1.Spring集成web环境
1.1ApplicationContext应用上下文获取方式

- 创建listener包,并创建ContextLoaerListener实现ServletContextListener接口
public class ContextLoaderListener implements ServletContextListener {
@Override
public void contextInitialized(ServletContextEvent servletContextEvent) {
ApplicationContext app = new ClassPathXmlApplicationContext("applicationContext.xml");
//将Spring的应用上下文存储到ServletContext域当中
ServletContext servletContext = servletContextEvent.getServletContext();
servletContext.setAttribute("app",app);
}
@Override
public void contextDestroyed(ServletContextEvent servletContextEvent) {
}
}
- 在web.xml中配置监听器
<!DOCTYPE web-app PUBLIC
"-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN"
"http://java.sun.com/dtd/web-app_2_3.dtd" >
<web-app>
<display-name>Archetype Created Web Application</display-name>
<listener>
<listener-class>com.brove.listener.ContextLoaderListener</listener-class>
</listener>
<servlet>
<servlet-name>UserServlet</servlet-name>
<servlet-class>com.brove.web.UserServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>UserServlet</servlet-name>
<url-pattern>/userServlet</url-pattern>
</servlet-mapping>
</web-app>
- 修改servlet代码
public class UserServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
ServletContext servletContext = req.getServletContext();
ApplicationContext app = (ApplicationContext) servletContext.getAttribute("app");
UserService userService = app.getBean(UserService.class);
userService.save();
}
}
1.2利用配置文件将Spring配置文件名字与创建Spring容器代码解耦等优化
下列代码中Spring容器配置文件applicationContext.xml可以为任何名字,后面的开发中可能会更改,所以我们可以利用web.xml配置文件将其与代码解耦,这样后期只需要修改配置文件即可。
public void contextInitialized(ServletContextEvent servletContextEvent) {
ApplicationContext app = new ClassPathXmlApplicationContext("applicationContext.xml");
//将Spring的应用上下文存储到ServletContext域当中
ServletContext servletContext = servletContextEvent.getServletContext();
servletContext.setAttribute("app",app);
}
- web.xml文件中配置初始化参数
<context-param>
<param-name>applicationcontext</param-name>
<param-value>applicationContext.xml</param-value>
</context-param>
- 修改Listener代码
public class ContextLoaderListener implements ServletContextListener {
@Override
public void contextInitialized(ServletContextEvent servletContextEvent) {
ServletContext servletContext = servletContextEvent.getServletContext();
//读取servletContext全局参数
String applicationcontext = servletContext.getInitParameter("applicationcontext");
ApplicationContext app = new ClassPathXmlApplicationContext(applicationcontext);
//将Spring的应用上下文存储到ServletContext域当中
servletContext.setAttribute("app",app);
}
@Override
public void contextDestroyed(ServletContextEvent servletContextEvent) {
}
}
- 创建WebApplicationContextUtils获取spring容器让容器的名字不再出现在servlet代码中
public class WebApplicationContextUtils {
public static ApplicationContext getWebApplicationContext(ServletContext servletContext){
return (ApplicationContext) servletContext.getAttribute("app");
}
}
- servlet修改后的代码
public class UserServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
ServletContext servletContext = req.getServletContext();
// ApplicationContext app = (ApplicationContext) servletContext.getAttribute("app");
ApplicationContext app = WebApplicationContextUtils.getWebApplicationContext(servletContext);
UserService userService = app.getBean(UserService.class);
userService.save();
}
}
1.3Spring提供获取应用上下文的工具(相当于1.2优化的封装)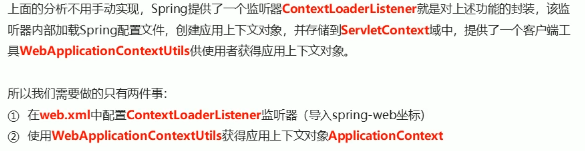
- 导入Spring继承web的坐标
- 配置ContextLoaderListener监听器
<!--配置监听器-->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
- 配置初始化参数
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:applicationContext.xml</param-value>
</context-param>
- 使用Spring提供的工具获得应用上下文
public class UserServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
ServletContext servletContext = req.getServletContext();
// ApplicationContext app = (ApplicationContext) servletContext.getAttribute("app");
// ApplicationContext app = WebApplicationContextUtils.getWebApplicationContext(servletContext);
WebApplicationContext app = WebApplicationContextUtils.getWebApplicationContext(servletContext);
UserService userService = app.getBean(UserService.class);
userService.save();
}
}
注意:这个地方的参数名字只能只能叫contextConfigLocation,因为已经写好的类中就叫这个名字,如果找不到这个名字对应的参数,应用程序会到WEB-INF目录下寻找
第一步创建项目
第二步完善目录结构
第三步添加依赖坐标
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.0.5.RELEASE</version>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.0.1</version>
</dependency>
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>javax.servlet.jsp-api</artifactId>
<version>2.2.1</version>
<scope>provided</scope>
</dependency>
第四步创建servlet对象
public class UserServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
ApplicationContext app = new ClassPathXmlApplicationContext("applicationContext.xml");
UserService userService = app.getBean(UserServiceImpl.class);
userService.save();
}
}
第五步在web.xml文件中配置servlet
<servlet>
<servlet-name>UserServlet</servlet-name>
<servlet-class>com.brove.web.UserServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>UserServlet</servlet-name>
<url-pattern>/userServlet</url-pattern>
</servlet-mapping>
第六步配置Tomcat,发布项目