播放音频
Android中播放音频文件一般都是使用MediaPlayer类来实现的
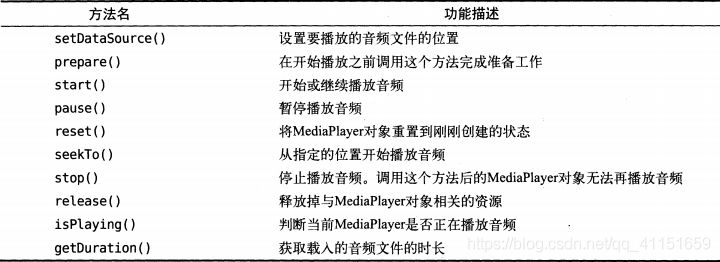
梳理一下MediaPlayer的工作流程。首先需要创建岀一个MediaPlayer对象,然后调用setDataSource()方法来设置音频文件的路径,再调用 prepare()方法使MediaPlayer进入到准备状态,接下来调用start()方法就可以开始播放音频,调用pause()方法就会暂停播放,调用reset()方法就会停止播放。
布局按钮:
<Button
android:id="@+id/play"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="播放"/>
<Button
android:id="@+id/pause"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="暂停"/>
<Button
android:id="@+id/stop"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="停止"/>
主活动添加一个变量
//音频
private MediaPlayer mediaPlayer = new MediaPlayer();
主活动:
//播放音频
//先获取权限
if(ContextCompat.checkSelfPermission(EightActivity.this, Manifest.permission.WRITE_EXTERNAL_STORAGE) != PackageManager.PERMISSION_GRANTED){
ActivityCompat.requestPermissions(EightActivity.this,new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE},1);
}else{
initMediaPlayer();
}
Button play = (Button)findViewById(R.id.play);
Button pause = (Button)findViewById(R.id.pause);
Button stop = (Button)findViewById(R.id.stop);
play.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if(!mediaPlayer.isPlaying()){
mediaPlayer.start();
}
}
});
pause.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if(mediaPlayer.isPlaying()){
mediaPlayer.pause();
}
}
});
stop.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (mediaPlayer.isPlaying()){
mediaPlayer.reset();
initMediaPlayer();
}
}
});
}
private void initMediaPlayer(){
try {
File file = new File(Environment.getExternalStorageDirectory().getAbsolutePath() +"/SystemDatabase/bgsounds/","麦兜旁白.mp3");
Log.i("音乐文件路径", file.getPath());
mediaPlayer.setDataSource(file.getPath());
mediaPlayer.prepare();
}catch (Exception e){
e.printStackTrace();
}
}
//权限
@Override
public void onRequestPermissionsResult(int requestCode,String[] permissions,int[] grantResults){
switch (requestCode){
case 1:
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED){
initMediaPlayer();
}else {
Toast.makeText(this,"拒绝授权将无法使用程序",Toast.LENGTH_SHORT).show();
finish();
}
break;
default:
}
}
@Override
protected void onDestroy(){
super.onDestroy();
if (mediaPlayer != null){
mediaPlayer.stop();
mediaPlayer.release();
}
}
记得权限:
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" /> <!--访问SD卡-->
播放视频
使用VideoView类来实现的
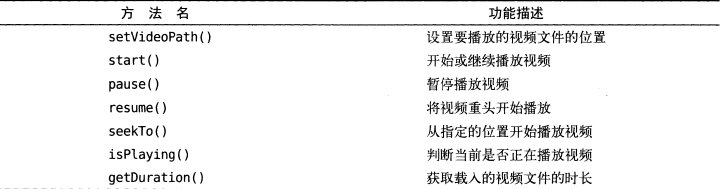
布局
<Button
android:id="@+id/playV"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="播放视频"/>
<Button
android:id="@+id/pauseV"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="暂停视频"/>
<Button
android:id="@+id/replayV"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="重放视频"/>
<VideoView
android:id="@+id/video_view"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
主活动:
public class EightVideoActivity extends AppCompatActivity implements View.OnClickListener{
private VideoView videoView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_eight_video);
videoView = (VideoView)findViewById(R.id.video_view);
Button play = (Button)findViewById(R.id.play);
Button pause = (Button)findViewById(R.id.pause);
Button replay = (Button)findViewById(R.id.replay);
play.setOnClickListener(this);
pause.setOnClickListener(this);
replay.setOnClickListener(this);
if(ContextCompat.checkSelfPermission(EightVideoActivity.this, Manifest.permission.WRITE_EXTERNAL_STORAGE) != PackageManager.PERMISSION_GRANTED){
ActivityCompat.requestPermissions(EightVideoActivity.this,new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE},1);
}else{
initVideopath();
}
}
private void initVideopath(){
File file = new File(Environment.getExternalStorageDirectory() + "/tencent/QQfile_recv/","love.mp4");
videoView.setVideoPath(file.getPath());
}
@Override
public void onRequestPermissionsResult(int requestCode,String[] permissions,int[] grantResults){
switch (requestCode){
case 1:
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED){
initVideopath();
}else{
Toast.makeText(this,"拒绝权限将无法使用程序",Toast.LENGTH_SHORT).show();
finish();
}
break;
default:
}
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.play:
if (!videoView.isPlaying()) {
videoView.start();
}
break;
case R.id.pause:
if (videoView.isPlaying()) {
videoView.pause();
}
break;
case R.id.replay:
if (videoView.isPlaying()) {
videoView.resume();
}
break;
}
}
@Override
protected void onDestroy(){
super.onDestroy();
if (videoView != null){
videoView.suspend();
}
}
}