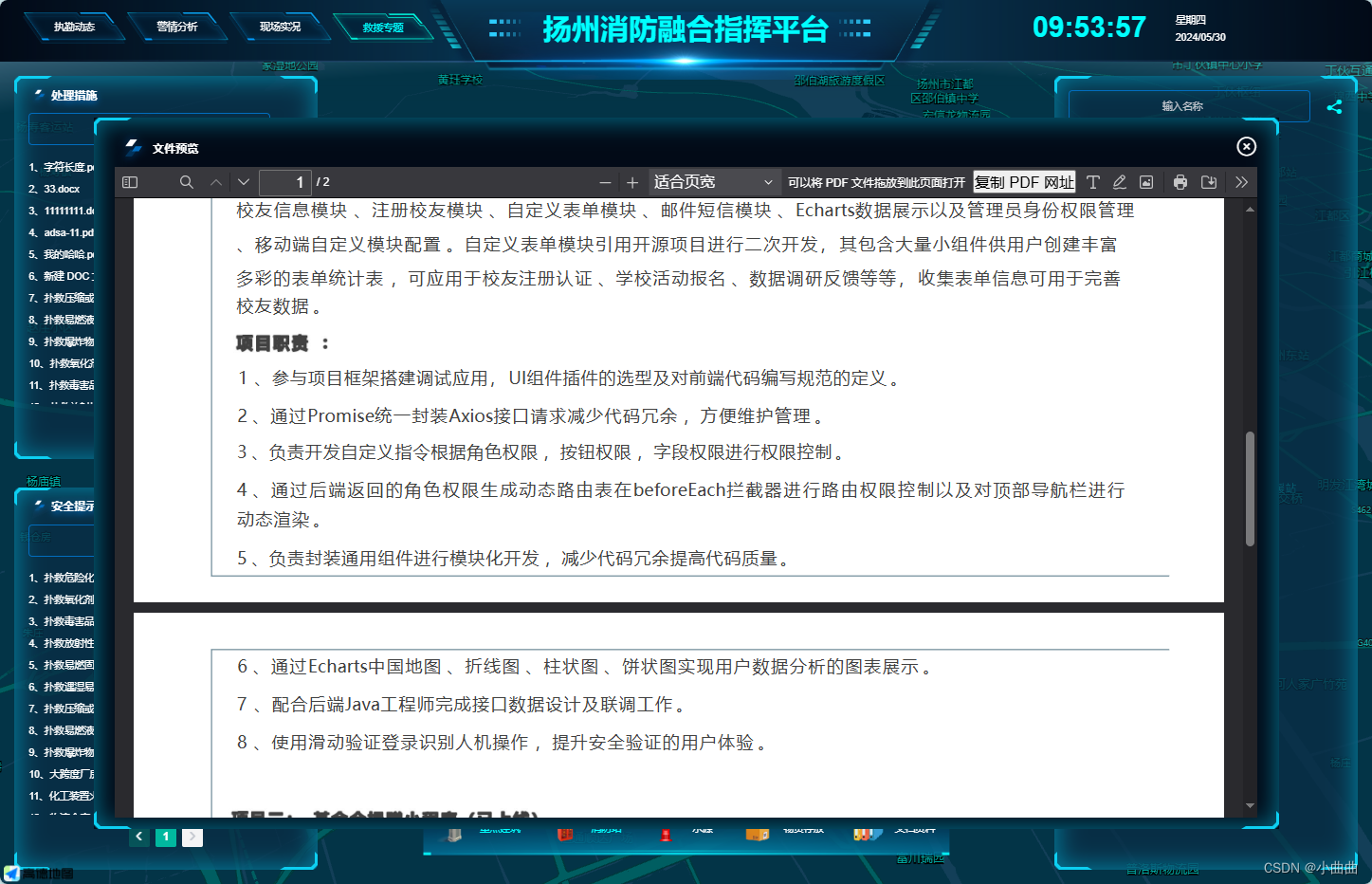
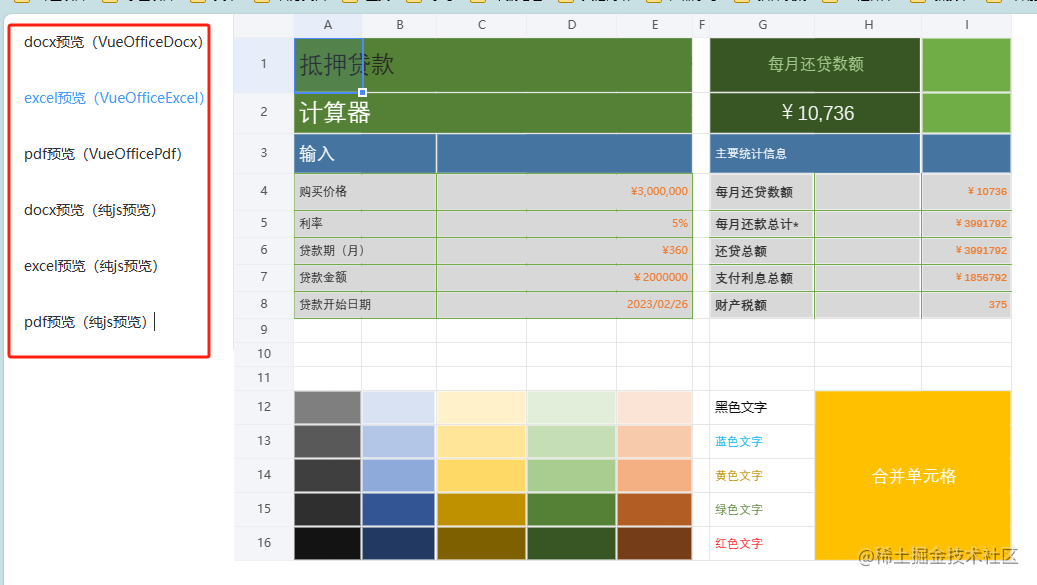
bug
- bug:pdf预览会出现后面空白页,建议使用 iframe标签直接显示pdf
- dwg格式(CAD)可以转化为图片格式预览
word
npm install @vue-office/docx
npm install @vue-office/excel
npm install @vue-office/pdf
<template>
<div>
<vue-office-docx
:src="docx"
style="height: 100vh"
@rendered="renderedHandler"
@error="errorHandler"
/>
</div>
</template>
<script>
import VueOfficeDocx from "@vue-office/docx";
console.log(VueOfficeDocx);
import "@vue-office/docx/lib/index.css";
export default {
name: "VueOfficeDocxDemo",
components: {
VueOfficeDocx,
},
data() {
return {
docx: "http://static.shanhuxueyuan.com/test.docx",
};
},
methods: {
renderedHandler() {
console.log("渲染完成");
},
errorHandler() {
console.log("渲染失败");
},
},
};
</script>
<style scoped>
</style>
pdf
<template>
<vue-office-pdf
:src="pdf"
@rendered="renderedHandler"
@error="errorHandler"
/>
</template>
<script>
import VueOfficePdf from '@vue-office/pdf'
export default {
name: "VueOfficePdfDemo",
components: {
VueOfficePdf
},
data() {
return {
pdf: 'http://static.shanhuxueyuan.com/test.pdf'
}
},
methods: {
renderedHandler() {
console.log("渲染完成")
},
errorHandler() {
console.log("渲染失败")
}
}
};
</script>
<style scoped>
</style>
excel
<template>
<div>
<vue-office-excel
:src="excel"
:options="options"
style="height: 100vh;"
@rendered="renderedHandler"
@error="errorHandler"
/>
</div>
</template>
<script>
import VueOfficeExcel from '@vue-office/excel'
import '@vue-office/excel/lib/index.css'
export default {
name: "VueOfficeExcelDemo",
components: {
