vue3 实现截图当前dom节点为图片与下载为pdf文件
utils / index.ts
- 安装依赖
js pnpm i html2canvas -D pnpm i jspdf -D
import html2canvas from 'html2canvas';
import jsPDF from 'jspdf';
import type { HTMLElement } from "vue"
export function downPicture(dom:HTMLElement,name:string) {
const shareContent = dom;
const width = shareContent.offsetWidth ;
const height = shareContent.offsetHeight ;
const canvas = document.createElement('canvas');
canvas.width = width * 1.4;
canvas.height = height * 1.4;
let a = document.createElement('a');
let opts = {
canvas,
scale: 1.4,
logging: false,
useCORS: true
};
return new Promise((res, rej)=>{
html2canvas(shareContent, opts).then((canvas) => {
let dom = document.body.appendChild(canvas);
dom.style.display = 'none';
a.style.display = 'none';
document.body.removeChild(dom);
let blob:Blob = dataURLToBlob(dom.toDataURL('image/png'));
a.setAttribute('href', URL.createObjectURL(blob));
a.setAttribute('download', name + '.png');
document.body.appendChild(a);
a.click();
URL.revokeObjectURL(blob);
document.body.removeChild(a);
res();
});
});
}
function dataURLToBlob(dataurl:string) {
var arr = dataurl.split(',');
var mime = arr[0].match(/:(.*?);/)[1];
var bstr = atob(arr[1]); var n = bstr.length; var u8arr = new Uint8Array(n);
while (n--) {
u8arr[n] = bstr.charCodeAt(n);
}
return new Blob([u8arr], {type: mime});
}
export function downloadPdf(excelUrl:string, reportName:string, callback:Function) {
fetch(excelUrl, {
headers: {
}
}).then(res => {
console.log(res.status);
if (res.status == 200) {
return res.blob().then(blob => {
var filename = `${reportName}.pdf`;
if (window.navigator.msSaveOrOpenBlob) {
navigator.msSaveBlob(blob, filename);
} else {
var a = document.createElement('a');
document.body.appendChild(a);
var url = window.URL.createObjectURL(blob);
a.href = url;
a.download = filename;
a.target = '_blank';
a.click();
a.remove();
window.URL.revokeObjectURL(url);
callback && callback();
}
});
} else {
return Promise.reject('服务器错误');
}
}).catch((e)=>{
callback && callback(e);
});
};
function exportDataPdf(el: HTMLElement, fileName: string) {
html2canvas(el, {
scale: 3,
useCORS: true,
