// HJ11 数字颠倒
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
System.out.println(new StringBuffer(String.valueOf(in.nextInt())).reverse().toString());
}
}
// HJ12 字符串反转
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
System.out.println(new StringBuffer(in.nextLine()).reverse().toString());
}
}
// HJ13 句子逆序
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
String line = in.nextLine();
String[] strs = line.split(" ");
StringBuffer sb = new StringBuffer();
for (int i = strs.length - 1; i >= 0; i--) {
sb.append(strs[i]);
if (i !=0 ) {
sb.append(" ");
}
}
System.out.println(sb.toString());
}
}
// HJ14 字符串排序
import java.util.ArrayList;
import java.util.Objects;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int num = in.nextInt();
String ee = in.nextLine();
ArrayList<String> list = new ArrayList<>();
for (int i = 0; i < num; i++) {
list.add(in.nextLine());
}
list.sort((o1, o2) -> {
int len = Math.min(o1.length(), o2.length());
for (int i = 0; i < len; i++) {
if (o1.charAt(i) > o2.charAt(i)) {
return 1;
}
if (o1.charAt(i) < o2.charAt(i)) {
return -1;
}
}
if (Objects.equals(o1.length(), o2.length())) {
return 0;
}
return o1.length() > o2.length() ? 1 : -1;
});
list.forEach(System.out::println);
}
}
// HJ15 求int型正整数在内存中存储时1的个数
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int num = in.nextInt();
int count = 0;
while (num != 0) {
int mod = num % 2;
if (mod == 1) {
count++;
}
num = num / 2;
}
System.out.println(count);
}
}
// HJ17 坐标移动
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
String line = in.nextLine();
String[] split = line.split(";");
List<String> list = Arrays.asList(split);
ArrayList<String> opratorList = new ArrayList<>();
for (String str : list) {
if (str.length() >= 2 && str.length() <= 3) {
char[] chars = str.toCharArray();
if (chars[0] == 'A' || chars[0] == 'D' || chars[0] == 'S' || chars[0] == 'W') {
try {
if (chars.length == 2) {
Integer.valueOf(chars[1]);
} else {
Integer.valueOf(chars[1] + "" + chars[2]);
}
opratorList.add(str);
} catch (Exception e) {
}
}
}
}
// A10: x-10
// D10: x+10
// W10: y+10
// S10: y-10
point start = new point(0, 0);
for (String op : opratorList) {
Integer num = Integer.valueOf(op.substring(1, op.length()));
if (op.startsWith("A")) {
start.setX(start.x - num);
}
if (op.startsWith("D")) {
start.setX(start.x + num);
}
if (op.startsWith("W")) {
start.setY(start.y + num);
}
if (op.startsWith("S")) {
start.setY(start.y - num);
}
}
System.out.println(start.getX() + "," + start.getY());
}
static class point {
private Integer x;
private Integer y;
public point(Integer x, Integer y) {
this.x = x;
this.y = y;
}
public Integer getX() {
return x;
}
public void setX(Integer x) {
this.x = x;
}
public Integer getY() {
return y;
}
public void setY(Integer y) {
this.y = y;
}
}
}
// HJ20 密码验证合格程序
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
while (in.hasNext()) {
String password = in.nextLine();
// 条件1
if (password.length() < 8) {
System.out.println("NG");
continue;
}
// 条件2
int[] flag = new int[4];
for (int i = 0; i < password.length(); i++) {
char c = password.charAt(i);
if ('0' <= c && c <= '9') {
flag[0] = 1;
} else if ('a' <= c && c <= 'z') {
flag[1] = 1;
} else if ('A' <= c && c <= 'Z') {
flag[2] = 1;
} else if (c != '\n' && c != ' ') {
flag[3] = 1;
}
}
if (Arrays.stream(flag).sum() < 3) {
System.out.println("NG");
continue;
}
// 条件3
if (isMatch(password)) {
System.out.println("NG");
continue;
}
System.out.println("OK");
}
}
public static boolean isMatch(String input) {
for (int i = 0; i < input.length() - 3; i++) {
// 将截取的子串与剩下的另半边子串查看包含关系
String sub = input.substring(i, i + 3);
String leftStr = input.substring(i + 3);
if (leftStr.contains(sub)) {
return true;
}
}
return false;
}
}
华为机试-HJ11~HJ20
最新推荐文章于 2025-01-20 17:54:49 发布
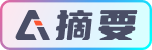