组件间数据传递
1. 父子组件
在一个组件内部定义另一个组件,称为父子组件
子组件只能在父组件内部使用
默认情况下,子组件无法访问父组件中的数据,每个组件实例的作用域是独立的
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>vue</title>
<script src="js/vue.js"></script>
</head>
<body>
<div class="itany">
<my-hello></my-hello>
</div>
<template id="hello">
<div>
<h3>我是hello组件</h3>
<my-world></my-world>
</div>
</template>
<template id="world">
<div>
<h3>我是world组件</h3>
</div>
</template>
</body>
<script>
var vm = new Vue({ //根组件
el: '.itany',
components: {
'my-hello': { //父组件
methods: {
getData(sex, height) {
this.sex = sex;
this.height = height;
}
},
data() {
return {
msg: 'web',
name: 'tom',
age: 23,
user: {
id: 9527,
username: '唐伯虎'
},
sex: '',
height: ''
}
},
template: '#hello',
components: {
'my-world': { //子组件
data() {
return {
sex: 'male',
height: 180.5
}
},
template: '#world',
}
}
}
}
});
</script>
</html>
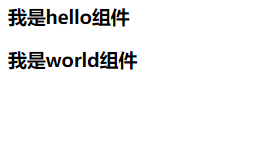
2. 组件间数据传递 (通信)
2.1 子组件访问父组件的数据
a)在调用子组件时,绑定想要获取的父组件中的数据
b)在子组件内部,使用props选项声明获取的数据,即接收来自父组件的数据
总结:父组件通过props向下传递数据给子组件
注:组件中的数据共有三种形式:data、props、computed
文档
https://cn.vuejs.org/v2/guide/components.html#%E9%80%9A%E8%BF%87-Prop-%E5%90%91%E5%AD%90%E7%BB%84%E4%BB%B6%E4%BC%A0%E9%80%92%E6%95%B0%E6%8D%AE
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>vue</title>
<script src="js/vue.js"></script>
</head>
<body>
<div id="itany">
<h2>父组件:{{name}}</h2>
<hr>
<!-- 第3步:父数据传给子 -->
<my-hello :name="name"></my-hello>
</div>
<template id="hello">
<div>
<!-- 第4步:显示父数据 -->
<h3>子组件:{{name}}</h3>
</div>
</template>
</body>
<script>
var vm = new Vue({ //父组件
el: '#itany',
data: { //第1步:定义数据
name: 'tom',
},
components: {
'my-hello': { //子组件
template: '#hello',
props: ['name'] //第2步:子获取父的数据
}
}
});
</script>
</html>
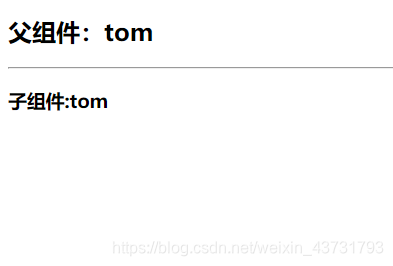
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>vue</title>
<script src="js/vue.js"></script>
</head>
<body>
<div class="itany">
<my-hello></my-hello>
</div>
<template id="hello">
<div>
<h3>我是hello组件</h3>
<h3>访问自己的数据:{{msg}},{{name}},{{age}},{{user.username}}</h3>
<my-world :message="msg" ></my-world>
</div>
</template>
<template id="world">
<div>
<h3>我是world组件</h3>
<h4>访问父组件中的数据:{{message}}</h4>
</div>
</template>
</body>
<script>
var vm = new Vue({ //根组件
el: '.itany',
components: {
'my-hello': { //父组件
methods: {
getData(sex, height) {
this.sex = sex;
this.height = height;
}
},
data() {
return {
msg: 'WEB',
name: 'tom',
age: 23,
user: {
id: 9527,
username: '唐伯虎'
},
}
},
template: '#hello',
components: {
'my-world': { //子组件
template: '#world',
props: ['message']
}
}
}
}
});
</script>
</html>
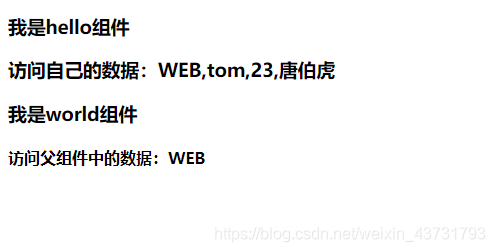
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>vue</title>
<script src="js/vue.js"></script>
</head>
<body>
<div class="itany">
<my-hello></my-hello>
</div>
<template id="hello">
<div>
<h3>我是hello组件</h3>
<h3>访问自己的数据:{{msg}},{{name}},{{age}},{{user.username}}</h3>
<my-world :message="msg" :name="name" :age="age" user="user"></my-world>
</div>
</template>
<template id="world">
<div>
<h3>我是world组件</h3>
<h4>访问父组件中的数据:{{message}},{{name}},{{age}},{{user.username}}</h4>
</div>
</template>
</body>
<script>
var vm = new Vue({ //根组件
el: '.itany',
components: {
'my-hello': { //父组件
methods: {
getData(sex, height) {
this.sex = sex;
this.height = height;
}
},
data() {
return {
msg: 'WEB',
name: 'tom',
age: 23,
user: {
id: 9527,
username: '唐伯虎'
},
}
},
template: '#hello',
components: {
'my-world': { //子组件
template: '#world',
props: ['message', 'name', 'age', 'user']
}
}
}
}
});
</script>
</html>
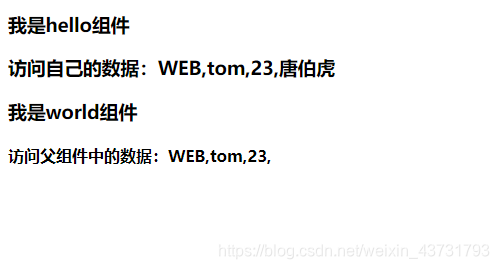
Prop 验证
文档
https://cn.vuejs.org/v2/guide/components-props.html#Prop-%E9%AA%8C%E8%AF%81
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>vue</title>
<script src="js/vue.js"></script>
</head>
<body>
<div class="itany">
<my-hello></my-hello>
</div>
<template id="hello">
<div>
<h3>我是hello组件</h3>
<h3>访问自己的数据:{{msg}},{{name}},{{age}},{{user.username}}</h3>
<my-world :message="msg" :name="name" :age="age" :user="user"></my-world>
</div>
</template>
<template id="world">
<div>
<h3>我是world组件</h3>
<h4>访问父组件中的数据:{{message}},{{name}},{{age}},{{user.username}}</h4>
</div>
</template>
</body>
<script>
var vm = new Vue({ //根组件
el: '.itany',
components: {
'my-hello': { //父组件
methods: {
getData(sex, height) {
this.sex = sex;
this.height = height;
}
},
data() {
return {
msg: 'WEB',
name: 'tom',
age: 23,
user: {
id: 9527,
username: '唐伯虎'
},
}
},
template: '#hello',
components: {
'my-world': { //子组件
template: '#world',
// props: ['message', 'name', 'age', 'user']//简单的字符串数组
props: { //也可以是对象,允许配置高级设置,如类型判断、数据校验、设置默认值
message: String,
name: {
type: String,
required: true
},
age: {
type: Number,
default: 18,
validator: function(value) {
return value >= 0;
}
},
user: {
type: Object,
default: function() { //对象或数组的默认值必须使用函数的形式来返回
return {
id: 3306,
username: '秋香'
};
}
}
},
}
}
}
}
});
</script>
</html>