2021.3.19
146. LRU 缓存机制
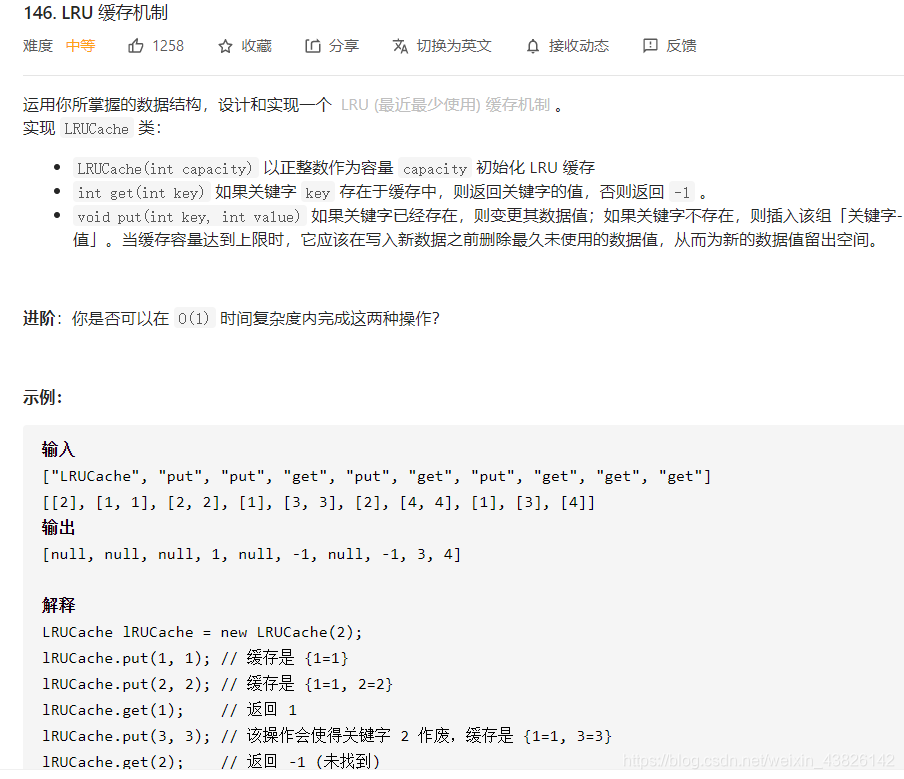
class LRUCache {
int capacity;
LinkedHashMap<Integer, Integer> cache = new LinkedHashMap<>(16,0.75f,true);
public LRUCache(int capacity) {
this.capacity = capacity;
}
public int get(int key) {
if (!cache.containsKey(key)){
return -1;
}
return cache.get(key);
}
public void put(int key, int value) {
if (cache.containsKey(key)){
cache.put(key,value);
return;
}
if (cache.size() >= this.capacity){
int oldestKey = cache.keySet().iterator().next();
cache.remove(oldestKey);
}
cache.put(key,value);
}
}
460. LFU 缓存
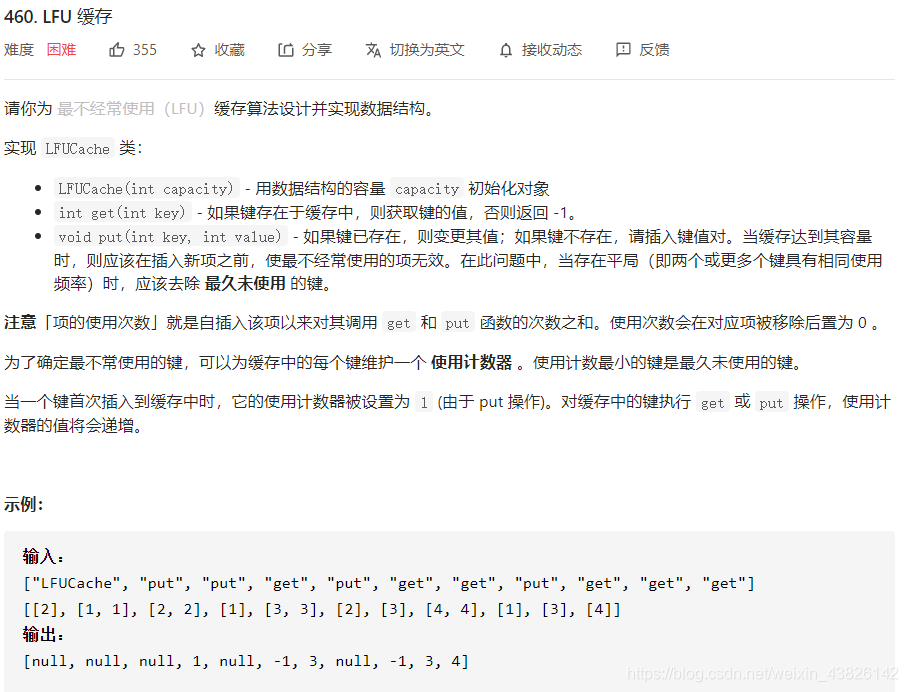
class LFUCache {
HashMap<Integer, Integer> keyToVal;
HashMap<Integer, Integer> keyToFreq;
HashMap<Integer, LinkedHashSet<Integer>> freqToKeys;
int minFreq;
int cap;
public LFUCache(int capacity) {
this.keyToVal = new HashMap<>();
this.keyToFreq = new HashMap<>();
this.freqToKeys = new HashMap<>();
this.cap = capacity;
this.minFreq = 0;
}
public int get(int key) {
if (!keyToVal.containsKey(key)) {
return -1;
}
increaseFreq(key);
return keyToVal.get(key);
}
public void put(int key, int value) {
if (this.cap <= 0) {
return;
}
if (keyToVal.containsKey(key)) {
keyToVal.put(key, value);
increaseFreq(key);
return;
}
if (this.cap <= keyToVal.size()) {
removeMinFreqKey();
}
keyToVal.put(key, value);
keyToFreq.put(key, 1);
freqToKeys.putIfAbsent(1, new LinkedHashSet<>());
freqToKeys.get(1).add(key);
this.minFreq = 1;
}
private void removeMinFreqKey() {
LinkedHashSet<Integer> keyList = freqToKeys.get(this.minFreq);
int deletedKey = keyList.iterator().next();
keyList.remove(deletedKey);
if (keyList.isEmpty()) {
freqToKeys.remove(this.minFreq);
}
keyToVal.remove(deletedKey);
keyToFreq.remove(deletedKey);
}
private void increaseFreq(int key) {
int freq = keyToFreq.get(key);
keyToFreq.put(key, freq + 1);
freqToKeys.get(freq).remove(key);
freqToKeys.putIfAbsent(freq + 1, new LinkedHashSet<>());
freqToKeys.get(freq + 1).add(key);
if (freqToKeys.get(freq).isEmpty()) {
freqToKeys.remove(freq);
if (freq == this.minFreq) {
this.minFreq++;
}
}
}
}