VueOfficeExcel
},
data() {
return {
options:{
xls: false,
minColLength: 0,
minRowLength: 0,
widthOffset: 10,
heightOffset: 10,
beforeTransformData: (workbookData) => {return workbookData},
transformData: (workbookData) => {return workbookData},
},
excel: 'http://static.shanhuxueyuan.com/test.xlsx'
}
},
methods: {
renderedHandler() {
console.log("渲染完成")
},
errorHandler() {
console.log("渲染失败")
}
}
};
</script>
<style scoped>
</style>
最终预览组件
<template>
<div class="w100" v-if="show">
<dv-border-box-10
:color="['rgba(2, 208, 249,.5)', '#0dcff2']"
class="w100 h100"
>
<div class="w100 h100 plrRem-30 ptbRem-16 flex flex-column">
<div class="flex1">
<div class="flex_l sizeRem-16 bold">
<img
src="../components/img/index1-title.png"
alt=""
style="width: 40px"
/>
文件预览
</div>
<i class="el-icon-circle-close sizeRem-30" @click="show = false"></i>
</div>
<div class="scroll flex-1">
<vue-office-docx
v-if="type == 1"
:src="url"
class="h100"
@rendered="renderedHandler"
@error="errorHandler"
/>
<!-- <vue-office-pdf
v-if="type == 2"
:src="url"
@rendered="renderedHandler"
@error="errorHandler"
/> -->
<iframe
v-if="type == 2"
:src="url + '#view=FitH,top'"
frameborder="0"
style="width: 100%; height: 100%; overflow-y: scroll"
></iframe>
<vue-office-excel
v-if="type == 3"
:src="url"
:options="options"
style="height: 100vh"
@rendered="renderedHandler"
@error="errorHandler"
/>
<el-image
v-if="type == 4"
class="w100 h100"
:src="url"
fit="contain"
:preview-src-list="[url]"
></el-image>
</div>
</div>
</dv-border-box-10>
</div>
</template>
<script>
