一、哈希表
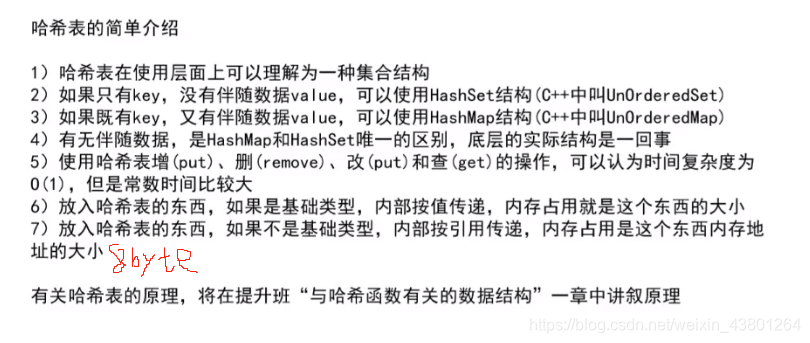
二、有序表
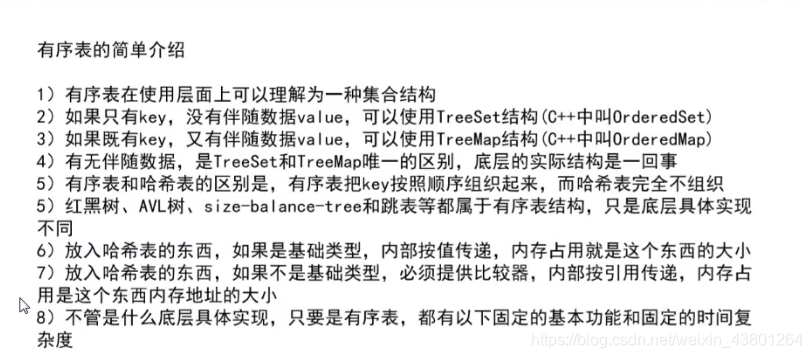
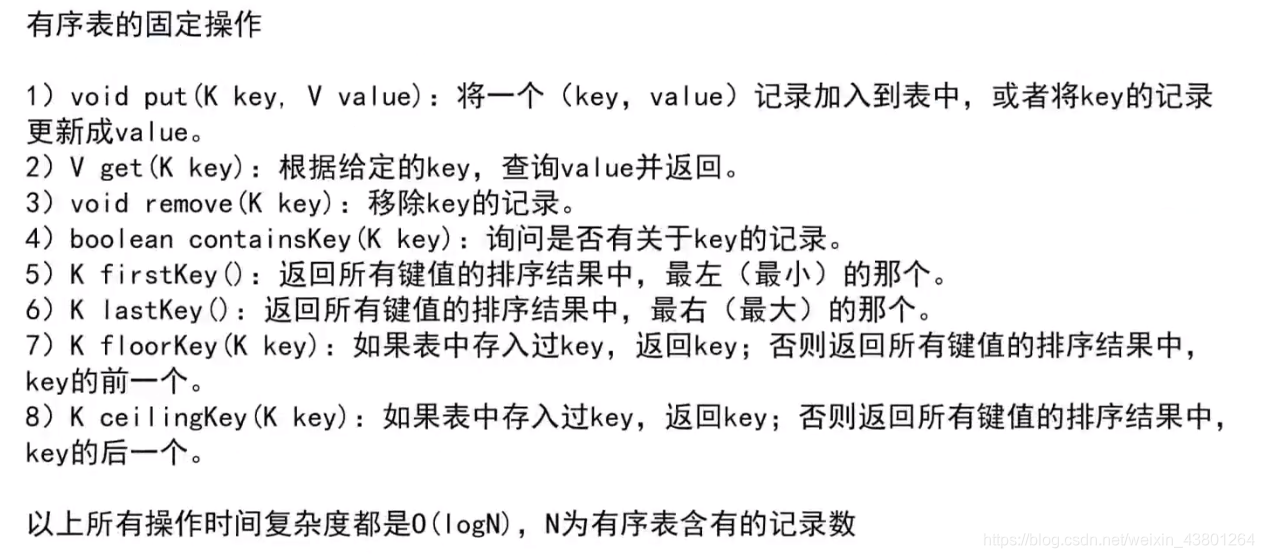
三、单链表和双链表
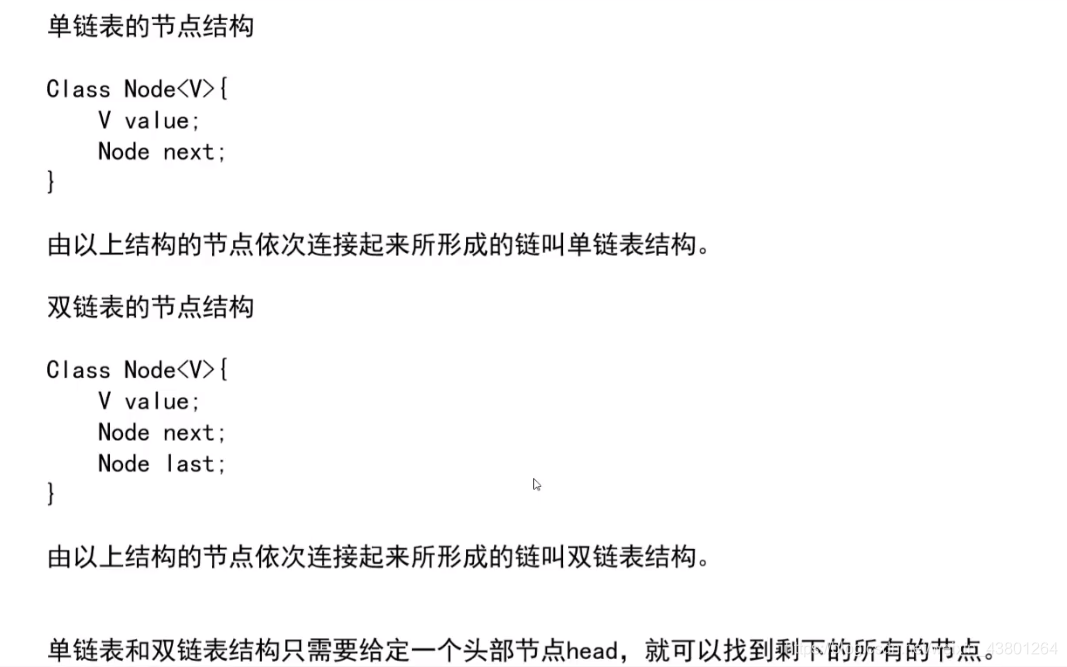
四、反转单链表和双链表(要求链表长度为N,时间复杂度为O(N),额外空间复杂度为O(1))
public class ReverseLinkedList {
public static class Node {
public int value;
public Node next;
public Node(int value) {
this.value = value;
}
}
public static Node reverseLinkedList(Node head) {
if(head == null){
return null;
}
Node pre = null;
Node next = null;
while(head != null){
next = head.next;
head.next = pre;
pre = head;
head = next;
}
return pre;
}
}
public class ReverseDoubleLinkedList {
public static class DoubleNode {
int val;
DoubleNode pre;
DoubleNode next;
public DoubleNode (int value) {
this.val = value;
}
}
public static DoubleNode reverseDoubleLinkedList(DoubleNode head) {
if(head == null){
retrun null;
}
DoubleNode tmp = null;
DoubleNode res = null;
while(head != null) {
tmp = head.next;
head.next = head.pre;
head.pre = tmp;
res = head;
head = tmp;
}
return res;
}
}
五、给定两个有序链表的头指针head1和head2,打印两个链表的公共部分(要求:两个链表长度和为N,时间复杂度为O(N),空间复杂度为O(1))
public class PrintCommonPart {
public static class Node {
public int value;
public Node next;
public Node(int data) {
this.value = data;
}
}
public static void printCommonPart(Node head1, Node head2) {
while (head1 != null && head2 != null) {
if (head1.value < head2.value) {
head1 = head1.next;
} else if (head1.value > head2.value) {
head2 = head2.next;
} else {
System.out.print(head1.value+" ");
head1 = head1.next;
head2 = head2.next;
}
}
System.out.println();
}
}
六、判断一个链表是否为回文结构
public class IsPalindrome {
public static class Node{
public int value;
public Node next;
public Node(int value){
this.value = date;
}
}
public static boolean isPalindrome1(Node head){
if(head == null || head.next == null){
return true;
}
Stack<Node> stack = new Stack<Node>();
Node cur = head;
while(cur != null){
stack.push(cur);
cur = cur.next;
}
while(head != null){
if(head.value != stack.pop().value){
return false;
}
head = head.next