1.FastDFS总结
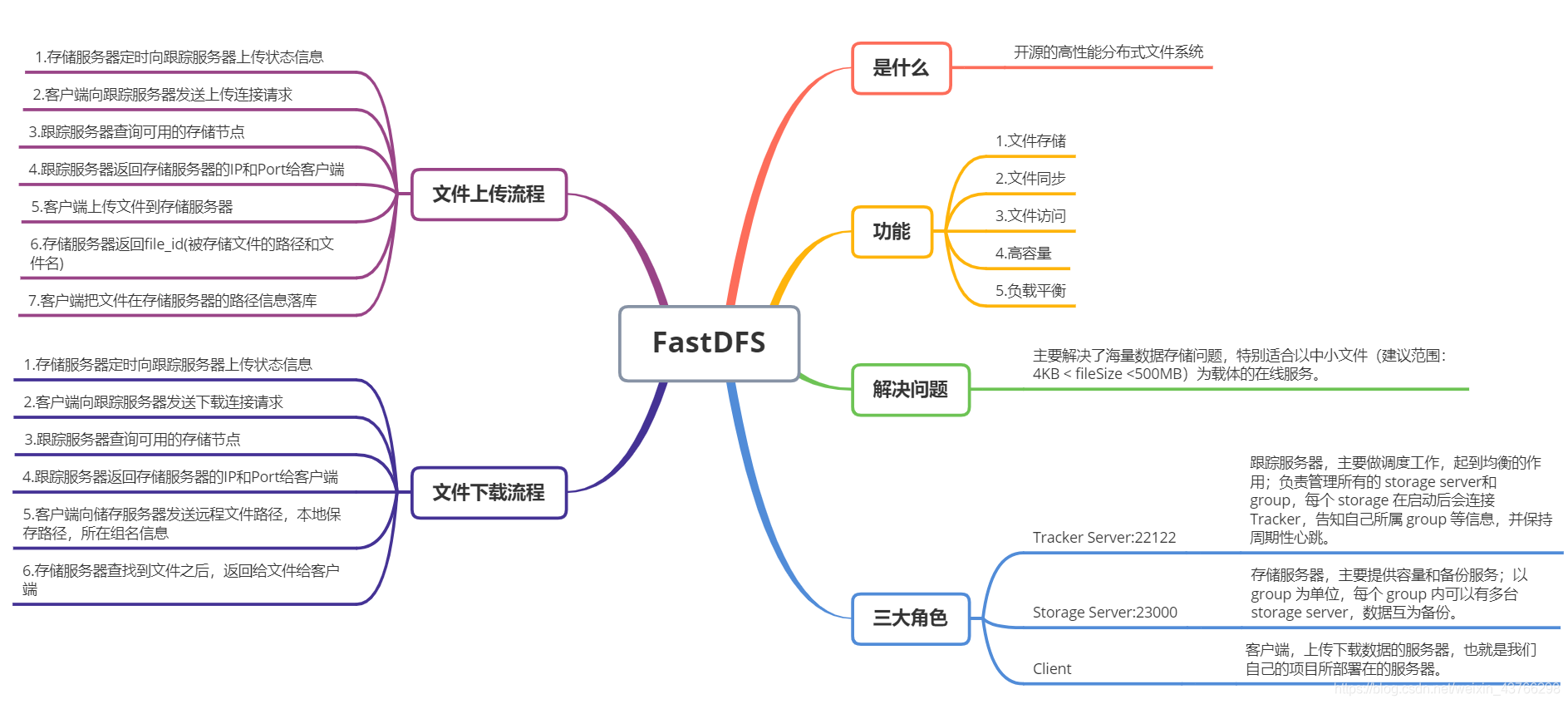
2.FastDFS使用流程
- 1.Linux上搭建FastDFS。
- 2.安装fastdfs_client-1.25jar包到Maven本地仓库。
mvn install:install-file -Dfile=D:\XLYY\fastdfs_client-1.25.jar -DgroupId=fastdfs_client -DartifactId=fastdfs_client -Dversion=1.25 -Dpackaging=jar
<dependency>
<groupId>fastdfs_client</groupId>
<artifactId>fastdfs_client</artifactId>
<version>1.25</version>
</dependency>
- 4.修改fdfs_client.conf文件,将该文件放在resources路径下
# 连接超时(以秒为单位)
connect_timeout=30
# 网络超时(以秒为单位)
network_timeout=60
# 存储日志文件的基本路径
base_path=/home/fastdfs
# 文件服务器地址
# tracker的默认端口号22122和storage的默认端口号23000
tracker_server=xx.xx.xx.xx:22122
#标准日志级别为syslog,不区分大小写,值列表:
### emerg for emergency
### alert
### crit for critical
### error
### warn for warning
### notice
### info
### debug
log_level=info
# 是否使用连接池 默认值为false 自V4.05起
use_connection_pool = false
#空闲时间超过此时间的连接将被关闭
#单位:秒
#默认值为3600
#自V4.05起
connection_pool_max_idle_time = 3600
#如果从跟踪服务器加载FastDFS参数
#自V4.05起
#默认值为false
load_fdfs_parameters_from_tracker=false
#如果使用存储ID代替IP地址
#与tracker.conf相同
#仅在load_fdfs_parameters_from_tracker为false时有效
#默认值为false
#自V4.05起
use_storage_id = false
# 指定存储ID的文件名,可以使用相对或绝对路径
# 与tracker.conf相同
# 仅在load_fdfs_parameters_from_tracker为false时有效
# 自V4.05起
storage_ids_filename = storage_ids.conf
# 设置该跟踪器服务器上的HTTP端口
http.tracker_server_port=8080
#使用“include”指令包含HTTP其他设置
## include http.conf
import org.csource.common.NameValuePair;
import org.csource.fastdfs.*;
import java.io.FileOutputStream;
import java.util.Date;
public class FastDFSClient {
private TrackerClient trackerClient = null;
private TrackerServer trackerServer = null;
private StorageServer storageServer = null;
private StorageClient1 storageClient = null;
public FastDFSClient(String conf) throws Exception {
if (conf.contains("classpath:")) {
conf = conf.replace("classpath:", this.getClass().getResource("/").getPath());
}
ClientGlobal.init(conf);
trackerClient = new TrackerClient();
trackerServer = trackerClient.getConnection();
storageServer = null;
storageClient = new StorageClient1(trackerServer, storageServer);
}
public String uploadFile(String fileName, String extName, NameValuePair[] metas) throws Exception {
String result = storageClient.upload_file1(fileName, extName, metas);
return result;
}
public String uploadFile(String fileName) throws Exception {
return uploadFile(fileName, null, null);
}
public String uploadFile(String fileName, String extName) throws Exception {
return uploadFile(fileName, extName, null);
}
public String uploadFile(byte[] fileContent, String extName, NameValuePair[] metas) throws Exception {
String result = storageClient.upload_file1(fileContent, extName, metas);
return result;
}
public String uploadFile(byte[] fileContent) throws Exception {
return uploadFile(fileContent, null, null);
}
public String uploadFile(byte[] fileContent, String extName) throws Exception {