allowTaint: true,
logging: false,
backgroundColor: '#ffffff'
}).then((canvas) => {
const contentWidth = canvas.width;
const contentHeight = canvas.height;
const pageHeight = (contentWidth / 592.28) * 841.89;
let leftHeight = contentHeight;
let position = 0;
const imgWidth = 595.28;
const imgHeight = (595.28 / contentWidth) * contentHeight;
const ctx = canvas.getContext('2d') as CanvasRenderingContext2D;
ctx.font = '20px Microsoft Yahei';
const pageData = canvas.toDataURL('image/jpeg', 1.0);
const pdf = new jsPDF("p", "pt", "a4");
if (leftHeight < pageHeight) {
pdf.addImage(pageData, 'JPEG', 0, 0, imgWidth, imgHeight);
} else {
while (leftHeight > 0) {
pdf.addImage(pageData, 'JPEG', 0, position, imgWidth, imgHeight);
leftHeight -= pageHeight;
position -= 841.89;
if (leftHeight > 0) {
pdf.addPage();
}
}
}
pdf.save(`${fileName}.pdf`);
})
}
let $utils = {
downPicture,
downloadPdf,
exportDataPdf
};
function getUtils() {
return $utils;
}
export { getUtils };
main.ts
import { createApp } from 'vue'
import { createPinia } from 'pinia'
import App from './App.vue'
import router from './router'
import { getUtils } from "./utils/index"
const app = createApp(App)
console.log('getUtils',getUtils);
app.config.globalProperties.$utils = getUtils()
app.use(router)
app.use(store)
app.mount('#app')
about.vue
<template>
<div ref="myDom" class="my-dom">
AboutIndex
我是{{ name1 }}
我是测试图片的
<img src="./img/test.png" alt="">
<van-button type="primary" @click="picDownload">下载图片</van-button>
<van-button type="primary" @click="pdfDownload">下载pdf</van-button>
</div>
</template>
<script setup lang="ts" name='AboutIndex'>
import { getCurrentInstance,ref } from "vue"
const name1 = ref<String>('ppp')
const { proxy } = getCurrentInstance()
console.log('proxy',proxy, proxy.$utils);
const myDom = ref(null)
const picDownload = async () => {
await proxy.$utils.downPicture( myDom.value,'pic图片啊');
}
const pdfDownload = async () => {
await proxy.$utils.exportDataPdf( myDom.value,'pdf文件啊');
}
</script>
<style scoped>
.my-dom {
padding: 20px;
}
</style>
```
## 效果
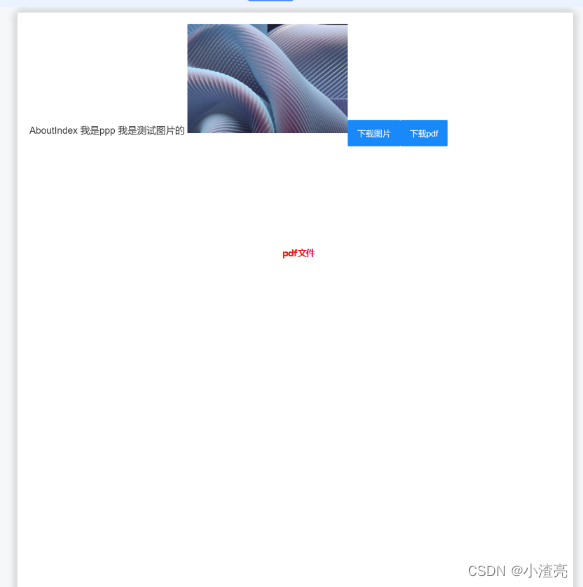
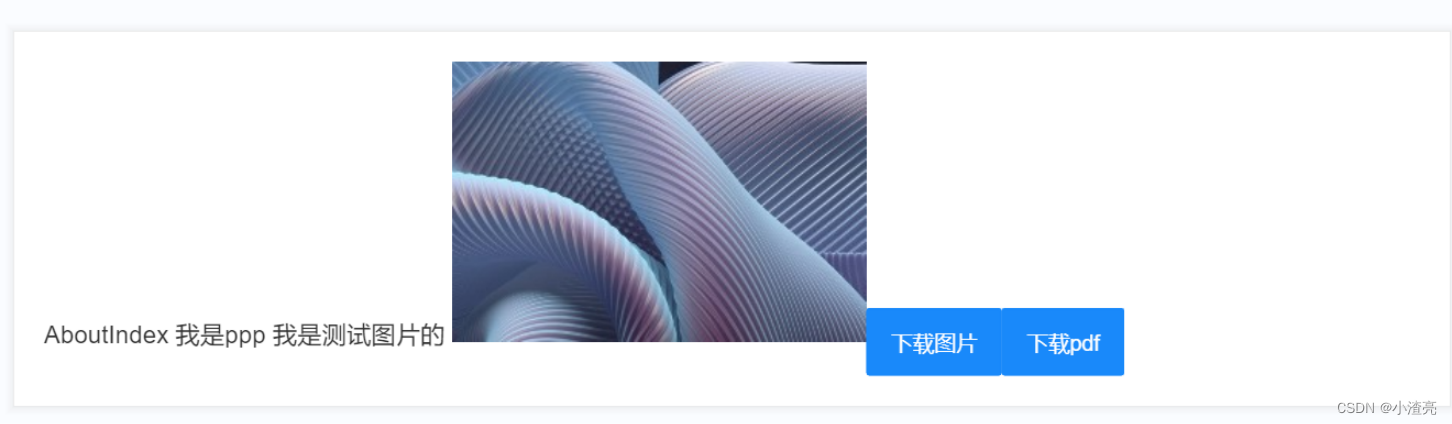