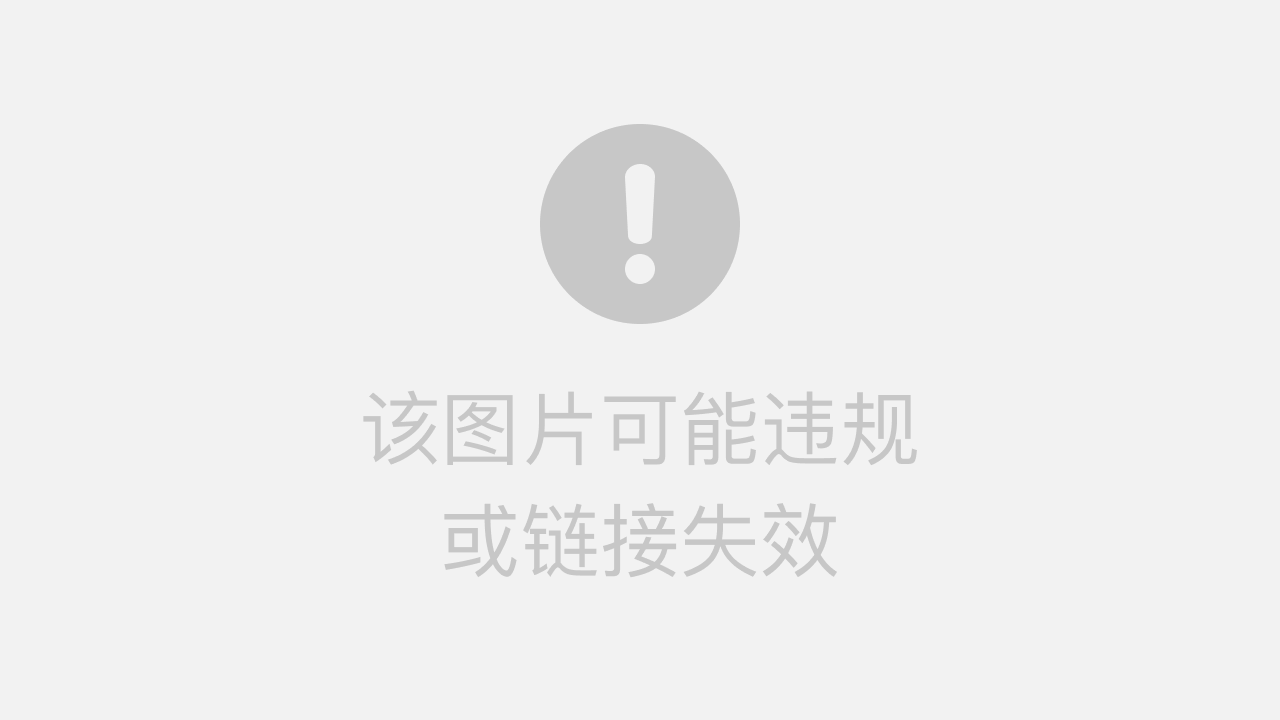
windows/linux通用工具类:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.springframework.stereotype.Component;
import java.util.List;
@Component
public class ChromeUtils {
private StringBuilder sb;
public String baiduSearch(String text) {
WebDriver driver = null;
// windows驱动
// String chromedriverFile = "D:\\Java\\chromedriver\\chromedriver-win64\\chromedriver.exe";
// linux驱动
String chromedriverFile = "/usr/bin/chromedriver-linux64/chromedriver";
try {
/**
* win调出浏览器
*/
// // 设置系统属性,告诉WebDriver使用Chrome浏览器
// System.setProperty("webdriver.chrome.driver", chromedriverFile);
// // 创建ChromeDriver实例
// driver = new ChromeDriver();
/**
* linux/win通用, 无需调出浏览器
*/
// 设置Chrome浏览器的启动参数
ChromeOptions options = new ChromeOptions();
options.addArguments("--no-sandbox"); // 禁用沙盒模式
options.addArguments("--disable-dev-shm-usage"); // 禁用/dev/shm使用
options.addArguments("--headless"); // 以无头模式运行
// 设置系统属性,告诉WebDriver使用Chrome浏览器
System.setProperty("webdriver.chrome.driver", chromedriverFile);
// 创建ChromeDriver实例时传递配置参数
driver = new ChromeDriver(options);
// 打开百度网页
driver.get("https://www.baidu.com");
// 定位搜索框元素
WebElement searchBox = driver.findElement(By.name("wd"));
// 在搜索框中输入关键词
searchBox.sendKeys(text);
// 提交搜索表单
searchBox.submit();
// Loop to scrape results from the first two pages
for (int page = 0; page < 2; page++) {
// 等待一些时间,以便查看搜索结果
Thread.sleep(5000);
// 定位搜索结果的父元素,这里使用百度搜索结果的<div>标签
List<WebElement> searchResults = driver.findElements(By.xpath("//div[@class='c-container']"));
this.sb = (sb != null) ? sb : new StringBuilder();
// 打印搜索结果的文本内容
for (WebElement result : searchResults) {
sb.append(result.getText());
}
// Go to the next page if it's not the last iteration
if (page < 1) {
// 等待 "下一页" 按钮可见
WebDriverWait wait = new WebDriverWait(driver, 60);
WebElement nextPageButton = wait.until(ExpectedConditions.elementToBeClickable(By.xpath("//a[@class='n' and contains(text(),'下一页')]")));
// Go to the next page
nextPageButton.click();
}
}
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
if (driver != null) {
// 关闭浏览器
driver.quit();
}
}
return sb.toString().replaceAll("\n", "");
}
}