1.完整代码
#include<iostream>
#include<malloc.h>
using namespace std;
#define TElemType int
#define TRUE 1
#define FALSE 0
#define OK 1
#define ERROR 0
#define OVERFLOW -2
typedef struct BiTNode{
TElemType data;
struct BiTNode *lchild, *rchild;
}BiTNode, *BiTree;
int CreateBiTree(BiTree &T)
{
TElemType a;
scanf("%d", &a);
if ( 0 == a )
{
T = NULL;
}
else
{
T = (BiTree)malloc(sizeof(BiTNode));
if ( !T )
exit(OVERFLOW);
T->data = a;
CreateBiTree(T->lchild);
CreateBiTree(T->rchild);
}
return OK;
}
void PreOrderTraverse(BiTree T)
{
if ( T != NULL )
{
printf("%d ", T->data);
PreOrderTraverse(T->lchild);
PreOrderTraverse(T->rchild);
}
}
int Copy(BiTree T, BiTree &NewT)
{
if ( T == NULL )
{
NewT = NULL;
return ERROR;
}
else
{
NewT = new BiTNode;
NewT->data = T->data;
Copy(T->lchild, NewT->lchild);
Copy(T->rchild, NewT->rchild);
}
return OK;
}
int Depth(BiTree T)
{
int m, n;
if ( T == NULL )
return ERROR;
else
{
m = Depth(T->lchild);
n = Depth(T->rchild);
if ( m > n )
return (m+1);
else
return (n+1);
}
}
int NodeCount(BiTree T)
{
if ( T == NULL )
return ERROR;
else
return NodeCount(T->lchild) + NodeCount(T->rchild) + 1;
}
int LeafCount(BiTree T)
{
if ( T == NULL )
return 0;
if ( T->lchild == NULL && T->rchild == NULL )
return 1;
else
return LeafCount(T->lchild) + LeafCount(T->rchild);
}
int main()
{
BiTree T = NULL;
BiTree newT = NULL;
cout << "请按照先序遍历输入二叉树('0'无): ";
CreateBiTree(T);
Copy(T, newT);
cout << "先序遍历原二叉树: ";
PreOrderTraverse(T);
cout << "\n";
cout << "先序遍历复制的二叉树: ";
PreOrderTraverse(newT);
cout << "\n";
cout << "二叉树的深度为: ";
cout << Depth(T) << endl;
cout << "二叉树的结点总数为: ";
cout << NodeCount(T) << endl;
cout << "二叉树的叶子结点总数为: ";
cout << LeafCount(T) << endl;
return 0;
}
2.测试结果
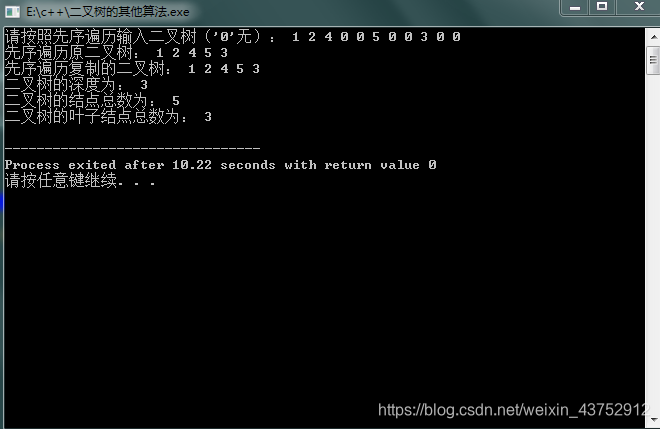