原生JS写一个贪吃蛇小游戏
index.css
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<!--
分为几个部分: 地图 食物
蛇(初始 三个 )
-->
<button id="btn">开始游戏</button>
<script src="./js/index.js></script>
<script>
// new Map()
// new Food()
// const snake = new Snake()
// snake.move()
new Main()
</script>
</body>
</html>
index.js
function css(ele, attr, value) {
// value 传入了 是设置 没有就是获取
// console.log(typeof value === "undefined")
if (typeof value === "undefined") {
// 没有传入第三个参数 那就是获取
return window.getComputedStyle ? window.getComputedStyle(ele)[attr] : ele.currentStyle[attr]
}
// 设置
// ele.style[attr] = parseInt(value) + "px"
ele.style[attr] = value
}
class Food{
constructor(options){
options = options || {}
this.width = options.width || 20
this.height = options.height || 20
this.x = 0
this.y = 0
this.map = document.querySelector("#map")
this.init()
}
init(){
document.querySelector("#food") ? this.map.removeChild(document.querySelector("#food")) : ""
const dv = document.createElement("div")
dv.id = "food"
css(dv,"width",this.width + "px")
css(dv,"height",this.height + "px")
css(dv,"position","absolute")
css(dv,"backgroundColor",getColor())
this.x = getRandom(0,this.map.offsetWidth / this.width - 1)
this.y = getRandom(0,this.map.offsetHeight / this.height - 1)
// let left = getRandom(0,this.map.offsetWidth / this.width - 1) * this.width
// let top = getRandom(0,this.map.offsetHeight / this.height - 1) * this.height
css(dv,"left",this.x * this.width + "px")
css(dv,"top",this.y * this.height + "px")
this.map.appendChild(dv)
}
}
class Main {
constructor() {
this.map = new Map()
this.food = new Food()
this.snake = new Snake({}, this.food)
this.init()
}
init() {
document.querySelector("#btn").onclick = () => {
this.snake.move()
this.bindEvent()
}
}
bindEvent() {
document.onkeydown = (e) => {
// e.keyCode
switch (e.keyCode) {
case 37:
this.snake.direction != "right" && (this.snake.direction = "left")
break
case 38:
this.snake.direction != "down" && (this.snake.direction = "up")
break
case 39:
this.snake.direction != "left" && (this.snake.direction = "right")
break
case 40:
this.snake.direction != "up" && (this.snake.direction = "down")
break
}
}
}
}
class Map{
constructor(options){
options = options || {}
this.width = options.width || 800
this.height = options.height || 600
this.bgColor = options.color || "#333"
this.init()
}
init(){
const dv = document.createElement("div")
dv.id = "map"
css(dv,"width",this.width + "px")
css(dv,"height",this.height + "px")
css(dv,"position","relative")
css(dv,"backgroundColor",this.bgColor)
document.body.appendChild(dv)
}
}
class Snake {
constructor(options, food) {
options = options || {}
this.width = options.width || 20
this.height = options.height || 20
this.food = food
this.map = document.querySelector("#map")
this.direction = "right"
this.timer = null
this.body = [
{
x: 3,
y: 2,
ele: document.createElement("div"),
color: "#0f0"
}, {
x: 2,
y: 2,
ele: document.createElement("div"),
color: "pink"
}, {
x: 1,
y: 2,
ele: document.createElement("div"),
color: "pink"
}
]
this.init()
}
init() {
this.body.forEach(item => {
css(item.ele, "width", this.width + "px")
css(item.ele, "height", this.height + "px")
css(item.ele, "backgroundColor", item.color)
css(item.ele, "position", "absolute")
css(item.ele, "borderRadius", "50%")
css(item.ele, "left", item.x * this.width + "px")
css(item.ele, "top", item.y * this.height + "px")
this.map.appendChild(item.ele)
})
}
move() {
let head = this.body[0]
// let start = this.body.length - 1
this.timer = setInterval(() => {
let start = this.body.length - 1
// for(let i = 1 ; i < this.body.length ; i++){
// this.body[i].x = this.body[i - 1].x
// this.body[i].y = this.body[i - 1].y
// // this.body[i].ele.style.left = this.body[i].x * this.width + "px"
// // this.body[i].ele.style.top = this.body[i].y * this.height + "px"
// }
for (let i = start; i > 0; i--) {
this.body[i].x = this.body[i - 1].x
this.body[i].y = this.body[i - 1].y
this.body[i].ele.style.left = this.body[i].x * this.width + "px"
this.body[i].ele.style.top = this.body[i].y * this.height + "px"
}
// 根据运动的方向设置蛇头的位置
switch (this.direction) {
case "right":
head.x++
head.ele.style.left = head.x * this.width + "px"
break
case "left":
head.x--
head.ele.style.left = head.x * this.width + "px"
break
case "up":
head.y--
head.ele.style.top = head.y * this.height + "px"
break
case "down":
head.y++
head.ele.style.top = head.y * this.height + "px"
break
}
// 检测是否碰壁
if ((this.direction === "left" && head.x < 0) || (this.direction === "right" && head.x > Math.floor(this.map.offsetWidth / this.width) - 1) || (this.direction === "up" && head.y < 0) || (this.direction === "down" && head.y > Math.floor(this.map.offsetHeight / this.height) - 1)) {
alert("GAMEOVER")
clearInterval(this.timer)
}
// 检测是否吃到食物
if (head.x === this.food.x && head.y === this.food.y) {
// 吃到食物以后
/*
1、让食物重新生成
2、创建一个身体 (把数据加到 this.body 里面)
*/
this.food.init()
this.body.push({
x: this.body[this.body.length - 1].x,
y: this.body[this.body.length - 1].y,
ele: document.createElement("div"),
color: getColor()
})
this.init()
}
console.log(this.food)
}, 100);
}
}
效果截图
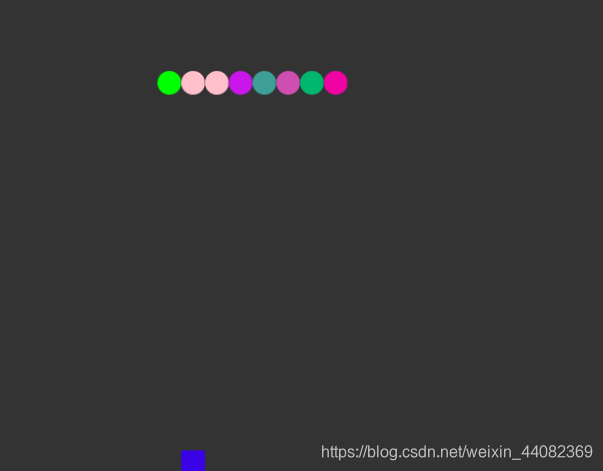