用堆栈的方式求组合数
#include <stdio.h>
#include<stdlib.h>
typedef struct
{
int *elem;
int max_size;
int top;
}SqStack;
void main()
{
void Creat_Stack(SqStack &S,int n);
void pop(SqStack &S,int &e);
void push(SqStack &S,int e);
int n,m,c;
printf("请输入n,m的值:");
scanf("%d %d",&n,&m);
SqStack S;
Creat_Stack(S,m);
while(m>=0)push(S,m--);
while(!(S.top==-1))
{
pop(S,m);
if(m==0)c=1;
else c=c*(n-m+1)/m;
}
printf("%2d和%2d的组合数结果为:%d\n",n,m,c);
}
void Creat_Stack(SqStack &S,int n)
{
S.max_size=n+1;
if(!(S.elem=(int *)malloc(sizeof(int)*S.max_size)))exit(-1);
S.top=-1;
}
void pop(SqStack &S,int &e)
{
if(S.top==-1)printf("ERROR!栈空!\n");
e=S.elem[S.top--];
}
void push(SqStack &S,int e)
{
if(S.top>=S.max_size-1)printf("ERROR!栈满!\n");
S.elem[++S.top]=e;
}
用堆栈的方式求两个数的最大公约数
#include <stdio.h>
#include<stdlib.h>
typedef struct
{
int *elem1;
int *elem2;
int max_size;
int top;
}SqStack;
void main()
{
void Creat_Stack(SqStack &S,int n);
void get_top(SqStack &S,int &a,int &b);
void push(SqStack &S,int a,int b);
SqStack S;
int a,b,a0,b0;
Creat_Stack(S,5);
printf("请输入a和b的值:");
scanf("%d%d",&a,&b);
a0=a;b0=b;
push(S,a,b);
while(1)
{
get_top(S,a,b);
if(b!=0)
push(S,b,a%b);
else break;
}
get_top(S,a,b);
printf("%2d和%2d的最大公约数为:%d\n",a0,b0,a);
}
void Creat_Stack(SqStack &S,int n)
{
S.max_size=n;
if(!(S.elem1=(int *)malloc(sizeof(int)*S.max_size)))exit(-1);
if(!(S.elem2=(int *)malloc(sizeof(int)*S.max_size)))exit(-1);
S.top=-1;
}
void get_top(SqStack &S,int &a,int &b)
{
if(S.top==-1)printf("ERROR!栈空!\n");
a=S.elem1[S.top];
b=S.elem2[S.top];
}
void push(SqStack &S,int a,int b)
{
if(S.top>=S.max_size-1)printf("ERROR!栈满!\n");
++S.top;
S.elem1[S.top]=a;
S.elem2[S.top]=b;
}
用堆栈的方式消去递归函数:
#include<stdio.h>
#include<stdlib.h>
typedef struct
{
int m;
int n;
}ElemTp;
typedef struct
{
ElemTp *elem;
int max_size;
int top;
}SqStack;
void main()
{
void Creat_Stack(SqStack &s,int n);
void pop(SqStack &s,ElemTp &e);
void push(SqStack &s,ElemTp e);
int m,n,y;
printf("请输入m,n的值:");
scanf("%d%d",&m,&n);
ElemTp e={m,n};
SqStack s;
Creat_Stack(s,m);
while(e.m>=0)
{
push(s,e);
e.m=e.m-1;e.n=2*e.n;
}
while(!(s.top==-1))
{
pop(s,e);
if(e.m==0&&e.n>=0)
y=0;
else
y=y+e.n;
}
printf("%d\n",y);
}
void Creat_Stack(SqStack &s,int n)
{
s.max_size=n+1;
if(!(s.elem=(ElemTp *)malloc(sizeof(ElemTp)*s.max_size)))exit(-1);
s.top=-1;
}
void pop(SqStack &s,ElemTp &e)
{
if(s.top==-1)printf("ERROR!栈空!\n");
e=s.elem[s.top--];
}
void push(SqStack &s,ElemTp e)
{
if(s.top>=s.max_size-1)printf("ERROR!栈满!\n");
s.elem[++s.top]=e;
}
用数组或递归消去递归函数: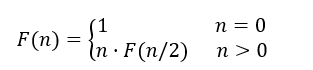
#include<stdio.h>
#include<stdlib.h>
void main()
{
int n;
int *p;
printf("请输入n的值:");
scanf("%d",&n);
p=(int *)malloc(sizeof(int)*(n+1));
for(int i=0;i<=n;i++)
{
if(i==0)p[i]=1;
else p[i]=i*p[i/2];
}
printf("函数的结果为:%d\n",p[n]);
}
#include<stdio.h>
#include<stdlib.h>
typedef struct
{
int *elem;
int max_size;
int top;
}SqStack;
void main()
{
void Creat_Stack(SqStack &s,int n);
void pop(SqStack &s,int &e);
void push(SqStack &s,int e);
int n,e,y;
SqStack s;
printf("请输入n的值:");
scanf("%d",&n);
Creat_Stack(s,n);
while(n>0)
{
push(s,n);
n=n/2;
}
push(s,0);
while(s.top!=-1)
{
pop(s,e);
if(e==0)y=1;
else y=y*e;
}
printf("函数的结果为:%d\n",y);
}
void Creat_Stack(SqStack &s,int n)
{
s.max_size=n+1;
if(!(s.elem=(int *)malloc(sizeof(int)*s.max_size)))exit(-1);
s.top=-1;
}
void pop(SqStack &s,int &e)
{
if(s.top==-1)printf("ERROR!栈空!\n");
e=s.elem[s.top--];
}
void push(SqStack &s,int e)
{
if(s.top>=s.max_size-1)printf("ERROR!栈满!\n");
s.elem[++s.top]=e;
}