vue3.0之Pinia(状态管理工具)
import { createApp } from 'vue'
import App from './App.vue'
import "./assets/css/reset.css"
import Card from './components/card/Card.vue'
import DragDirective from "./directive/drag.ts"
const app = createApp(App)
import { createPinia } from "pinia"
const store= createPinia()
app.use(store)
app.use(DragDirective).component('Card',Card).mount('#app')
创建一个pinia状态管理仓库
- src / store / index.ts
- src / store / store-namespce.ts
index.ts
import { defineStore } from 'pinia'
import { Names } from './store-namespce'
export const useTestStore = defineStore(Names.Test, {
state:()=>{
return {
current:1,
name:"xzl"
}
},
getters:{
},
actions:{
}
})
store-namespce.ts
export const enum Names {
Test = 'TEST'
}
访问 pinia之中的 state数据
<template>
<div>
我是app
<div>{{ Test.current }} -- {{ Test.name }}</div>
</div>
</template>
<script setup lang="ts">
import { useTestStore } from './store/index'
const Test = useTestStore()
- 效果
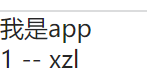
修改pinia之中的 state 总共有5种方式
- 推荐使用的是
- 方式5:触发 actions
- 方式3:工程函数的形式 修改 里面包含 逻辑 -> 推荐之
- 方式1:直接修改 pinia 里面的 state 数据
store-namespce.ts
export const enum Names {
Test = 'TEST'
}
store / indes.ts
import { defineStore } from 'pinia'
import { Names } from './store-namespce'
export const useTestStore = defineStore(Names.Test, {
state:()=>{
return {
current:1,
name:"xzl"
}
},
getters:{
},
actions:{
setCurrent (num:Number) {
this.current = num
}
}
})
App.vue
<template>
<!-- <Layout></Layout> -->
<div>
我是app
<div>{{ Test.current }} -- {{ Test.name }}</div>
<button @click="changeNum">修改</button>
</div>
</template>
<script setup lang="ts">
import { useTestStore } from './store/index'
const Test = useTestStore()
const changeNum = () => {
Test.setCurrent(999)
}
</script>
<style lang="scss">
html,
body,
#app {
height: 100%;
width: 100%;
overflow: hidden;
}
</style>
解构store 函数 使得解构的数据具有响应式
store / index.ts
import { defineStore } from 'pinia'
import { Names } from './store-namespce'
export const useTestStore = defineStore(Names.Test, {
state:()=>{
return {
current:1,
name:"xzl"
}
},
getters:{
},
actions:{
}
})
app.vue
<template>
<div>
我是app
<div>{{ current }} -- {{ Test.name }}</div>
<button @click="changeNum">修改</button>
</div>
</template>
<script setup lang="ts">
import { useTestStore } from './store/index'
const Test = useTestStore()
import { storeToRefs } from 'pinia'
let { current, name } = storeToRefs(Test)
const changeNum = () => {
current.value++
Test.$patch(() => {
if (current.value % 2 === 0) {
name.value = '偶数-小小狗'
} else {
name.value = '基数-小小狗'
}
})
}
</script>
<style lang="scss">
html,
body,
#app {
height: 100%;
width: 100%;
overflow: hidden;
}
</style>
- 效果
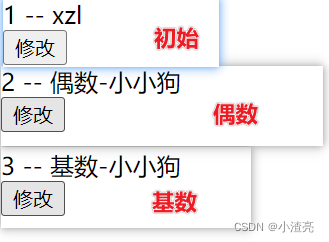
pinia实现一个 简单的异步 修改 state数据 (与同步)
store / index.ts
import { defineStore } from 'pinia'
import { Names } from './store-namespce'
type UserInfo = {
name:String
age:Number
}
let user:UserInfo = {
name:"xxx",
age:11
}
const Login = ():Promise<UserInfo> =>{
return new Promise((resolve)=>{
setTimeout(()=>{
resolve({
name:"xzl",
age:20
})
},2000)
})
}
export const useTestStore = defineStore(Names.Test, {
state:()=>{
return {
user:<UserInfo>{},
userInfo:<UserInfo>{},
}
},
getters:{
},
actions:{
setUser (){
this.user = user
},
async setUserAsync () {
const res = await Login()
this.userInfo = res;
console.log("res", res);
}
}
})
app.vue
<template>
<!-- <Layout></Layout> -->
<div>
我是app
<div>user - {{ Test.user }}</div>
<button @click="changeUser">修改用户</button>
<div>userInfo - {{ Test.userInfo }}</div>
<button @click="setUserAsync">修改用户async</button>
</div>
</template>
<script setup lang="ts">
import { useTestStore } from './store/index'
const Test = useTestStore()
const changeUser = () => {
Test.setUser()
}
const setUserAsync = () => {
Test.setUserAsync()
}
</script>
<style lang="scss">
html,
body,
#app {
height: 100%;
width: 100%;
overflow: hidden;
}
</style>
- 效果
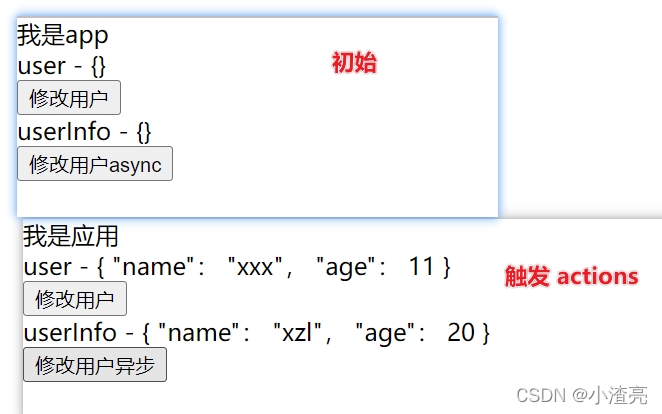
多个 actions之间 调用
store / index.ts
import { defineStore } from 'pinia'
import { Names } from './store-namespce'
type UserInfo = {
name:String
age:Number
}
const Login = ():Promise<UserInfo> =>{
return new Promise((resolve)=>{
setTimeout(()=>{
resolve({
name:"xzl",
age:20
})
},2000)
})
}
export const useTestStore = defineStore(Names.Test, {
state:()=>{
return {
user:<UserInfo>{},
userInfo:<UserInfo>{},
}
},
getters:{
},
actions:{
async setUserAsync () {
const res = await Login()
this.userInfo = res;
this.setName()
},
setName (){
this.userInfo.name = "采狗"
}
}
})
pinia 之中的 getters 写法
store / index.ts
import { defineStore } from 'pinia'
import { Names } from './store-namespce'
export const useTestStore = defineStore(Names.Test, {
state:()=>{
return {
name:"小菜鸡"
}
},
getters:{
getNewName():string {
return `${this.name}`
}
},
actions:{
changeName(name:string){
this.name = name
}
}
})
app.vue
<template>
<!-- <Layout></Layout> -->
<div>
我是app
<div>getNewName - {{ Test.getNewName }}</div>
<button @click="changeName">修改name</button>
</div>
</template>
<script setup lang="ts">
import { useTestStore } from './store/index'
const Test = useTestStore()
const changeName = () => {
Test.changeName('大菜鸡')
}
</script>
- 效果
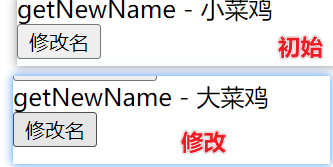