144 - 589 - 429 - 174。
144. 二叉树的前序遍历
// 21.20
// 21.23
class Solution {
public:
vector<int> preorderTraversal(TreeNode* root) {
if(root == nullptr) return {};
stack<TreeNode*> stk;
vector<int> ans;
stk.push(root);
while(!stk.empty()) {
TreeNode* tmp = stk.top(); stk.pop();
ans.push_back(tmp->val);
if(tmp->right) stk.push(tmp->right);
if(tmp->left) stk.push(tmp->left);
}
return ans;
}
};
589. N叉树的前序遍历
// 21.04
// 21.27
class Solution {
vector<int> ans;
public:
vector<int> preorder(Node* root) {
if(root == nullptr) return {};
stack<Node*> stk;
vector<int> ans;
stk.push(root);
while(!stk.empty()) {
Node* tmp = stk.top(); stk.pop();
ans.push_back(tmp->val);
for(auto it = tmp->children.rbegin(); it != tmp->children.rend(); it++) {
stk.push(*it);
}
}
return ans;
}
};
429. N叉树的层序遍历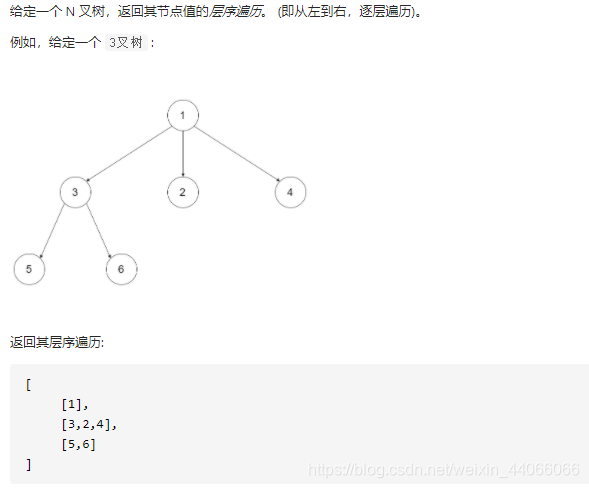
// 20.54
// 20.58
class Solution {
public:
vector<vector<int>> levelOrder(Node* root) {
if(root == nullptr) return {};
queue<Node*> que;
vector<vector<int>> ans;
que.push(root);
while(!que.empty()) {
int size = que.size();
vector<int> tmp;
for(int i = 0; i < size; i++) {
Node* curr = que.front(); que.pop();
tmp.push_back(curr->val);
for(auto x : curr->children)
que.push(x);
}
ans.push_back(tmp);
}
return ans;
}
};
174. 地下城游戏
动规,与之前小机器人走方格很像
// 21.33
// 22.06
class Solution {
public:
int calculateMinimumHP(vector<vector<int>>& graph) {
if(graph.empty()) return 0;
int n = graph.size();
int m = graph[0].size();
graph[n - 1][m - 1] = (graph[n - 1][m - 1] < 0)? 1 - graph[n - 1][m - 1] : 1;
for(int i = n - 2; i >= 0; i--) {
int &curr = graph[i][m - 1];
int &next = graph[i + 1][m - 1];
if(curr <= 0) curr = next - curr;
else if(curr >= next) curr = 1;
else curr = next - curr;
}
for(int k = m - 2; k >= 0; k--) {
int &curr = graph[n - 1][k];
int &next = graph[n - 1][k + 1];
if(curr <= 0) curr = next - curr;
else if(curr >= next) curr = 1;
else curr = next - curr;
}
for(int i = n - 2; i >= 0; i--) {
for(int k = m - 2; k >= 0; k--) {
int next = min(graph[i + 1][k], graph[i][k + 1]);
int &curr = graph[i][k];
if(curr <= 0) curr = next - curr;
else if(curr >= next) curr = 1;
else curr = next - curr;
}
}
return graph[0][0];
}
};