温故而知新,可以为师矣
本文为拉钩教育java高薪培训班学习笔记与总结
1、Dubbo 架构概述
1.1 什么是Dubbo
Apache Dubbo
是一款高性能的
Java RPC框架。其前身是阿里巴巴公司开源的一个高性能、轻量级的开源
Java RPC
框架,可以和
Spring
框架无缝集成。
1.2 dubbo 的特性
参考官网首页
特性一览
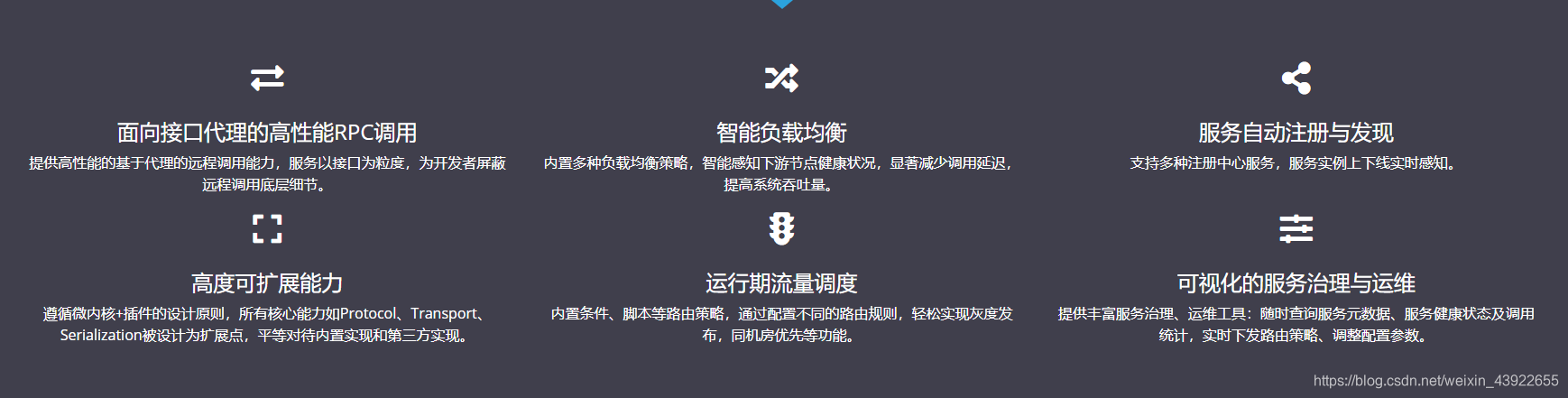
1.3 Dubbo 的服务治理
服务治理(
SOA governance),企业为了确保项目顺利完成而实施的过程,包括最佳实践、架构原则、治理规程、规律以及其他决定性的因素。服务治理指的是用来管理
SOA
的采用和实现的过程。
参考官网
服务治理
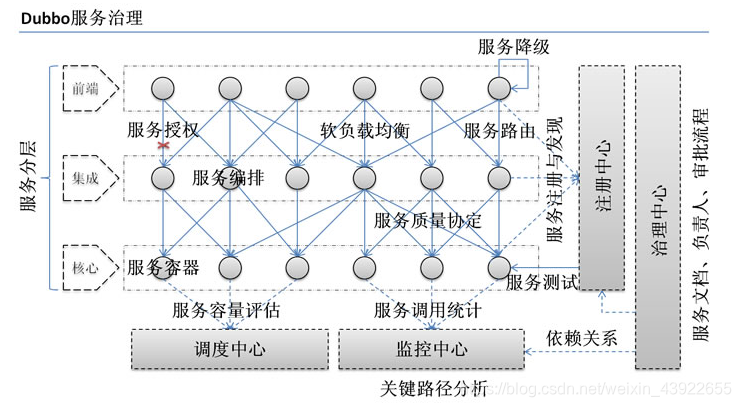
2. Dubbo 处理流程
参考官网
处理流程
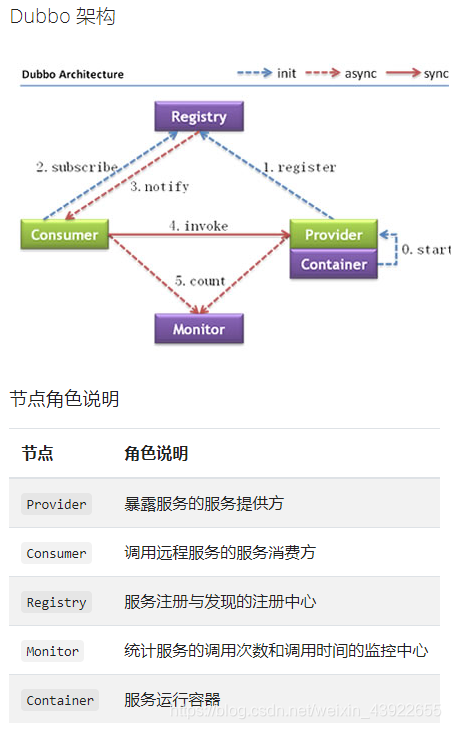
调用关系说明
虚线 代表异步调用
实线代表同步访问
蓝色虚线
是在启动时完成的功能
红色虚线
是程序运行中执行的功能
- 服务容器负责启动,加载,运行服务提供者。
- 服务提供者在启动时,向注册中心注册自己提供的服务。
- 服务消费者在启动时,向注册中心订阅自己所需的服务。
- 注册中心返回服务提供者地址列表给消费者,如果有变更,注册中心将基于长连接推送变更数据给消费者。
- 服务消费者,从提供者地址列表中,基于软负载均衡算法,选一台提供者进行调用,如果调用失败,再选另一台调用。
- 服务消费者和提供者,在内存中累计调用次数和调用时间,定时每分钟发送一次统计数据到监控中心。
Dubbo 架构具有以下几个特点,分别是连通性、健壮性、伸缩性、以及向未来架构的升级性。
3、服务注册中心Zookeeper
通过前面的Dubbo架构图可以看到,Registry(服务注册中心)在其中起着至关重要的作用。Dubbo官方推荐使用Zookeeper作为服务注册中心。Zookeeper 是 Apache Hadoop 的子项目,作为 Dubbo 服务的注册中心,工业强度较高,可用于生产环境,并推荐使用。
4、Dubbo开发实战
4.1 实战案例介绍分析
在
Dubbo
中所有的的服务调用都是基于接口去进行双方交互的。双方协定好
Dubbo调用中的接口,提供者来提供实现类并且注册到注册中心上。
调用方则只需要引入该接口,并且同样注册到相同的注册中心上
(
消费者
)。即可利用注册中心来实现集群感知功能,之后消费者即可对提供者进行调用。
我们所有的项目都是基于
Maven去进行创建,这样相互在引用的时候只需要以依赖的形式进行展现就可以了。
并且这里我们会通过
maven
的父工程来统一依赖的版本。
程序实现分为以下几步骤
:
1.