import VueOfficeDocx from "@vue-office/docx";
import "@vue-office/docx/lib/index.css";
import VueOfficeExcel from "@vue-office/excel";
import "@vue-office/excel/lib/index.css";
export default {
name: "VueOfficeDocxDemo",
components: {
VueOfficeDocx,
VueOfficeExcel,
},
data() {
return {
type: null,
show: false,
url: "",
docx: "http://static.shanhuxueyuan.com/test.docx",
pdf: "http://static.shanhuxueyuan.com/test.pdf",
options: {
xls: false,
minColLength: 0,
minRowLength: 0,
widthOffset: 10,
heightOffset: 10,
beforeTransformData: (workbookData) => {
return workbookData;
},
transformData: (workbookData) => {
return workbookData;
},
},
excel: "http://static.shanhuxueyuan.com/test.xlsx",
};
},
mounted() {},
beforeDestroy() {},
methods: {
init(url, solo) {
if (!solo) {
this.url =
(location.protocol.includes("https")
? location.origin
: "http://192.168.0.19:12020") + url;
} else {
this.url = url;
}
let type = url.split(".")[url.split(".").length - 1].split("?")[0];
console.log(type, 666, this.url, location.origin, location.protocol);
this.show = true;
if (["doc", "docx"].includes(type)) {
this.type = 1;
} else if (["pdf"].includes(type)) {
this.type = 2;
} else if (["xls", "xlsx"].includes(type)) {
this.type = 3;
this.options.xls = type == "xls" ? true : false;
} else {
this.type = 4;
}
},
renderedHandler() {
console.log("渲染完成");
},
errorHandler() {
this.$message({
message: "渲染失败,请检查文件是否有误",
type: "error",
customClass: "messageIndex",
});
},
close() {
this.show = false;
},
},
};
</script>
<style lang="scss" scoped>
@import "../components/css/rem.scss";
</style>