return uploadFile(fileContent, extName, null);
}
public void download(String filePath, String savePath,String groupName) {
try {
byte[] bytes = storageClient.download_file(groupName,filePath);
FileOutputStream fos = new FileOutputStream(savePath);
fos.write(bytes,0,bytes.length);
} catch (Exception e) {
e.printStackTrace();
}
}
public Boolean deleteFile(String filePath,String groupName) {
try {
int i = storageClient.delete_file(groupName,filePath);
return i == 0;
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
public String getFileInfo(String filePath,String groupName) {
try {
FileInfo fileInfo = storageClient.get_file_info(groupName,filePath);
String sourceIpAddr = fileInfo.getSourceIpAddr();
long fileSize = fileInfo.getFileSize();
Date createTimestamp = fileInfo.getCreateTimestamp();
long crc32 = fileInfo.getCrc32();
System.out.println(sourceIpAddr);
System.out.println(createTimestamp);
System.out.println(crc32);
return filePath;
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
@RestController
@Slf4j
public class FileUploadController {
@Value("${IMAGE_URL_PREFIX}")
private String IMAGE_URL_PREFIX;
@PostMapping("/file_upload")
public ResponseBean<Object> fileUpload(@RequestParam("file") MultipartFile file){
String originalFilename = file.getOriginalFilename();
log.warn("上传的文件名称是:{}",originalFilename);
String extName = originalFilename.substring(originalFilename.lastIndexOf(".") + 1);
log.warn("文件的后缀名是:{}",extName);
try {
FastDFSClient fastDFSClient = new FastDFSClient("classpath:conf/fdfs_client.conf");
String urlSuffix = fastDFSClient.uploadFile(file.getBytes(), extName);
String url = IMAGE_URL_PREFIX+urlSuffix;
log.warn("文件上传成功后的地址是: {}",url);
return ResponseBean.ok("上传文件成功!!!").putData(url);
}catch (Exception e){
log.error(e.getMessage(),e);
}
return ResponseBean.error("文件上传失败!!!");
}
}
public class AdminLoginController {
@Resource
private AdminFeignClient adminFeignClient;
public static final String GROUP_NAME="group1";
public static final String FASTDFS_CONF_LOCATION="classpath:conf/fdfs_client.conf";
@ResponseBody
@PostMapping("/admin_change_photo")
public ResponseBean<Object> changePhoto(Admin admin,
String oldPhoto,
HttpSession session){
Admin loginUser = (Admin)session.getAttribute("admin");
admin.setId(loginUser.getId());
admin.setUsername(loginUser.getUsername());
int i = adminFeignClient.changePhoto(admin);
if (i >0){
loginUser.setPhoto(admin.getPhoto());
session.setAttribute("admin",loginUser);
}
if (i > 0 && !StringUtils.isEmpty(oldPhoto) && !oldPhoto.equals(admin.getPhoto())){
try{
FastDFSClient fastDFSClient = new FastDFSClient(FASTDFS_CONF_LOCATION);
Boolean isDelete = fastDFSClient.deleteFile(oldPhoto.substring(32), GROUP_NAME);
log.warn("删除旧图像的结果是: {}",isDelete);
}catch (Exception e){
log.error("FastDFS出现异常",e);
}
}
return i > 0 ? ok(SUCCESSFUL_OPERATION): error(INTERNAL_SERVER_ERROR);
}
}