建立
maven
工程 并且 创建
API
模块
:
用于规范双方接口协定
2.
提供
provider
模块,引入
API模块,并且对其中的服务进行实现。将其注册到注册中心上,对外来统一提供服务。
3.
提供
consumer
模块,引入
API
模块,并且引入与提供者相同的注册中心。再进行服务调用。
4.2 代码
API接口定义
1.定义maven
<groupId>com.lagou</groupId>
<artifactId>service-api</artifactId>
<version>1.0-SNAPSHOT</version>
2.定义接口
public interface HelloService {
String sayHello(String name);
}
创建接口提供者
1.引入API模块
<dependency>
<groupId>com.lagou</groupId>
<artifactId>service-api</artifactId>
<version>${project.version}</version>
</dependency>
2.引入Dubbo相关依赖
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo</artifactId>
</dependency>
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo-registry-zookeeper</artifactId>
</dependency>
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo-rpc-dubbo</artifactId>
</dependency>
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo-remoting-netty4</artifactId>
</dependency>
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo-serialization-hessian2</artifactId>
</dependency>
3.编写实现类。注意这里使用了Dubbo中的 @Service 注解来声明他是一个服务的提供者。
@Service
public class HelloServiceImpl implements HelloService {
@Override
public String sayHello(String name) {
return "hello: " + name;
}
}
4.编写配置文件,用于配置dubbo。比如这里我就叫 dubbo-provider.properties ,放入到 resources 目录下。
dubbo.application.name=dubbo-demo-annotation-provider
dubbo.protocol.name=dubbo
dubbo.protocol.port=20880
- dubbo.application.name: 当前提供者的名称
- dubbo.protocol.name: 对外提供的时候使用的协议
- dubbo.protocol.port: 该服务对外暴露的端口是什么,在消费者使用时,则会使用这个端口,并且使用指定的协议与提供者建立连接。
5.
编写启动的
main 函数。这里面做的比较简单,主要要注意注解方式中的注册中心这里是使用的本机
2181
端口来作为注册中心
public class DubboPureMain {
public static void main(String[] args) throws Exception {
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(ProviderConfiguration.class);
context.start();
System.in.read();
}
@Configuration
@EnableDubbo(scanBasePackages = "com.lagou.service.impl")
@PropertySource("classpath:/dubbo-provider.properties")
static class ProviderConfiguration {
@Bean
public RegistryConfig registryConfig() {
RegistryConfig registryConfig = new RegistryConfig();
registryConfig.setAddress("zookeeper://127.0.0.1:2181");
return registryConfig;
}
}
}
创建消费者
1.引入API模块,同服务提供者。
2.引入Dubbo相关依赖,同服务提供者。
3. 编写服务,用于真实的引用dubbo接口并使用。因为这里是示例,所以比较简单一些。这里面@Reference 中所指向的就是真实的第三方服务接口。
@Component
public class ConsumerComponent {
@Reference
private HelloService helloService;
public String sayHello(String name) {
return helloService.sayHello(name);
}
}
4. 编写消费者的配置文件。这里比较简单,主要就是指定了当前消费者的名称和注册中心的位置。通过这个注册中心地址,消费者就会注册到这里并且也可以根据这个注册中心找到真正的提供者列表。
dubbo.application.name=service-consumer
dubbo.registry.address=zookeeper://127.0.0.1:2181
5.
编写启动类,这其中就会当用户在控制台输入了一次换行后,则会发起一次请求。
public class AnnotationConsumerMain {
public static void main(String[] args) throws IOException, InterruptedException {
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(ConsumerConfiguration.class);
context.start();
ConsumerComponent service = context.getBean(ConsumerComponent.class);
while (true) {
System.in.read();
try {
String hello = service.sayHello("world");
System.out.println("result :" + hello);
} catch (Exception e) {
e.printStackTrace();
}
}
}
@Configuration
@EnableDubbo(scanBasePackages = "com.lagou.service")
@PropertySource("classpath:/dubbo-consumer.properties")
@ComponentScan(value = {"com.lagou.bean.consumer"})
static class ConsumerConfiguration {
}
}